Step Away from the Screen: How to Solve Problems with Pencil and Paper
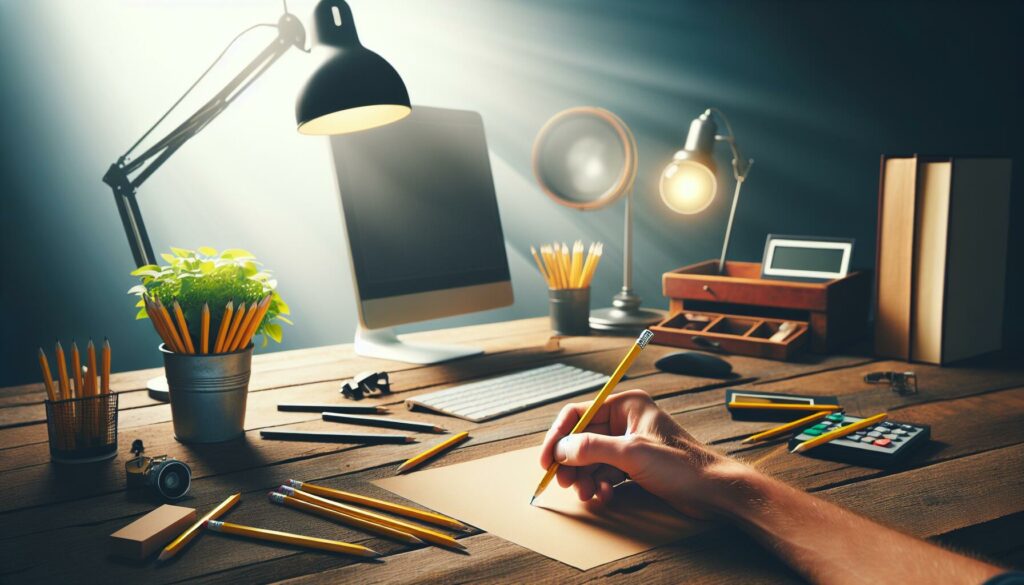
In the digital age, where coding bootcamps and online tutorials dominate the landscape of programming education, it’s easy to forget one of the most powerful tools at our disposal: the humble pencil and paper. While computers are indispensable for writing and testing code, sometimes the best way to approach complex problems is to step away from the screen and return to basics. This article will explore why and how solving problems with pencil and paper can significantly enhance your coding skills and problem-solving abilities.
The Power of Analog Problem-Solving
Before diving into the specifics of using pencil and paper for coding problems, let’s consider why this old-school approach can be so effective:
- Reduced Distractions: When you’re working on paper, there are no notifications, no temptation to check emails, and no IDE suggesting completions. It’s just you and your thoughts.
- Improved Focus: The physical act of writing engages different parts of your brain compared to typing, potentially leading to deeper concentration and retention.
- Flexibility in Representation: On paper, you’re free to draw diagrams, scribble notes, and visualize problems in ways that might be cumbersome on a computer.
- Slowing Down the Thought Process: Writing by hand is slower than typing, which can force you to think more carefully about each step of your solution.
- Enhanced Memory: Studies have shown that writing things down by hand can improve memory and understanding of concepts.
When to Use Pencil and Paper
While not every coding task requires a pencil-and-paper approach, there are several scenarios where it can be particularly beneficial:
- Algorithm Design: When you’re trying to come up with a new algorithm or optimize an existing one, sketching out your ideas on paper can help you visualize the flow and identify potential issues.
- Data Structure Visualization: Complex data structures like trees, graphs, or multi-dimensional arrays are often easier to understand and manipulate when drawn out.
- Problem Decomposition: Breaking down a large problem into smaller, manageable parts is a crucial skill in programming. Using paper to create diagrams or lists can aid in this process.
- Pseudocode Writing: Before jumping into actual code, writing pseudocode on paper can help you structure your thoughts and plan your implementation.
- Debugging: When you’re stuck on a particularly tricky bug, stepping away from the computer and walking through the problem on paper can provide new insights.
- Interview Preparation: Many technical interviews, especially for major tech companies, involve solving problems on a whiteboard. Practicing with pencil and paper can help you prepare for this format.
Techniques for Effective Paper-Based Problem-Solving
Now that we’ve established the benefits of using pencil and paper, let’s explore some techniques to make the most of this approach:
1. Diagramming
Visual representations can be incredibly powerful for understanding and solving problems. Some useful diagramming techniques include:
- Flowcharts: Ideal for representing the flow of control in an algorithm.
- Tree Diagrams: Useful for recursive problems, hierarchical data structures, or decision trees.
- State Diagrams: Help in understanding problems involving state machines or complex object lifecycles.
- Data Structure Sketches: Drawing out data structures can help you understand how they change as your algorithm progresses.
2. Pseudocode
Writing pseudocode is a great way to plan your implementation without getting bogged down in syntax details. When writing pseudocode on paper:
- Use clear, concise statements to describe each step of your algorithm.
- Include control structures like loops and conditionals.
- Don’t worry about perfect syntax – focus on the logic.
- Use indentation to show nesting and structure.
Here’s an example of how you might write pseudocode for a simple binary search algorithm:
function binarySearch(array, target):
left = 0
right = length of array - 1
while left <= right:
mid = (left + right) / 2
if array[mid] == target:
return mid
else if array[mid] < target:
left = mid + 1
else:
right = mid - 1
return -1 // Target not found
3. Test Cases
Developing test cases on paper can help you catch edge cases and verify your algorithm’s correctness. Consider:
- Normal cases: Typical inputs your algorithm should handle.
- Edge cases: Extreme or boundary conditions (e.g., empty arrays, single-element arrays).
- Error cases: Inputs that should cause your algorithm to fail or return an error.
Write out several test cases and manually trace through your algorithm to see if it produces the expected results.
4. Time and Space Complexity Analysis
Paper is an excellent medium for analyzing the efficiency of your algorithms. You can:
- Count the number of operations in your pseudocode to estimate time complexity.
- Identify nested loops or recursive calls that might lead to higher time complexity.
- List out the variables and data structures used to estimate space complexity.
- Write out the big O notation for your algorithm and simplify it step by step.
5. Problem-Solving Frameworks
Applying structured problem-solving frameworks can help you approach problems systematically. One popular framework is the UMPIRE method:
- Understand the problem
- Match the problem to potential algorithms or data structures
- Plan the algorithm
- Implement the solution (in pseudocode)
- Review the solution
- Evaluate the solution’s performance
Using paper to work through each step of this framework can help ensure you’ve thoroughly considered the problem from all angles.
Real-World Example: Solving the “Two Sum” Problem
Let’s walk through how you might use pencil and paper to solve a classic coding problem: the “Two Sum” problem. This problem asks you to find two numbers in an array that add up to a specific target sum.
Step 1: Understand the Problem
Write down the problem statement and any given constraints:
Problem: Given an array of integers and a target sum, return the indices of two numbers that add up to the target.
Input: Array of integers, target sum
Output: Two indices
Constraints:
- Each input has exactly one solution
- You may not use the same element twice
- Return the answer in any order
Step 2: Brainstorm Approaches
Sketch out potential approaches:
- Brute force: Check every pair of numbers (nested loops)
- Sorting and two-pointer technique
- Hash table for complement lookup
Step 3: Plan the Algorithm
Let’s choose the hash table approach. Write out the steps:
- Create an empty hash table
- Iterate through the array
- For each number, calculate its complement (target – number)
- If the complement is in the hash table, return current index and complement’s index
- If not, add the current number and its index to the hash table
- If no solution is found, return an indication of failure
Step 4: Implement in Pseudocode
Write out the pseudocode:
function twoSum(nums, target):
complementMap = empty hash table
for i from 0 to length of nums - 1:
complement = target - nums[i]
if complement in complementMap:
return [complementMap[complement], i]
else:
complementMap[nums[i]] = i
return [-1, -1] // No solution found
Step 5: Test with Example
Draw out a sample array and target, then manually trace through the algorithm:
nums = [2, 7, 11, 15], target = 9
Iteration 1: i = 0, nums[i] = 2
complement = 9 - 2 = 7
7 not in complementMap
Add {2: 0} to complementMap
Iteration 2: i = 1, nums[i] = 7
complement = 9 - 7 = 2
2 is in complementMap
Return [complementMap[2], 1] = [0, 1]
Step 6: Analyze Complexity
Write out the time and space complexity analysis:
Time Complexity: O(n)
- We iterate through the array once
- Hash table lookups are O(1) on average
Space Complexity: O(n)
- In the worst case, we might store n-1 elements in the hash table
Transitioning Back to the Computer
Once you’ve worked through the problem on paper, transitioning back to coding on a computer becomes much smoother. You’ll have a clear plan to follow, potential pitfalls identified, and a deeper understanding of the problem and your solution.
When you start coding:
- Implement your solution based on the pseudocode you’ve written.
- Use the test cases you’ve developed to verify your implementation.
- Refine and optimize your code as needed, referring back to your paper notes for insights.
Conclusion: The Lasting Value of Pencil and Paper
In the fast-paced world of technology, where new programming languages and frameworks seem to emerge daily, the skills developed through pencil-and-paper problem-solving remain timeless. This approach enhances your ability to think algorithmically, visualize complex structures, and approach problems methodically – skills that are valuable regardless of the specific technologies you’re working with.
Moreover, the process of working through problems on paper can help you develop a deeper intuition for algorithmic thinking. Over time, you’ll find that you can more quickly recognize patterns, anticipate edge cases, and estimate the efficiency of potential solutions.
As you progress in your coding journey, whether you’re a beginner tackling your first algorithms or an experienced developer preparing for technical interviews, don’t underestimate the power of stepping away from the screen. Keep a notebook handy, sharpen your pencils, and give your mind the space to explore problems free from the constraints of a digital environment. You might be surprised at the insights and solutions that emerge when you return to this fundamental problem-solving tool.
Remember, the goal of platforms like AlgoCademy is not just to teach you how to code, but to help you become a better problem solver and computational thinker. By incorporating pencil-and-paper techniques into your learning process, you’re developing skills that will serve you well throughout your programming career, from tackling complex algorithms to designing scalable systems.
So the next time you’re faced with a challenging coding problem, consider reaching for a pencil instead of your keyboard. Your future self – armed with clearer thoughts, better-structured solutions, and a deeper understanding of the problem at hand – will thank you.