How to Build Algorithmic Muscle: Training Your Brain to Design Solutions from Scratch
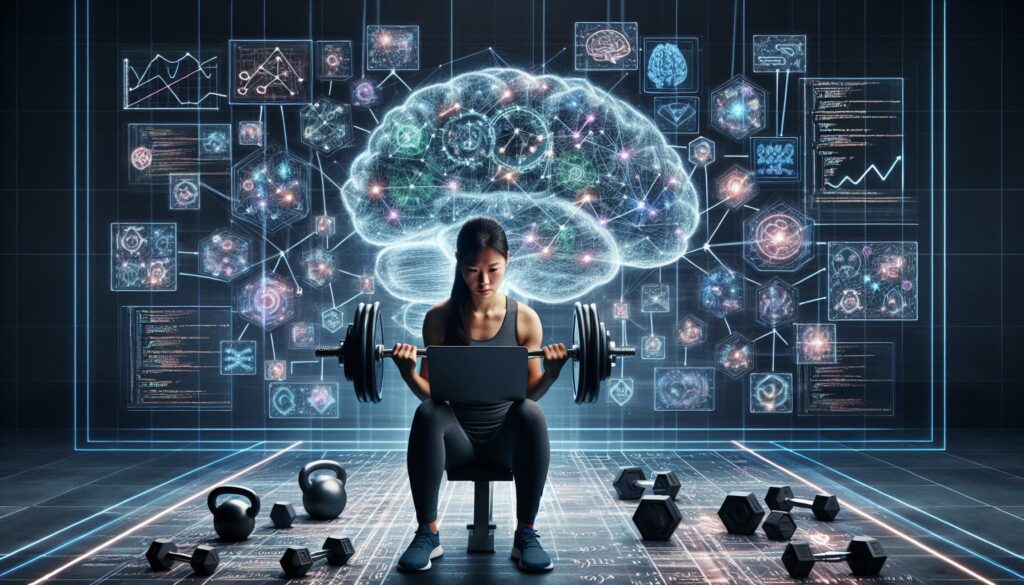
In the ever-evolving world of technology, the ability to think algorithmically and design efficient solutions is a highly sought-after skill. Whether you’re a beginner coder or an experienced developer preparing for technical interviews at major tech companies, building your algorithmic muscle is crucial. This comprehensive guide will walk you through the process of training your brain to approach problems systematically and create innovative solutions from scratch.
Understanding Algorithmic Thinking
Before diving into the techniques for building algorithmic muscle, it’s essential to understand what algorithmic thinking entails. Algorithmic thinking is a problem-solving approach that involves breaking down complex problems into smaller, manageable steps. It’s the foundation of computer science and is vital for designing efficient solutions to computational problems.
Key Components of Algorithmic Thinking
- Problem decomposition
- Pattern recognition
- Abstraction
- Algorithm design
- Evaluation and optimization
By mastering these components, you’ll be better equipped to tackle a wide range of coding challenges and design innovative solutions.
Developing a Problem-Solving Mindset
The first step in building your algorithmic muscle is to cultivate a problem-solving mindset. This involves approaching each coding challenge with curiosity and determination, rather than feeling overwhelmed or discouraged.
Tips for Developing a Problem-Solving Mindset
- Embrace challenges as opportunities for growth
- Practice patience and persistence
- Learn to break down complex problems into smaller, manageable tasks
- Cultivate a growth mindset and believe in your ability to improve
- Seek out diverse problem-solving experiences
By adopting these habits, you’ll be better prepared to tackle algorithmic challenges and design solutions from scratch.
Mastering Fundamental Data Structures and Algorithms
A solid understanding of fundamental data structures and algorithms is crucial for building your algorithmic muscle. These building blocks form the foundation of more complex solutions and are essential for efficient problem-solving.
Essential Data Structures to Master
- Arrays and Strings
- Linked Lists
- Stacks and Queues
- Trees and Graphs
- Hash Tables
- Heaps
Fundamental Algorithms to Learn
- Sorting algorithms (e.g., Quicksort, Mergesort, Heapsort)
- Searching algorithms (e.g., Binary Search, Depth-First Search, Breadth-First Search)
- Dynamic Programming
- Greedy Algorithms
- Divide and Conquer
- Recursion
To truly master these concepts, it’s important to not only understand their theoretical aspects but also implement them from scratch. This hands-on approach will deepen your understanding and improve your ability to apply these concepts to novel problems.
Practicing Problem-Solving Techniques
Regular practice is key to building your algorithmic muscle. By consistently challenging yourself with diverse problems, you’ll develop the skills and intuition needed to design solutions from scratch.
Effective Problem-Solving Techniques
- Understand the problem thoroughly
- Identify the inputs and desired outputs
- Break down the problem into smaller subproblems
- Consider multiple approaches and evaluate their trade-offs
- Implement a solution, starting with a brute-force approach if necessary
- Optimize and refine your solution
- Test your solution with various inputs, including edge cases
Applying these techniques consistently will help you develop a systematic approach to problem-solving and improve your ability to design efficient algorithms.
Leveraging Online Coding Platforms and Resources
There are numerous online platforms and resources available to help you build your algorithmic muscle. These tools provide a structured learning environment, a vast array of practice problems, and often offer community support.
Popular Coding Platforms
- LeetCode
- HackerRank
- CodeSignal
- AlgoExpert
- Project Euler
These platforms offer a wide range of problems, from beginner to advanced levels, allowing you to progressively challenge yourself and track your progress.
Educational Resources
- AlgoCademy: Offers interactive coding tutorials and AI-powered assistance
- Coursera: Provides online courses on algorithms and data structures
- MIT OpenCourseWare: Offers free access to MIT’s Introduction to Algorithms course
- GeeksforGeeks: Provides comprehensive tutorials and practice problems
Utilizing these resources can provide structure to your learning journey and expose you to a wide variety of problem-solving approaches.
Implementing Solutions from Scratch
One of the most effective ways to build your algorithmic muscle is to implement solutions from scratch. This approach forces you to think deeply about the problem and consider various implementation details.
Steps for Implementing Solutions from Scratch
- Start with a clear problem statement
- Design a high-level algorithm
- Choose appropriate data structures
- Implement the core logic
- Handle edge cases and error conditions
- Optimize for efficiency and readability
- Test thoroughly with various inputs
Let’s walk through an example of implementing a solution from scratch for a common algorithmic problem: finding the longest palindromic substring in a given string.
Problem: Longest Palindromic Substring
Given a string s, find the longest palindromic substring in s.
Solution Implementation
class Solution:
def longestPalindrome(self, s: str) -> str:
if not s:
return ""
start = 0
max_length = 1
def expand_around_center(left: int, right: int) -> int:
while left >= 0 and right < len(s) and s[left] == s[right]:
left -= 1
right += 1
return right - left - 1
for i in range(len(s)):
length1 = expand_around_center(i, i)
length2 = expand_around_center(i, i + 1)
length = max(length1, length2)
if length > max_length:
start = i - (length - 1) // 2
max_length = length
return s[start:start + max_length]
This solution uses the “expand around center” technique to find the longest palindromic substring. By implementing this solution from scratch, we gain a deeper understanding of the problem and the algorithm’s inner workings.
Analyzing Time and Space Complexity
An essential aspect of building your algorithmic muscle is learning to analyze the time and space complexity of your solutions. This skill allows you to evaluate the efficiency of your algorithms and make informed decisions about trade-offs between different approaches.
Key Concepts in Complexity Analysis
- Big O notation
- Best-case, average-case, and worst-case scenarios
- Time complexity vs. space complexity
- Amortized analysis
Practice analyzing the complexity of your solutions and comparing them to alternative approaches. This will help you develop intuition for efficient algorithm design.
Participating in Coding Competitions and Hackathons
Participating in coding competitions and hackathons is an excellent way to challenge yourself and build your algorithmic muscle in a high-pressure environment. These events often present unique problems that require creative thinking and efficient implementation.
Benefits of Participating in Coding Competitions
- Exposure to diverse problem types
- Practice working under time constraints
- Opportunity to learn from other participants
- Motivation to improve your skills
- Potential for networking and career opportunities
Some popular coding competition platforms include Codeforces, TopCoder, and Google Code Jam. Regular participation in these events can significantly accelerate your growth as a problem solver.
Studying and Implementing Advanced Algorithms
As you progress in your journey to build algorithmic muscle, it’s important to study and implement more advanced algorithms. These algorithms often provide efficient solutions to complex problems and can greatly expand your problem-solving toolkit.
Advanced Algorithms to Study
- Network Flow Algorithms (e.g., Ford-Fulkerson, Edmonds-Karp)
- String Matching Algorithms (e.g., KMP, Rabin-Karp)
- Advanced Graph Algorithms (e.g., Dijkstra’s, Bellman-Ford, Floyd-Warshall)
- Computational Geometry Algorithms
- Approximation Algorithms for NP-Hard Problems
Implementing these advanced algorithms from scratch will deepen your understanding and improve your ability to design complex solutions.
Developing a Systematic Approach to Problem-Solving
As you continue to build your algorithmic muscle, it’s crucial to develop a systematic approach to problem-solving. This approach will help you tackle new challenges more effectively and efficiently.
Steps in a Systematic Problem-Solving Approach
- Understand the problem thoroughly
- Analyze the constraints and requirements
- Brainstorm potential approaches
- Choose the most promising approach
- Design a high-level algorithm
- Implement the solution
- Test and debug
- Optimize and refine
By following this systematic approach, you’ll be better equipped to handle a wide range of algorithmic challenges.
Learning from Others and Sharing Your Knowledge
Building your algorithmic muscle is not a solitary journey. Engaging with the coding community, learning from others, and sharing your knowledge can significantly accelerate your growth.
Ways to Learn from Others and Share Knowledge
- Participate in coding forums and discussion groups
- Read and analyze other people’s solutions to coding problems
- Attend coding meetups and conferences
- Contribute to open-source projects
- Write blog posts or create videos explaining algorithms
- Mentor other learners or participate in pair programming sessions
By actively engaging with the coding community, you’ll gain new perspectives, learn about different problem-solving approaches, and reinforce your own understanding by explaining concepts to others.
Preparing for Technical Interviews
For many developers, building algorithmic muscle is closely tied to preparing for technical interviews, especially at major tech companies. While the skills you develop through consistent practice will serve you well in interviews, there are some specific strategies you can employ to maximize your chances of success.
Tips for Technical Interview Preparation
- Review common interview topics and practice relevant problems
- Master the art of thinking aloud while solving problems
- Practice writing clean, readable code on a whiteboard or in a simple text editor
- Develop strategies for handling unfamiliar problems
- Practice explaining your thought process and justifying your design decisions
- Familiarize yourself with the specific interview processes of target companies
- Conduct mock interviews with peers or mentors
Remember that technical interviews are not just about finding the correct solution, but also about demonstrating your problem-solving approach and communication skills.
Conclusion: Continuous Improvement and Lifelong Learning
Building your algorithmic muscle is an ongoing process that requires dedication, persistence, and a commitment to continuous learning. As you progress in your journey, remember that the goal is not just to solve specific problems, but to develop a robust problem-solving mindset that you can apply to any challenge you encounter.
By following the strategies outlined in this guide – from mastering fundamental concepts to implementing advanced algorithms, participating in coding competitions, and engaging with the coding community – you’ll be well on your way to becoming a skilled algorithmic thinker and problem solver.
Remember that building algorithmic muscle is not just about preparing for interviews or solving coding challenges. It’s about developing a valuable skill set that will serve you throughout your career in technology. As you continue to grow and learn, you’ll find that your ability to design efficient solutions from scratch becomes an invaluable asset in tackling real-world problems and creating innovative software solutions.
So, embrace the challenge, stay curious, and keep pushing yourself to learn and improve. With consistent effort and the right approach, you can train your brain to become a powerful algorithmic problem-solving machine, ready to tackle any coding challenge that comes your way.