From Observation to Algorithm: How to Design a Solution Based on What You See
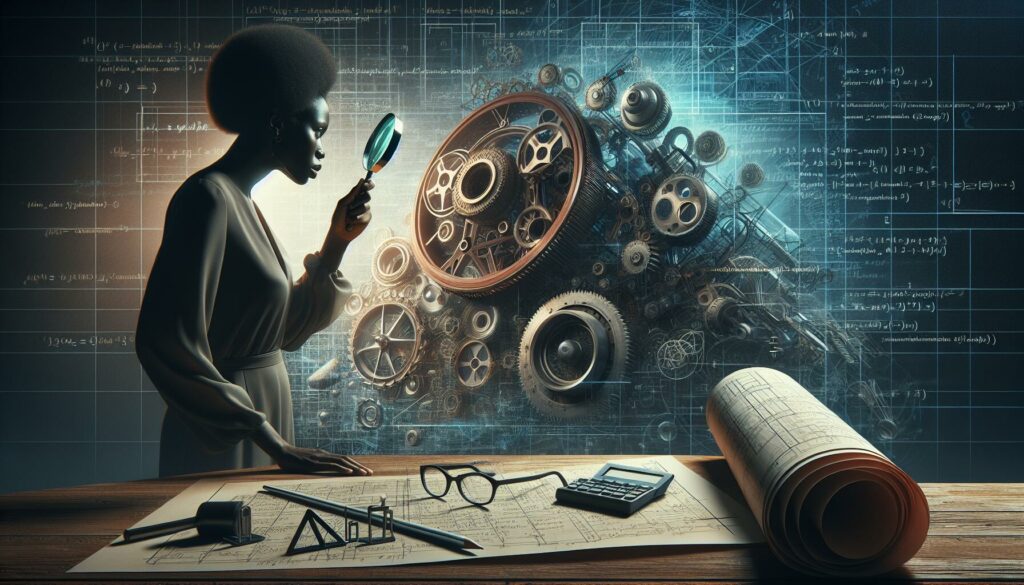
In the world of computer science and programming, the ability to transform real-world observations into efficient algorithms is a crucial skill. This process, often referred to as “algorithmic thinking,” is at the heart of problem-solving in software development. In this comprehensive guide, we’ll explore how to design solutions based on what you observe, a skill that’s invaluable for everyone from coding beginners to seasoned developers preparing for technical interviews at top tech companies.
Understanding the Importance of Observation in Algorithm Design
Before diving into the process of creating algorithms from observations, it’s essential to understand why this skill is so important:
- Real-world applicability: Many programming challenges are based on real-world scenarios. The ability to observe and analyze these scenarios is crucial for developing effective solutions.
- Efficient problem-solving: By carefully observing a problem, you can often identify patterns or shortcuts that lead to more efficient algorithms.
- Innovation: Some of the most groundbreaking algorithms have come from keen observations of natural phenomena or everyday processes.
- Interview preparation: Technical interviews, especially at major tech companies, often include questions that require candidates to design algorithms based on given scenarios.
The Process: From Observation to Algorithm
Let’s break down the process of turning an observation into an algorithm into several key steps:
1. Careful Observation and Analysis
The first step is to observe the problem or scenario carefully. This involves:
- Identifying the key elements or actors in the scenario
- Noting any patterns or repetitive actions
- Recognizing constraints or limitations
- Understanding the desired outcome or goal
For example, let’s say you’re observing a sorting process in a library. You might notice:
- Books are the key elements
- They are being compared and swapped based on their titles
- The goal is to arrange them in alphabetical order
- The constraint is that only adjacent books can be swapped
2. Abstracting the Problem
Once you’ve made your observations, the next step is to abstract the problem. This means identifying the core components and operations that are essential to solving the problem, while discarding unnecessary details.
In our library example, we might abstract the problem as follows:
- We have a list of items (books)
- Each item has a comparable property (title)
- We need to order these items based on this property
- We can only compare and swap adjacent items
3. Identifying Known Patterns or Algorithms
With the abstracted problem in mind, consider if it resembles any known algorithmic patterns or existing algorithms. This step leverages your knowledge of common algorithmic techniques and data structures.
In our sorting example, this abstraction closely resembles the problem solved by bubble sort, a simple sorting algorithm.
4. Designing the Algorithm
Now that you’ve identified a potential algorithmic approach, it’s time to design your algorithm. This involves:
- Outlining the steps of the algorithm
- Considering edge cases and potential issues
- Thinking about efficiency and potential optimizations
For our bubble sort example, the algorithm might look like this:
1. Start with the first item in the list
2. Compare it with the next item
3. If they are in the wrong order, swap them
4. Move to the next item and repeat steps 2-3
5. Once you reach the end of the list, start over from the beginning
6. Repeat this process until no swaps are needed
5. Implementing and Testing
The final step is to implement your algorithm in code and test it thoroughly. This involves:
- Writing clean, readable code
- Testing with various inputs, including edge cases
- Analyzing the time and space complexity of your solution
Here’s a simple implementation of bubble sort in Python:
def bubble_sort(arr):
n = len(arr)
for i in range(n):
for j in range(0, n-i-1):
if arr[j] > arr[j+1]:
arr[j], arr[j+1] = arr[j+1], arr[j]
return arr
# Test the algorithm
books = ["Zen", "Art", "of", "Programming"]
sorted_books = bubble_sort(books)
print(sorted_books) # Output: ["Art", "of", "Programming", "Zen"]
Common Patterns and Techniques in Algorithm Design
As you practice turning observations into algorithms, you’ll start to recognize common patterns and techniques. Here are some of the most frequently used:
1. Divide and Conquer
This technique involves breaking a problem into smaller subproblems, solving them, and then combining the results. It’s often observed in efficient sorting algorithms like merge sort and quicksort.
2. Dynamic Programming
Dynamic programming is used when a problem can be broken down into overlapping subproblems. It involves storing the results of expensive function calls and returning the cached result when the same inputs occur again.
3. Greedy Algorithms
Greedy algorithms make the locally optimal choice at each step, hoping to find a global optimum. They’re often used in optimization problems and can be observed in scenarios where immediate gain is prioritized.
4. Backtracking
Backtracking is used when you need to find all (or some) solutions to a problem. It incrementally builds candidates and abandons those that cannot possibly lead to a valid solution.
5. Graph Algorithms
Many real-world problems can be modeled as graphs. Recognizing these structures can lead to the application of well-known graph algorithms like Dijkstra’s algorithm for shortest paths or depth-first search for exploring data relationships.
Case Studies: From Observation to Algorithm
Let’s look at a couple more examples of how real-world observations can lead to algorithmic solutions:
Case Study 1: The Restaurant Waiting List
Observation: At a popular restaurant, customers put their names on a waiting list. When a table becomes available, the host calls out the next name on the list.
Abstraction: This scenario represents a first-in-first-out (FIFO) structure where the first person to arrive is the first to be seated.
Algorithm: This observation naturally leads to the implementation of a queue data structure. Here’s a simple implementation in Python:
class RestaurantQueue:
def __init__(self):
self.queue = []
def add_customer(self, name):
self.queue.append(name)
def seat_next_customer(self):
if not self.is_empty():
return self.queue.pop(0)
else:
return "No customers waiting"
def is_empty(self):
return len(self.queue) == 0
# Usage
restaurant = RestaurantQueue()
restaurant.add_customer("Alice")
restaurant.add_customer("Bob")
print(restaurant.seat_next_customer()) # Output: Alice
print(restaurant.seat_next_customer()) # Output: Bob
print(restaurant.seat_next_customer()) # Output: No customers waiting
Case Study 2: Finding the Fastest Route
Observation: A delivery driver needs to find the quickest route between multiple locations in a city, considering traffic and road conditions.
Abstraction: This scenario can be abstracted as a graph problem, where locations are nodes and roads are edges with weights representing travel time.
Algorithm: This observation leads us to consider shortest path algorithms, specifically Dijkstra’s algorithm for finding the optimal route. Here’s a simplified implementation:
import heapq
def dijkstra(graph, start, end):
distances = {node: float('infinity') for node in graph}
distances[start] = 0
pq = [(0, start)]
previous = {node: None for node in graph}
while pq:
current_distance, current_node = heapq.heappop(pq)
if current_node == end:
path = []
while current_node:
path.append(current_node)
current_node = previous[current_node]
return path[::-1], current_distance
if current_distance > distances[current_node]:
continue
for neighbor, weight in graph[current_node].items():
distance = current_distance + weight
if distance < distances[neighbor]:
distances[neighbor] = distance
previous[neighbor] = current_node
heapq.heappush(pq, (distance, neighbor))
return None, float('infinity')
# Example usage
graph = {
'A': {'B': 5, 'C': 2},
'B': {'A': 5, 'C': 1, 'D': 3},
'C': {'A': 2, 'B': 1, 'D': 6},
'D': {'B': 3, 'C': 6}
}
path, distance = dijkstra(graph, 'A', 'D')
print(f"Shortest path: {path}")
print(f"Total distance: {distance}")
Challenges in Algorithm Design from Observation
While the process of designing algorithms from observations can be powerful, it’s not without its challenges:
1. Incomplete or Misleading Observations
Real-world scenarios are often complex and may not present all relevant information upfront. It’s crucial to ask questions and seek clarification to ensure your observations are complete and accurate.
2. Overcomplicating the Solution
Sometimes, in an attempt to account for every observed detail, we might overcomplicate our algorithms. It’s important to strike a balance between comprehensiveness and simplicity.
3. Bias Towards Familiar Solutions
There’s a tendency to fit new problems into familiar patterns, which might not always be the most efficient approach. Stay open to novel solutions that might better fit the specific scenario.
4. Scalability Concerns
A solution that works well for observed small-scale scenarios might not scale efficiently. Always consider how your algorithm will perform with larger inputs.
Improving Your Observation-to-Algorithm Skills
Developing the ability to design algorithms from observations is a skill that improves with practice. Here are some strategies to enhance this skill:
1. Practice Problem Solving
Regularly engage with coding challenges and algorithmic problems. Platforms like AlgoCademy offer a wealth of problems that can help you hone your skills.
2. Study Existing Algorithms
Familiarize yourself with classic algorithms and data structures. Understanding how these work can help you recognize similar patterns in new problems.
3. Analyze Real-World Processes
Make a habit of observing and analyzing processes in your daily life. Try to think about how you would automate or optimize these processes algorithmically.
4. Collaborate and Discuss
Engage in discussions with other programmers. Explaining your thought process and hearing others’ perspectives can broaden your approach to problem-solving.
5. Implement and Experiment
Don’t just think about solutions – implement them. Coding your algorithms and testing them with various inputs can provide valuable insights and help you refine your approach.
Conclusion
The ability to design algorithms based on observations is a fundamental skill in computer science and software development. It bridges the gap between real-world problems and computational solutions, enabling us to create efficient and effective programs.
As you continue your journey in coding education and skills development, remember that this process – from careful observation to abstraction, from identifying patterns to implementing solutions – is at the core of algorithmic thinking. Whether you’re a beginner learning the basics of coding or an experienced developer preparing for technical interviews at major tech companies, honing this skill will serve you well.
Keep practicing, stay curious, and always be on the lookout for patterns and processes in the world around you. With time and experience, you’ll find that transforming observations into algorithms becomes second nature, opening up a world of problem-solving possibilities in your programming career.