From Theory to Practice: How to Turn Watched Code into Written Code
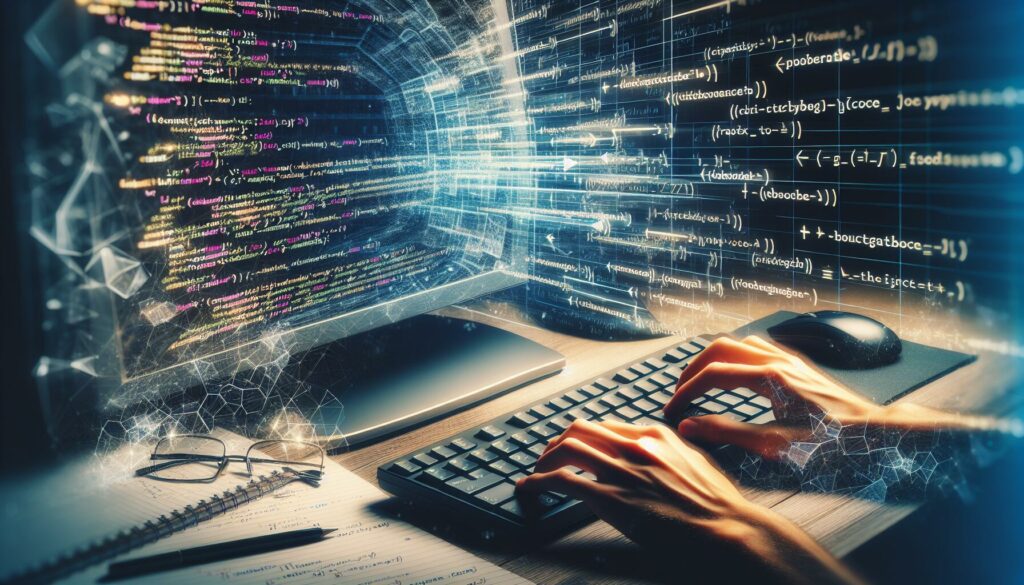
In the world of programming and software development, there’s a significant difference between watching someone code and actually writing code yourself. Many aspiring programmers find themselves in a situation where they can follow along with tutorials, understand the concepts being presented, but struggle when it comes to implementing those concepts in their own projects. This phenomenon, often referred to as the “tutorial trap,” can be a major roadblock in a programmer’s journey. In this comprehensive guide, we’ll explore strategies and techniques to help you bridge the gap between passively consuming coding content and actively creating your own code.
Understanding the Challenge
Before we dive into solutions, it’s important to understand why turning watched code into written code can be challenging:
- Passive vs. Active Learning: Watching tutorials is largely a passive activity, while writing code requires active engagement and problem-solving.
- Lack of Context: Tutorials often present isolated examples, while real-world coding requires understanding the broader context and making decisions.
- Overwhelming Complexity: Complete projects shown in tutorials can seem daunting when you’re starting from scratch.
- Dependency on Guidance: Tutorials provide step-by-step instructions, but real coding often requires figuring things out on your own.
Strategies for Turning Watched Code into Written Code
1. Active Watching
The first step in bridging the gap is to change how you watch coding tutorials:
- Take Notes: Instead of passively watching, actively take notes on key concepts, syntax, and problem-solving approaches.
- Pause and Predict: Before the instructor implements a solution, pause the video and try to predict or write the code yourself.
- Summarize in Your Own Words: After watching a section, try to explain the concept or implementation in your own words, as if teaching someone else.
2. Code Along, Then Code Alone
A two-step approach can help solidify your understanding:
- Code Along: First, follow the tutorial exactly, typing out each line of code as you watch.
- Code Alone: After completing the tutorial, close it and try to recreate the project from memory, referring to your notes if needed.
3. Break It Down
Large projects can be overwhelming. Break them down into smaller, manageable tasks:
- Identify the main components or features of the project.
- Create a list of smaller tasks for each component.
- Focus on implementing one small task at a time.
For example, if you’re building a todo list app, you might break it down like this:
- Create the basic HTML structure
- Style the app with CSS
- Add functionality to create a new todo item
- Implement the ability to mark items as complete
- Add a delete function for todo items
- Implement local storage to save todos
4. Practice Active Recall
Instead of immediately referring back to the tutorial when you get stuck, try these steps:
- Attempt to recall the solution from memory.
- If you can’t remember, try to problem-solve on your own using what you do remember.
- Check your notes for hints or key concepts.
- As a last resort, refer back to the tutorial, but focus on understanding the “why” behind the solution.
5. Modify and Expand
Once you’ve recreated the project from the tutorial, challenge yourself to modify or expand it:
- Add a new feature that wasn’t in the original tutorial.
- Change the styling or layout of the project.
- Optimize the code for better performance.
- Adapt the project to solve a slightly different problem.
6. Use Pseudocode
Before diving into writing actual code, practice writing pseudocode. This helps you focus on the logic and structure of your program without getting bogged down in syntax details.
Here’s an example of pseudocode for a simple guessing game:
INITIALIZE secret_number with a random number between 1 and 100
INITIALIZE attempts to 0
WHILE true
INCREMENT attempts
PROMPT user for a guess
IF guess equals secret_number
PRINT "Congratulations! You guessed the number in {attempts} attempts."
BREAK
ELSE IF guess < secret_number
PRINT "Too low! Try again."
ELSE
PRINT "Too high! Try again."
END IF
END WHILE
7. Embrace Error Messages
When you encounter errors (and you will), don’t get discouraged. Instead:
- Read the error message carefully to understand what’s going wrong.
- Use the error message as a clue to guide your debugging process.
- Search online for similar error messages if you’re stuck.
- Learn to use debugging tools like console.log() or breakpoints to investigate issues.
8. Join Coding Challenges
Participate in coding challenges or exercises that force you to write code from scratch:
- Use platforms like LeetCode, HackerRank, or Codewars for daily coding problems.
- Join coding competitions or hackathons to practice coding under time pressure.
- Create your own challenges based on real-world scenarios you’re interested in.
9. Collaborate and Seek Feedback
Working with others can significantly accelerate your learning:
- Join coding communities or study groups to share knowledge and challenges.
- Participate in pair programming sessions to learn different approaches and techniques.
- Share your code on platforms like GitHub and ask for code reviews.
- Offer to help others with their coding problems, which can reinforce your own understanding.
10. Build Real Projects
The ultimate way to turn watched code into written code is to work on real projects:
- Start with small projects that solve problems you personally face.
- Gradually increase the complexity of your projects as you gain confidence.
- Contribute to open-source projects to gain experience working with larger codebases.
- Build a portfolio of projects to showcase your skills and track your progress.
Practical Examples: From Watched to Written
Let’s look at a few examples of how you might transform watched code into your own written code:
Example 1: Basic Calculator
Suppose you’ve watched a tutorial on building a basic calculator. Here’s how you might approach turning that into your own code:
- Recreate the Basic Functionality: Start by implementing the core calculator functions you saw in the tutorial.
- Add a New Feature: Extend the calculator by adding a square root function that wasn’t in the original tutorial.
- Improve the UI: Redesign the calculator interface to make it more visually appealing or user-friendly.
- Implement Error Handling: Add error checking for scenarios like division by zero that may not have been covered in the tutorial.
Here’s a simple example of how you might implement the square root function:
function calculateSquareRoot() {
const number = parseFloat(document.getElementById("display").value);
if (number < 0) {
document.getElementById("display").value = "Error: Cannot calculate square root of a negative number";
} else {
const result = Math.sqrt(number);
document.getElementById("display").value = result;
}
}
Example 2: Todo List Application
If you’ve watched a tutorial on creating a todo list app, here’s how you could extend it:
- Implement Basic Functionality: Start by recreating the core features from the tutorial (add, complete, delete todos).
- Add Categories: Extend the app to allow users to categorize their todos.
- Implement Sorting: Add the ability to sort todos by date, priority, or category.
- Add a Search Function: Implement a search feature to find specific todos.
Here’s an example of how you might implement a simple search function:
function searchTodos() {
const searchTerm = document.getElementById("searchInput").value.toLowerCase();
const todoList = document.getElementById("todoList");
const todos = todoList.getElementsByTagName("li");
for (let i = 0; i < todos.length; i++) {
const todoText = todos[i].textContent.toLowerCase();
if (todoText.includes(searchTerm)) {
todos[i].style.display = "";
} else {
todos[i].style.display = "none";
}
}
}
Common Pitfalls to Avoid
As you work on turning watched code into written code, be aware of these common pitfalls:
- Copy-Paste Syndrome: Resist the urge to simply copy and paste code from tutorials. Type it out manually to reinforce your learning.
- Perfectionism: Don’t aim for perfect code from the start. Focus on getting a working solution first, then refine and optimize.
- Tunnel Vision: Don’t get stuck trying to replicate the tutorial exactly. Be open to alternative approaches and solutions.
- Skipping the Basics: Make sure you have a solid understanding of fundamental concepts before tackling complex projects.
- Neglecting Documentation: Practice reading and using official documentation, rather than relying solely on tutorials.
Tools and Resources
To support your journey from watched code to written code, consider using these tools and resources:
- Version Control: Use Git and GitHub to track changes in your code and collaborate with others.
- IDEs and Code Editors: Familiarize yourself with powerful development environments like Visual Studio Code, PyCharm, or WebStorm.
- Online Coding Platforms: Use platforms like CodePen or JSFiddle to quickly experiment with code snippets.
- Documentation Tools: Learn to use documentation sites like MDN Web Docs for JavaScript or Python’s official documentation.
- Code Visualization Tools: Use tools like PythonTutor to visualize code execution and understand how programs work step-by-step.
Conclusion
Transitioning from watching code to writing your own is a crucial step in your programming journey. It requires active engagement, practice, and persistence. By following the strategies outlined in this guide, you can bridge the gap between passive learning and active coding. Remember that becoming proficient at writing code is a gradual process, and every line of code you write brings you closer to your goals.
As you continue to develop your skills, focus on building real projects that interest you and solve actual problems. This practical application of your knowledge will not only reinforce what you’ve learned but also expose you to new challenges that will further enhance your coding abilities.
Finally, don’t forget that the programming community is vast and supportive. Engage with other developers, share your experiences, and don’t hesitate to ask for help when you need it. With dedication and the right approach, you’ll find yourself not just watching code, but confidently writing and creating your own innovative solutions.