Why You Should Spend More Time Understanding the Problem Than Writing the Code
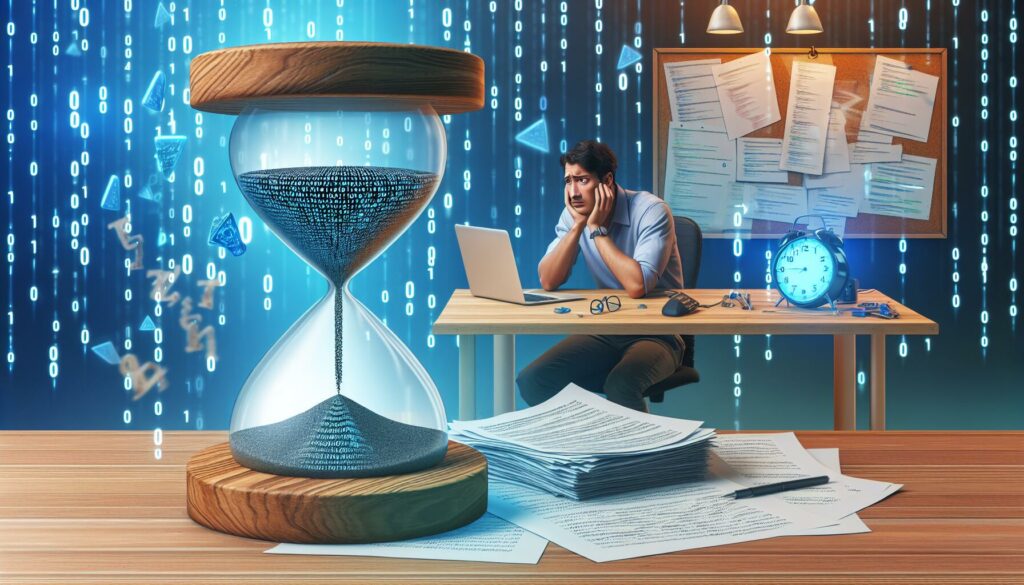
In the world of programming and software development, there’s a common misconception that the most crucial part of solving a problem is writing the code. However, experienced developers and successful tech companies know a secret: the key to efficient and effective problem-solving lies in thoroughly understanding the problem before diving into coding. This article will explore why dedicating more time to problem comprehension is not just beneficial but essential for programmers at all levels, from beginners to those preparing for technical interviews at major tech companies.
The Importance of Problem Understanding in Programming
When faced with a coding challenge, whether it’s a small personal project or a complex algorithm during a technical interview, the natural instinct for many programmers is to start writing code immediately. This approach, while tempting, often leads to inefficient solutions, wasted time, and frustration. Here’s why spending more time on understanding the problem is crucial:
1. It Leads to More Efficient Solutions
By thoroughly analyzing the problem, you can identify the core issues and requirements. This understanding allows you to develop a more targeted and efficient solution. Often, a well-understood problem can be solved with less code and in less time than one that’s hastily approached.
2. It Helps Identify Edge Cases
A deep understanding of the problem helps you anticipate potential edge cases and special scenarios. This foresight can save you from having to repeatedly revise your code to handle unexpected inputs or conditions.
3. It Improves Problem-Solving Skills
The process of breaking down and analyzing a problem enhances your overall problem-solving abilities. These skills are transferable and highly valued in the tech industry, especially during technical interviews.
4. It Reduces Debugging Time
When you fully grasp the problem, you’re less likely to make fundamental mistakes in your approach. This can significantly reduce the time spent on debugging and refactoring later in the development process.
Strategies for Better Problem Understanding
Now that we’ve established the importance of problem understanding, let’s explore some effective strategies to improve this crucial skill:
1. Read the Problem Statement Multiple Times
It may seem obvious, but many programmers rush through the problem description. Read it carefully, multiple times if necessary. Each read-through can reveal new insights or details you might have missed initially.
2. Identify Key Information
As you read, highlight or note down the key pieces of information:
- Input format and constraints
- Expected output
- Any specific requirements or limitations
- Performance expectations (time/space complexity)
3. Restate the Problem in Your Own Words
Try to explain the problem to yourself or someone else in your own words. This exercise can help you identify any areas where your understanding might be lacking and force you to think about the problem from different angles.
4. Break Down the Problem
Divide the problem into smaller, manageable sub-problems. This approach, known as “divide and conquer,” can make complex problems more approachable and help you identify potential modular solutions.
5. Use Visual Aids
For many problems, especially those involving data structures or algorithms, visual representations can be incredibly helpful. Draw diagrams, flowcharts, or use other visual aids to map out the problem and potential solutions.
6. Consider Multiple Approaches
Before settling on a solution, brainstorm multiple approaches to solving the problem. This exercise can help you identify the most efficient solution and prepare you for follow-up questions in interview scenarios.
7. Think About Edge Cases
Challenge yourself to think about potential edge cases or special scenarios. What happens with extremely large or small inputs? How does your solution handle empty sets or null values?
The Problem-Understanding Process in Action
Let’s walk through an example of how this process might look in practice. Consider the following problem statement:
Given an array of integers, find the contiguous subarray with the largest sum.
This is the famous “Maximum Subarray Problem.” Let’s apply our problem-understanding strategies:
1. Read Multiple Times and Identify Key Information
- Input: An array of integers (positive and negative)
- Output: The sum of the contiguous subarray with the largest sum
- Note: We need to find a contiguous subarray, not just any subset
2. Restate the Problem
“We need to find a sequence of consecutive numbers in the array that, when added together, give the largest possible sum.”
3. Break Down the Problem
- We need to consider all possible subarrays
- For each subarray, we need to calculate its sum
- We need to keep track of the maximum sum we’ve seen
4. Use Visual Aids
Let’s visualize this with a small example:
Array: [-2, 1, -3, 4, -1, 2, 1, -5, 4]
Subarrays:
[-2]
[-2, 1]
[-2, 1, -3]
...
[4, -1, 2, 1]
...
[4]
Maximum subarray: [4, -1, 2, 1] with sum 6
5. Consider Multiple Approaches
- Brute Force: Check all possible subarrays (O(n^3) time complexity)
- Improved Brute Force: Optimize sum calculation (O(n^2) time complexity)
- Divide and Conquer: Recursively divide the array (O(n log n) time complexity)
- Kadane’s Algorithm: Dynamic programming approach (O(n) time complexity)
6. Think About Edge Cases
- What if all numbers are negative?
- What if the array is empty?
- What if there are multiple subarrays with the same maximum sum?
By going through this process, we’ve gained a deep understanding of the problem before writing a single line of code. This understanding will guide our implementation and help us write more efficient, bug-free code.
The Role of Problem Understanding in Technical Interviews
For those preparing for technical interviews, especially at major tech companies often referred to as FAANG (Facebook/Meta, Amazon, Apple, Netflix, Google), the ability to thoroughly understand and analyze a problem is crucial. Here’s why:
1. It Demonstrates Analytical Skills
Interviewers are not just interested in your coding abilities; they want to see how you approach and analyze problems. Spending time to understand the problem showcases your analytical skills and thought process.
2. It Allows for Better Communication
By fully grasping the problem, you can articulate your thoughts and approach more clearly to the interviewer. This communication is a key aspect of the interview process.
3. It Leads to More Optimal Solutions
A thorough understanding often leads to identifying more efficient solutions, which is highly valued in technical interviews.
4. It Prepares You for Follow-up Questions
Interviewers often ask follow-up questions or present variations of the original problem. A deep understanding of the initial problem makes it easier to adapt to these changes.
Common Pitfalls of Rushing to Code
To further emphasize the importance of problem understanding, let’s look at some common pitfalls that can occur when programmers rush to code without fully grasping the problem:
1. Solving the Wrong Problem
It’s surprisingly easy to misinterpret a problem statement and end up solving something slightly (or entirely) different from what was asked. This is particularly problematic in interview situations or when working on client projects.
2. Overlooking Crucial Details
Problem statements often contain subtle but important details that can significantly impact the solution. Rushing can lead to missing these critical points.
3. Choosing Suboptimal Algorithms
Without a full understanding of the problem, you might choose an algorithm that works but is far from optimal in terms of time or space complexity.
4. Writing Unnecessarily Complex Code
A lack of clear understanding often results in overly complicated solutions. Remember, the best code is often the simplest code that solves the problem effectively.
5. Difficulty in Debugging
When you don’t fully understand the problem, identifying and fixing bugs becomes much more challenging. You might find yourself making changes without really knowing why they’re necessary.
Balancing Understanding and Coding
While we’ve emphasized the importance of problem understanding, it’s also crucial to find the right balance. Here are some tips to help you strike that balance:
1. Set a Time Limit for Initial Analysis
Depending on the complexity of the problem, give yourself a set amount of time (e.g., 5-15 minutes) for initial analysis before starting to code.
2. Use Pseudocode as a Bridge
After your initial analysis, write pseudocode to outline your approach. This serves as a bridge between problem understanding and actual coding.
3. Implement in Small Steps
Start implementing your solution in small, manageable steps. This allows you to verify your understanding as you go and make adjustments if needed.
4. Regularly Revisit the Problem Statement
As you code, periodically refer back to the original problem statement to ensure you’re staying on track.
5. Be Prepared to Pivot
If you realize your initial understanding was flawed, be willing to step back, reassess, and change your approach if necessary.
Tools and Techniques for Enhancing Problem Understanding
To further improve your problem-understanding skills, consider incorporating these tools and techniques into your practice:
1. Mind Mapping
Use mind mapping tools to visually organize the various aspects of the problem and potential solution approaches.
2. Rubber Duck Debugging
Explain the problem to an inanimate object (like a rubber duck) or a non-technical person. This forces you to articulate the problem clearly and often leads to new insights.
3. Problem Journals
Keep a journal where you document problem statements, your analysis process, and the lessons learned. This can be invaluable for identifying patterns in your problem-solving approach over time.
4. Collaborative Problem Solving
Discuss problems with peers or in coding forums. Different perspectives can often shed light on aspects of the problem you might have overlooked.
5. Algorithmic Thinking Exercises
Engage in exercises specifically designed to improve algorithmic thinking, such as those found on platforms like AlgoCademy. These can help you develop a more structured approach to problem analysis.
Conclusion
In the world of programming, the ability to thoroughly understand a problem before diving into code is a skill that separates great developers from good ones. By investing time in comprehending the nuances of a problem, you set yourself up for more efficient coding, better solutions, and ultimately, greater success in your programming endeavors.
Whether you’re a beginner learning the ropes, a professional developer working on complex projects, or an aspiring candidate preparing for technical interviews at top tech companies, honing your problem-understanding skills is crucial. Remember, the time spent on understanding is not time wasted – it’s an investment that pays dividends throughout the entire problem-solving process.
As you continue your journey in programming, challenge yourself to resist the urge to immediately start coding when faced with a problem. Instead, take a step back, analyze, and truly understand what you’re trying to solve. With practice, this approach will become second nature, elevating your programming skills and making you a more effective problem solver in any coding scenario.