Why Your First Step Should Be to Break the Problem Down, Not Solve It
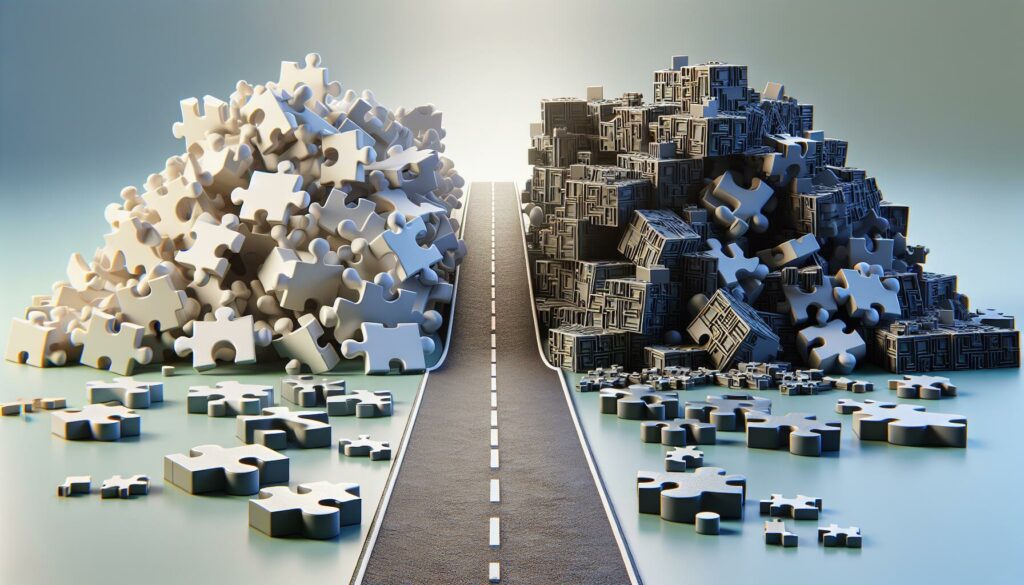
In the world of coding and programming, we often encounter complex problems that can seem overwhelming at first glance. Whether you’re a beginner just starting your journey or an experienced developer preparing for technical interviews at top tech companies, the ability to approach problems systematically is crucial. This article will explore why breaking down a problem should be your first step, rather than immediately jumping into solving it, and how this approach can significantly improve your coding skills and problem-solving abilities.
The Importance of Problem Decomposition
Problem decomposition is the process of breaking a complex problem into smaller, more manageable parts. This technique is not only essential in programming but is also a fundamental skill in algorithmic thinking and general problem-solving. Here’s why it’s so important:
- Reduces complexity: By breaking down a large problem, you make it less daunting and more approachable.
- Improves understanding: Decomposition forces you to analyze the problem thoroughly, leading to a better understanding of its components and requirements.
- Facilitates planning: It helps in creating a clear roadmap for solving the problem, making it easier to estimate time and resources needed.
- Enhances modularity: Breaking down problems often leads to more modular code, which is easier to maintain and debug.
- Promotes reusability: Smaller components are often more reusable in other contexts or problems.
The Pitfalls of Rushing to Solve
When faced with a coding challenge, especially in high-pressure situations like technical interviews, there’s a temptation to start coding immediately. However, this approach can lead to several issues:
- Overlooking important details: Rushing can cause you to miss crucial aspects of the problem.
- Inefficient solutions: Without proper analysis, you might implement a suboptimal solution.
- Difficulty in explaining: If you can’t break down the problem, it’s harder to articulate your thought process to others.
- Increased debugging time: A poorly planned solution often requires more time to debug and fix.
- Missed opportunities for optimization: Quick solutions might not consider all possibilities for efficiency improvements.
Steps to Effectively Break Down a Problem
Now that we understand the importance of problem decomposition, let’s look at a step-by-step approach to breaking down coding problems:
1. Read and Understand the Problem Statement
Take the time to thoroughly read and understand the problem statement. Identify the inputs, expected outputs, and any constraints or special conditions.
2. Identify the Main Components
Break the problem into its main components or subtasks. For example, if you’re building a sorting algorithm, you might identify components like dividing the array, comparing elements, and merging sorted portions.
3. Sketch Out a High-Level Plan
Create a rough outline of how these components will work together. This could be a flow chart, pseudocode, or even just bullet points.
4. Consider Edge Cases
Think about potential edge cases or special scenarios that your solution needs to handle. This step often reveals additional components or considerations you might have missed initially.
5. Determine Data Structures and Algorithms
For each component, consider what data structures and algorithms might be appropriate. This is where your knowledge of various coding concepts comes into play.
6. Refine and Iterate
Go back through your breakdown and refine it. You might find ways to simplify or optimize certain components.
Example: Breaking Down a String Reversal Problem
Let’s apply these steps to a common coding problem: reversing a string. While this might seem simple, breaking it down can reveal important considerations and lead to a more robust solution.
Step 1: Understand the Problem
Input: A string
Output: The same string with characters in reverse order
Constraints: Consider Unicode characters, not just ASCII
Step 2: Identify Main Components
- Handling input string
- Reversing the character order
- Handling Unicode characters
- Returning the reversed string
Step 3: High-Level Plan
- Validate input
- Convert string to an array of characters (considering Unicode)
- Reverse the array
- Join the array back into a string
- Return the result
Step 4: Consider Edge Cases
- Empty string
- String with only one character
- String with special characters or emojis
- Very long strings (consider performance)
Step 5: Determine Data Structures and Algorithms
For this problem, we can use an array to manipulate the characters. The reversal can be done using a two-pointer approach or built-in array reversal methods, depending on the language.
Step 6: Refine
After consideration, we might decide to add error handling for invalid inputs and optimize for very long strings.
Implementing the Solution
Now that we’ve broken down the problem, implementing the solution becomes much more straightforward. Here’s an example implementation in JavaScript:
function reverseString(str) {
// Validate input
if (typeof str !== 'string') {
throw new Error('Input must be a string');
}
// Handle edge cases
if (str.length <= 1) {
return str;
}
// Convert to array, reverse, and join
return [...str].reverse().join('');
}
// Test cases
console.log(reverseString('Hello, World!')); // Output: !dlroW ,olleH
console.log(reverseString('')); // Output:
console.log(reverseString('🌟')); // Output: 🌟
console.log(reverseString('a')); // Output: a
This solution handles the main components we identified, including Unicode characters and edge cases. By breaking down the problem first, we were able to create a more robust and efficient solution.
Benefits in Technical Interviews
The approach of breaking down problems before solving them is particularly valuable in technical interviews, especially for positions at major tech companies (often referred to as FAANG – Facebook, Amazon, Apple, Netflix, Google). Here’s why:
- Demonstrates thought process: Interviewers are often more interested in how you approach problems than in the final solution. Breaking down the problem allows you to showcase your analytical skills.
- Facilitates communication: By articulating your breakdown, you keep the interviewer engaged and demonstrate your ability to communicate technical concepts clearly.
- Shows thoroughness: Considering edge cases and potential optimizations during the breakdown phase shows attention to detail and a comprehensive approach to problem-solving.
- Reduces stress: Having a clear plan before coding can help reduce anxiety and make the coding portion of the interview smoother.
- Improves time management: A well-thought-out breakdown can help you manage your time more effectively during the interview.
Incorporating Problem Breakdown in Your Learning Process
To make problem breakdown a natural part of your coding process, consider incorporating these practices into your learning routine:
1. Practice with Diverse Problems
Expose yourself to a wide range of coding problems. Platforms like AlgoCademy offer a variety of challenges that can help you practice breaking down different types of problems.
2. Use Pseudocode
Before writing actual code, get into the habit of writing pseudocode. This helps you focus on the logic and structure of your solution without getting bogged down in syntax details.
3. Explain Your Approach
Practice explaining your problem breakdown out loud or to a study partner. This improves your ability to articulate your thought process, which is crucial in interviews.
4. Analyze Existing Solutions
When learning new algorithms or studying solutions to complex problems, try to reverse-engineer the problem-solving process. Ask yourself, “How might the developer have broken down this problem?”
5. Leverage AI-Powered Assistance
Tools like AI-powered coding assistants can provide guidance on breaking down problems. However, use these as learning aids rather than relying on them entirely.
6. Review and Reflect
After solving a problem, reflect on your breakdown process. Were there aspects you missed initially? How could you improve your approach next time?
Common Pitfalls to Avoid
While breaking down problems is crucial, there are some common mistakes to watch out for:
- Over-complicating: Sometimes, in an attempt to be thorough, you might break down a problem into unnecessarily small components. Strive for a balance between detail and practicality.
- Ignoring constraints: Make sure your breakdown takes into account any constraints mentioned in the problem statement, such as time or space complexity requirements.
- Failing to adapt: Be prepared to adjust your breakdown if you realize it’s not optimal as you start implementing the solution.
- Spending too much time on breakdown: While thorough analysis is important, don’t get stuck in analysis paralysis. Set a reasonable time limit for your initial breakdown.
Conclusion
Breaking down a problem before attempting to solve it is a fundamental skill in programming and problem-solving. This approach not only leads to more effective solutions but also enhances your understanding of the problem and improves your ability to communicate your thought process. Whether you’re a beginner learning to code or an experienced developer preparing for technical interviews at top tech companies, mastering the art of problem decomposition will significantly boost your coding skills and problem-solving abilities.
Remember, the goal is not just to solve the problem, but to understand it deeply and approach it systematically. By making problem breakdown your first step, you set yourself up for success in coding challenges, technical interviews, and real-world programming tasks. As you continue your journey in coding education and skills development, prioritize this crucial step in your problem-solving toolkit. With practice and persistence, breaking down problems will become second nature, paving the way for more efficient, elegant, and robust solutions in your coding endeavors.