How to Communicate the Roadmap: Outlining Your Solution Before You Start Coding
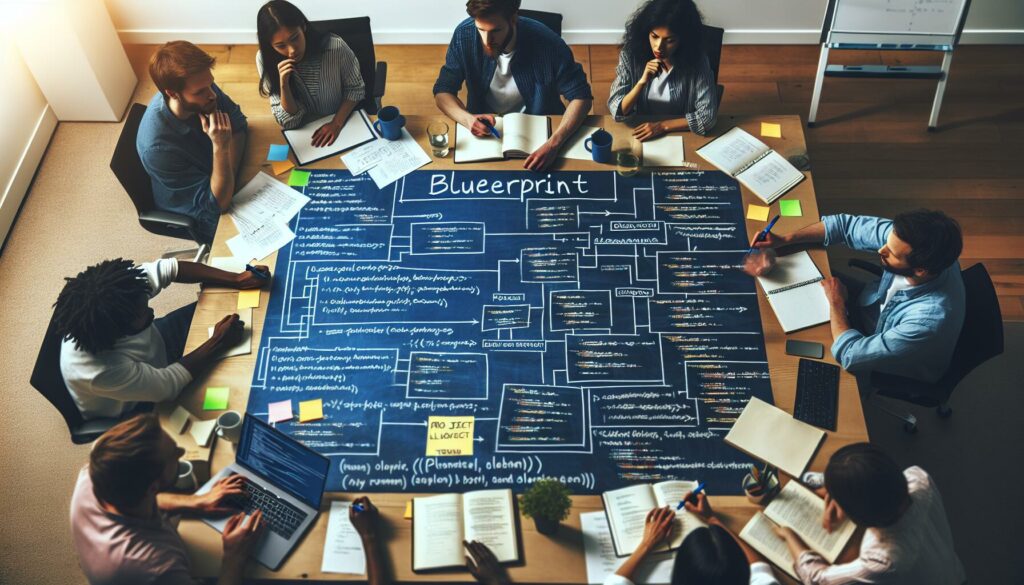
In the world of software development and technical interviews, the ability to effectively communicate your thought process is just as crucial as your coding skills. One of the most critical steps in this process is outlining your solution before you start coding. This practice, often referred to as creating a roadmap, not only helps you organize your thoughts but also demonstrates your problem-solving approach to your interviewer. In this comprehensive guide, we’ll explore the importance of laying out a high-level plan and provide you with valuable tips on how to clearly communicate your intended steps.
Why Creating a Roadmap is Essential
Before we dive into the how-to, let’s understand why creating a roadmap is so important:
- Demonstrates Analytical Thinking: By outlining your approach, you show that you can break down complex problems into manageable steps.
- Improves Code Quality: A well-thought-out plan often leads to cleaner, more efficient code.
- Saves Time: While it might seem counterintuitive, spending time on planning can actually save you time during the coding process.
- Facilitates Communication: A clear roadmap helps you articulate your ideas to interviewers, teammates, or stakeholders.
- Identifies Potential Issues: Planning ahead allows you to spot potential roadblocks before you encounter them in your code.
Steps to Create and Communicate Your Roadmap
1. Understand the Problem
Before you can create a roadmap, you need to fully grasp the problem at hand. This involves:
- Carefully reading the problem statement
- Identifying the inputs and expected outputs
- Clarifying any ambiguities with the interviewer
For example, if you’re asked to implement a function to find the nth Fibonacci number, you might say:
“Before I start, I want to make sure I understand the problem correctly. We need to write a function that takes an integer n as input and returns the nth Fibonacci number. The Fibonacci sequence starts with 0 and 1, and each subsequent number is the sum of the two preceding ones. Is that correct?”
2. Consider Edge Cases
Think about potential edge cases and how your solution will handle them. This shows that you’re considering the robustness of your solution. You might say:
“I’d like to consider some edge cases. What should we return if n is 0 or negative? Also, should we handle potential overflow for large values of n?”
3. Brainstorm Approaches
Before settling on a solution, consider multiple approaches. This demonstrates your ability to think critically and weigh different options. Communicate this process to your interviewer:
“I can think of a few ways to approach this problem. We could use a recursive solution, which would be elegant but might have performance issues for large n. Alternatively, we could use an iterative approach with a loop, which would be more efficient. There’s also a possibility of using dynamic programming to optimize further. Let me think through these options…”
4. Choose and Explain Your Approach
After considering different methods, choose the one you think is best and explain your reasoning:
“I think the best approach here would be an iterative solution using a loop. It’s more efficient than recursion as it avoids the overhead of function calls and potential stack overflow for large n. It’s also simpler to implement than dynamic programming in this case, and the performance gain wouldn’t be significant for most inputs.”
5. Outline the Steps
Now that you’ve chosen an approach, outline the steps you’ll take to implement it. Be clear and concise:
“Here’s how I plan to implement this solution:
1. First, I’ll handle the edge cases we discussed earlier.
2. Then, I’ll initialize variables for the first two Fibonacci numbers.
3. I’ll use a loop that runs n-2 times (since we’ve already handled the first two numbers).
4. In each iteration, I’ll calculate the next Fibonacci number and update our variables.
5. Finally, I’ll return the nth Fibonacci number.”
6. Discuss Time and Space Complexity
Before you start coding, it’s crucial to analyze and communicate the time and space complexity of your proposed solution:
“This solution will have a time complexity of O(n) because we’re doing a constant amount of work n times. The space complexity will be O(1) as we’re only using a fixed number of variables regardless of the input size.”
7. Seek Feedback
Before diving into the code, it’s always a good idea to check in with your interviewer:
“Does this approach sound reasonable to you? Is there anything you’d like me to clarify or reconsider before I start coding?”
Tips for Effective Communication
Now that we’ve covered the steps to create and present your roadmap, let’s look at some tips to make your communication even more effective:
1. Use Clear and Concise Language
Avoid jargon unless you’re sure the interviewer is familiar with it. Use simple, clear language to explain your thoughts. If you need to use a technical term, briefly explain what it means.
2. Think Out Loud
Interviewers are interested in your thought process. Even if you’re unsure about something, vocalize your thoughts. You might say something like:
“I’m considering using a hash map here to improve lookup time, but I’m not sure if the space trade-off is worth it for this particular problem. Let me think through this…”
3. Use Visual Aids
If possible, use diagrams or pseudocode to illustrate your ideas. This can be especially helpful for complex algorithms or data structures. You might say:
“Would it be helpful if I sketch out a quick diagram to illustrate this binary tree traversal?”
4. Break Down Complex Ideas
If your solution involves a complex algorithm, break it down into smaller, more manageable parts. Explain each part separately before putting them together.
5. Relate to Real-World Scenarios
If appropriate, relate the problem or your solution to real-world scenarios. This demonstrates your ability to see the bigger picture. For example:
“This problem is similar to how we might implement a caching system in a web application to improve performance.”
6. Be Open to Feedback
If the interviewer suggests a different approach or points out a flaw in your reasoning, be open to it. Show that you can adapt and incorporate feedback. You might say:
“That’s a great point. I hadn’t considered that edge case. Let me revise my approach to account for that.”
Common Pitfalls to Avoid
While communicating your roadmap, be aware of these common pitfalls:
1. Rushing to Code
Don’t jump straight into coding without proper planning. This often leads to mistakes and inefficiencies that could have been avoided with a clear roadmap.
2. Being Too Vague
Avoid general statements without specifics. Instead of saying “I’ll use a loop,” specify what kind of loop and what it will do.
3. Ignoring Constraints
Make sure to consider and address any constraints mentioned in the problem statement, such as time or space complexity requirements.
4. Not Considering Alternative Approaches
Even if you think you know the best solution, briefly consider and mention alternative approaches. This shows breadth of knowledge and critical thinking.
5. Overcomplicating the Solution
Sometimes, a simple solution is best. Don’t try to show off by proposing an overly complex solution if a simpler one will do.
Putting It All Together: A Sample Dialogue
Let’s look at how this might play out in an actual interview scenario. The interviewer has just asked you to implement a function to reverse a linked list.
You: “Thank you for the problem. Before I start, I’d like to make sure I understand the requirements correctly. We need to implement a function that takes the head of a singly linked list as input and returns the head of the reversed list. Is that correct?”
Interviewer: “That’s correct.”
You: “Great. Are there any constraints on the list? For example, do we need to handle empty lists or lists with only one node?”
Interviewer: “Yes, your solution should handle those cases as well.”
You: “Understood. Let me think through some approaches. We could potentially solve this recursively, which would be elegant but might have issues with very long lists due to stack overflow. Alternatively, we could use an iterative approach, which would be more space-efficient. Given that we don’t have any specific space constraints, I think the iterative approach would be more robust in this case. Does that sound reasonable?”
Interviewer: “Yes, please proceed with the iterative approach.”
You: “Alright. Here’s how I plan to implement this solution:
1. First, I’ll handle the edge cases of an empty list or a list with only one node.
2. Then, I’ll initialize three pointers: one for the previous node (initially null), one for the current node (initially the head), and one for the next node.
3. I’ll iterate through the list, in each step:
a. Save the next node
b. Update the current node’s next pointer to point to the previous node
c. Move the previous and current pointers one step forward
4. After the loop, the previous pointer will be at the new head of the reversed list, so I’ll return that.
This solution will have a time complexity of O(n) where n is the number of nodes in the list, as we’re traversing the list once. The space complexity will be O(1) as we’re only using a fixed number of pointers regardless of the input size. Does this approach make sense?”
Interviewer: “Yes, that sounds good. Please go ahead and implement it.”
You: “Great, I’ll start coding now. I’ll explain each step as I go along.”
Conclusion
Communicating your roadmap effectively is a crucial skill in technical interviews and professional software development. By clearly outlining your approach before you start coding, you demonstrate your problem-solving skills, analytical thinking, and ability to plan efficiently. Remember to understand the problem thoroughly, consider multiple approaches, outline your steps clearly, and be open to feedback. With practice, you’ll become more comfortable with this process, leading to more successful interviews and more efficient coding in your career.
At AlgoCademy, we emphasize the importance of these skills in our coding education and programming skills development programs. Our interactive coding tutorials and AI-powered assistance are designed to help you not only improve your coding skills but also enhance your ability to think algorithmically and communicate your solutions effectively. Whether you’re a beginner learning to code or preparing for technical interviews with major tech companies, mastering the art of communicating your roadmap is an invaluable skill that will serve you well throughout your programming journey.