Stop Thinking About Code and Start Thinking About Processes
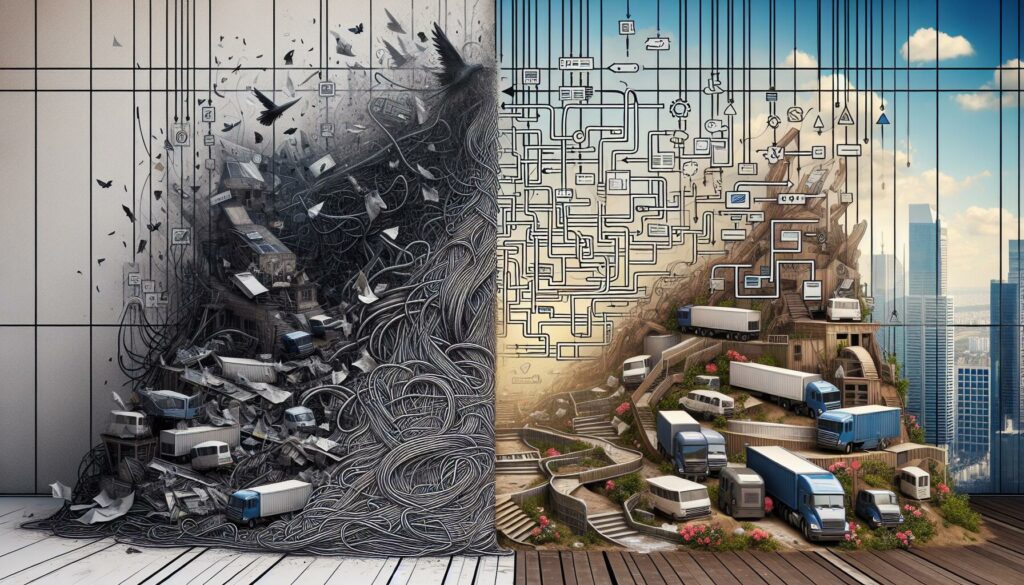
In the world of programming and software development, it’s easy to get caught up in the intricacies of code. We often find ourselves obsessing over syntax, debating the merits of different programming languages, and striving to write the most elegant and efficient lines of code possible. While these aspects are undoubtedly important, there’s a paradigm shift that can dramatically improve your effectiveness as a developer: stop thinking about code and start thinking about processes.
This approach aligns perfectly with the philosophy behind AlgoCademy, a platform dedicated to coding education and programming skills development. AlgoCademy emphasizes algorithmic thinking and problem-solving, which are at the heart of process-oriented development. By shifting our focus from code to processes, we can unlock new levels of productivity, creativity, and efficiency in our work.
The Limitations of Code-Centric Thinking
Before we delve into the benefits of process-oriented thinking, let’s examine why a code-centric approach can be limiting:
- Tunnel Vision: When we focus solely on code, we often lose sight of the bigger picture. We might create elegant solutions to small problems while missing opportunities for larger, more impactful improvements.
- Reinventing the Wheel: Code-centric thinking can lead us to write custom solutions for problems that have already been solved. This wastes time and resources.
- Difficulty in Collaboration: When team members are too focused on their individual code contributions, it can be challenging to integrate different parts of a project seamlessly.
- Resistance to Change: Developers who are overly attached to their code may resist necessary changes or improvements, hindering the evolution of the project.
- Neglecting User Needs: A focus on code can sometimes lead to losing sight of the end-user’s needs and experiences.
The Power of Process-Oriented Thinking
Now, let’s explore how shifting to a process-oriented mindset can transform your approach to development:
1. Holistic Problem Solving
When you think in terms of processes, you naturally adopt a more holistic view of the problems you’re trying to solve. Instead of jumping straight into coding, you start by asking questions like:
- What is the overall goal of this project or feature?
- How does this piece fit into the larger system?
- What are the inputs and outputs of this process?
- Who are the stakeholders involved, and what are their needs?
This broader perspective allows you to design more comprehensive and effective solutions. It’s similar to the approach taken in AlgoCademy’s interactive coding tutorials, which emphasize understanding the problem before diving into the code.
2. Improved Efficiency and Reusability
Process-oriented thinking encourages you to break down complex tasks into smaller, more manageable steps. This decomposition often reveals commonalities between different parts of your project or even between different projects entirely. By identifying these shared processes, you can:
- Create reusable components or modules
- Develop standardized workflows
- Implement design patterns that solve common problems
This approach not only saves time but also leads to more consistent and maintainable code. It’s akin to mastering algorithmic patterns in coding interviews, a key focus of AlgoCademy’s preparation resources for technical interviews at major tech companies.
3. Enhanced Collaboration and Communication
When team members think in terms of processes, it becomes easier to collaborate effectively. Process-oriented thinking provides a common language for discussing project architecture, workflow, and integration points. This shared understanding facilitates:
- More productive team discussions
- Clearer delegation of tasks
- Smoother integration of different components
- Improved documentation and knowledge sharing
This collaborative approach is reflected in AlgoCademy’s emphasis on practical coding skills and problem-solving techniques that are valuable in real-world development scenarios.
4. Adaptability and Scalability
Process-oriented thinking naturally lends itself to creating more adaptable and scalable solutions. When you design with processes in mind, you’re more likely to:
- Create modular systems that can be easily modified or extended
- Implement flexible architectures that can accommodate changing requirements
- Design scalable solutions that can handle increased load or complexity
This adaptability is crucial in today’s fast-paced tech environment, where requirements can change rapidly and systems need to scale quickly. It’s a key skill that AlgoCademy helps develop through its focus on algorithmic thinking and problem-solving strategies.
5. User-Centric Development
Process-oriented thinking naturally aligns with user-centric development practices. When you think about processes, you’re more likely to consider:
- The user’s journey through your application
- The flow of information and actions in your system
- Points of interaction and potential friction in the user experience
This user-focused approach leads to more intuitive and effective solutions, which is ultimately the goal of any development project. AlgoCademy’s emphasis on practical coding skills supports this by helping developers create solutions that are not just technically sound but also user-friendly.
Implementing Process-Oriented Thinking in Your Development Practice
Now that we’ve explored the benefits of process-oriented thinking, let’s look at some practical ways to implement this approach in your development practice:
1. Start with Flowcharts and Diagrams
Before you write a single line of code, try visualizing the process you’re trying to implement. Use flowcharts, sequence diagrams, or even simple sketches to map out the flow of data and actions in your system. This visual representation can help you identify potential issues, redundancies, or opportunities for optimization before you start coding.
For example, if you’re designing a user registration system, you might create a flowchart like this:
[User enters data] -> [Validate input] -> [Check if user exists]
|
+-> [If new user] -> [Create account] -> [Send confirmation email]
|
+-> [If existing user] -> [Show error message]
This simple diagram can guide your implementation and help you consider all the necessary steps and edge cases.
2. Use Pseudocode to Outline Processes
Before diving into actual code, try writing out your processes in pseudocode. This allows you to focus on the logic and flow of your solution without getting bogged down in syntax details. AlgoCademy’s approach to problem-solving often involves this step, encouraging learners to think through the process before implementation.
Here’s an example of pseudocode for a simple sorting algorithm:
function sortArray(array):
for each index i from 1 to length of array - 1:
key = array[i]
j = i - 1
while j >= 0 and array[j] > key:
array[j + 1] = array[j]
j = j - 1
array[j + 1] = key
return array
This pseudocode outlines the process of the insertion sort algorithm, focusing on the steps involved rather than the specific programming language syntax.
3. Implement Modular Design
Break your system down into smaller, self-contained modules or components. Each module should represent a specific process or functionality. This modular approach makes your code more manageable, reusable, and easier to test.
For instance, in a web application, you might have separate modules for:
- User authentication
- Data validation
- Database operations
- API communication
Each of these modules encapsulates a specific process, making the overall system more organized and easier to maintain.
4. Use Design Patterns
Design patterns are proven solutions to common software design problems. They represent best practices developed by experienced software developers. By thinking in terms of these established patterns, you’re adopting a process-oriented mindset that focuses on the overall structure and behavior of your system.
Some common design patterns include:
- Singleton: Ensures a class has only one instance and provides a global point of access to it.
- Observer: Defines a one-to-many dependency between objects so that when one object changes state, all its dependents are notified and updated automatically.
- Factory Method: Defines an interface for creating an object, but lets subclasses decide which class to instantiate.
AlgoCademy’s focus on algorithmic thinking aligns well with the use of design patterns, as both involve recognizing and applying proven solutions to common problems.
5. Embrace Test-Driven Development (TDD)
Test-Driven Development is a software development process that relies on the repetition of a very short development cycle. First, the developer writes a failing automated test case that defines a desired improvement or new function. Then, they produce the minimum amount of code to pass that test, and finally refactor the new code to acceptable standards.
TDD naturally encourages process-oriented thinking because it requires you to:
- Think about the desired outcome before writing code
- Break down complex functionality into testable units
- Consider edge cases and potential issues upfront
Here’s a simple example of TDD in Python:
import unittest
def add(a, b):
return a + b
class TestAddFunction(unittest.TestCase):
def test_add_positive_numbers(self):
self.assertEqual(add(2, 3), 5)
def test_add_negative_numbers(self):
self.assertEqual(add(-1, -1), -2)
def test_add_zero(self):
self.assertEqual(add(5, 0), 5)
if __name__ == '__main__':
unittest.main()
In this example, we define tests for our add
function before implementing it. This ensures that we’ve thought through different scenarios and expected outcomes before writing the actual code.
6. Document Processes, Not Just Code
When documenting your work, focus on describing the processes and workflows in addition to the code itself. This could include:
- Flowcharts or diagrams illustrating system architecture
- Written descriptions of key processes and their purposes
- Decision trees for complex logic
- User stories or use cases that describe how the system is intended to be used
This type of documentation helps maintain a process-oriented mindset and makes it easier for others (including your future self) to understand and work with your code.
7. Regular Code Reviews and Refactoring
Engage in regular code reviews and refactoring sessions with a focus on processes. During these sessions, ask questions like:
- Does this code effectively implement the intended process?
- Are there any redundant or unnecessary steps in the process?
- Could this process be simplified or optimized?
- Is this process consistent with similar processes elsewhere in the system?
This ongoing evaluation and improvement of processes helps maintain the overall quality and efficiency of your codebase.
The Role of AlgoCademy in Process-Oriented Development
AlgoCademy’s approach to coding education and skills development aligns closely with the principles of process-oriented thinking. Here’s how AlgoCademy supports this mindset:
1. Focus on Algorithmic Thinking
AlgoCademy places a strong emphasis on algorithmic thinking, which is essentially about designing step-by-step procedures for solving problems. This approach naturally encourages learners to think in terms of processes rather than just code.
2. Interactive Problem-Solving
The platform’s interactive coding tutorials guide learners through the process of breaking down complex problems into manageable steps. This approach mirrors the process-oriented thinking used in real-world software development.
3. Emphasis on Data Structures
Understanding data structures is crucial for process-oriented thinking. AlgoCademy’s focus on data structures helps learners understand how to organize and manage data efficiently, which is a key aspect of designing effective processes.
4. Preparation for Technical Interviews
AlgoCademy’s resources for preparing for technical interviews at major tech companies (often referred to as FAANG) often involve solving complex algorithmic problems. These exercises require learners to think through processes carefully, considering efficiency and scalability – key aspects of process-oriented development.
5. AI-Powered Assistance
The AI-powered assistance provided by AlgoCademy can help learners understand different approaches to solving problems. This exposure to various solution strategies encourages flexible, process-oriented thinking.
Conclusion
Shifting from a code-centric to a process-oriented mindset can significantly enhance your effectiveness as a developer. By focusing on processes, you can create more robust, scalable, and maintainable solutions. This approach aligns well with the principles taught by platforms like AlgoCademy, which emphasize algorithmic thinking and problem-solving skills.
Remember, code is ultimately just a tool for implementing processes. By understanding and optimizing these processes, you can write better code and create more effective solutions. So the next time you approach a development task, try to resist the urge to dive straight into coding. Instead, take a step back, think about the processes involved, and let that guide your implementation. Your future self (and your teammates) will thank you for it.
As you continue your journey in software development, whether through self-study, formal education, or platforms like AlgoCademy, keep this process-oriented mindset at the forefront. It will serve you well in tackling complex problems, collaborating with others, and ultimately in creating software that truly meets the needs of its users.