How to Write the First Line of Code in Any Project: A Comprehensive Guide
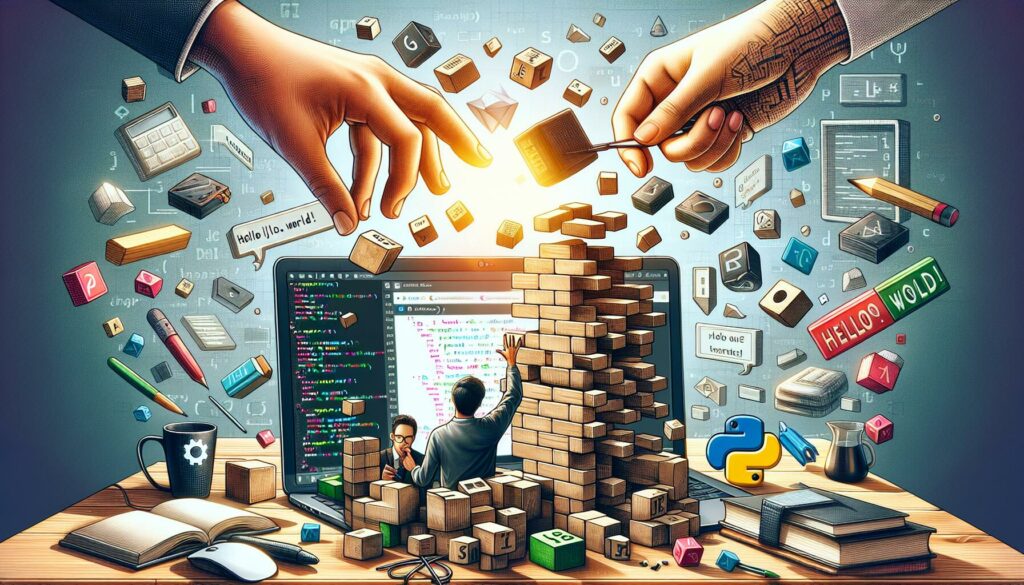
Starting a new coding project can be both exciting and daunting. Whether you’re a beginner just learning to code or an experienced developer tackling a new challenge, the first line of code you write sets the tone for your entire project. In this comprehensive guide, we’ll explore the importance of that initial line, best practices for getting started, and how to approach different types of projects with confidence.
Table of Contents
- Understanding the Importance of the First Line
- Preparation Before Writing Code
- Common First Lines in Different Programming Languages
- Approaching Different Project Types
- Best Practices for Starting a Project
- Common Mistakes to Avoid
- Tools and Resources for Getting Started
- Conclusion
Understanding the Importance of the First Line
The first line of code in any project is more than just a starting point; it’s a statement of intent. It can set the stage for the entire structure of your program, define the programming language or framework you’re using, and even impact the overall efficiency of your code. Here’s why the first line matters:
- Setting the tone: Your first line often indicates the programming language, coding style, and sometimes even the project’s purpose.
- Establishing structure: In many languages, the first line can define namespaces, modules, or classes that will shape the rest of your code.
- Ensuring compatibility: Especially in scripting languages, the first line might specify the interpreter or version to use.
- Documentation: Sometimes, the first line is a comment that provides crucial information about the file or project.
Understanding the significance of this initial step can help you start your project on the right foot and maintain good coding practices throughout development.
Preparation Before Writing Code
Before you write that first line of code, there are several important steps you should take to ensure you’re well-prepared:
- Define your project goals: Clearly outline what you want to achieve with your code. Having a clear objective will guide your initial decisions.
- Choose your programming language: Select the language that best suits your project’s needs and your skill level.
- Set up your development environment: Install necessary tools, IDEs, and dependencies required for your chosen language and project type.
- Plan your project structure: Sketch out a basic architecture or flowchart of how your code will be organized.
- Research best practices: Familiarize yourself with coding standards and conventions for your chosen language and project type.
- Version control setup: Initialize a Git repository to track changes from the very beginning.
By taking these preparatory steps, you’ll be in a much better position to write that crucial first line of code with confidence and purpose.
Common First Lines in Different Programming Languages
The first line of code can vary significantly depending on the programming language you’re using. Here are some common first lines you might encounter or write in various popular languages:
Python
In Python, the first line is often a shebang (in Unix-like systems) or an encoding declaration:
#!/usr/bin/env python3
# -*- coding: utf-8 -*-
JavaScript
In modern JavaScript, you might start with a strict mode declaration:
"use strict";
Java
Java files typically begin with a package declaration:
package com.example.myproject;
C++
C++ programs often start with include statements:
#include <iostream>
Ruby
Ruby files might begin with a shebang or an encoding comment:
#!/usr/bin/env ruby
# encoding: utf-8
Go
Go files start with a package declaration:
package main
PHP
PHP files typically begin with the PHP opening tag:
<?php
Remember, these are just common examples. The actual first line of your code may vary depending on your specific project requirements and coding standards.
Approaching Different Project Types
Different types of projects may require different approaches when it comes to writing the first line of code. Let’s explore how to start various common project types:
Web Development
For a basic HTML file:
<!DOCTYPE html>
For a CSS file, you might start with a reset or normalize statement:
* { box-sizing: border-box; margin: 0; padding: 0; }
For a JavaScript file in a web project:
document.addEventListener("DOMContentLoaded", function() {
// Your code here
});
Mobile App Development
In Swift for iOS:
import UIKit
In Kotlin for Android:
package com.example.myapp
Data Science Project
In a Jupyter Notebook or Python script:
import pandas as pd
import numpy as np
import matplotlib.pyplot as plt
Command-Line Application
In Python:
import argparse
def main():
parser = argparse.ArgumentParser(description="Your program description")
# Add arguments here
args = parser.parse_args()
# Your main code here
if __name__ == "__main__":
main()
Game Development
Using Unity with C#:
using UnityEngine;
Machine Learning Project
In Python with TensorFlow:
import tensorflow as tf
These examples demonstrate how the first lines can vary significantly based on the project type. Always consider the specific requirements and best practices of your project domain when writing your initial code.
Best Practices for Starting a Project
Regardless of the project type or programming language, there are several best practices to keep in mind when writing your first lines of code:
- Start with documentation: Begin your file with a comment block that describes the purpose of the file, author information, and any other relevant details.
- Use meaningful names: If you’re declaring variables or functions in your first lines, ensure they have clear, descriptive names.
- Follow language conventions: Adhere to the style guide and best practices of your chosen programming language.
- Keep it simple: Don’t try to accomplish too much in the first few lines. Start with the essentials and build from there.
- Consider dependencies: If your project requires external libraries or modules, import or include them at the beginning of your file.
- Use version control: Make your first commit after writing the initial setup code.
- Think about configuration: If your project needs configuration settings, consider setting them up early in your code.
- Plan for testing: Even from the first line, keep testability in mind. Structure your code in a way that will be easy to unit test later.
By following these best practices, you’ll set a solid foundation for your project and make it easier to maintain and expand your code as it grows.
Common Mistakes to Avoid
When writing the first lines of code for a project, there are several common pitfalls that developers, especially beginners, should be aware of:
- Overcomplicating the start: Trying to do too much too soon can lead to confusion and overcomplicated code. Start simple and build complexity gradually.
- Ignoring project structure: Failing to plan the overall structure of your project can lead to disorganized code as your project grows.
- Neglecting version control: Not initializing a Git repository or making your first commit can make it difficult to track changes and collaborate later.
- Skipping documentation: Failing to add comments or docstrings from the beginning can make your code harder to understand and maintain.
- Using outdated syntax: Make sure you’re using the most up-to-date syntax and best practices for your chosen language and framework.
- Hardcoding configuration: Embedding configuration details directly in your code instead of using separate configuration files or environment variables.
- Ignoring error handling: Not considering how to handle potential errors or exceptions from the start.
- Forgetting about scalability: Writing code that works for small inputs but fails to consider how it will perform with larger datasets or increased usage.
By being aware of these common mistakes, you can take steps to avoid them and start your project on a stronger footing.
Tools and Resources for Getting Started
To help you write your first lines of code effectively, consider using these tools and resources:
Integrated Development Environments (IDEs)
- Visual Studio Code: A versatile, free IDE that supports multiple languages and has a rich ecosystem of extensions.
- PyCharm: An excellent IDE for Python development, with both free and paid versions available.
- IntelliJ IDEA: A powerful IDE for Java development, also supporting many other languages.
- Xcode: The go-to IDE for iOS and macOS development.
Version Control Systems
- Git: The most widely used version control system.
- GitHub: A platform for hosting and collaborating on Git repositories.
- GitLab: Another popular Git repository manager with built-in CI/CD capabilities.
Online Resources
- Stack Overflow: A community-driven Q&A platform for programming-related questions.
- MDN Web Docs: Comprehensive documentation for web technologies.
- Language-specific documentation: Official documentation for your chosen programming language (e.g., Python.org, JavaScript.info).
Code Generators and Boilerplate Tools
- Create React App: For quickly setting up a new React project.
- Spring Initializr: For generating Spring Boot project structures.
- Yeoman: A general-purpose scaffolding tool for various project types.
Learning Platforms
- Codecademy: Interactive coding courses for various languages and technologies.
- freeCodeCamp: Free coding bootcamp with a comprehensive curriculum.
- AlgoCademy: Specialized platform for learning algorithmic thinking and preparing for technical interviews.
These tools and resources can provide valuable guidance and support as you begin writing your first lines of code and developing your project.
Conclusion
Writing the first line of code in any project is a crucial step that sets the tone for your entire development process. By understanding the importance of this initial step, preparing adequately, following best practices, and avoiding common mistakes, you can start your coding journey on the right foot.
Remember that every great software project, no matter how complex, started with a single line of code. Whether you’re building a simple script or embarking on a large-scale application, the principles we’ve discussed in this guide will help you begin with confidence and purpose.
As you continue to develop your coding skills, platforms like AlgoCademy can provide valuable resources and guidance, especially when it comes to algorithmic thinking and preparing for technical interviews. Keep practicing, stay curious, and don’t be afraid to experiment with different approaches as you write those all-important first lines of code.
Happy coding, and may your first lines lead to many successful projects!