The Power of Incremental Progress: How to Show Your Work Even When You Haven’t Solved the Problem
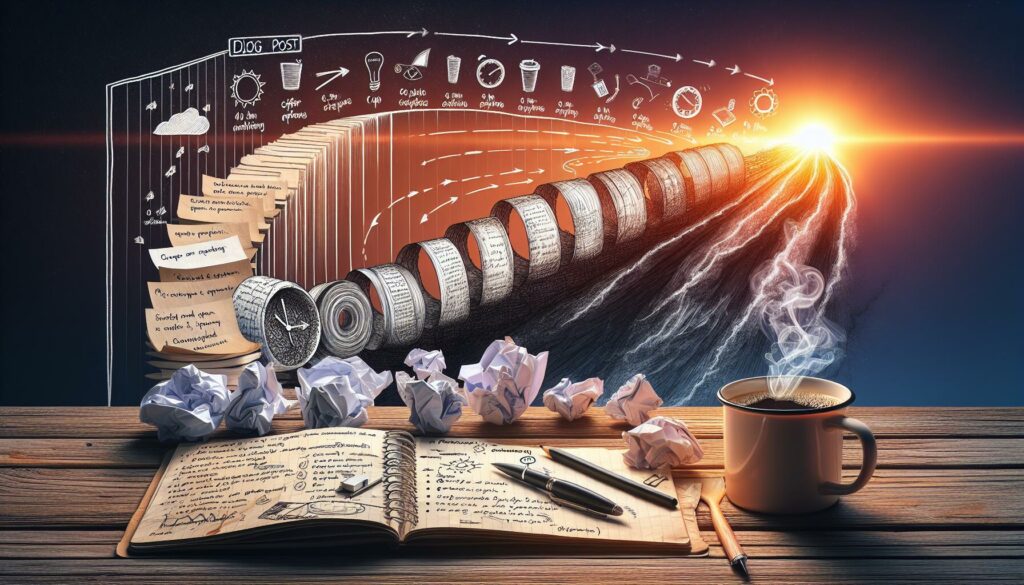
In the world of coding and software development, we often encounter complex problems that can’t be solved in a single sitting. Whether you’re a beginner tackling your first coding challenge or an experienced developer preparing for a technical interview at a FAANG company, the ability to demonstrate progress and partial solutions is a crucial skill. This article will explore the importance of showing your work, even when you haven’t fully solved the problem, and how this approach can significantly benefit your coding journey.
The Myth of the Instant Solution
There’s a common misconception that great programmers can solve any problem instantly. This myth can be discouraging for learners and even experienced developers who find themselves stuck on a challenging problem. The reality is that even the most skilled programmers often need time to work through complex issues, experimenting with different approaches and refining their solutions incrementally.
At AlgoCademy, we emphasize the importance of the problem-solving process rather than just the final solution. This approach aligns with how real-world software development works and how technical interviews are often conducted at major tech companies.
Why Showing Your Work Matters
Demonstrating your progress, even when you haven’t reached the final solution, is valuable for several reasons:
- It showcases your problem-solving approach: By showing your work, you demonstrate how you break down complex problems and approach them systematically.
- It highlights your thought process: Partial solutions and incremental progress reveal your logical thinking and how you navigate challenges.
- It demonstrates persistence: Showing multiple attempts or approaches proves your resilience and determination to solve the problem.
- It invites collaboration: By sharing your progress, you open the door for feedback and suggestions from others, which can lead to better solutions.
- It helps in learning and improvement: Documenting your progress allows you to reflect on your approach and identify areas for improvement.
Strategies for Showing Your Work
Here are some effective strategies for demonstrating your progress, even when you haven’t fully solved the problem:
1. Start with Pseudocode
Before diving into actual coding, write out your approach in pseudocode. This high-level outline of your solution shows your understanding of the problem and your planned approach. Even if you don’t complete the implementation, the pseudocode demonstrates your problem-solving skills.
Example pseudocode for a function to find the maximum element in an array:
function findMaxElement(array):
if array is empty:
return null
maxElement = first element of array
for each element in array:
if element > maxElement:
maxElement = element
return maxElement
2. Implement a Brute Force Solution
If you can’t immediately come up with an optimal solution, start with a brute force approach. While it may not be the most efficient, it shows that you can solve the problem and provides a starting point for optimization.
Here’s a simple brute force solution to find all pairs in an array that sum to a target value:
function findPairs(arr, target) {
const pairs = [];
for (let i = 0; i < arr.length; i++) {
for (let j = i + 1; j < arr.length; j++) {
if (arr[i] + arr[j] === target) {
pairs.push([arr[i], arr[j]]);
}
}
}
return pairs;
}
3. Break Down the Problem
If the main problem seems overwhelming, break it down into smaller, manageable sub-problems. Solve these sub-problems one by one, showing your progress at each step. This approach demonstrates your ability to handle complex tasks by dividing them into smaller, more manageable pieces.
For example, if you’re implementing a sorting algorithm like Merge Sort, you might break it down into these steps:
- Implement a function to merge two sorted arrays
- Implement the recursive division of the array
- Combine the merge function with the recursive division
Here’s how you might implement the merge function:
function merge(left, right) {
let result = [];
let leftIndex = 0;
let rightIndex = 0;
while (leftIndex < left.length && rightIndex < right.length) {
if (left[leftIndex] < right[rightIndex]) {
result.push(left[leftIndex]);
leftIndex++;
} else {
result.push(right[rightIndex]);
rightIndex++;
}
}
return result.concat(left.slice(leftIndex)).concat(right.slice(rightIndex));
}
4. Use Comments to Explain Your Thinking
As you work through the problem, use comments to explain your thought process, any assumptions you’re making, and why you’re choosing certain approaches. This not only helps others understand your code but also demonstrates your ability to communicate your ideas clearly.
function isPalindrome(str) {
// Convert to lowercase and remove non-alphanumeric characters
str = str.toLowerCase().replace(/[^a-z0-9]/g, '');
// Initialize pointers at the start and end of the string
let left = 0;
let right = str.length - 1;
// Compare characters from both ends moving inwards
while (left < right) {
if (str[left] !== str[right]) {
// If characters don't match, it's not a palindrome
return false;
}
left++;
right--;
}
// If we've made it through the whole string, it's a palindrome
return true;
}
5. Implement Edge Cases
Even if you can’t solve the entire problem, make sure to handle edge cases and show how your solution would work for these scenarios. This demonstrates your attention to detail and ability to consider various inputs.
function divide(a, b) {
// Handle division by zero
if (b === 0) {
throw new Error("Cannot divide by zero");
}
// Handle integer overflow for extremely large numbers
if (a === Number.MIN_SAFE_INTEGER && b === -1) {
return Number.MAX_SAFE_INTEGER;
}
// Perform the division
return Math.trunc(a / b);
}
6. Optimize Incrementally
If you start with a brute force solution, show how you would optimize it step by step. Even if you don’t reach the most optimal solution, demonstrating your ability to improve code efficiency is valuable.
For example, let’s optimize a function that finds the nth Fibonacci number:
// Initial recursive solution (inefficient)
function fibRecursive(n) {
if (n <= 1) return n;
return fibRecursive(n - 1) + fibRecursive(n - 2);
}
// Optimized solution using dynamic programming
function fibDynamic(n) {
if (n <= 1) return n;
let prev = 0, curr = 1;
for (let i = 2; i <= n; i++) {
let next = prev + curr;
prev = curr;
curr = next;
}
return curr;
}
7. Use Version Control
If you’re working on a larger project or over an extended period, use version control systems like Git to track your progress. Commit your changes frequently with descriptive commit messages that explain what you’ve done and why. This creates a clear history of your problem-solving process.
git commit -m "Implement basic array traversal for sum calculation"
git commit -m "Add edge case handling for empty arrays"
git commit -m "Optimize time complexity using two-pointer technique"
The Benefits of Incremental Progress in Coding Interviews
When it comes to coding interviews, especially for positions at major tech companies, the ability to show incremental progress is particularly valuable. Here’s why:
1. It Demonstrates Problem-Solving Skills
Interviewers are often more interested in your problem-solving approach than whether you can produce a perfect solution immediately. By showing your work step-by-step, you give them insight into how you tackle complex problems.
2. It Allows for Collaboration
In many interviews, the interviewer may provide hints or ask questions about your approach. By showing your progress, you create opportunities for this kind of collaborative problem-solving, which is an important skill in real-world development.
3. It Shows Resilience
Tech interviews can be stressful, and it’s common to encounter problems you can’t immediately solve. By continuing to work through the problem and show your progress, you demonstrate resilience and the ability to perform under pressure.
4. It Provides Talking Points
Even if you don’t reach the final solution, your partial progress gives you something to discuss with the interviewer. You can explain your approach, discuss alternative methods you considered, and talk about how you might optimize your solution given more time.
Practical Tips for Showing Progress in Coding Interviews
Here are some practical tips for demonstrating your progress during a coding interview:
1. Think Aloud
Verbalize your thought process as you work through the problem. This gives the interviewer insight into your reasoning and allows them to provide guidance if needed.
2. Use the Whiteboard Effectively
If you’re in an in-person interview with a whiteboard, use it to sketch out your ideas, draw diagrams, or write pseudocode before you start coding. This visual representation of your thoughts can be very helpful.
3. Start with a Simple Approach
Begin with a straightforward solution, even if it’s not the most efficient. You can then discuss how you would optimize it, demonstrating your ability to iterate and improve your code.
4. Test Your Code
As you write your solution, periodically stop to test it with sample inputs. This shows your attention to detail and your ability to catch and fix errors.
5. Discuss Trade-offs
If you’re considering multiple approaches, discuss the trade-offs between them. This shows your ability to evaluate different solutions and make informed decisions.
6. Ask Clarifying Questions
Don’t hesitate to ask questions about the problem or requirements. This demonstrates your thoroughness and ensures you’re solving the right problem.
Learning from Partial Solutions
Even when you don’t reach a complete solution, there’s a lot to be learned from partial progress. Here’s how you can make the most of these experiences:
1. Reflect on Your Approach
After the interview or coding session, take some time to reflect on your approach. What worked well? Where did you get stuck? This self-analysis can help you improve for future challenges.
2. Research Alternative Solutions
Once you’ve had a chance to work on the problem, research other possible solutions. This can help you expand your problem-solving toolkit and learn new techniques.
3. Practice Similar Problems
If you struggled with a particular type of problem, seek out similar challenges to practice. Platforms like AlgoCademy offer a wide range of coding problems to help you build your skills.
4. Seek Feedback
If possible, ask for feedback on your approach. Whether it’s from an interviewer, a mentor, or a peer, constructive feedback can provide valuable insights for improvement.
Conclusion
In the world of coding and software development, the ability to show your work and demonstrate incremental progress is a valuable skill. Whether you’re learning to code, preparing for technical interviews, or working on complex projects, embracing this approach can lead to better problem-solving skills, more effective learning, and improved communication with your peers and potential employers.
Remember, even the most experienced developers encounter challenges they can’t immediately solve. What sets them apart is their ability to approach problems systematically, show their work, and learn from both their successes and their struggles.
By focusing on the process of problem-solving rather than just the end result, you’ll not only become a better coder but also a more resilient and adaptable professional. So the next time you’re faced with a challenging coding problem, don’t be discouraged if you can’t solve it right away. Instead, embrace the opportunity to show your work, demonstrate your problem-solving approach, and learn from the experience.
Keep coding, keep learning, and remember that every step forward, no matter how small, is progress worth celebrating.