The Art of Starting From Scratch: Learning How to Approach a Problem Without Guidance
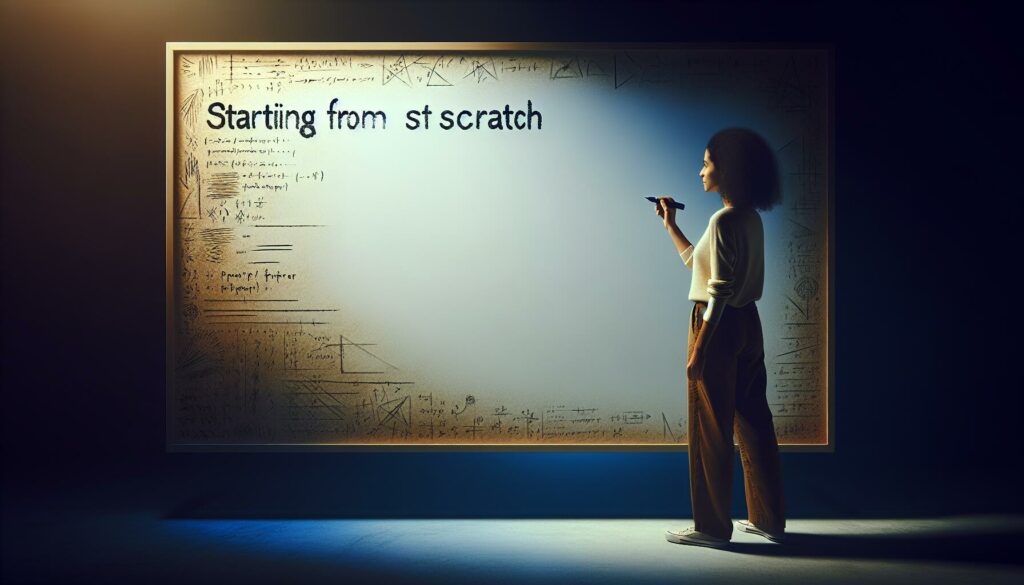
In the world of coding and software development, one of the most valuable skills you can cultivate is the ability to approach a problem from scratch, without relying on step-by-step guidance. This skill is not only crucial for passing technical interviews at top tech companies like FAANG (Facebook, Amazon, Apple, Netflix, and Google), but it’s also essential for becoming a proficient and innovative programmer. In this comprehensive guide, we’ll explore the art of starting from scratch and provide you with strategies to tackle programming challenges independently.
Why Starting From Scratch Matters
Before we dive into the techniques, let’s understand why the ability to start from scratch is so important:
- Real-world scenarios: In professional settings, you’ll often encounter unique problems that don’t have pre-existing solutions.
- Innovation: Starting from scratch fosters creativity and can lead to novel solutions that push the boundaries of what’s possible.
- Problem-solving skills: It strengthens your analytical thinking and helps you break down complex problems into manageable parts.
- Adaptability: The skill of approaching problems without guidance makes you more versatile and able to tackle a wide range of challenges.
- Interview preparation: Technical interviews, especially at FAANG companies, often require candidates to solve problems they’ve never seen before.
The Process of Starting From Scratch
Now, let’s break down the process of approaching a problem without guidance into several key steps:
1. Understand the Problem
The first and most crucial step is to fully understand the problem at hand. This involves:
- Reading the problem statement carefully, multiple times if necessary
- Identifying the inputs and expected outputs
- Clarifying any ambiguities or assumptions
- Asking questions to gather more information if needed
For example, if you’re given a problem to find the longest palindromic substring in a string, make sure you understand what a palindrome is, what constitutes a substring, and what to do in case of multiple palindromes of the same length.
2. Break Down the Problem
Once you understand the problem, break it down into smaller, more manageable sub-problems. This technique, known as “divide and conquer,” helps you tackle complex issues step by step. For our palindrome example:
- How to check if a string is a palindrome
- How to generate all possible substrings
- How to keep track of the longest palindrome found
3. Identify Patterns and Analogies
Look for patterns in the problem that might remind you of similar problems you’ve solved before. Drawing analogies can help you apply known solutions to new contexts. In our palindrome example, you might recognize that checking for palindromes is similar to comparing characters from both ends of a string, moving inwards.
4. Start with a Naive Solution
Don’t be afraid to start with a brute-force or naive solution. It’s often easier to optimize a working solution than to come up with an optimal one right away. For the palindrome problem, a naive approach might involve generating all substrings and checking each one for palindrome properties.
5. Optimize and Refine
Once you have a working solution, look for ways to optimize it. Consider:
- Time complexity: Can you reduce the number of operations?
- Space complexity: Can you use less memory?
- Edge cases: Does your solution handle all possible inputs?
For our palindrome example, you might realize that you can optimize by expanding around centers instead of generating all substrings.
6. Test and Validate
Always test your solution with various inputs, including edge cases. This helps ensure your solution is robust and correct. For the palindrome problem, test with:
- Empty strings
- Strings with no palindromes
- Strings with multiple palindromes of different lengths
- Very long strings to check for performance
Strategies for Approaching Problems Without Guidance
Now that we’ve covered the general process, let’s explore some specific strategies that can help you approach problems from scratch:
1. Visualize the Problem
Many programming problems can be better understood through visualization. Draw diagrams, flowcharts, or use visual aids to represent the problem and potential solutions. For example, in graph problems, actually drawing out the graph can provide insights into the solution.
2. Use Pseudocode
Before diving into actual coding, write out your solution in pseudocode. This allows you to focus on the logic without getting bogged down in syntax details. Here’s an example of pseudocode for our palindrome problem:
function findLongestPalindrome(string s):
longestPalindrome = ""
for each character c in s:
oddLengthPalindrome = expandAroundCenter(s, c, c)
evenLengthPalindrome = expandAroundCenter(s, c, c+1)
currentLongest = longer of oddLengthPalindrome and evenLengthPalindrome
if length of currentLongest > length of longestPalindrome:
longestPalindrome = currentLongest
return longestPalindrome
function expandAroundCenter(string s, left, right):
while left >= 0 and right < length of s and s[left] == s[right]:
left--
right++
return substring of s from left+1 to right
3. Think Aloud
Verbalize your thought process, even if you’re working alone. This can help you organize your thoughts and sometimes leads to unexpected insights. Many technical interviews also require you to think aloud, so it’s a good habit to develop.
4. Start with Examples
Work through small, concrete examples by hand. This can help you understand the problem better and may reveal patterns or edge cases you hadn’t considered. For our palindrome problem, you might start with simple strings like “racecar” or “abba” before moving to more complex cases.
5. Use Analogies
Try to relate the problem to real-world scenarios or other problems you’re familiar with. This can often provide a fresh perspective. For instance, finding the shortest path in a graph can be analogous to finding the quickest route on a map.
6. Reverse Engineering
Sometimes, it’s helpful to work backwards from the desired output to the input. This can be particularly useful for problems involving data transformations or state changes.
7. Simplify and Generalize
If the problem seems too complex, try solving a simpler version first, then gradually add complexity. For example, if you’re asked to find the kth largest element in an unsorted array, you might start by solving for the largest element, then the second largest, before generalizing to the kth largest.
Common Pitfalls to Avoid
When starting from scratch, be aware of these common pitfalls:
- Overthinking: Don’t get paralyzed by trying to find the perfect solution immediately. Start with something simple and iterate.
- Ignoring constraints: Always keep in mind the problem constraints, such as time and space complexity requirements.
- Skipping edge cases: Consider all possible inputs, including empty sets, negative numbers, or extremely large values.
- Premature optimization: Focus on getting a working solution first before optimizing. Premature optimization can lead to unnecessary complexity.
- Not testing: Always test your solution with various inputs to ensure it works correctly.
Tools and Techniques to Enhance Your Problem-Solving Skills
To improve your ability to start from scratch, consider using these tools and techniques:
1. Practice Regularly
Consistent practice is key to improving your problem-solving skills. Use platforms like AlgoCademy, LeetCode, or HackerRank to solve a variety of problems regularly.
2. Time Yourself
Practice solving problems within time constraints to simulate interview conditions and improve your efficiency.
3. Learn Data Structures and Algorithms
A strong foundation in data structures and algorithms is crucial for approaching problems from scratch. Familiarize yourself with common structures like arrays, linked lists, trees, and graphs, as well as algorithms for searching, sorting, and graph traversal.
4. Study Solution Patterns
While each problem is unique, many share common patterns. Learn and practice using techniques like two-pointer, sliding window, dynamic programming, and divide and conquer.
5. Code Review
After solving a problem, review solutions from others. This can expose you to different approaches and coding styles.
6. Mock Interviews
Participate in mock interviews where you have to solve problems in real-time without preparation. This simulates the experience of tackling unfamiliar problems under pressure.
Applying Your Skills in Real-World Scenarios
The ability to start from scratch isn’t just useful for coding interviews; it’s a valuable skill in real-world software development. Here are some scenarios where this skill is particularly important:
1. Prototyping New Features
When developing new features, you often need to create prototypes quickly without much guidance. Your ability to approach problems from scratch can help you rapidly iterate on ideas.
2. Debugging Complex Issues
When faced with a difficult bug, you may need to approach the problem from multiple angles, often without a clear starting point. The skills you develop in starting from scratch can help you navigate these challenging situations.
3. System Design
Designing large-scale systems often requires you to start with a blank slate and consider various approaches. The ability to break down complex problems and consider different solutions is crucial in system design.
4. Code Optimization
When tasked with optimizing existing code, you may need to reimagine the entire approach from scratch to achieve significant improvements.
5. Cross-functional Problem Solving
In collaborative environments, you may be called upon to solve problems outside your immediate expertise. The ability to approach unfamiliar problems methodically is invaluable in these situations.
Conclusion
The art of starting from scratch is a fundamental skill for any programmer, especially those aiming for positions at top tech companies. It involves a combination of problem-solving techniques, critical thinking, and creativity. By understanding the problem, breaking it down, and systematically working through potential solutions, you can approach even the most challenging coding problems with confidence.
Remember, the key to mastering this skill is practice. Each time you face a new problem, embrace the challenge of starting from scratch. Over time, you’ll develop a toolkit of strategies and approaches that will serve you well in both technical interviews and real-world programming scenarios.
As you continue your journey in coding education and skills development, platforms like AlgoCademy can provide valuable resources and practice opportunities. However, the true test of your abilities will come when you’re faced with a blank editor and a problem you’ve never seen before. Embrace these moments as opportunities to grow and refine your problem-solving skills.
With dedication and consistent practice, you’ll find that the art of starting from scratch becomes not just a skill, but an exciting and rewarding aspect of your programming journey. So the next time you’re faced with a challenging problem, take a deep breath, trust in your abilities, and start building your solution from the ground up. You might be surprised at what you can achieve when you approach a problem without guidance.