Why You Can’t Code if You Don’t Understand Data Structures
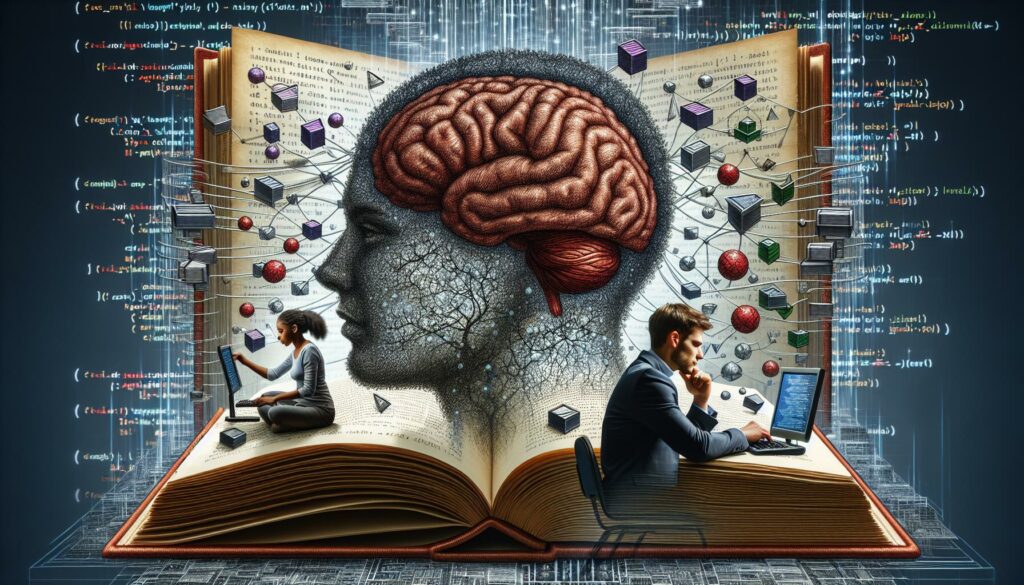
In the world of programming, data structures are the building blocks that form the foundation of efficient and effective code. They are so crucial that it’s often said, “You can’t code if you don’t understand data structures.” This statement might seem bold, but it holds a significant truth that every aspiring programmer needs to grasp. In this comprehensive guide, we’ll explore why data structures are indispensable in coding and how they impact your ability to write efficient, scalable, and maintainable software.
What Are Data Structures?
Before diving into why data structures are so important, let’s first define what they are. Data structures are specialized formats for organizing, processing, retrieving, and storing data. They provide a way to manage large amounts of data efficiently for uses such as large databases and internet indexing services. Some common examples of data structures include:
- Arrays
- Linked Lists
- Stacks
- Queues
- Trees
- Graphs
- Hash Tables
Each of these structures has its own set of characteristics, advantages, and use cases. Understanding when and how to use each one is a critical skill for any programmer.
The Impact of Data Structures on Code Efficiency
One of the primary reasons why understanding data structures is crucial is their direct impact on code efficiency. The choice of data structure can significantly affect the time and space complexity of your algorithms. Let’s look at a simple example to illustrate this point.
Suppose you need to implement a search function for a list of items. If you use an unsorted array, the search operation would have a time complexity of O(n), where n is the number of elements. This means that in the worst case, you might need to check every single element in the array.
However, if you use a binary search tree instead, you can reduce the time complexity to O(log n), which is much faster for large datasets. Here’s a simple implementation of both approaches in Python:
# Unsorted array search
def linear_search(arr, target):
for i in range(len(arr)):
if arr[i] == target:
return i
return -1
# Binary search tree search
class Node:
def __init__(self, value):
self.value = value
self.left = None
self.right = None
def bst_search(root, target):
if root is None or root.value == target:
return root
if root.value < target:
return bst_search(root.right, target)
return bst_search(root.left, target)
As you can see, the implementation and efficiency of these two search methods are quite different. Choosing the right data structure can make your code run orders of magnitude faster, especially when dealing with large amounts of data.
Data Structures and Problem-Solving
Understanding data structures is not just about writing efficient code; it’s also about problem-solving. Many complex programming problems become much simpler when you approach them with the right data structure in mind. Let’s consider a few examples:
1. Implementing a Undo/Redo Functionality
If you’re building a text editor and need to implement undo/redo functionality, a stack data structure would be perfect. Each action can be pushed onto the stack, and undoing would simply involve popping the last action off the stack.
2. Breadth-First Search in a Graph
If you need to find the shortest path between two points in a graph (think of a social network or a map), a queue would be the ideal data structure to use in a breadth-first search algorithm.
3. Implementing a Dictionary
For fast lookups in a dictionary-like structure, a hash table would be the go-to data structure, providing O(1) average time complexity for insertions and lookups.
In each of these cases, understanding the properties of different data structures allows you to quickly identify the best tool for the job, leading to more elegant and efficient solutions.
Data Structures in Technical Interviews
If you’re aiming for a career in software development, particularly at major tech companies often referred to as FAANG (Facebook, Amazon, Apple, Netflix, Google), a solid understanding of data structures is non-negotiable. These companies are known for their rigorous technical interviews, which often involve complex problem-solving tasks that require a deep understanding of data structures and algorithms.
For example, you might be asked to implement a least recently used (LRU) cache, which requires a combination of a hash map and a doubly linked list. Without a good grasp of these data structures and how they can work together, such a problem would be nearly impossible to solve within the time constraints of an interview.
Here’s a basic implementation of an LRU cache in Python:
class Node:
def __init__(self, key, value):
self.key = key
self.value = value
self.prev = None
self.next = None
class LRUCache:
def __init__(self, capacity):
self.capacity = capacity
self.cache = {}
self.head = Node(0, 0)
self.tail = Node(0, 0)
self.head.next = self.tail
self.tail.prev = self.head
def get(self, key):
if key in self.cache:
node = self.cache[key]
self._remove(node)
self._add(node)
return node.value
return -1
def put(self, key, value):
if key in self.cache:
self._remove(self.cache[key])
node = Node(key, value)
self._add(node)
self.cache[key] = node
if len(self.cache) > self.capacity:
lru = self.head.next
self._remove(lru)
del self.cache[lru.key]
def _remove(self, node):
node.prev.next = node.next
node.next.prev = node.prev
def _add(self, node):
node.prev = self.tail.prev
node.next = self.tail
self.tail.prev.next = node
self.tail.prev = node
This implementation combines a hash map (the cache
dictionary) for fast lookups and a doubly linked list to maintain the order of elements. Without understanding these data structures and how they can be used together, implementing such a cache would be extremely challenging.
Data Structures and Code Maintainability
Another crucial aspect of understanding data structures is how they contribute to code maintainability. Well-chosen data structures make your code more readable, easier to debug, and simpler to modify or extend in the future.
For instance, if you’re working with a collection of items that frequently need to be sorted, using a binary search tree or a heap instead of an array can make your code more maintainable. These structures maintain their sorted order as elements are added or removed, eliminating the need for frequent re-sorting operations.
Consider this example of maintaining a sorted list of scores:
import heapq
class ScoreTracker:
def __init__(self):
self.scores = []
def add_score(self, score):
heapq.heappush(self.scores, -score) # We use negative scores for a max heap
def get_top_k(self, k):
return [-score for score in heapq.nsmallest(k, self.scores)]
# Usage
tracker = ScoreTracker()
tracker.add_score(85)
tracker.add_score(95)
tracker.add_score(80)
print(tracker.get_top_k(2)) # Output: [95, 85]
In this example, we use a heap to efficiently maintain a sorted list of scores. This approach is much more maintainable than repeatedly sorting an array every time a new score is added or when we need to retrieve the top scores.
Data Structures and Memory Management
Understanding data structures also plays a crucial role in effective memory management. Different data structures have different memory requirements and access patterns, which can significantly impact your program’s performance, especially when dealing with large datasets or memory-constrained environments.
For example, arrays provide contiguous memory allocation, which can be beneficial for cache performance but may lead to memory fragmentation if elements are frequently inserted or deleted. On the other hand, linked lists allow for more flexible memory allocation but may have poorer cache performance due to non-contiguous memory access.
Consider this comparison of memory usage between an array and a linked list:
import sys
# Array
arr = []
for i in range(1000):
arr.append(i)
print(f"Array size: {sys.getsizeof(arr)} bytes")
# Linked List
class Node:
def __init__(self, value):
self.value = value
self.next = None
class LinkedList:
def __init__(self):
self.head = None
def append(self, value):
if not self.head:
self.head = Node(value)
return
current = self.head
while current.next:
current = current.next
current.next = Node(value)
ll = LinkedList()
for i in range(1000):
ll.append(i)
ll_size = sys.getsizeof(ll) + sum(sys.getsizeof(Node(i)) for i in range(1000))
print(f"Linked List size: {ll_size} bytes")
The output of this code will show that the linked list typically uses more memory than the array for the same number of elements. However, the linked list might be more memory-efficient in scenarios where elements are frequently inserted or deleted from the middle of the list.
Data Structures and Scalability
As your programs grow in size and complexity, the importance of choosing the right data structures becomes even more critical. Scalability is a key concern in modern software development, especially with the increasing prevalence of big data and distributed systems.
For instance, if you’re building a system that needs to handle millions of requests per second, using a simple array to store and search through data would quickly become a bottleneck. Instead, you might need to use more advanced data structures like B-trees or distributed hash tables.
Consider this example of using a B-tree for efficient data storage and retrieval:
class BTreeNode:
def __init__(self, leaf=False):
self.leaf = leaf
self.keys = []
self.child = []
class BTree:
def __init__(self, t):
self.root = BTreeNode(True)
self.t = t
def insert(self, k):
root = self.root
if len(root.keys) == (2 * self.t) - 1:
temp = BTreeNode()
self.root = temp
temp.child.insert(0, root)
self.split_child(temp, 0)
self.insert_non_full(temp, k)
else:
self.insert_non_full(root, k)
def insert_non_full(self, x, k):
i = len(x.keys) - 1
if x.leaf:
x.keys.append((None, None))
while i >= 0 and k < x.keys[i]:
x.keys[i + 1] = x.keys[i]
i -= 1
x.keys[i + 1] = k
else:
while i >= 0 and k < x.keys[i]:
i -= 1
i += 1
if len(x.child[i].keys) == (2 * self.t) - 1:
self.split_child(x, i)
if k > x.keys[i]:
i += 1
self.insert_non_full(x.child[i], k)
def split_child(self, x, i):
t = self.t
y = x.child[i]
z = BTreeNode(y.leaf)
x.child.insert(i + 1, z)
x.keys.insert(i, y.keys[t - 1])
z.keys = y.keys[t: (2 * t) - 1]
y.keys = y.keys[0: t - 1]
if not y.leaf:
z.child = y.child[t: 2 * t]
y.child = y.child[0: t - 1]
def print_tree(self, x, l=0):
print(f"Level {l}", end=": ")
for i in x.keys:
print(i, end=" ")
print()
l += 1
if len(x.child) > 0:
for i in x.child:
self.print_tree(i, l)
# Usage
B = BTree(3)
for i in range(10):
B.insert((i, 2 * i))
B.print_tree(B.root)
This B-tree implementation allows for efficient insertion and search operations even with large amounts of data, making it suitable for database systems and other applications that require handling large datasets.
Data Structures and Algorithm Design
Data structures and algorithms go hand in hand. Many advanced algorithms rely on specific data structures to achieve their efficiency. Without a solid understanding of data structures, it becomes extremely difficult to design and implement efficient algorithms.
For example, Dijkstra’s algorithm for finding the shortest path in a graph typically uses a priority queue (often implemented as a heap) to efficiently select the next node to process. Similarly, many graph algorithms use adjacency lists or matrices to represent the graph structure.
Here’s an example of Dijkstra’s algorithm using a priority queue:
import heapq
def dijkstra(graph, start):
distances = {node: float('infinity') for node in graph}
distances[start] = 0
pq = [(0, start)]
while pq:
current_distance, current_node = heapq.heappop(pq)
if current_distance > distances[current_node]:
continue
for neighbor, weight in graph[current_node].items():
distance = current_distance + weight
if distance < distances[neighbor]:
distances[neighbor] = distance
heapq.heappush(pq, (distance, neighbor))
return distances
# Example usage
graph = {
'A': {'B': 4, 'C': 2},
'B': {'D': 3, 'E': 1},
'C': {'B': 1, 'D': 5},
'D': {'E': 2},
'E': {}
}
print(dijkstra(graph, 'A'))
In this implementation, we use a priority queue (implemented with a heap) to efficiently select the node with the smallest tentative distance at each step. Without this data structure, the algorithm would be much less efficient, especially for large graphs.
Conclusion
Understanding data structures is not just a nice-to-have skill for programmers—it’s an absolute necessity. Data structures form the backbone of efficient, scalable, and maintainable code. They are crucial for problem-solving, optimizing performance, managing memory, ensuring scalability, and designing efficient algorithms.
Whether you’re a beginner just starting your coding journey or an experienced developer looking to enhance your skills, investing time in learning and mastering data structures will pay dividends throughout your programming career. It will not only make you a better coder but also open up opportunities in top tech companies known for their rigorous technical interviews.
Remember, in the world of programming, data structures are your building blocks. The better you understand them, the more impressive and efficient the structures you can build. So, dive deep into data structures, practice implementing them, and most importantly, learn to recognize which structure is best suited for each problem you encounter. Your future self—and your code—will thank you for it.