How to Build Your Own Algorithm Rather Than Following a Pre-Built One
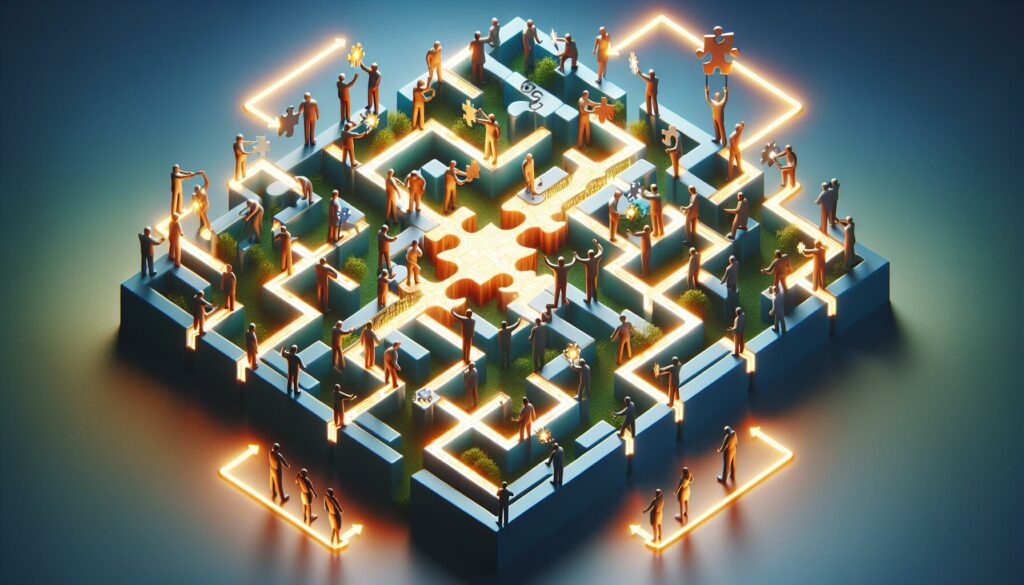
In the world of programming and computer science, algorithms are the building blocks that power our digital landscape. While it’s common to rely on pre-built algorithms for many tasks, there’s immense value in learning how to construct your own. This skill not only deepens your understanding of computational thinking but also equips you with the ability to solve unique problems that may not have off-the-shelf solutions. In this comprehensive guide, we’ll explore the process of building your own algorithm, from conception to implementation, and discuss why this skill is crucial for aspiring programmers and seasoned developers alike.
Why Build Your Own Algorithm?
Before diving into the how-to, let’s consider why you might want to build your own algorithm:
- Deeper Understanding: Creating algorithms from scratch helps you understand the underlying principles of problem-solving in computer science.
- Customization: Custom algorithms can be tailored to specific problems that pre-built solutions might not address efficiently.
- Innovation: Developing new algorithms can lead to breakthroughs in efficiency and novel approaches to problem-solving.
- Competitive Edge: In technical interviews, especially for FAANG companies, the ability to develop algorithms on the spot is highly valued.
- Flexibility: Your own algorithms can be more easily modified and optimized for different scenarios.
The Process of Building Your Own Algorithm
Let’s break down the process of creating an algorithm into manageable steps:
1. Define the Problem Clearly
The first step in building any algorithm is to have a crystal-clear understanding of the problem you’re trying to solve. This involves:
- Identifying the inputs and outputs
- Understanding any constraints or special conditions
- Defining the scope of the problem
For example, let’s say we want to create an algorithm to find the most frequent element in an array. Our problem definition might look like this:
- Input: An array of integers
- Output: The most frequent element in the array
- Constraint: If there are multiple elements with the same highest frequency, return any one of them
2. Research Existing Solutions
While the goal is to create your own algorithm, it’s always beneficial to research existing solutions. This can provide insights into different approaches and potential pitfalls. However, be cautious not to simply copy existing solutions – use them as inspiration and learning tools.
3. Brainstorm Approaches
Now comes the creative part. Think about different ways to solve the problem. Consider various data structures and algorithmic paradigms that might be applicable. For our frequency problem, we might consider:
- Using a hash map to count occurrences
- Sorting the array and counting consecutive elements
- Using a frequency array if the range of numbers is known and limited
4. Choose an Approach and Plan the Algorithm
After brainstorming, select the approach that seems most promising. For our example, let’s choose the hash map approach. Now, plan out the steps of your algorithm:
- Initialize an empty hash map
- Iterate through the array, counting occurrences of each element in the hash map
- Find the key in the hash map with the highest value
- Return that key as the most frequent element
5. Write Pseudocode
Before diving into actual code, it’s often helpful to write pseudocode. This allows you to focus on the logic without getting bogged down in syntax. Here’s how our pseudocode might look:
function findMostFrequent(array):
initialize empty hash map
for each element in array:
if element exists in hash map:
increment its count
else:
add element to hash map with count 1
initialize maxFreq = 0 and mostFreqElement = null
for each key-value pair in hash map:
if value > maxFreq:
maxFreq = value
mostFreqElement = key
return mostFreqElement
6. Implement the Algorithm
Now it’s time to translate your pseudocode into actual code. Here’s how we might implement our algorithm in Python:
def find_most_frequent(arr):
frequency = {}
for num in arr:
if num in frequency:
frequency[num] += 1
else:
frequency[num] = 1
max_freq = 0
most_freq_element = None
for num, freq in frequency.items():
if freq > max_freq:
max_freq = freq
most_freq_element = num
return most_freq_element
7. Test and Debug
After implementation, it’s crucial to test your algorithm with various inputs, including edge cases. For our frequency algorithm, we might test:
- An array with a clear most frequent element:
[1, 2, 3, 2, 2, 4, 2]
- An array with multiple elements having the same highest frequency:
[1, 2, 3, 1, 2, 3]
- An array with all elements being the same:
[5, 5, 5, 5]
- An empty array:
[]
Debug and refine your algorithm based on the test results.
8. Analyze Time and Space Complexity
Understanding the efficiency of your algorithm is crucial. Analyze its time and space complexity using Big O notation. For our hash map approach:
- Time Complexity: O(n), where n is the length of the input array (we iterate through the array once)
- Space Complexity: O(n) in the worst case, where all elements are unique
9. Optimize (if necessary)
If the performance of your algorithm doesn’t meet the requirements, consider ways to optimize it. This might involve using different data structures, eliminating redundant operations, or completely rethinking your approach.
Advanced Techniques for Algorithm Development
As you become more proficient in building algorithms, you can explore more advanced techniques:
1. Divide and Conquer
This technique involves breaking down a problem into smaller subproblems, solving them, and then combining the solutions. Famous algorithms like Merge Sort and Quick Sort use this approach.
2. Dynamic Programming
Dynamic Programming is used for optimization problems. It involves breaking down a problem into simpler subproblems and storing the results for future use. This is particularly useful for problems with overlapping subproblems and optimal substructure.
3. Greedy Algorithms
Greedy algorithms make the locally optimal choice at each step, hoping to find a global optimum. While not always guaranteed to find the best solution, they can be very efficient for certain problems.
4. Backtracking
Backtracking is used to solve problems incrementally, building candidates for the solution and abandoning those that fail to satisfy the constraints. It’s commonly used in solving puzzles like Sudoku or in problems like finding all possible subsets of a set.
Common Pitfalls to Avoid
When building your own algorithms, be aware of these common pitfalls:
- Overcomplicating the Solution: Sometimes, the simplest approach is the best. Don’t add unnecessary complexity.
- Ignoring Edge Cases: Always consider edge cases like empty inputs, single-element inputs, or inputs with special characteristics.
- Premature Optimization: Focus on getting a correct solution first, then optimize if necessary.
- Not Considering Scalability: An algorithm that works for small inputs might not scale well for larger datasets.
- Reinventing the Wheel: While building your own algorithms is valuable, recognize when using a well-established algorithm is more appropriate.
The Role of Data Structures
Choosing the right data structure is often as important as the algorithm itself. Different data structures offer different trade-offs in terms of time complexity for various operations. Common data structures to consider include:
- Arrays and Lists
- Hash Tables
- Trees (Binary Trees, AVL Trees, Red-Black Trees)
- Graphs
- Heaps
- Stacks and Queues
- Books: “Introduction to Algorithms” by Cormen, Leiserson, Rivest, and Stein is a comprehensive resource.
- Online Platforms: Websites like LeetCode, HackerRank, and CodeSignal offer algorithmic problems to practice.
- MOOCs: Courses on platforms like Coursera and edX often cover algorithm design and analysis.
- Competitive Programming: Participating in coding competitions can sharpen your algorithm development skills.
- Machine Learning: Developing custom algorithms for data preprocessing, feature selection, or model optimization.
- Financial Technology: Creating algorithms for high-frequency trading or risk assessment.
- Game Development: Designing algorithms for AI behavior, pathfinding, or procedural content generation.
- Network Routing: Optimizing data transmission in computer networks.
- Bioinformatics: Analyzing genetic sequences and protein structures.
- Regular Practice: Solve algorithmic problems regularly, even if it’s just one problem a day.
- Code Reviews: If possible, have your algorithms reviewed by peers or mentors.
- Study Classic Algorithms: Understanding classic algorithms provides insights that can be applied to new problems.
- Analyze Real-World Systems: Look at how algorithms are used in real-world applications and try to understand their design choices.
- Participate in Coding Communities: Engage with other developers through forums, local meetups, or online communities.
Understanding these data structures and when to use them is crucial for effective algorithm design.
Learning Resources for Algorithm Development
To further improve your algorithm-building skills, consider these resources:
Real-World Applications
Building your own algorithms isn’t just an academic exercise. In the real world, custom algorithms are used in various domains:
The Importance of Continuous Learning and Practice
Building algorithms is a skill that improves with practice. Here are some tips for continuous improvement:
Conclusion
Building your own algorithms is a powerful skill that goes beyond simply knowing how to code. It encompasses problem-solving, critical thinking, and creativity. While it may seem daunting at first, with practice and persistence, you can develop the ability to craft efficient, elegant solutions to complex problems.
Remember, the goal isn’t always to create something entirely new – often, it’s about adapting and combining existing ideas in novel ways to solve specific problems. As you continue to develop this skill, you’ll find that it not only makes you a better programmer but also enhances your analytical thinking in all areas of life.
So, the next time you encounter a programming challenge, resist the urge to immediately reach for a pre-built solution. Take the time to analyze the problem, design your own algorithm, and bring it to life through code. This process of creation and problem-solving is at the heart of computer science and is a skill that will serve you well throughout your programming career.