How to Approach a Coding Project Like an Architect: Design First, Build Later
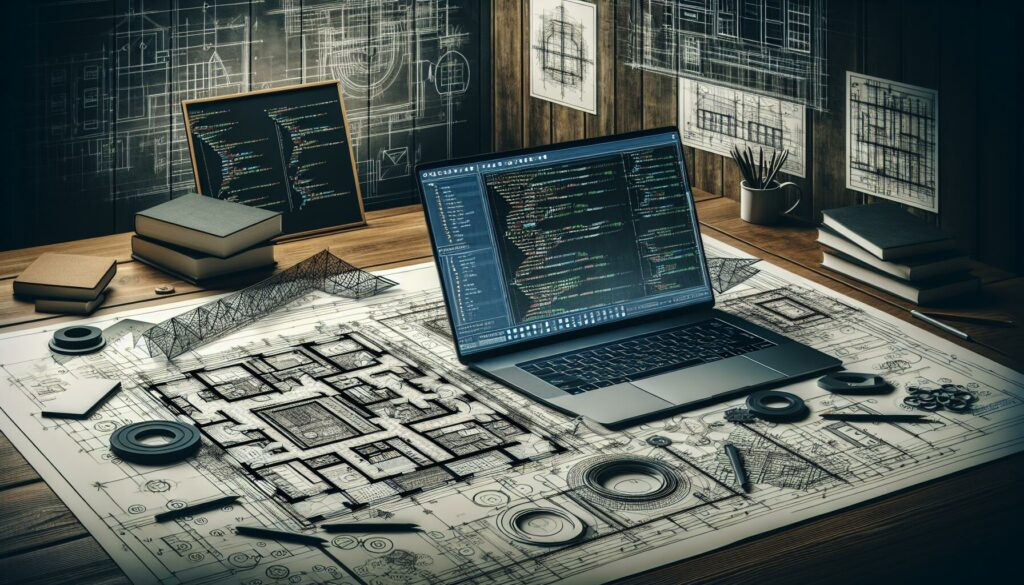
In the world of software development, the temptation to dive straight into coding can be overwhelming. However, seasoned professionals know that taking a step back and approaching a project with an architect’s mindset can lead to more robust, scalable, and maintainable solutions. This article will explore how to tackle coding projects like an architect, emphasizing the importance of design before implementation.
Understanding the Architect’s Approach
Just as an architect doesn’t start building a house without a blueprint, a software developer shouldn’t start coding without a clear design. The architect’s approach involves several key steps:
- Gathering requirements
- Conceptualizing the overall structure
- Creating detailed plans
- Considering potential challenges and constraints
- Iterating on the design before construction begins
By adopting this methodology, developers can create more thoughtful, efficient, and effective solutions.
The Benefits of Designing First
Taking the time to design before coding offers numerous advantages:
- Clearer project vision and goals
- Identification of potential issues early on
- More efficient use of development time
- Easier collaboration with team members
- Greater flexibility for future changes and scaling
Let’s delve deeper into each step of the architect’s approach and how it applies to coding projects.
1. Gathering Requirements
Before any design work begins, it’s crucial to have a thorough understanding of the project requirements. This involves:
- Communicating with stakeholders
- Defining user stories and use cases
- Identifying functional and non-functional requirements
- Establishing project constraints (e.g., timeline, budget, technology stack)
For example, if you’re building a web application for a small business, you might gather requirements like:
- User authentication and authorization
- Product catalog management
- Shopping cart functionality
- Payment processing integration
- Responsive design for mobile devices
By clearly defining these requirements upfront, you create a solid foundation for the design phase.
2. Conceptualizing the Overall Structure
Once you have a clear understanding of the requirements, it’s time to conceptualize the high-level structure of your project. This involves:
- Identifying major components or modules
- Determining how these components will interact
- Choosing appropriate architectural patterns (e.g., MVC, microservices)
- Considering scalability and performance requirements
For our web application example, you might decide on a structure like this:
- Frontend: React.js single-page application
- Backend: Node.js API server
- Database: MongoDB for product and user data
- Authentication: JWT-based system
- Payment Processing: Integration with Stripe API
This high-level overview provides a roadmap for more detailed design work.
3. Creating Detailed Plans
With the overall structure in place, it’s time to create more detailed plans. This step involves:
- Designing database schemas
- Creating API specifications
- Outlining class structures and relationships
- Designing user interfaces and user flows
Let’s look at an example of how you might design the database schema for the product catalog:
// Product Schema
{
_id: ObjectId,
name: String,
description: String,
price: Number,
category: String,
imageUrl: String,
createdAt: Date,
updatedAt: Date
}
// Category Schema
{
_id: ObjectId,
name: String,
description: String,
parentCategory: ObjectId (reference to Category)
}
This level of detail helps clarify the data structure and relationships before any code is written.
4. Considering Potential Challenges and Constraints
An architect always considers potential issues that might arise during construction. In software development, this means:
- Identifying potential performance bottlenecks
- Considering security implications
- Planning for error handling and edge cases
- Evaluating the impact of chosen technologies
For our e-commerce application, some considerations might include:
- How to handle high traffic during sales events
- Securing sensitive user data and payment information
- Implementing robust error handling for payment processing
- Ensuring compatibility across different browsers and devices
By anticipating these challenges early, you can design solutions proactively rather than reactively.
5. Iterating on the Design
The final step before coding begins is to iterate on the design. This involves:
- Reviewing the design with team members and stakeholders
- Conducting design reviews or walkthroughs
- Making necessary adjustments based on feedback
- Ensuring the design aligns with best practices and coding standards
This iterative process helps refine the design and catch potential issues before they become costly to fix during development.
Implementing the Design: From Blueprint to Code
Once the design phase is complete, it’s time to start coding. However, the architect’s mindset doesn’t end here. As you implement your design, keep these principles in mind:
1. Modular Development
Break your code into modular, reusable components. This approach makes your code easier to understand, test, and maintain. For example, in our e-commerce application, you might create separate modules for user authentication, product management, and order processing.
2. Consistent Coding Standards
Adhere to consistent coding standards throughout your project. This includes naming conventions, code formatting, and documentation practices. Tools like ESLint and Prettier can help enforce these standards automatically.
3. Version Control
Use version control systems like Git to track changes, collaborate with team members, and manage different versions of your code. Implement a branching strategy that aligns with your development workflow.
4. Testing
Implement a comprehensive testing strategy, including unit tests, integration tests, and end-to-end tests. Test-driven development (TDD) can be particularly effective in ensuring your code meets the designed specifications.
5. Documentation
Maintain clear and up-to-date documentation throughout the development process. This includes code comments, README files, and API documentation. Tools like JSDoc can help generate documentation from your code comments.
Example: Implementing a Product Service
Let’s look at how we might implement a product service based on our earlier design:
// productService.js
const mongoose = require('mongoose');
const productSchema = new mongoose.Schema({
name: { type: String, required: true },
description: { type: String, required: true },
price: { type: Number, required: true },
category: { type: String, required: true },
imageUrl: { type: String, required: true },
}, { timestamps: true });
const Product = mongoose.model('Product', productSchema);
class ProductService {
async createProduct(productData) {
const product = new Product(productData);
return await product.save();
}
async getProductById(id) {
return await Product.findById(id);
}
async updateProduct(id, updateData) {
return await Product.findByIdAndUpdate(id, updateData, { new: true });
}
async deleteProduct(id) {
return await Product.findByIdAndDelete(id);
}
async listProducts(filters = {}) {
return await Product.find(filters);
}
}
module.exports = new ProductService();
This implementation closely follows our database schema design and provides a clean, modular interface for managing products in our application.
Continuous Improvement and Refactoring
Even after the initial implementation, the architect’s mindset continues to play a crucial role. As you develop and maintain your project:
- Regularly review and refactor your code to improve its structure and efficiency
- Stay open to feedback and be willing to iterate on your design
- Keep an eye on emerging technologies and best practices that could benefit your project
- Conduct post-implementation reviews to learn from successes and challenges
Remember, good architecture is not static; it evolves with the needs of the project and the capabilities of the technology stack.
Tools for Architectural Design in Software Development
Several tools can aid in the architectural design process:
- UML Diagrams: Tools like LucidChart or Draw.io for creating visual representations of your system architecture
- Wireframing Tools: Figma or Sketch for designing user interfaces
- API Design Tools: Swagger or Postman for designing and documenting APIs
- Database Modeling Tools: MySQL Workbench or MongoDB Compass for designing database schemas
- Project Management Tools: Jira or Trello for tracking tasks and progress
- Over-engineering: Be cautious not to over-complicate your design. Strive for simplicity while meeting all requirements.
- Balancing flexibility and specificity: Design for future growth, but don’t sacrifice current needs for hypothetical future scenarios.
- Time constraints: In fast-paced environments, there may be pressure to skip the design phase. Advocate for its importance while finding ways to streamline the process.
- Changing requirements: Be prepared to adapt your design as requirements evolve. Agile methodologies can help manage this challenge.
- Analyze the current system’s functionality and pain points
- Interview stakeholders to understand new requirements and future goals
- Identify performance bottlenecks and scalability issues
- Propose a microservices architecture to replace the monolithic structure
- Plan for a gradual migration to minimize disruption to the business
- Choose modern technologies: Node.js for backend services, React for the frontend, and Docker for containerization
- Break down the system into microservices: User Service, Product Service, Order Service, etc.
- Design APIs for inter-service communication
- Plan for data migration from the old system to new databases
- Implement a robust logging and monitoring system for the distributed architecture
- Design a strategy for handling distributed transactions
- Plan for service discovery and load balancing
- Present the design to the development team and stakeholders
- Conduct a security review of the proposed architecture
- Refine the migration strategy based on feedback
These tools can help you visualize and communicate your design more effectively.
Challenges in Architectural Design
While the benefits of architectural design are clear, it’s not without its challenges:
Case Study: Redesigning a Legacy System
Let’s consider a scenario where you’re tasked with redesigning a legacy e-commerce system. The current system is a monolithic PHP application that’s becoming difficult to maintain and scale. Here’s how you might approach this project with an architect’s mindset:
1. Requirement Gathering
2. Conceptual Design
3. Detailed Design
4. Addressing Challenges
5. Iteration and Review
By following this architectural approach, you can transform a legacy system into a modern, scalable application while minimizing risks and disruptions.
Conclusion: The Architect’s Mindset in Coding
Approaching a coding project like an architect – designing first and building later – offers numerous benefits. It leads to more thoughtful, scalable, and maintainable solutions. By taking the time to gather requirements, conceptualize the structure, create detailed plans, consider challenges, and iterate on the design, you set yourself up for success in the implementation phase.
Remember, good architecture is about making informed decisions that balance current needs with future possibilities. It’s about creating a solid foundation that can support growth and change. As you develop your skills, strive to cultivate this architect’s mindset. It will serve you well throughout your career in software development.
Whether you’re working on a small personal project or a large-scale enterprise application, taking the time to think like an architect will ultimately save time, reduce errors, and result in higher-quality software. So the next time you’re tempted to dive straight into coding, take a step back, put on your architect’s hat, and start with design. Your future self (and your team) will thank you for it.