What to Do When the Problem Seems Overwhelming: Breaking It Down Into Manageable Parts
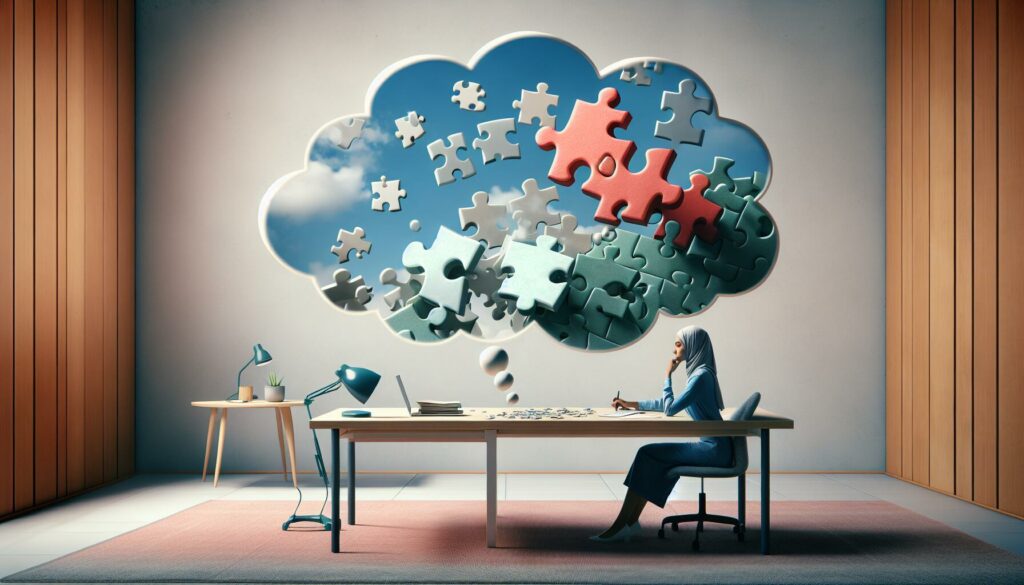
As aspiring programmers and coding enthusiasts, we often encounter complex problems that can seem insurmountable at first glance. Whether you’re tackling a challenging algorithm, designing a complex system, or preparing for a technical interview with a major tech company, the sheer scale of the task can be daunting. However, one of the most valuable skills you can develop is the ability to break down these overwhelming problems into smaller, more manageable parts. This approach not only makes the problem-solving process more achievable but also aligns perfectly with the principles taught by platforms like AlgoCademy, which focuses on developing strong algorithmic thinking and practical coding skills.
Understanding the Importance of Problem Decomposition
Problem decomposition is a fundamental concept in computer science and programming. It involves taking a large, complex problem and breaking it down into smaller, more manageable sub-problems. This technique is crucial for several reasons:
- It makes the problem less intimidating and more approachable.
- It allows you to focus on solving one part of the problem at a time.
- It helps in identifying patterns and reusable components.
- It facilitates better organization and planning of your code.
- It makes debugging and testing easier.
Let’s dive into the steps you can take to effectively break down overwhelming problems and tackle them with confidence.
Step 1: Understand the Problem
Before you can break down a problem, you need to fully understand what it’s asking. This step is crucial and often overlooked. Here’s how to approach it:
- Read the problem statement carefully: Make sure you understand every word and phrase.
- Identify the inputs and outputs: What information are you given, and what do you need to produce?
- Consider constraints: Are there any limitations or special conditions you need to account for?
- Ask questions: If anything is unclear, don’t hesitate to seek clarification. In a real-world scenario or during an interview, asking thoughtful questions demonstrates your analytical skills.
For example, let’s say you’re given a problem to implement a search functionality for a large dataset. Your first step would be to clearly define what type of search is required (e.g., exact match, partial match, fuzzy search), what kind of data you’re searching through, and what the expected output should look like.
Step 2: Visualize the Problem
Once you understand the problem, try to visualize it. This can help you see the big picture and identify potential sub-problems. Some techniques for visualization include:
- Flowcharts: Diagram the flow of data or processes.
- Pseudocode: Write out the logic in plain language.
- Diagrams: Sketch out data structures or system components.
- Examples: Work through small, concrete examples of the problem.
For our search functionality problem, you might create a flowchart showing the steps from user input to displaying search results, or diagram the data structure you’ll use to store and retrieve the searchable data.
Step 3: Identify Sub-Problems
Now that you have a clear understanding and visualization of the problem, start breaking it down into smaller, more manageable sub-problems. Look for natural divisions in the problem or steps in the process. For our search functionality example, some sub-problems might include:
- Designing the data structure to store the searchable data
- Implementing the search algorithm
- Creating a user interface for input
- Formatting and displaying search results
- Optimizing for performance with large datasets
Each of these sub-problems is more manageable and can be tackled independently.
Step 4: Prioritize and Order Sub-Problems
Once you’ve identified the sub-problems, prioritize them based on importance and dependencies. Some questions to consider:
- Which sub-problems are critical to the core functionality?
- Are there any sub-problems that depend on others being solved first?
- Which sub-problems align with your current knowledge and skills?
- Are there any sub-problems that might require additional research or learning?
For our search functionality, you might prioritize designing the data structure and implementing the basic search algorithm before tackling optimization or user interface design.
Step 5: Solve Sub-Problems One at a Time
Now that you have a clear plan, start solving each sub-problem individually. This is where the power of breaking down the problem really shines. Instead of feeling overwhelmed by the entire task, you can focus on solving one manageable piece at a time.
For each sub-problem:
- Plan your approach: Decide on the algorithm or method you’ll use.
- Write pseudocode: Outline the logic before diving into actual coding.
- Implement the solution: Write the actual code for the sub-problem.
- Test thoroughly: Make sure your solution works correctly for various inputs.
- Refactor if necessary: Look for ways to improve your code’s efficiency or readability.
Let’s look at how you might approach the sub-problem of implementing a basic search algorithm:
def basic_search(data, query):
results = []
for item in data:
if query.lower() in item.lower():
results.append(item)
return results
This simple implementation solves the core problem of finding matching items. You can later improve upon this by implementing more advanced search techniques or optimizing for performance.
Step 6: Integrate Solutions
As you solve each sub-problem, start integrating the solutions back into the larger problem. This step-by-step integration allows you to build up your complete solution gradually, making it easier to catch and fix any issues along the way.
For our search functionality, you might integrate the basic search algorithm with the data structure you designed, then add the user interface components, and finally implement performance optimizations.
Step 7: Test the Complete Solution
Once you’ve integrated all the sub-solutions, it’s time to test your complete solution thoroughly. This includes:
- Testing with various inputs, including edge cases
- Verifying that all requirements are met
- Checking for any unexpected interactions between sub-components
- Performance testing, especially if dealing with large datasets
For our search functionality, you’d want to test with different types of queries, large and small datasets, and ensure that the results are accurate and returned in a timely manner.
Step 8: Reflect and Refine
After solving the problem, take some time to reflect on your approach:
- What worked well in your problem-solving process?
- Were there any sub-problems that were particularly challenging?
- Are there any parts of your solution that could be improved or optimized?
- What did you learn that you can apply to future problems?
This reflection process is crucial for continuous improvement and is a key aspect of developing strong problem-solving skills.
Applying These Techniques in Coding Interviews
The ability to break down complex problems is particularly valuable in coding interviews, especially for major tech companies often referred to as FAANG (Facebook/Meta, Amazon, Apple, Netflix, Google). These companies are known for presenting candidates with challenging algorithmic problems that can seem overwhelming at first glance.
When faced with such a problem in an interview:
- Take a moment to understand: Don’t rush to code immediately. Take the time to fully understand the problem and ask clarifying questions if needed.
- Think out loud: Verbalize your thought process as you break down the problem. This gives the interviewer insight into your problem-solving approach.
- Start with a high-level approach: Outline the main steps or components of your solution before diving into details.
- Tackle one part at a time: Implement and test your solution incrementally, starting with the core functionality.
- Be open to feedback: If the interviewer provides hints or suggestions, be receptive and incorporate them into your approach.
Remember, in many cases, the interviewer is more interested in your problem-solving process than in seeing a perfect, complete solution.
Leveraging Tools and Resources
As you develop your problem-solving skills, it’s valuable to leverage tools and resources that can support your learning journey. Platforms like AlgoCademy offer several features that align well with the process of breaking down complex problems:
- Interactive tutorials: These can help you practice breaking down problems in a structured environment.
- AI-powered assistance: When you’re stuck on a sub-problem, AI guidance can provide hints or alternative approaches without giving away the entire solution.
- Step-by-step guidance: This mirrors the process of solving sub-problems one at a time, reinforcing good problem-solving habits.
- Problem banks: Access to a variety of problems allows you to practice your skills on different types and complexities of challenges.
By consistently practicing with these tools and applying the technique of breaking down problems, you’ll gradually build the confidence and skills needed to tackle even the most daunting coding challenges.
Conclusion
Breaking down overwhelming problems into manageable parts is a crucial skill for any programmer or aspiring software engineer. It’s a technique that not only makes complex problems more approachable but also improves your overall problem-solving abilities and code organization.
Remember, the key steps are:
- Understand the problem thoroughly
- Visualize the problem and its components
- Identify sub-problems
- Prioritize and order the sub-problems
- Solve each sub-problem individually
- Integrate the solutions
- Test the complete solution
- Reflect and refine your approach
By consistently applying these techniques and leveraging resources like AlgoCademy, you’ll be well-prepared to tackle complex coding challenges, ace technical interviews, and succeed in your programming career. Remember, every complex problem is just a collection of simpler problems waiting to be solved. With practice and perseverance, you’ll develop the skills to confidently approach any coding challenge that comes your way.