Turning Frustration into Progress: The Power of Incremental Problem-Solving
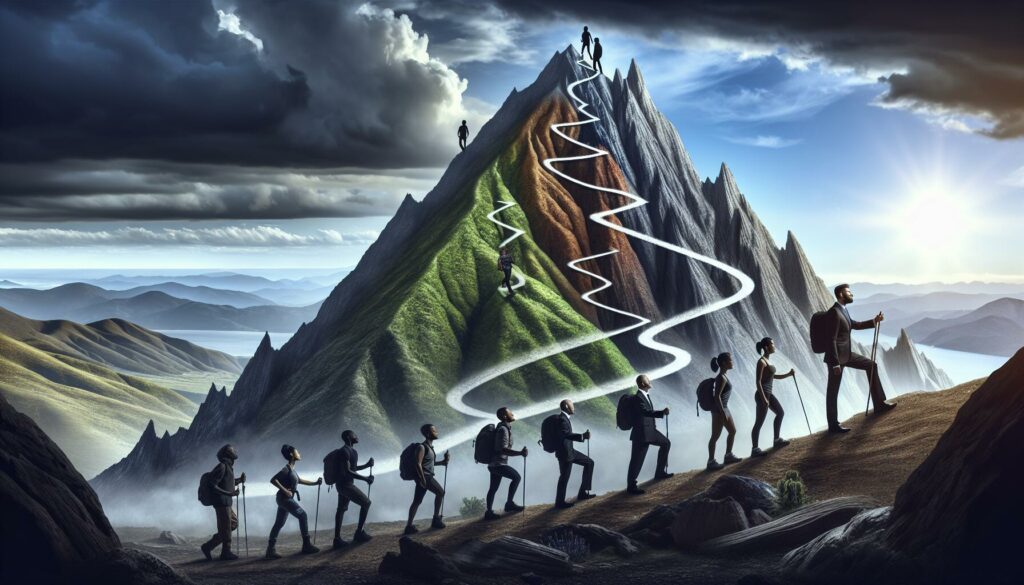
In the world of coding and programming, frustration is an all-too-familiar companion. Whether you’re a beginner grappling with your first lines of code or an experienced developer tackling complex algorithms, the journey of problem-solving in programming can often feel like an uphill battle. However, what if we told you that this frustration could be your greatest ally in your quest for coding mastery? Welcome to the realm of incremental problem-solving, where every roadblock becomes a stepping stone to success.
Understanding the Nature of Coding Frustration
Before we dive into the power of incremental problem-solving, it’s crucial to understand why coding can be so frustrating in the first place. Programming is a unique blend of logic, creativity, and precision. It requires you to think in abstract terms while adhering to strict syntactical rules. This combination can lead to several common sources of frustration:
- Syntax errors that seem impossible to track down
- Logic errors that produce unexpected results
- Complex algorithms that appear insurmountable
- Debugging processes that feel like finding a needle in a haystack
- The pressure of deadlines and performance expectations
These challenges can leave even the most seasoned programmers feeling stuck and demotivated. However, it’s precisely these moments of frustration that present the greatest opportunities for growth and learning.
The Concept of Incremental Problem-Solving
Incremental problem-solving is an approach that breaks down complex problems into smaller, more manageable pieces. Instead of trying to solve an entire problem at once, you focus on solving one small part at a time. This method is particularly effective in programming because it aligns with the modular nature of code itself.
Here’s how incremental problem-solving works in practice:
- Identify the overall problem or goal
- Break the problem down into smaller, distinct components
- Tackle each component one at a time
- Test and validate each solution
- Integrate the components to solve the larger problem
By approaching problems in this way, you transform an overwhelming task into a series of achievable goals. This not only makes the process more manageable but also provides a steady stream of small victories that can help maintain motivation and momentum.
The Benefits of Incremental Problem-Solving in Coding
Adopting an incremental approach to problem-solving in coding offers numerous benefits:
1. Reduced Cognitive Load
By focusing on one small piece of the puzzle at a time, you reduce the cognitive load on your brain. This allows you to think more clearly and creatively about each specific challenge.
2. Faster Debugging
When you write and test code in small increments, it’s much easier to identify and fix errors. You can isolate issues more quickly, leading to more efficient debugging processes.
3. Improved Learning and Retention
Solving smaller problems allows you to focus on specific concepts and techniques. This targeted learning approach often leads to better understanding and retention of programming principles.
4. Increased Motivation
Each small problem you solve is a victory. These frequent successes can boost your confidence and motivation, helping you push through more challenging aspects of your coding journey.
5. Better Code Quality
Incremental problem-solving often results in more modular, well-structured code. This can lead to improved readability, maintainability, and overall code quality.
Practical Strategies for Incremental Problem-Solving
Now that we understand the benefits, let’s explore some practical strategies for implementing incremental problem-solving in your coding practice:
1. Start with Pseudocode
Before diving into actual coding, break down your problem into steps using pseudocode. This high-level outline can serve as a roadmap for your incremental approach.
// Pseudocode example for a simple sorting algorithm
1. Input: Array of unsorted numbers
2. For each element in the array:
3. Compare with adjacent element
4. If out of order, swap
5. Repeat steps 2-4 until no more swaps are needed
6. Output: Sorted array
2. Use the “Divide and Conquer” Technique
When faced with a complex problem, divide it into smaller sub-problems. Solve each sub-problem independently, then combine the solutions to address the original problem.
3. Implement Test-Driven Development (TDD)
Write tests for each small component of your code before implementing the functionality. This ensures that you’re solving one specific problem at a time and can easily verify your progress.
// Example of a simple test in JavaScript using Jest
test("adds 1 + 2 to equal 3", () => {
expect(sum(1, 2)).toBe(3);
});
4. Utilize Version Control
Use version control systems like Git to commit your code after solving each small problem. This allows you to track your progress and easily revert changes if needed.
git add .
git commit -m "Implemented function to calculate average"
5. Leverage Coding Platforms and Tools
Take advantage of platforms like AlgoCademy that provide interactive coding tutorials and step-by-step guidance. These resources can help you break down complex problems and learn incrementally.
Overcoming Common Challenges in Incremental Problem-Solving
While incremental problem-solving is a powerful approach, it’s not without its challenges. Here are some common obstacles you might face and strategies to overcome them:
1. Losing Sight of the Big Picture
Challenge: When focusing on small components, it’s easy to lose track of the overall goal.
Solution: Regularly review your pseudocode or problem statement. Create a visual representation of your problem and its components to maintain perspective.
2. Over-Engineering Simple Solutions
Challenge: Sometimes, breaking down a problem can lead to unnecessary complexity for simple tasks.
Solution: Always ask yourself if your current approach is the simplest way to solve the problem. Don’t be afraid to refactor and simplify your code as you go.
3. Integration Difficulties
Challenge: Combining individually solved components can sometimes lead to unexpected issues.
Solution: Plan your integration steps carefully. Use interfaces or contracts between components to ensure they work together seamlessly.
4. Perfectionism Paralysis
Challenge: Trying to perfect each small component can lead to slow progress overall.
Solution: Embrace the concept of “good enough for now.” Focus on getting a working solution first, then iterate and improve.
Real-World Application: Solving a Complex Algorithmic Problem
Let’s apply the incremental problem-solving approach to a real-world coding challenge: implementing a binary search tree (BST) with various operations. This is a common problem in technical interviews and a fundamental concept in data structures.
Step 1: Define the Problem
Create a binary search tree class with methods for insertion, deletion, and searching.
Step 2: Break Down the Problem
- Create a Node class
- Implement the BST class structure
- Implement the insertion method
- Implement the search method
- Implement the deletion method
Step 3: Implement Each Component
Let’s go through each component incrementally:
1. Create a Node class
class Node {
constructor(value) {
this.value = value;
this.left = null;
this.right = null;
}
}
2. Implement the BST class structure
class BinarySearchTree {
constructor() {
this.root = null;
}
}
3. Implement the insertion method
insert(value) {
const newNode = new Node(value);
if (this.root === null) {
this.root = newNode;
return this;
}
let current = this.root;
while (true) {
if (value === current.value) return undefined;
if (value < current.value) {
if (current.left === null) {
current.left = newNode;
return this;
}
current = current.left;
} else {
if (current.right === null) {
current.right = newNode;
return this;
}
current = current.right;
}
}
}
4. Implement the search method
search(value) {
if (this.root === null) return false;
let current = this.root;
while (current) {
if (value === current.value) return true;
if (value < current.value) {
current = current.left;
} else {
current = current.right;
}
}
return false;
}
5. Implement the deletion method
The deletion method is more complex and involves several cases. We’ll implement a simplified version here:
remove(value) {
this.root = this.removeNode(this.root, value);
}
removeNode(node, value) {
if (node === null) return null;
if (value < node.value) {
node.left = this.removeNode(node.left, value);
return node;
} else if (value > node.value) {
node.right = this.removeNode(node.right, value);
return node;
} else {
// Node to delete found
// Case 1: Leaf node
if (node.left === null && node.right === null) {
return null;
}
// Case 2: Node with only one child
if (node.left === null) return node.right;
if (node.right === null) return node.left;
// Case 3: Node with two children
let tempNode = this.findMin(node.right);
node.value = tempNode.value;
node.right = this.removeNode(node.right, tempNode.value);
return node;
}
}
findMin(node) {
while (node.left !== null) {
node = node.left;
}
return node;
}
Step 4: Test Each Component
After implementing each method, it’s crucial to test it independently. Here’s an example of how you might test the insertion and search methods:
const bst = new BinarySearchTree();
bst.insert(10);
bst.insert(5);
bst.insert(15);
bst.insert(2);
bst.insert(7);
console.log(bst.search(7)); // Should return true
console.log(bst.search(9)); // Should return false
Step 5: Integrate and Test the Entire Solution
Once all components are implemented and tested individually, you can test the entire BST implementation with various operations:
const bst = new BinarySearchTree();
bst.insert(10);
bst.insert(5);
bst.insert(15);
bst.insert(2);
bst.insert(7);
console.log(bst.search(7)); // Should return true
console.log(bst.search(9)); // Should return false
bst.remove(5);
console.log(bst.search(5)); // Should return false
console.log(bst.search(7)); // Should return true
The Role of AlgoCademy in Incremental Problem-Solving
Platforms like AlgoCademy play a crucial role in fostering incremental problem-solving skills. Here’s how AlgoCademy supports this approach:
1. Structured Learning Paths
AlgoCademy provides carefully curated learning paths that break down complex programming concepts into manageable lessons. This structure naturally encourages an incremental approach to learning and problem-solving.
2. Interactive Coding Exercises
The platform offers interactive coding exercises that allow you to practice solving small, focused problems. This hands-on approach reinforces the incremental problem-solving mindset.
3. AI-Powered Assistance
AlgoCademy’s AI-powered assistance can provide hints and guidance when you’re stuck on a particular step of a problem. This feature helps you overcome obstacles without losing sight of the incremental approach.
4. Progress Tracking
The platform tracks your progress through various topics and problems, allowing you to see your incremental growth over time. This visual representation of progress can be highly motivating.
5. Community Support
AlgoCademy’s community features allow you to connect with other learners and share your incremental problem-solving strategies. This collaborative environment can provide new perspectives and approaches to breaking down complex problems.
Conclusion: Embracing the Incremental Journey
Turning frustration into progress through incremental problem-solving is not just a technique; it’s a mindset. It’s about embracing the journey of continuous improvement, celebrating small victories, and viewing challenges as opportunities for growth.
As you continue your coding journey, remember that every great programmer started with small steps. The complex algorithms and impressive projects you admire were built incrementally, one line of code at a time. By adopting this approach and leveraging resources like AlgoCademy, you’re not just learning to code; you’re developing a powerful problem-solving skill that will serve you well throughout your career.
So the next time you face a daunting coding challenge, take a deep breath, break it down, and tackle it incrementally. You’ll be surprised at how quickly frustration can turn into progress, and how those small steps can lead to giant leaps in your programming skills.
Happy coding, and remember: every line of code is a step forward on your path to mastery!