Handling Unfamiliar Topics: How to Approach a Problem Based on a Language or Concept You Don’t Know Well
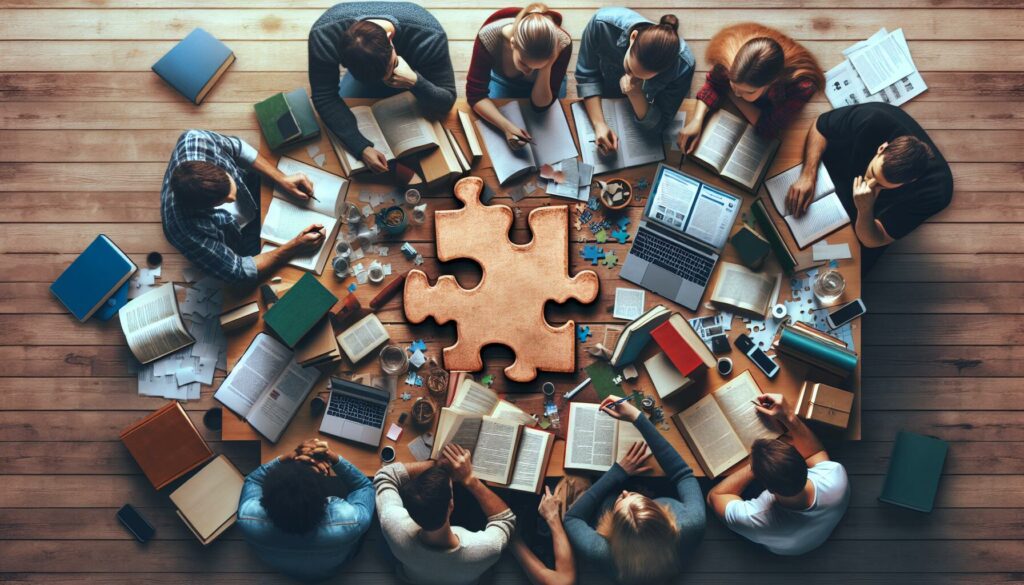
In the ever-evolving world of programming, it’s common to encounter problems or tasks that involve unfamiliar languages, concepts, or algorithms. Whether you’re a beginner or an experienced developer, facing the unknown can be daunting. However, with the right approach and mindset, you can tackle these challenges effectively and expand your knowledge in the process. In this comprehensive guide, we’ll explore strategies for handling unfamiliar topics in coding and provide practical tips for explaining your thought process when faced with the unfamiliar.
The Importance of Embracing the Unfamiliar
Before we dive into specific strategies, it’s crucial to understand why embracing unfamiliar topics is essential for your growth as a programmer:
- Continuous Learning: Technology evolves rapidly, and new languages, frameworks, and concepts emerge regularly. Being comfortable with the unfamiliar helps you stay adaptable and relevant in the field.
- Problem-Solving Skills: Tackling unfamiliar problems enhances your overall problem-solving abilities, making you a more versatile and valuable developer.
- Career Opportunities: Many job opportunities require familiarity with multiple languages and technologies. Being able to quickly adapt to new concepts can open doors to exciting career prospects.
- Personal Growth: Overcoming challenges in unfamiliar territories builds confidence and resilience, contributing to your personal and professional growth.
Strategies for Approaching Unfamiliar Coding Problems
1. Break Down the Problem
When faced with an unfamiliar coding problem, the first step is to break it down into smaller, more manageable parts. This approach helps you identify familiar elements and isolate the unfamiliar aspects.
- Identify the core problem: What is the main task or goal of the problem?
- List the known components: What parts of the problem do you understand or recognize?
- Isolate the unfamiliar elements: What specific concepts, syntax, or algorithms are new to you?
- Create a step-by-step plan: Outline the general steps needed to solve the problem, even if you’re unsure about the implementation details.
2. Research and Gather Information
Once you’ve identified the unfamiliar aspects, it’s time to gather information and resources to fill in the knowledge gaps.
- Official documentation: Start with the official documentation of the language, framework, or concept you’re dealing with. This often provides the most accurate and up-to-date information.
- Online tutorials and courses: Platforms like AlgoCademy, Coursera, edX, and freeCodeCamp offer a wealth of tutorials and courses on various programming topics.
- Developer communities: Websites like Stack Overflow, Reddit (r/learnprogramming, r/coding), and GitHub Discussions can be valuable resources for asking questions and learning from others’ experiences.
- Books and articles: For in-depth understanding, consider reading books or articles on the specific topic you’re struggling with.
- Video tutorials: Platforms like YouTube and Udemy offer visual explanations that can be helpful for understanding complex concepts.
3. Start with Pseudocode
Before diving into the actual implementation, try writing pseudocode to outline your approach. This helps you focus on the logic and structure of your solution without getting bogged down by syntax details.
// Example pseudocode for a simple sorting algorithm
function sort(array):
for each element in array:
compare with next element
if current element > next element:
swap elements
repeat until no more swaps are needed
return sorted array
4. Leverage Your Existing Knowledge
Even when dealing with unfamiliar concepts, you can often apply general programming principles and patterns you already know. Look for similarities with familiar languages or algorithms and try to adapt them to the new context.
5. Implement a Minimal Working Example
Start by implementing the simplest possible version of your solution. This allows you to test your understanding and build confidence before tackling more complex aspects of the problem.
6. Use Debugging and Testing
As you work on your solution, make extensive use of debugging tools and write tests to verify your code’s behavior. This helps you catch errors early and understand how the unfamiliar elements work in practice.
7. Seek Feedback and Collaboration
Don’t hesitate to seek feedback from peers, mentors, or online communities. Explaining your approach and getting input from others can provide valuable insights and alternative perspectives.
Explaining Your Thought Process
When working on unfamiliar problems, especially in interview or collaborative settings, it’s crucial to effectively communicate your thought process. Here are some tips for articulating your approach:
1. Think Aloud
Practice verbalizing your thoughts as you work through the problem. This helps others understand your reasoning and allows them to provide guidance or feedback.
2. Use Analogies
Draw parallels between the unfamiliar concept and something more familiar. This can help both you and your audience better understand the problem.
3. Visualize the Problem
Use diagrams, flowcharts, or other visual aids to represent the problem and your proposed solution. This can be particularly helpful for complex algorithms or data structures.
4. Explain Your Assumptions
Clearly state any assumptions you’re making about the problem or the unfamiliar concepts. This demonstrates your analytical thinking and helps identify potential misunderstandings.
5. Discuss Trade-offs
When considering different approaches, explain the pros and cons of each. This shows that you’re thinking critically about the problem, even if you’re not entirely sure about the implementation details.
6. Be Honest About Uncertainties
It’s okay to admit when you’re unsure about something. Explain what you do know and what you’d need to research further to complete the solution.
Applying General Problem-Solving Techniques
When faced with unfamiliar coding problems, you can apply several general problem-solving techniques to approach the challenge systematically:
1. The IDEAL Problem-Solving Framework
The IDEAL framework, developed by Bransford and Stein, provides a structured approach to problem-solving:
- I – Identify the problem
- D – Define the problem
- E – Explore possible strategies
- A – Act on the strategies
- L – Look back and evaluate the results
This framework can be particularly useful when dealing with unfamiliar coding problems, as it encourages a methodical approach to understanding and solving the challenge.
2. Divide and Conquer
The divide and conquer strategy involves breaking down a complex problem into smaller, more manageable subproblems. This approach is especially useful when dealing with unfamiliar concepts, as it allows you to focus on solving one piece of the puzzle at a time.
// Example of divide and conquer in pseudocode
function solveComplexProblem(problem):
if problem is simple enough to solve directly:
return directSolution(problem)
else:
subproblems = divideIntoParts(problem)
for each subproblem in subproblems:
subSolution = solveComplexProblem(subproblem)
return combineSubSolutions(subSolutions)
3. Abstraction and Generalization
When faced with an unfamiliar problem, try to abstract away the specific details and focus on the general principles or patterns involved. This can help you apply familiar concepts to new situations.
4. Incremental Development
Start with a basic implementation and gradually add features or optimize the solution. This approach allows you to build your understanding of the unfamiliar concepts step by step.
5. Pattern Recognition
Look for patterns or similarities between the unfamiliar problem and problems you’ve solved before. This can help you identify potential solution strategies or algorithms that might be applicable.
Practical Examples: Tackling Unfamiliar Coding Challenges
Let’s explore a couple of practical examples to illustrate how you might approach unfamiliar coding problems:
Example 1: Implementing a Binary Search Tree in a New Language
Suppose you’re familiar with the concept of binary search trees but need to implement one in a language you’re not experienced with, like Rust.
- Break down the problem:
- Understand the basic structure of a binary search tree
- Identify the core operations: insertion, deletion, search
- Determine how to represent nodes and the tree structure in Rust
- Research:
- Study Rust’s syntax for defining structs and implementing methods
- Look up how Rust handles pointers and references
- Find examples of tree-like structures in Rust
- Start with pseudocode:
struct Node: value: int left: Option<Node> right: Option<Node> struct BinarySearchTree: root: Option<Node> impl BinarySearchTree: fn insert(value): if root is None: create new Node with value else: recursively insert based on BST properties fn search(value): recursively search based on BST properties fn delete(value): recursively find and delete node, rebalancing as needed
- Implement a minimal working example:
- Start by implementing the Node and BinarySearchTree structs
- Implement the insert function first, as it’s fundamental to building the tree
- Test and debug:
- Write test cases to verify the insert function works correctly
- Use Rust’s built-in testing framework and debugging tools
- Iterate and expand:
- Once insert is working, move on to implementing search and delete functions
- Continuously test and refine your implementation
Example 2: Implementing a Machine Learning Algorithm
Imagine you need to implement a basic k-means clustering algorithm, but you’re not familiar with machine learning concepts or the specific algorithm.
- Break down the problem:
- Understand the goal of k-means clustering (grouping similar data points)
- Identify the main steps: initialization, assignment, update
- Determine what mathematical operations are needed (e.g., calculating distances)
- Research:
- Study the k-means algorithm and its underlying principles
- Look for existing implementations or pseudocode to understand the general structure
- Research libraries or tools that might be helpful (e.g., NumPy for Python)
- Start with pseudocode:
function kMeansClustering(data, k): initialize k centroids randomly while not converged: for each data point: assign to nearest centroid for each centroid: update position based on assigned points return centroids and assignments
- Implement a minimal working example:
- Start with a simple 2D dataset for easier visualization and debugging
- Implement the basic algorithm structure without optimizations
- Test and visualize:
- Use matplotlib or another plotting library to visualize the clusters
- Test with different datasets and numbers of clusters
- Iterate and optimize:
- Refine the implementation based on your growing understanding
- Research and implement optimizations or variations of the algorithm
Conclusion: Embracing the Learning Journey
Handling unfamiliar topics and problems is an essential skill for any programmer. By approaching these challenges with a structured methodology, leveraging your existing knowledge, and being open to learning, you can tackle even the most daunting unfamiliar problems.
Remember that every experienced programmer was once a beginner facing unfamiliar concepts. The key is to embrace the learning process, be patient with yourself, and persistently work through the challenges. With practice, you’ll develop the confidence and skills to approach any coding problem, regardless of its familiarity.
As you continue your coding journey, platforms like AlgoCademy can be invaluable resources for honing your problem-solving skills and expanding your knowledge across various programming concepts. By regularly challenging yourself with new and unfamiliar problems, you’ll not only become a more versatile programmer but also cultivate a growth mindset that will serve you well throughout your career in the ever-evolving field of technology.