How to Develop Algorithmic Thinking Without LeetCode
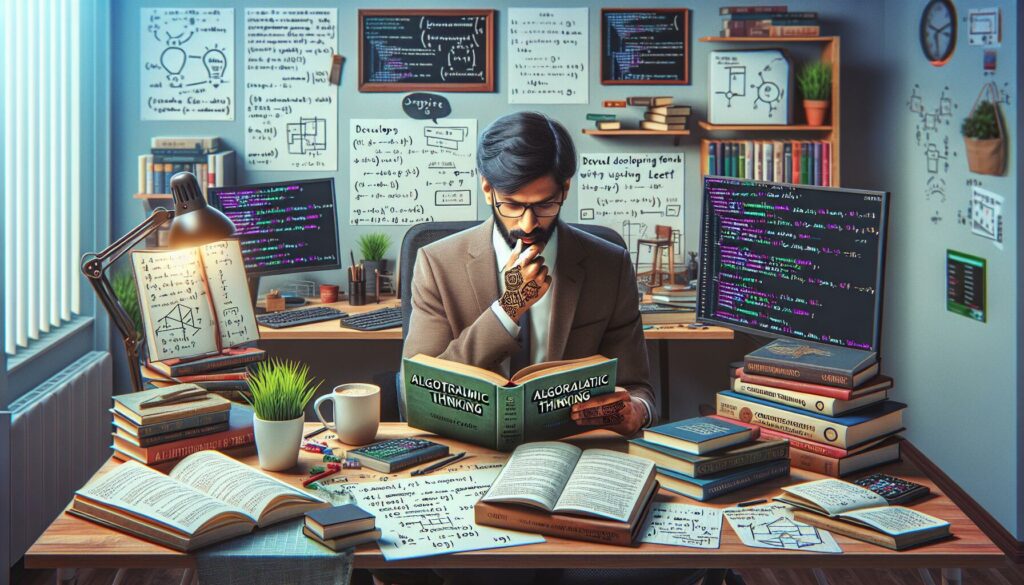
In the world of coding and software development, algorithmic thinking is a crucial skill that separates great programmers from the rest. While platforms like LeetCode have gained popularity for honing these skills, especially for technical interviews, they’re not the only path to developing strong algorithmic thinking. In this comprehensive guide, we’ll explore alternative methods and strategies to cultivate this essential skill set without relying solely on LeetCode-style problem-solving.
Understanding Algorithmic Thinking
Before diving into the methods, let’s clarify what we mean by algorithmic thinking. It’s the ability to break down complex problems into smaller, manageable steps and devise efficient solutions. This skill goes beyond mere coding; it’s about approaching problems systematically and creatively.
Key Components of Algorithmic Thinking:
- Problem decomposition
- Pattern recognition
- Abstract thinking
- Logical reasoning
- Efficiency optimization
Now that we understand what we’re aiming to develop, let’s explore various strategies to enhance these skills without relying on LeetCode.
1. Embrace Real-World Problem Solving
One of the most effective ways to develop algorithmic thinking is by tackling real-world problems. Unlike LeetCode’s often abstract puzzles, real-world scenarios provide context and practical applications for your solutions.
How to Implement:
- Personal Projects: Start a project that solves a problem you personally face. This could be a tool to automate a task or an app to organize your daily life.
- Open Source Contributions: Participate in open-source projects. This exposes you to diverse codebases and problem-solving approaches.
- Hackathons: Join hackathons where you’ll need to build solutions under time constraints, forcing you to think algorithmically and efficiently.
By engaging with real-world problems, you’ll naturally develop the ability to break down complex issues and devise step-by-step solutions – the essence of algorithmic thinking.
2. Study Classic Algorithms and Data Structures
While LeetCode focuses on applying algorithms, there’s immense value in studying the fundamentals of algorithms and data structures independently.
Approach:
- Read Classic Texts: Books like “Introduction to Algorithms” by Cormen et al. or “Algorithms” by Robert Sedgewick provide in-depth knowledge.
- Online Courses: Platforms like Coursera, edX, and MIT OpenCourseWare offer comprehensive algorithm courses.
- Implement From Scratch: Try implementing classic algorithms and data structures in your preferred programming language. This hands-on approach deepens understanding.
Understanding the theory behind algorithms equips you with the tools to approach a wide range of problems systematically.
3. Practice Pseudocode and Flowcharting
Algorithmic thinking isn’t about coding in a specific language; it’s about logical problem-solving. Pseudocode and flowcharts are excellent tools for developing this skill.
Benefits:
- Focuses on logic rather than syntax
- Improves ability to communicate ideas
- Helps visualize problem-solving steps
Exercise:
Take everyday tasks (like making a sandwich or navigating to work) and break them down into detailed pseudocode or flowcharts. This practice trains your brain to think in logical, step-by-step processes.
4. Engage in Competitive Programming (Beyond LeetCode)
While LeetCode is popular, there are other platforms for competitive programming that can enhance your algorithmic thinking.
Alternatives to Explore:
- HackerRank: Offers a wide range of programming challenges across various domains.
- CodeForces: Known for its regular coding competitions and challenging problems.
- TopCoder: Provides algorithmic challenges and hosts competitions with real-world applications.
These platforms often provide more diverse problem sets and can offer a fresh perspective on algorithmic challenges.
5. Analyze and Optimize Existing Code
Reviewing and improving existing code is an excellent way to develop algorithmic thinking. It trains you to identify inefficiencies and think about alternative approaches.
How to Practice:
- Review open-source projects on GitHub
- Participate in code reviews at work or in coding communities
- Take existing algorithms and try to optimize them for better performance
This practice not only improves your algorithmic thinking but also enhances your ability to read and understand others’ code – a crucial skill in collaborative development environments.
6. Learn and Apply Design Patterns
Design patterns are proven solutions to common problems in software design. Understanding and applying these patterns can significantly boost your algorithmic thinking.
Key Benefits:
- Provides structured approaches to problem-solving
- Enhances code organization and efficiency
- Improves ability to recognize and solve recurring problems
Resources:
- “Design Patterns: Elements of Reusable Object-Oriented Software” by the Gang of Four
- Online tutorials and courses focused on design patterns
- Practice implementing patterns in your projects
7. Explore Different Programming Paradigms
Expanding your knowledge of various programming paradigms can significantly enhance your algorithmic thinking by providing different perspectives on problem-solving.
Paradigms to Explore:
- Functional Programming: Learn languages like Haskell or F# to understand immutability and pure functions.
- Logic Programming: Try Prolog to experience declarative problem-solving.
- Object-Oriented Programming: If you’re from a functional background, dive deep into OOP principles.
Each paradigm offers unique approaches to breaking down and solving problems, broadening your algorithmic toolkit.
8. Tackle Mathematical and Logical Puzzles
Mathematical and logical puzzles are excellent for developing the abstract thinking skills crucial for algorithmic problem-solving.
Types of Puzzles:
- Sudoku and its variants
- Logic grid puzzles
- Mathematical word problems
- Chess puzzles
These puzzles train your brain to recognize patterns, think several steps ahead, and approach problems from multiple angles – all essential components of algorithmic thinking.
9. Practice Explaining Algorithms
The ability to explain an algorithm clearly is a strong indicator of understanding. It also reinforces your own grasp of the concept.
Methods to Practice:
- Teach Others: Volunteer to tutor or mentor beginners in programming.
- Write Blog Posts: Start a technical blog explaining algorithms and problem-solving techniques.
- Create Video Tutorials: Explain algorithms through video content, forcing you to break down concepts clearly.
This practice not only solidifies your understanding but also improves your communication skills – a valuable asset in any technical role.
10. Simulate Real Interview Scenarios
While we’re focusing on alternatives to LeetCode, it’s still valuable to prepare for technical interviews in a more holistic way.
Approaches:
- Mock Interviews: Practice with peers or mentors, focusing on problem-solving approach rather than just the solution.
- Whiteboard Practice: Solve problems on a whiteboard to simulate interview conditions.
- Time-Constrained Problem Solving: Set time limits for solving problems to improve efficiency under pressure.
These practices help develop not just algorithmic thinking, but also the ability to communicate your thought process effectively.
11. Analyze Algorithm Complexities
Understanding the efficiency of algorithms is crucial for developing strong algorithmic thinking skills.
Key Concepts to Master:
- Big O notation
- Time complexity analysis
- Space complexity analysis
- Best, worst, and average case scenarios
Practice Exercise:
Take algorithms you’ve implemented or studied and analyze their time and space complexities. Compare different solutions to the same problem and discuss the trade-offs.
12. Explore Graph Theory and Its Applications
Graph theory is a fundamental area of computer science with wide-ranging applications in algorithmic problem-solving.
Topics to Cover:
- Graph representations (adjacency lists, matrices)
- Graph traversal algorithms (DFS, BFS)
- Shortest path algorithms (Dijkstra’s, Bellman-Ford)
- Minimum spanning trees
- Network flow problems
Understanding graph theory provides powerful tools for modeling and solving complex problems in areas like social networks, transportation systems, and more.
13. Implement a Mini Programming Language
Creating a simple programming language or interpreter is an excellent way to deepen your understanding of algorithms and computational thinking.
Steps to Consider:
- Design the syntax and grammar of your language
- Implement a lexer and parser
- Create an abstract syntax tree (AST)
- Develop an interpreter or compiler for your language
This project touches on various aspects of computer science and forces you to think about how programming languages work at a fundamental level.
14. Solve Puzzles in Constraint Programming
Constraint programming is a paradigm where relations between variables are stated in the form of constraints. It’s particularly useful for solving complex combinatorial problems.
Example Problems:
- Scheduling problems
- Resource allocation
- Configuration puzzles
Tools like MiniZinc can be used to practice constraint programming, offering a different perspective on problem-solving compared to traditional imperative programming.
15. Implement Classic Data Structures from Scratch
While you might use built-in data structures in everyday programming, implementing them from scratch deepens your understanding of their inner workings and the algorithms that power them.
Data Structures to Implement:
- Linked Lists (singly and doubly linked)
- Stacks and Queues
- Binary Search Trees
- Hash Tables
- Heaps
- Graphs
For each implementation, consider different operations and their time complexities. This exercise not only improves your understanding of data structures but also enhances your ability to choose the right structure for specific problems.
Conclusion
Developing strong algorithmic thinking skills is a journey that extends far beyond solving LeetCode problems. By engaging in diverse activities – from tackling real-world projects to studying theoretical concepts, from competitive programming to teaching others – you can cultivate a robust and versatile approach to problem-solving.
Remember, the goal is not just to solve specific types of coding challenges, but to develop a mindset that allows you to approach any problem systematically and creatively. This holistic approach to learning will not only prepare you for technical interviews but will also make you a more effective and innovative programmer in your day-to-day work.
As you embark on this journey, keep in mind that consistency is key. Regular practice across various domains will gradually strengthen your algorithmic thinking muscles. Embrace the challenges, stay curious, and don’t be afraid to tackle problems that seem daunting at first. With time and dedication, you’ll find yourself approaching complex problems with confidence and ingenuity.
Happy coding, and may your algorithmic thinking skills continue to grow and evolve!