Why You Can’t Build Projects Without First Mastering the Fundamentals
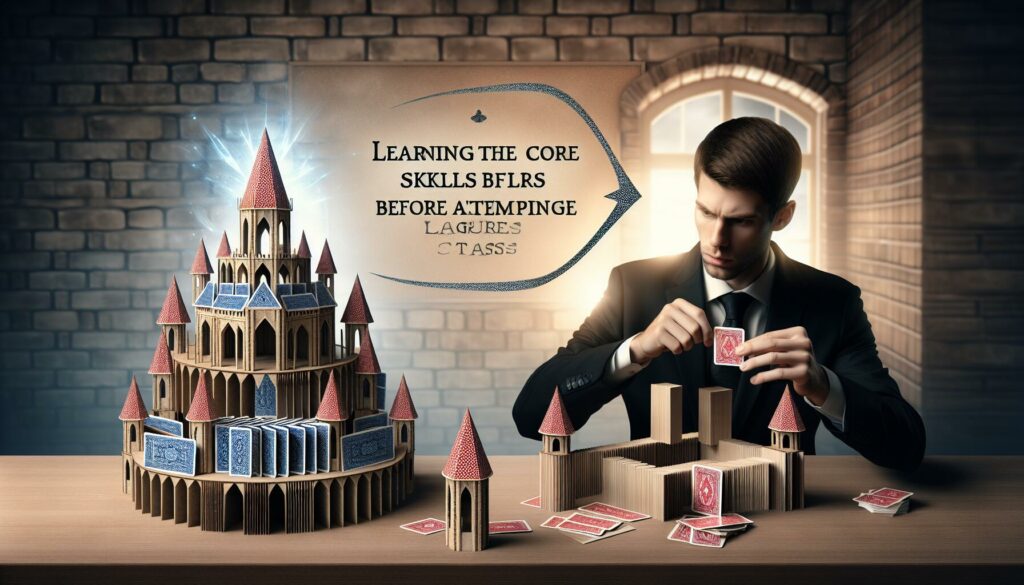
In the world of coding and software development, there’s a common misconception that you can jump straight into building projects without first mastering the fundamentals. This idea is especially prevalent among beginners who are eager to create something tangible and impressive. However, this approach often leads to frustration, inefficient code, and a lack of understanding of why certain solutions work (or don’t work). In this article, we’ll explore why mastering the fundamentals is crucial before diving into project building, and how platforms like AlgoCademy can help you build a solid foundation for your coding journey.
The Importance of Fundamentals in Coding
Imagine trying to build a house without understanding the basics of construction, materials, or architecture. You might be able to stack some bricks or nail some boards together, but the result would likely be unstable, inefficient, and potentially dangerous. The same principle applies to coding. Without a strong grasp of the fundamentals, you may be able to cobble together a working program, but it will likely be:
- Inefficient and slow
- Difficult to maintain and debug
- Prone to errors and vulnerabilities
- Hard to scale or expand
Let’s delve deeper into why mastering the fundamentals is so crucial for aspiring programmers.
1. Understanding the Building Blocks
Programming languages are built on fundamental concepts such as variables, data types, control structures, and functions. These are the building blocks of any program, regardless of its complexity. Without a solid understanding of these basics, you’ll struggle to write coherent and effective code.
For example, consider the concept of variables. A beginner might use variables without fully grasping scope, mutability, or memory management. This can lead to issues like:
- Unintended side effects in your code
- Memory leaks
- Difficulty in debugging
By mastering these fundamentals, you’ll be able to write cleaner, more efficient code from the start.
2. Problem-Solving Skills
Coding is essentially problem-solving. When you’re building a project, you’re constantly faced with challenges that need to be overcome. Without a strong foundation in algorithmic thinking and problem-solving strategies, you’ll find yourself stuck more often than not.
Platforms like AlgoCademy focus on developing these critical thinking skills through interactive coding tutorials and algorithmic challenges. By working through these exercises, you’ll learn how to:
- Break down complex problems into smaller, manageable parts
- Identify patterns and apply appropriate solutions
- Optimize your code for better performance
- Debug effectively when things go wrong
3. Efficiency and Best Practices
When you’re just starting out, it’s easy to fall into the trap of writing code that “just works” without considering efficiency or best practices. However, as your projects grow in complexity, this approach can lead to significant problems.
By mastering the fundamentals, you’ll learn:
- Time and space complexity analysis
- Proper code organization and structure
- Design patterns and when to use them
- How to write readable and maintainable code
These skills are invaluable when working on larger projects or collaborating with other developers.
4. Adaptability and Learning New Technologies
The tech world is constantly evolving, with new languages, frameworks, and tools emerging regularly. When you have a strong grasp of the fundamentals, you’re better equipped to adapt to these changes and learn new technologies quickly.
For instance, if you understand the basic concepts of object-oriented programming, you’ll find it much easier to switch between languages like Java, Python, or C++. Similarly, a solid understanding of data structures will help you work with various databases and storage solutions more effectively.
The Pitfalls of Skipping the Fundamentals
Now that we’ve established the importance of mastering the fundamentals, let’s look at some of the common pitfalls that occur when beginners try to skip this crucial step.
1. Reinventing the Wheel
Without a strong foundation, you might find yourself solving problems that have already been solved efficiently. For example, you might spend hours implementing a sorting algorithm from scratch, unaware that there are built-in functions or libraries that can do the job more efficiently.
Consider this Python example of sorting a list:
# Inefficient bubble sort implementation
def bubble_sort(arr):
n = len(arr)
for i in range(n):
for j in range(0, n-i-1):
if arr[j] > arr[j+1]:
arr[j], arr[j+1] = arr[j+1], arr[j]
return arr
# Using Python's built-in sort function
sorted_list = sorted(my_list)
The built-in sorted()
function is not only more concise but also significantly faster, especially for large lists.
2. Security Vulnerabilities
Security is a critical aspect of software development that is often overlooked by beginners. Without understanding the fundamentals of secure coding practices, you might inadvertently create vulnerabilities in your projects.
For example, consider this PHP code that doesn’t properly sanitize user input:
<?php
$username = $_POST['username'];
$query = "SELECT * FROM users WHERE username = '$username'";
$result = mysqli_query($connection, $query);
?>
This code is vulnerable to SQL injection attacks. A user could input malicious SQL code in the username field, potentially gaining unauthorized access to the database. Understanding the fundamentals of web security would help you avoid such critical mistakes.
3. Performance Issues
As your projects grow in size and complexity, performance becomes increasingly important. Without a solid understanding of algorithms and data structures, you might write code that works for small inputs but becomes painfully slow as the input size increases.
Consider this JavaScript example of finding the sum of all numbers in an array:
// Inefficient approach
function sumArray(arr) {
let sum = 0;
for (let i = 0; i < arr.length; i++) {
sum += arr[i];
}
return sum;
}
// More efficient approach using reduce
function sumArrayEfficient(arr) {
return arr.reduce((sum, num) => sum + num, 0);
}
While both functions produce the same result, the second approach is more concise and generally more efficient, especially for large arrays.
4. Difficulty in Debugging and Maintenance
When you don’t have a strong grasp of the fundamentals, debugging becomes a nightmare. You might find yourself making random changes to your code, hoping to fix issues without really understanding what’s causing them.
Moreover, as your projects grow, maintaining and updating your code becomes increasingly challenging. You might struggle to add new features or modify existing ones without breaking other parts of your application.
How AlgoCademy Can Help You Master the Fundamentals
AlgoCademy is designed to help aspiring programmers build a strong foundation in coding and algorithmic thinking. Here’s how it can help you master the fundamentals:
1. Structured Learning Path
AlgoCademy provides a structured curriculum that takes you from basic concepts to advanced algorithms and data structures. This step-by-step approach ensures that you build a solid foundation before moving on to more complex topics.
2. Interactive Coding Tutorials
The platform offers interactive coding tutorials that allow you to practice what you learn in real-time. This hands-on approach helps reinforce your understanding of key concepts and improves retention.
3. Problem-Solving Challenges
AlgoCademy features a wide range of coding challenges that test your problem-solving skills. These challenges are designed to mimic real-world scenarios and technical interview questions, helping you apply your knowledge in practical situations.
4. AI-Powered Assistance
The platform leverages AI technology to provide personalized guidance and feedback. This feature can help you identify areas where you need improvement and offer tailored suggestions to enhance your learning.
5. Focus on FAANG-Level Preparation
AlgoCademy’s curriculum is geared towards preparing you for technical interviews at major tech companies. This focus ensures that you’re learning industry-relevant skills and best practices.
Building Projects: When and How to Start
While it’s crucial to master the fundamentals before diving into complex projects, that doesn’t mean you should avoid coding projects altogether. In fact, small projects can be an excellent way to reinforce your learning and apply your skills. Here’s a suggested approach:
1. Start with Micro-Projects
Begin with very small, focused projects that apply a specific concept you’ve learned. For example:
- Create a simple calculator to practice basic arithmetic operations and user input
- Build a to-do list application to understand data storage and manipulation
- Develop a basic web scraper to learn about HTTP requests and parsing HTML
2. Gradually Increase Complexity
As you become more comfortable with the basics, start taking on slightly more complex projects. For instance:
- Create a simple game like Tic-Tac-Toe or Hangman
- Build a basic CRUD (Create, Read, Update, Delete) application
- Develop a simple API that interacts with a database
3. Combine Multiple Concepts
Once you have a good grasp of various fundamental concepts, start working on projects that combine multiple areas of knowledge. For example:
- Create a full-stack web application with user authentication
- Develop a data visualization tool that processes and displays complex datasets
- Build a simple recommendation system using machine learning algorithms
4. Contribute to Open Source
Contributing to open-source projects is an excellent way to apply your skills in a real-world context. Start with small contributions like bug fixes or documentation improvements, and gradually work your way up to more significant features.
Conclusion
While the allure of jumping straight into building impressive projects is strong, the importance of mastering the fundamentals cannot be overstated. A solid foundation in coding basics, algorithms, and data structures is crucial for writing efficient, maintainable, and scalable code.
Platforms like AlgoCademy provide a structured approach to learning these fundamentals, combining theoretical knowledge with practical application. By following a well-designed curriculum and gradually increasing the complexity of your projects, you’ll be well-prepared to take on more significant challenges and excel in technical interviews.
Remember, every expert programmer started as a beginner. The key is to be patient, persistent, and committed to continuous learning. Master the fundamentals, and you’ll be well on your way to becoming a skilled and confident developer capable of tackling any project that comes your way.
So, before you dive into that ambitious project idea, take the time to build a strong foundation. Your future self (and your code) will thank you for it!