The Missing Piece: Why You Need to Learn Algorithm Design Before Writing Code
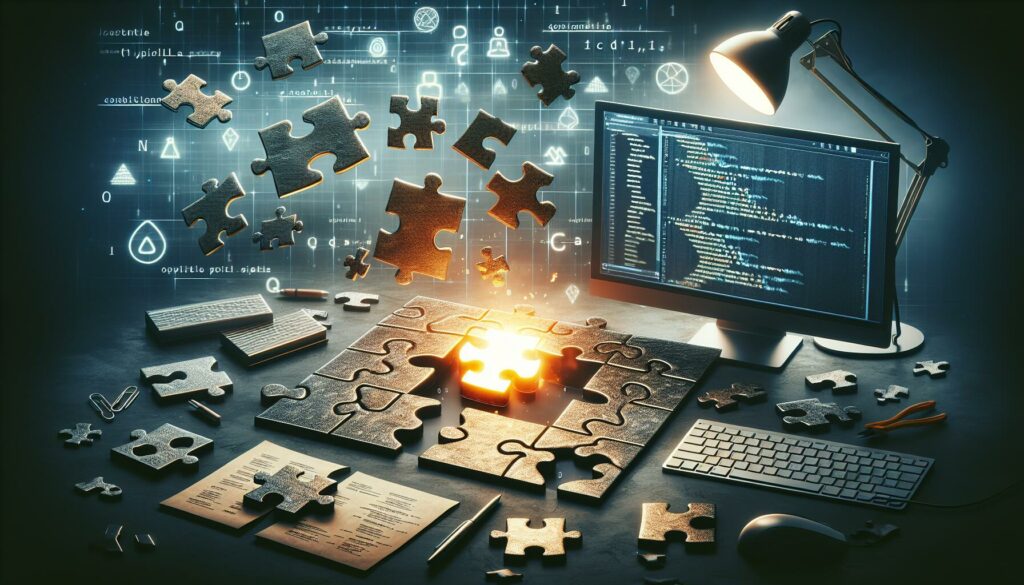
In the fast-paced world of software development, it’s easy to get caught up in the excitement of writing code and building applications. Many aspiring programmers dive headfirst into learning programming languages, frameworks, and tools, eager to see their ideas come to life on the screen. However, there’s a crucial element that often gets overlooked in this rush to code: algorithm design.
Algorithm design is the foundation upon which efficient and effective software is built. It’s the process of creating step-by-step procedures for solving problems and performing tasks. Without a solid understanding of algorithm design, even the most proficient coder may struggle to create optimal solutions to complex problems.
In this comprehensive guide, we’ll explore why learning algorithm design before diving into coding is essential for your growth as a programmer. We’ll discuss the benefits of mastering algorithm design, how it impacts your coding skills, and provide practical tips for incorporating algorithm design into your learning journey.
The Importance of Algorithm Design in Programming
Before we delve into the reasons why algorithm design should precede coding, let’s first understand what algorithm design entails and why it’s so crucial in the world of programming.
What is Algorithm Design?
Algorithm design is the process of developing a set of well-defined instructions to solve a specific problem or perform a particular task. It involves breaking down complex problems into smaller, manageable steps and determining the most efficient way to solve them. Algorithm design focuses on creating a logical sequence of operations that can be implemented in any programming language.
The Role of Algorithms in Software Development
Algorithms are the backbone of software development. They are the blueprints that guide the implementation of code and determine how efficiently a program will run. Good algorithm design leads to:
- Improved performance and efficiency
- Scalable solutions that can handle large datasets
- Cleaner, more maintainable code
- Better problem-solving skills
- Increased adaptability to different programming languages and paradigms
Now that we understand the significance of algorithm design, let’s explore why it’s crucial to learn this skill before focusing solely on writing code.
5 Reasons to Learn Algorithm Design Before Coding
1. Develop a Problem-Solving Mindset
Learning algorithm design cultivates a problem-solving mindset that is essential for success in programming. When you focus on algorithm design, you train your brain to approach problems systematically and break them down into manageable components. This skill is invaluable when tackling complex coding challenges.
By mastering algorithm design, you’ll be able to:
- Analyze problems more effectively
- Identify patterns and similarities between different problems
- Develop multiple solutions and choose the most optimal one
- Anticipate potential issues and edge cases
These skills will serve you well throughout your programming career, regardless of the specific languages or technologies you work with.
2. Write More Efficient Code
One of the primary benefits of learning algorithm design before coding is the ability to write more efficient code. When you understand the underlying principles of algorithm design, you can create solutions that are not only functional but also optimized for performance.
Consider the following example of two algorithms for finding the maximum number in an array:
// Algorithm 1: Naive approach
function findMax(arr) {
let max = arr[0];
for (let i = 1; i < arr.length; i++) {
if (arr[i] > max) {
max = arr[i];
}
}
return max;
}
// Algorithm 2: Optimized approach
function findMaxOptimized(arr) {
return Math.max(...arr);
}
While both algorithms achieve the same result, the second approach is more efficient and concise. By understanding algorithm design principles, you can identify opportunities for optimization and write code that performs better, especially when dealing with large datasets.
3. Improve Code Readability and Maintainability
Algorithm design encourages you to think about the structure and organization of your code before you start writing it. This pre-planning leads to cleaner, more readable, and more maintainable code. When you have a clear algorithm in mind, you can:
- Create well-structured functions and modules
- Use appropriate data structures
- Implement clear control flow
- Write self-documenting code
These benefits make it easier for you and other developers to understand, debug, and modify the code in the future. Consider the following example of a poorly designed algorithm versus a well-designed one:
// Poorly designed algorithm
function processData(data) {
let result = [];
for (let i = 0; i < data.length; i++) {
if (data[i] % 2 === 0) {
result.push(data[i] * 2);
} else {
result.push(data[i] * 3);
}
}
return result;
}
// Well-designed algorithm
function processData(data) {
const processEven = (num) => num * 2;
const processOdd = (num) => num * 3;
return data.map((num) => num % 2 === 0 ? processEven(num) : processOdd(num));
}
The well-designed algorithm is more readable, maintainable, and easier to modify if requirements change.
4. Enhance Problem-Solving Skills for Technical Interviews
If you’re aiming to work for top tech companies like Google, Amazon, or Facebook (often referred to as FAANG), mastering algorithm design is crucial. These companies are known for their rigorous technical interviews, which often focus heavily on algorithm design and problem-solving skills.
By learning algorithm design before diving into coding, you’ll be better prepared to:
- Analyze and solve complex problems under time pressure
- Communicate your thought process effectively
- Optimize solutions for time and space complexity
- Handle edge cases and potential issues
These skills are essential for success in technical interviews and can significantly improve your chances of landing a job at a top tech company.
5. Develop Language-Agnostic Programming Skills
One of the most significant advantages of focusing on algorithm design before coding is that it helps you develop language-agnostic programming skills. When you understand the fundamental principles of algorithm design, you can apply them to any programming language or paradigm.
This versatility is invaluable in today’s rapidly evolving tech landscape, where new programming languages and frameworks emerge regularly. By mastering algorithm design, you’ll be able to:
- Adapt quickly to new programming languages
- Understand and implement design patterns more easily
- Approach problems from multiple perspectives
- Choose the most appropriate language or tool for a given task
These skills will make you a more versatile and valuable programmer, capable of tackling a wide range of challenges across different technologies.
How to Incorporate Algorithm Design into Your Learning Journey
Now that we’ve established the importance of learning algorithm design before coding, let’s explore some practical ways to incorporate this skill into your learning journey:
1. Start with the Basics
Begin by learning the fundamental concepts of algorithm design, such as:
- Time and space complexity analysis (Big O notation)
- Common data structures (arrays, linked lists, stacks, queues, trees, graphs)
- Basic algorithmic paradigms (divide and conquer, dynamic programming, greedy algorithms)
- Sorting and searching algorithms
Resources like AlgoCademy, online courses, and textbooks can provide a solid foundation in these areas.
2. Practice Problem-Solving
Regularly engage in problem-solving exercises to hone your algorithm design skills. Platforms like LeetCode, HackerRank, and CodeSignal offer a wide range of algorithmic problems to solve. Start with easier problems and gradually work your way up to more complex ones.
3. Analyze Existing Algorithms
Study well-known algorithms and try to understand their design principles. Analyze their time and space complexity, and consider how they can be optimized or adapted to solve similar problems. This practice will help you develop a repertoire of algorithmic techniques that you can apply to new problems.
4. Implement Algorithms in Multiple Languages
Once you’ve designed an algorithm, try implementing it in different programming languages. This exercise will help you separate the algorithm design process from the coding process and reinforce the language-agnostic nature of algorithms.
5. Participate in Coding Competitions
Join coding competitions or hackathons to challenge yourself and apply your algorithm design skills in a time-constrained environment. These events can help you improve your problem-solving speed and efficiency.
6. Collaborate and Discuss
Engage in discussions with other programmers about algorithm design. Join online communities, attend meetups, or participate in study groups to share ideas and learn from others’ approaches to problem-solving.
Tools and Resources for Learning Algorithm Design
To help you get started with algorithm design, here are some valuable tools and resources:
1. Online Platforms
- AlgoCademy: Offers interactive coding tutorials and AI-powered assistance for learning algorithms and data structures.
- Coursera: Provides courses on algorithms and data structures from top universities.
- edX: Offers algorithm design courses from institutions like MIT and Harvard.
2. Books
- “Introduction to Algorithms” by Thomas H. Cormen, Charles E. Leiserson, Ronald L. Rivest, and Clifford Stein
- “Algorithms” by Robert Sedgewick and Kevin Wayne
- “Grokking Algorithms” by Aditya Bhargava
3. Visualization Tools
- VisuAlgo: Offers interactive visualizations of various algorithms and data structures.
- Algorithm Visualizer: Allows you to visualize algorithms in action and understand their inner workings.
4. Coding Practice Platforms
- LeetCode: Provides a vast collection of coding problems with a focus on algorithm design.
- HackerRank: Offers coding challenges and competitions to test your algorithm design skills.
- CodeSignal: Features a variety of coding tasks and assessments to improve your problem-solving abilities.
Common Pitfalls to Avoid When Learning Algorithm Design
As you embark on your journey to master algorithm design, be aware of these common pitfalls:
1. Focusing Too Much on Memorization
While it’s important to understand common algorithms, don’t fall into the trap of trying to memorize every algorithm you encounter. Instead, focus on understanding the underlying principles and problem-solving techniques. This approach will allow you to adapt and create new algorithms when faced with novel problems.
2. Neglecting Time and Space Complexity Analysis
It’s easy to get caught up in making an algorithm work without considering its efficiency. Always analyze the time and space complexity of your solutions and strive to optimize them when possible. This practice will help you develop more scalable and efficient algorithms.
3. Overlooking Edge Cases
When designing algorithms, it’s crucial to consider all possible inputs and edge cases. Failing to account for these scenarios can lead to bugs and errors in your code. Always test your algorithms with a variety of inputs, including extreme cases and empty sets.
4. Rushing to Code
Resist the urge to start coding immediately when faced with a problem. Take the time to fully understand the problem, consider multiple approaches, and design your algorithm before writing any code. This methodical approach will often lead to better solutions and save time in the long run.
5. Ignoring the Importance of Data Structures
Choosing the right data structure is often as important as the algorithm itself. Make sure to study and understand various data structures and their properties. The correct choice of data structure can significantly impact the efficiency of your algorithm.
Conclusion: The Path to Becoming a Better Programmer
Learning algorithm design before diving into coding is not just a recommendation—it’s a crucial step in becoming a proficient and versatile programmer. By mastering algorithm design, you’ll develop a problem-solving mindset, write more efficient code, improve code readability and maintainability, enhance your technical interview skills, and cultivate language-agnostic programming abilities.
As you progress in your programming journey, remember that algorithm design is an ongoing process of learning and improvement. Continuously challenge yourself with new problems, study advanced algorithmic techniques, and stay updated with the latest developments in the field.
By investing time and effort into learning algorithm design, you’re not just filling a gap in your skillset—you’re laying a solid foundation for a successful and rewarding career in software development. Whether you’re aiming to work at a top tech company or build your own innovative applications, the skills you gain from mastering algorithm design will prove invaluable throughout your programming journey.
So, before you rush to write your next line of code, take a step back and consider the algorithm behind it. Your future self—and your code—will thank you for it.