How to Move from Tutorial Obsession to Real Problem-Solving Skills
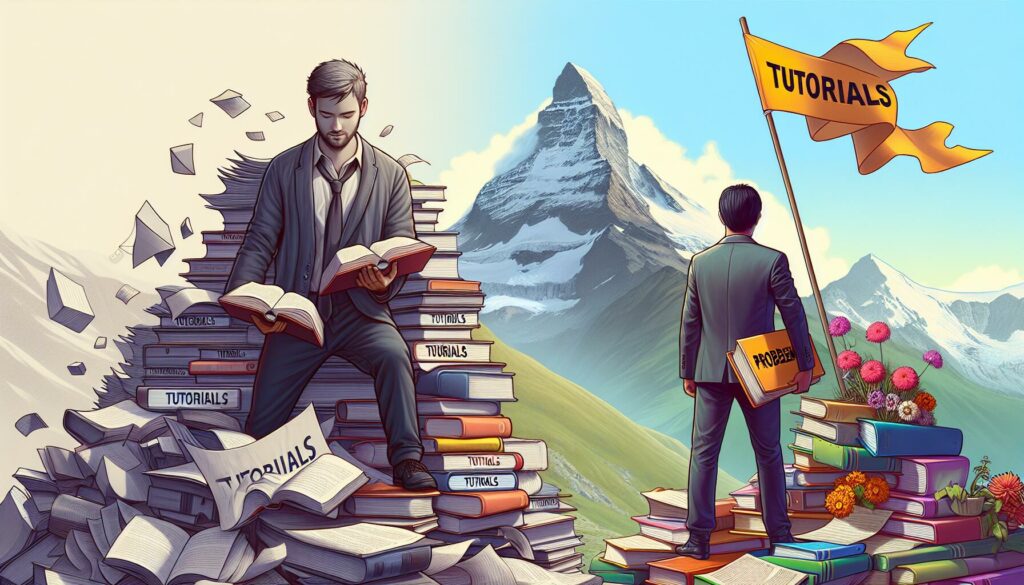
In the world of programming and software development, there’s a common phenomenon known as “tutorial hell” or “tutorial purgatory.” This is when aspiring developers find themselves stuck in an endless loop of watching tutorials, reading documentation, and following along with guided projects, but struggle to apply their knowledge to real-world problems. If you’ve ever felt like you’re spinning your wheels, constantly consuming content but not making progress, you’re not alone. In this comprehensive guide, we’ll explore how to break free from tutorial obsession and develop genuine problem-solving skills that will propel your coding career forward.
Understanding the Tutorial Trap
Before we dive into solutions, it’s essential to understand why the tutorial trap is so common and why it’s problematic:
- Comfort Zone: Tutorials provide a structured, safe environment where you’re guided step-by-step. This can be comforting, especially for beginners.
- Illusion of Progress: Completing tutorials gives a sense of accomplishment, even if you’re not truly internalizing the concepts.
- Passive Learning: Many tutorials encourage passive consumption rather than active problem-solving.
- Lack of Real-World Context: Tutorials often present idealized scenarios that don’t reflect the messiness of real-world coding challenges.
While tutorials have their place in the learning process, relying on them exclusively can hinder your growth as a developer. Let’s explore strategies to move beyond tutorials and develop robust problem-solving skills.
1. Set Clear Learning Goals
The first step in breaking free from tutorial dependence is to establish clear, actionable learning goals. Instead of vague objectives like “learn Python” or “master web development,” set specific targets:
- Build a functional e-commerce website using Django within 3 months
- Solve 50 algorithmic problems on platforms like LeetCode or HackerRank
- Contribute to an open-source project by fixing 3 issues in the next 6 weeks
Having concrete goals gives you direction and helps you focus on acquiring the skills needed to achieve them, rather than aimlessly consuming tutorials.
2. Practice Active Learning
Active learning involves engaging with the material more deeply than simply watching or reading. Here are some strategies to make your learning more active:
- Take notes: Summarize key concepts in your own words.
- Explain concepts: Try teaching what you’ve learned to someone else or writing a blog post about it.
- Code along: When following a tutorial, don’t just copy-paste. Type out the code yourself and experiment with modifications.
- Ask questions: When you encounter something you don’t understand, dig deeper. Use documentation, forums, or mentors to clarify your doubts.
3. Build Projects from Scratch
One of the most effective ways to develop problem-solving skills is to build projects from the ground up. Start with a simple idea and gradually increase complexity. Here’s a process you can follow:
- Ideation: Come up with a project idea that interests you and aligns with your learning goals.
- Planning: Break down the project into smaller, manageable tasks.
- Research: Identify the technologies and concepts you’ll need to learn.
- Implementation: Start coding, tackling one feature at a time.
- Debugging: When you encounter errors (and you will), learn to read error messages and use debugging tools.
- Iteration: Refine your code, add new features, and optimize performance.
Remember, the goal is not to create a perfect project but to learn through the process of building something from scratch.
4. Embrace the Struggle
Problem-solving skills are developed through struggle and perseverance. When you encounter a challenging problem, resist the urge to immediately look up a solution or tutorial. Instead:
- Break the problem down into smaller, more manageable parts.
- Use pseudocode to outline your approach before writing actual code.
- Experiment with different solutions, even if they’re not perfect.
- Use rubber duck debugging: explain your code and problem to an inanimate object (or patient friend) to clarify your thinking.
Remember, the struggle is where real learning happens. Embrace it as an opportunity for growth.
5. Utilize Coding Challenges and Platforms
Platforms like LeetCode, HackerRank, and CodeWars offer a wealth of coding challenges that can help you develop problem-solving skills. These platforms provide:
- A wide range of problems at various difficulty levels
- Immediate feedback on your solutions
- Access to other developers’ solutions for comparison and learning
- A structured way to practice algorithmic thinking
Start with easier problems and gradually work your way up to more complex challenges. Focus on understanding the underlying concepts rather than just finding the correct answer.
6. Join Coding Communities
Engaging with other developers can significantly accelerate your learning and problem-solving skills. Consider:
- Joining online communities like Stack Overflow, Reddit’s programming subreddits, or Discord servers focused on coding
- Participating in local meetups or coding bootcamps
- Contributing to open-source projects
- Finding a coding buddy or mentor
These interactions expose you to different perspectives, coding styles, and problem-solving approaches. They also provide opportunities to explain your code and help others, which reinforces your own understanding.
7. Develop a Problem-Solving Framework
Having a structured approach to problem-solving can make tackling new challenges less daunting. Here’s a simple framework you can adapt:
- Understand the problem: Clearly define what you’re trying to achieve.
- Plan your approach: Outline the steps you’ll take to solve the problem.
- Break it down: Divide the problem into smaller, manageable sub-problems.
- Pseudocode: Write out your solution in plain language before coding.
- Implement: Start coding your solution, focusing on one sub-problem at a time.
- Test and debug: Verify your solution works and fix any issues.
- Refactor and optimize: Look for ways to improve your code’s efficiency and readability.
- Reflect: Consider what you learned and how you might approach similar problems in the future.
Consistently applying this framework will help you approach problems more systematically and build your confidence in tackling new challenges.
8. Learn to Read and Understand Code
Developing the ability to read and understand code written by others is crucial for problem-solving. It exposes you to different coding styles, design patterns, and problem-solving approaches. Here are some ways to practice:
- Read through open-source projects on GitHub
- Study solutions to coding challenges on platforms like LeetCode
- Review pull requests in collaborative projects
- Participate in code reviews at work or in open-source communities
As you read code, ask yourself:
- What is this code trying to accomplish?
- How does it work?
- Why did the developer choose this particular approach?
- How might I have solved this problem differently?
9. Implement Data Structures and Algorithms from Scratch
While it’s important to know how to use built-in data structures and algorithms, implementing them from scratch can deepen your understanding and improve your problem-solving skills. Try building:
- Linked lists
- Stacks and queues
- Binary search trees
- Hash tables
- Sorting algorithms (e.g., bubble sort, merge sort, quicksort)
- Graph algorithms (e.g., depth-first search, breadth-first search)
This exercise forces you to think about the underlying mechanics of these fundamental computer science concepts, which can inform your approach to a wide range of programming problems.
10. Practice Explaining Your Code
Being able to articulate your thought process and explain your code is a valuable skill that reinforces your understanding and improves your problem-solving abilities. Try:
- Writing comments that explain the “why” behind your code, not just the “what”
- Documenting your projects with clear README files
- Recording yourself explaining a piece of code or a problem-solving approach
- Pair programming with a friend or colleague
- Answering questions on forums like Stack Overflow
The process of explaining forces you to clarify your thinking and often reveals gaps in your understanding that you can then address.
11. Learn to Use Debugging Tools Effectively
Debugging is an essential part of problem-solving in programming. Familiarize yourself with debugging tools and techniques:
- Learn to use your IDE’s built-in debugger
- Practice using logging statements effectively
- Understand how to read and interpret error messages
- Learn about common debugging techniques like binary search debugging
Effective debugging not only helps you fix issues but also deepens your understanding of how your code works.
12. Cultivate a Growth Mindset
Finally, developing strong problem-solving skills requires persistence and a willingness to learn from failures. Cultivate a growth mindset by:
- Viewing challenges as opportunities for growth rather than obstacles
- Celebrating small victories and progress
- Learning from mistakes and failed attempts
- Seeking feedback and constructive criticism
- Staying curious and always looking for new things to learn
Remember that becoming a skilled problem solver is a journey, not a destination. Every problem you tackle, whether you solve it immediately or struggle with it for days, is an opportunity to grow and improve.
Conclusion
Moving from tutorial obsession to real problem-solving skills is a crucial step in your development as a programmer. It requires a shift in mindset and approach, from passive consumption to active engagement with coding challenges. By setting clear goals, building projects from scratch, embracing the struggle of problem-solving, and consistently practicing, you can develop the skills and confidence to tackle real-world programming challenges.
Remember, the key is to start small and gradually increase the complexity of the problems you tackle. Don’t be afraid to make mistakes – they’re an essential part of the learning process. With persistence and the right approach, you’ll find yourself moving beyond tutorials and developing the robust problem-solving skills that are the hallmark of successful developers.
As you embark on this journey, platforms like AlgoCademy can be valuable resources. They offer interactive coding tutorials and tools that bridge the gap between guided learning and independent problem-solving. Use these resources as stepping stones, combining them with the strategies outlined in this guide to build a solid foundation in algorithmic thinking and practical coding skills.
The road from tutorial dependence to problem-solving proficiency may be challenging, but it’s also incredibly rewarding. Each problem you solve independently is a step towards becoming the confident, skilled developer you aspire to be. So, take that first step today – choose a project, tackle a coding challenge, or contribute to an open-source project. Your future self will thank you for the skills and confidence you’re building now.