How to Structure a Clear and Concise Explanation During a Coding Interview
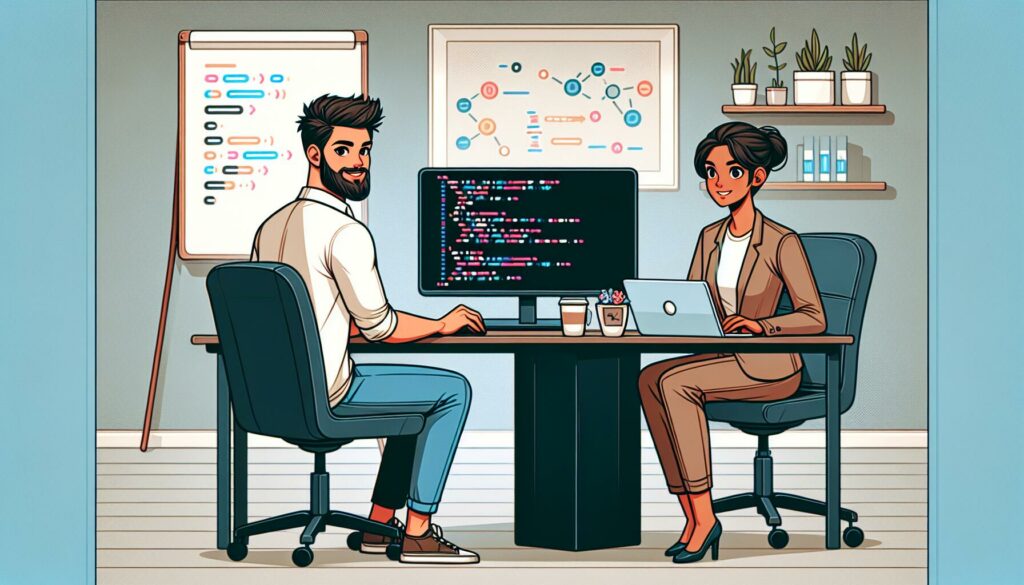
When it comes to coding interviews, your ability to solve problems is just as important as your ability to explain your thought process clearly and concisely. In fact, many interviewers place a high value on candidates who can articulate their ideas effectively. This skill demonstrates not only your technical prowess but also your potential to be a great team player and collaborator. In this comprehensive guide, we’ll explore how to structure your explanations during a coding interview, ensuring you convey your thoughts in a clear, organized, and impactful manner.
Why Clear Communication Matters in Coding Interviews
Before we dive into the specifics of structuring your explanation, let’s understand why this skill is crucial:
- Demonstrates problem-solving skills: A well-structured explanation showcases your ability to break down complex problems into manageable parts.
- Shows thought process: Interviewers want to see how you think, not just the final solution.
- Proves communication skills: In real-world scenarios, developers often need to explain their code to non-technical stakeholders.
- Highlights collaboration potential: Clear communication is essential for effective teamwork in software development.
- Allows for feedback: When you explain clearly, interviewers can provide meaningful feedback or guide you if you’re on the wrong track.
The STAR Framework for Structuring Your Explanation
To help you organize your thoughts and present them coherently, we recommend using the STAR framework. Originally used for behavioral interviews, this framework can be adapted for coding interviews to ensure you cover all necessary aspects of your solution. Here’s how it works:
- S – Situation: Understand and restate the problem
- T – Task: Identify the specific requirements and constraints
- A – Approach: Explain your strategy for solving the problem
- R – Result: Implement the solution and discuss the outcome
Let’s break down each step of this framework in detail.
1. Situation: Understanding the Problem
The first step in any coding interview is to ensure you fully understand the problem at hand. This involves:
- Restating the problem in your own words
- Asking clarifying questions
- Identifying the inputs and expected outputs
For example, if given a problem to find the longest substring without repeating characters, you might say:
“So, if I understand correctly, we need to write a function that takes a string as input and returns the length of the longest substring within it that doesn’t have any repeating characters. Is that right?”
This step shows the interviewer that you’re actively engaged and helps prevent misunderstandings that could lead you down the wrong path.
2. Task: Identifying Requirements and Constraints
Once you understand the problem, identify any specific requirements or constraints. This might include:
- Time complexity requirements
- Space complexity limitations
- Input size considerations
- Any special cases or edge cases to handle
For our example problem, you might say:
“Before we proceed, I’d like to clarify a few things. Are there any specific requirements for time or space complexity? Also, should we consider empty strings or strings with non-ASCII characters?”
This step demonstrates your attention to detail and your ability to think about potential edge cases.
3. Approach: Explaining Your Strategy
Now that you understand the problem and its constraints, it’s time to explain your approach. This is where you’ll outline your strategy for solving the problem. Here’s how to structure this part:
- High-level overview: Start with a brief, high-level description of your approach.
- Step-by-step breakdown: Explain each step of your algorithm in more detail.
- Data structures and algorithms: Discuss any specific data structures or algorithms you plan to use and why.
- Time and space complexity analysis: Explain the expected time and space complexity of your solution.
For our example problem, you might say:
“I propose using a sliding window approach with a hash set to solve this problem efficiently. Here’s how it would work:
1. We’ll use two pointers, left and right, both starting at the beginning of the string.
2. We’ll also use a hash set to keep track of characters in the current window.
3. We’ll move the right pointer, adding characters to the set as we go.
4. If we encounter a duplicate character, we’ll start moving the left pointer, removing characters from the set until the duplicate is removed.
5. We’ll keep track of the maximum length seen so far.This approach should give us a time complexity of O(n) where n is the length of the string, as we’re only traversing the string once. The space complexity would be O(min(m, n)), where m is the size of the character set, as in the worst case, we might need to store all unique characters in the set.”
This detailed explanation shows your problem-solving skills and your ability to think through the solution systematically.
4. Result: Implementing the Solution
The final step is to implement your solution and discuss the results. Here’s how to approach this:
- Code implementation: Write clean, readable code that implements your proposed solution.
- Explain as you code: Provide a running commentary as you write your code, explaining each part.
- Test cases: Discuss and implement test cases, including edge cases.
- Debugging: If you encounter any issues, explain your debugging process.
- Optimization: If time allows, discuss potential optimizations or alternative approaches.
Here’s an example of how you might explain your code implementation:
“Let me implement the solution we discussed. I’ll use Python for this example:
def longest_substring_without_repeats(s): char_set = set() left = right = max_length = 0 while right < len(s): if s[right] not in char_set: char_set.add(s[right]) max_length = max(max_length, right - left + 1) right += 1 else: char_set.remove(s[left]) left += 1 return max_length
Now, let’s walk through this implementation:
1. We initialize an empty set to keep track of characters in our current window.
2. We use two pointers, left and right, both starting at 0.
3. We iterate through the string using the right pointer:
– If the current character is not in the set, we add it and update our max_length if necessary.
– If it is in the set, we remove characters from the left until we’ve removed the duplicate.
4. Finally, we return the maximum length we’ve found.Let’s test this with a few examples:
print(longest_substring_without_repeats("abcabcbb")) # Should output 3 print(longest_substring_without_repeats("bbbbb")) # Should output 1 print(longest_substring_without_repeats("pwwkew")) # Should output 3 print(longest_substring_without_repeats("")) # Should output 0
These test cases cover various scenarios, including the empty string edge case.”
This step-by-step explanation of your code implementation demonstrates your coding skills and your ability to think through different scenarios.
Tips for Effective Communication During Coding Interviews
Now that we’ve covered the STAR framework for structuring your explanation, here are some additional tips to enhance your communication during coding interviews:
1. Practice Active Listening
Effective communication is a two-way street. Make sure you’re not just focused on explaining, but also on listening to the interviewer. They may provide valuable hints or ask important questions that can guide your solution.
2. Use Clear and Concise Language
Avoid using overly complex terminology or jargon unless you’re sure the interviewer is familiar with it. Aim for clarity and simplicity in your explanations.
3. Visualize Your Thought Process
Sometimes, a picture is worth a thousand words. Don’t hesitate to use diagrams or flowcharts to illustrate your approach. This can be particularly helpful when explaining complex algorithms or data structures.
4. Think Aloud
Even if you’re not sure about something, vocalize your thoughts. This gives the interviewer insight into your problem-solving process and allows them to provide guidance if needed.
5. Be Open to Feedback
If the interviewer suggests a different approach or points out an issue with your solution, be receptive to their feedback. Show that you can incorporate new information and adapt your approach accordingly.
6. Manage Your Time Effectively
Be mindful of the time you spend on each part of your explanation. While it’s important to be thorough, you also need to ensure you have enough time to implement and test your solution.
7. Use Analogies When Appropriate
If you’re explaining a complex concept, consider using analogies to make it more relatable. This can help bridge the gap between technical concepts and real-world understanding.
8. Highlight Trade-offs
When discussing your approach, be sure to mention any trade-offs you’re making. For example, you might choose a solution that’s easier to implement but slightly less efficient, or vice versa. Explaining these trade-offs shows your ability to consider different factors in your decision-making process.
Common Pitfalls to Avoid
While striving for clear and concise explanations, be aware of these common pitfalls:
1. Rambling
It’s easy to get carried away and start rambling, especially when you’re nervous. Stick to the STAR framework to keep your explanation focused and on-topic.
2. Assuming Too Much Knowledge
Don’t assume the interviewer knows every detail of what you’re talking about. It’s better to err on the side of over-explaining than under-explaining.
3. Ignoring the Interviewer
Pay attention to the interviewer’s body language and verbal cues. If they look confused or try to interject, pause and allow them to ask questions or provide input.
4. Rushing Through the Explanation
While you want to be concise, don’t rush through your explanation. Take the time to ensure each point is clearly conveyed.
5. Getting Defensive
If the interviewer challenges your approach or points out an error, don’t get defensive. Instead, view it as an opportunity to demonstrate your ability to handle feedback and improve your solution.
Practicing Your Explanation Skills
Like any skill, the ability to provide clear and concise explanations improves with practice. Here are some ways to hone this skill:
1. Mock Interviews
Participate in mock interviews with friends, mentors, or through online platforms. This will help you get comfortable explaining your thought process under pressure.
2. Teach Others
Try explaining coding concepts to others, especially those with less technical background. This will force you to break down complex ideas into simpler terms.
3. Record Yourself
Record yourself solving coding problems and explaining your process. Review the recordings to identify areas for improvement in your communication.
4. Write Blog Posts or Create Videos
Creating content about coding concepts can help you organize your thoughts and practice explaining technical ideas clearly.
5. Participate in Coding Forums
Engage in online coding forums where you can explain your solutions to others and receive feedback on your explanations.
Conclusion
Structuring a clear and concise explanation during a coding interview is a crucial skill that can set you apart from other candidates. By following the STAR framework (Situation, Task, Approach, Result) and incorporating the tips and strategies outlined in this guide, you can effectively communicate your problem-solving process and showcase your technical skills.
Remember, the goal is not just to solve the problem, but to demonstrate your thought process, your ability to communicate complex ideas, and your potential as a collaborative team member. With practice and mindful application of these techniques, you’ll be well-equipped to excel in your coding interviews and beyond.
Keep in mind that clear communication is a valuable skill not just for interviews, but throughout your career in software development. Whether you’re explaining your code to team members, presenting ideas to stakeholders, or mentoring junior developers, the ability to structure clear and concise explanations will serve you well in all aspects of your professional life.