The Art of Simplification: How to Break Complex Problems into Simple Steps
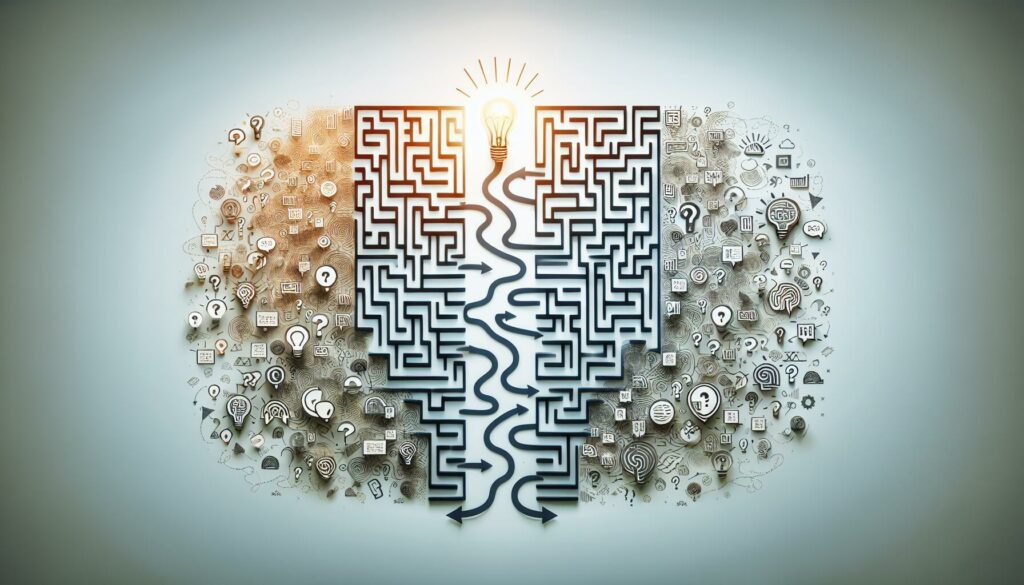
In the world of coding and software development, tackling complex problems is an everyday challenge. Whether you’re a beginner just starting your journey or an experienced programmer preparing for technical interviews at major tech companies, the ability to break down intricate problems into manageable steps is an invaluable skill. This art of simplification not only makes problem-solving more approachable but also enhances your overall coding proficiency. In this comprehensive guide, we’ll explore the techniques and strategies to master this essential skill, with a focus on how it applies to coding education and programming skills development.
Understanding the Importance of Simplification
Before diving into the specifics of how to simplify complex problems, it’s crucial to understand why this skill is so important in the realm of coding and software development.
1. Improved Problem-Solving
Breaking down complex problems into smaller, more manageable parts allows you to tackle each component individually. This approach makes the overall problem less daunting and more solvable.
2. Enhanced Code Readability and Maintainability
When you simplify problems, you’re more likely to write cleaner, more modular code. This results in improved readability and easier maintenance in the long run.
3. Better Collaboration
Simplified problems and solutions are easier to explain to team members, leading to more effective collaboration and knowledge sharing.
4. Efficient Debugging
When issues arise, having a simplified structure makes it easier to isolate and fix bugs.
5. Improved Learning and Skill Development
For those learning to code or preparing for technical interviews, breaking down complex problems helps in understanding fundamental concepts and algorithms more thoroughly.
Techniques for Breaking Down Complex Problems
Now that we understand the importance of simplification, let’s explore some effective techniques for breaking down complex problems into simple steps.
1. Understand the Problem Thoroughly
Before attempting to solve any problem, it’s crucial to fully understand what’s being asked. This involves:
- Reading the problem statement carefully
- Identifying the inputs and expected outputs
- Recognizing any constraints or special conditions
- Asking clarifying questions if anything is unclear
2. Divide and Conquer
The divide and conquer approach is a fundamental technique in problem-solving and algorithm design. It involves:
- Breaking the main problem into smaller sub-problems
- Solving each sub-problem independently
- Combining the solutions to solve the original problem
This technique is particularly useful for recursive problems and is the basis for many efficient algorithms like Merge Sort and Quick Sort.
3. Use Abstraction
Abstraction involves hiding complex implementation details behind simpler interfaces. In programming, this can be achieved through:
- Creating functions or methods that encapsulate specific tasks
- Designing classes and objects that represent complex entities
- Using appropriate naming conventions to make code self-explanatory
4. Create a Step-by-Step Plan
Before diving into coding, outline the steps you’ll need to take to solve the problem. This could involve:
- Writing pseudocode
- Creating a flowchart
- Listing the main functions or components you’ll need to implement
5. Start with a Naive Solution
Sometimes, the best way to approach a complex problem is to start with a simple, even if inefficient, solution. This helps in:
- Understanding the problem better
- Identifying potential edge cases
- Providing a baseline for optimization
6. Use Analogies and Visualizations
Complex problems can often be simplified by relating them to familiar concepts or visualizing them. This might involve:
- Drawing diagrams or sketches
- Using real-world analogies to explain abstract concepts
- Creating visual representations of data structures or algorithms
Applying Simplification Techniques in Coding Education
The art of simplification is particularly valuable in the context of coding education and skill development. Let’s explore how these techniques can be applied in learning environments like AlgoCademy.
1. Breaking Down Coding Concepts
When learning new programming concepts, it’s essential to break them down into smaller, more digestible parts. For example, when learning about Object-Oriented Programming (OOP), you might break it down as follows:
- Understanding classes and objects
- Exploring encapsulation
- Learning about inheritance
- Grasping polymorphism
By tackling each concept individually, learners can build a solid foundation before combining these ideas to understand OOP as a whole.
2. Simplifying Algorithm Implementation
When learning complex algorithms, it’s helpful to break them down into steps. Let’s take the example of implementing a binary search algorithm:
def binary_search(arr, target):
left = 0
right = len(arr) - 1
while left <= right:
mid = (left + right) // 2
if arr[mid] == target:
return mid
elif arr[mid] < target:
left = mid + 1
else:
right = mid - 1
return -1 # Target not found
To simplify this, we can break it down into steps:
- Initialize the left and right pointers
- Calculate the middle index
- Compare the middle element with the target
- Adjust the search range based on the comparison
- Repeat until the target is found or the search range is exhausted
3. Using Interactive Tutorials
Platforms like AlgoCademy often provide interactive coding tutorials that break down complex topics into smaller, manageable lessons. These tutorials might:
- Introduce concepts gradually
- Provide step-by-step guidance
- Offer immediate feedback on code submissions
- Use visualizations to explain complex ideas
4. Leveraging AI-Powered Assistance
AI-powered coding assistants can help in simplifying complex problems by:
- Suggesting simpler alternatives to complex code
- Explaining code snippets in plain language
- Breaking down multi-step solutions into more manageable parts
- Providing targeted hints and explanations
Simplification in Technical Interview Preparation
For those preparing for technical interviews, especially at major tech companies (often referred to as FAANG), the ability to simplify complex problems is crucial. Here’s how simplification techniques can be applied in this context:
1. Understanding the Problem Statement
In technical interviews, it’s essential to fully grasp the problem before attempting to solve it. This involves:
- Asking clarifying questions
- Repeating the problem in your own words to ensure understanding
- Identifying key constraints and requirements
2. Breaking Down the Solution Approach
Once you understand the problem, break down your approach into clear steps. For example, if asked to implement a function to reverse a linked list, you might break it down as follows:
- Initialize three pointers: prev, current, and next
- Traverse the list
- For each node:
- Store the next node
- Reverse the current node’s pointer
- Move prev and current one step forward
- Return the new head (prev)
3. Communicating Your Thought Process
In technical interviews, it’s not just about solving the problem, but also about demonstrating your problem-solving skills. By breaking down your approach into simple steps, you can more effectively communicate your thought process to the interviewer.
4. Handling Edge Cases
Complex problems often come with various edge cases. By simplifying the problem, you can more easily identify and handle these edge cases. For example, in the linked list reversal problem, you might consider:
- An empty list
- A list with only one node
- A list with two nodes
5. Optimizing Solutions
After implementing a basic solution, interviewers often ask for optimizations. By breaking down the problem and your initial solution, you can more easily identify areas for improvement. This might involve:
- Reducing time complexity
- Optimizing space usage
- Improving code readability
Real-World Application of Simplification in Software Development
The art of simplification extends beyond coding education and interviews; it’s a crucial skill in real-world software development. Let’s explore how these techniques apply in professional settings:
1. System Design
When designing large-scale systems, breaking down the architecture into smaller, manageable components is essential. This might involve:
- Identifying key services or microservices
- Defining clear interfaces between components
- Planning data flow and storage
- Considering scalability and fault tolerance
2. Agile Development
Agile methodologies inherently involve breaking down complex projects into smaller, manageable sprints. This simplification allows teams to:
- Focus on delivering incremental value
- Adapt to changing requirements more easily
- Identify and address issues early in the development process
3. Code Refactoring
Simplification is at the heart of code refactoring. When improving existing code, developers often:
- Break down large functions into smaller, more focused ones
- Extract common functionality into reusable components
- Simplify complex logic for better readability and maintainability
4. Debugging Complex Issues
When faced with difficult bugs, simplification techniques can be invaluable. This might involve:
- Isolating the problem to a specific component or function
- Creating a minimal reproducible example
- Breaking down the debugging process into steps (e.g., input validation, data flow analysis, output verification)
Tools and Techniques for Enhancing Simplification Skills
To further develop your ability to simplify complex problems, consider the following tools and techniques:
1. Mind Mapping
Mind mapping is a visual technique for organizing information and breaking down complex ideas. It can be particularly useful for:
- Brainstorming solution approaches
- Identifying relationships between different components of a problem
- Organizing thoughts during the planning phase
2. Rubber Duck Debugging
This technique involves explaining your code or problem to an inanimate object (traditionally a rubber duck). The process of verbalizing the problem often helps in:
- Identifying overlooked issues
- Breaking down complex logic into simpler terms
- Gaining new perspectives on the problem
3. Pseudocode and Flowcharts
Using pseudocode or flowcharts before actual coding can help in:
- Outlining the logical steps of an algorithm
- Identifying potential edge cases
- Communicating ideas with team members
4. Test-Driven Development (TDD)
TDD encourages breaking down problems into small, testable units. This approach:
- Forces you to think about the problem in discrete steps
- Helps in identifying edge cases early
- Encourages writing modular, focused code
Conclusion
The art of simplification is a cornerstone of effective problem-solving in software development. Whether you’re a beginner learning to code, a job seeker preparing for technical interviews, or an experienced developer tackling complex projects, the ability to break down problems into simple steps is invaluable.
By applying techniques like divide and conquer, abstraction, and step-by-step planning, you can approach even the most daunting challenges with confidence. Remember that simplification is not just about making things easier; it’s about gaining a deeper understanding of the problem at hand and crafting more elegant, efficient solutions.
As you continue your journey in coding education and skills development, platforms like AlgoCademy can provide valuable resources and interactive tools to help you practice and refine your simplification skills. By mastering this art, you’ll not only become a more effective problem-solver but also a more versatile and valuable software developer in today’s rapidly evolving tech landscape.
Keep practicing, stay curious, and never underestimate the power of breaking down complex problems into simple, manageable steps. Happy coding!