How to Avoid the Blank Page Panic When Starting a New Coding Problem
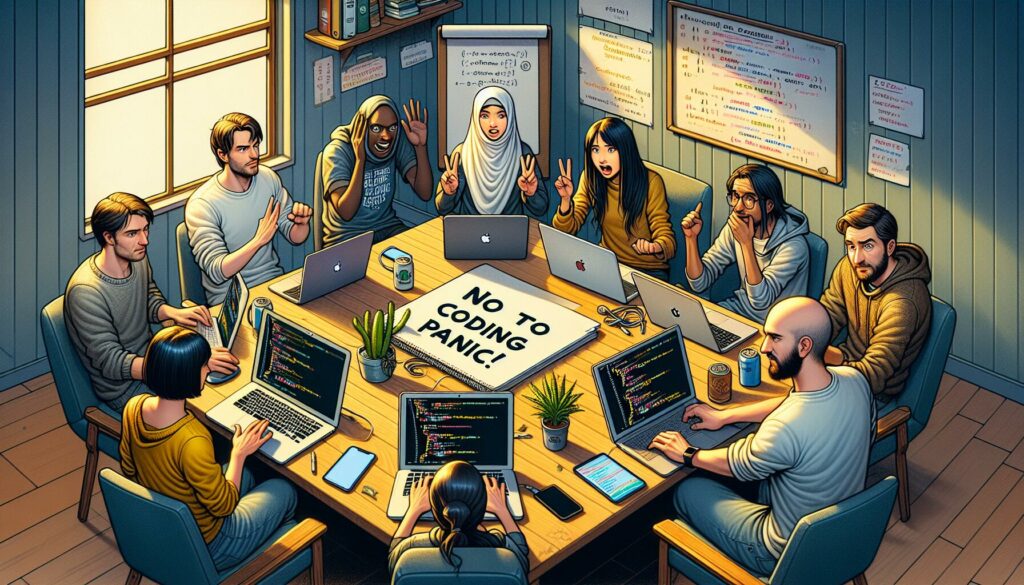
Every programmer, whether a beginner or a seasoned professional, has faced the dreaded “blank page panic” at some point in their career. It’s that moment when you’re presented with a new coding problem, and your mind goes blank. The cursor blinks mockingly on the empty screen, and you feel a surge of anxiety as you wonder where to begin. But fear not! This comprehensive guide will equip you with strategies to overcome this common hurdle and approach new coding problems with confidence.
Understanding the Blank Page Panic
Before we dive into the solutions, it’s essential to understand what causes this panic. The blank page panic is often rooted in:
- Fear of the unknown
- Feeling overwhelmed by the problem’s complexity
- Pressure to come up with a perfect solution immediately
- Lack of a structured approach to problem-solving
Recognizing these factors is the first step in overcoming them. Now, let’s explore effective strategies to combat the blank page panic and kickstart your problem-solving process.
1. Break Down the Problem
One of the most effective ways to overcome the initial paralysis is to break the problem down into smaller, manageable parts. This approach, known as “divide and conquer,” makes even the most complex problems less intimidating.
Steps to break down a problem:
- Read the problem statement carefully
- Identify the main components or requirements
- List out these components
- Consider how these parts might interact
For example, if you’re tasked with creating a function to reverse a string, you might break it down like this:
- Input: Receive a string
- Process: Reverse the characters in the string
- Output: Return the reversed string
By breaking the problem into these steps, you’ve already given yourself a roadmap to follow, making the blank page much less intimidating.
2. Start with Pseudocode
Pseudocode is a plain language description of the steps in an algorithm. It’s an excellent way to start tackling a problem without getting bogged down in syntax details.
Benefits of using pseudocode:
- Helps organize your thoughts
- Provides a blueprint for your actual code
- Allows you to focus on logic without syntax distractions
- Makes it easier to spot potential issues early
Here’s an example of pseudocode for our string reversal problem:
function reverseString(input):
initialize empty result string
for each character in input string (starting from the end):
add character to result string
return result string
With this pseudocode, you’ve already outlined the basic structure of your solution, making it much easier to start writing the actual code.
3. Use the IOCE Method
IOCE stands for Input, Output, Constraints, and Edge Cases. This method provides a structured approach to understanding and solving coding problems.
How to apply the IOCE method:
- Input: What data is being passed into your function or program?
- Output: What should your function or program return?
- Constraints: Are there any limitations or specific requirements?
- Edge Cases: What are some extreme or unusual scenarios to consider?
Let’s apply this to our string reversal problem:
Input: A string (e.g., "hello")
Output: The reversed string (e.g., "olleh")
Constraints:
- The input string can contain any characters
- The function should work for strings of any length, including empty strings
Edge Cases:
- Empty string
- String with one character
- String with special characters or spaces
By going through this process, you’ve already started to think critically about the problem and potential challenges, giving you a clearer direction for your solution.
4. Start with a Simple Solution
When faced with a coding problem, it’s tempting to immediately try to come up with the most efficient or elegant solution. However, this approach can often lead to paralysis. Instead, start with the simplest solution you can think of, even if it’s not the most optimal.
Benefits of starting simple:
- Helps overcome initial inertia
- Provides a working solution to build upon
- Allows you to understand the problem better
- Makes it easier to identify areas for optimization
For our string reversal problem, a simple solution might look like this:
function reverseString(str) {
let reversed = '';
for (let i = str.length - 1; i >= 0; i--) {
reversed += str[i];
}
return reversed;
}
This solution may not be the most efficient, but it works and gives you a starting point. From here, you can consider optimizations or alternative approaches.
5. Use Visualization Tools
Sometimes, the blank page panic stems from difficulty visualizing the problem or solution. In such cases, using visualization tools can be incredibly helpful.
Types of visualization tools:
- Flowcharts
- Diagrams
- Whiteboarding (physical or digital)
- Scratch paper for quick sketches
These tools can help you:
- Understand the problem better
- Visualize data structures and algorithms
- Identify potential issues or edge cases
- Communicate your ideas more effectively
For our string reversal problem, a simple flowchart might look like this:
[Start] -> [Input String] -> [Initialize Empty Result] ->
[Loop Through Input from End] -> [Add Each Character to Result] ->
[Return Result] -> [End]
This visual representation can make the problem and solution much clearer, helping you overcome the initial blank page hurdle.
6. Leverage Pattern Recognition
As you gain more experience in coding, you’ll start to recognize patterns in problems. This pattern recognition can be a powerful tool in overcoming the blank page panic.
Common coding patterns:
- Two-pointer technique
- Sliding window
- Divide and conquer
- Dynamic programming
- Greedy algorithms
When you encounter a new problem, try to identify if it fits into any of these known patterns. This can give you a starting point for your solution.
For our string reversal problem, we might recognize that it can be solved using the two-pointer technique:
function reverseString(str) {
const arr = str.split('');
let left = 0;
let right = arr.length - 1;
while (left < right) {
[arr[left], arr[right]] = [arr[right], arr[left]];
left++;
right--;
}
return arr.join('');
}
By recognizing and applying this pattern, we’ve come up with an in-place reversal solution that’s more efficient than our initial approach.
7. Use Test-Driven Development (TDD)
Test-Driven Development is an approach where you write tests for your code before writing the actual code. This might seem counterintuitive when you’re facing a blank page, but it can be an excellent way to overcome initial paralysis.
Steps in TDD:
- Write a test that defines a function or improvements of a function, which should fail initially.
- Write the minimum amount of code necessary to make the test pass.
- Refactor the code to acceptable standards.
Benefits of TDD:
- Provides a clear starting point
- Helps you think about the problem in concrete terms
- Ensures your code works as expected
- Makes it easier to refactor and improve your code
For our string reversal problem, we might start with these tests:
test('reverseString reverses a simple string', () => {
expect(reverseString('hello')).toBe('olleh');
});
test('reverseString handles empty string', () => {
expect(reverseString('')).toBe('');
});
test('reverseString handles single character', () => {
expect(reverseString('a')).toBe('a');
});
test('reverseString handles string with spaces', () => {
expect(reverseString('hello world')).toBe('dlrow olleh');
});
By writing these tests first, you’ve already started to think about how your function should behave, giving you a clear direction for your implementation.
8. Use Rubber Duck Debugging
Rubber duck debugging is a method of debugging code by explaining it, line-by-line, to an inanimate object (traditionally a rubber duck). While this technique is often used for debugging, it can also be helpful when starting a new problem.
How to use rubber duck debugging:
- Get a rubber duck (or any object, or even an imaginary one)
- Explain the problem to the duck in detail
- Walk through your thought process for solving the problem
- Articulate any challenges or uncertainties you have
This process can help you:
- Clarify your understanding of the problem
- Identify gaps in your knowledge or approach
- Generate new ideas or solutions
- Overcome mental blocks
For our string reversal problem, you might explain to the duck:
“Okay, duck, we need to reverse a string. We start with a string like ‘hello’ and we want to end up with ‘olleh’. We need to figure out how to take each character from the end of the string and put it at the beginning of a new string. Or maybe we could swap the characters in place? What do you think, duck?”
This process of articulating the problem out loud can often lead to insights and help you overcome the initial blank page panic.
9. Implement Time-Boxing
Time-boxing is a time management technique where you allocate a fixed time period, called a time box, to a planned activity. This can be an effective way to overcome the paralysis that comes with facing a blank page.
How to use time-boxing:
- Set a timer for a short period (e.g., 10-15 minutes)
- Focus intensely on the problem during this time
- When the timer goes off, take a short break
- Repeat the process
Benefits of time-boxing:
- Reduces the pressure of having to solve the entire problem at once
- Helps maintain focus and avoid distractions
- Prevents overthinking and perfectionism
- Provides natural break points for reflection
For our string reversal problem, you might set a 15-minute timer to come up with a basic solution, followed by a 5-minute break, then another 15-minute session to optimize or refactor your code.
10. Leverage AI-Powered Assistance
In today’s world, AI-powered coding assistants can be valuable tools to help overcome the blank page panic. Platforms like AlgoCademy offer AI-powered assistance that can provide hints, suggest approaches, or even generate starter code.
Benefits of AI assistance:
- Provides a starting point when you’re stuck
- Offers alternative approaches you might not have considered
- Helps explain complex concepts or algorithms
- Can generate test cases to validate your solution
However, it’s important to use AI assistance as a tool for learning and problem-solving, not as a crutch. Always strive to understand the solutions it provides and use them as a stepping stone to develop your own problem-solving skills.
Conclusion
The blank page panic is a common experience for programmers of all levels, but it doesn’t have to be a roadblock in your coding journey. By employing these strategies – breaking down the problem, using pseudocode, applying the IOCE method, starting with simple solutions, using visualization tools, leveraging pattern recognition, practicing TDD, using rubber duck debugging, implementing time-boxing, and leveraging AI assistance – you can overcome this initial hurdle and approach new coding problems with confidence.
Remember, the key is to start somewhere, anywhere. Don’t let the pursuit of perfection prevent you from making progress. Each line of code you write, each problem you tackle, is a step forward in your programming journey. Embrace the challenge, stay curious, and keep coding!
Happy problem-solving!