The Role of Test Cases: Why You Should Test Your Code Before Declaring It Done
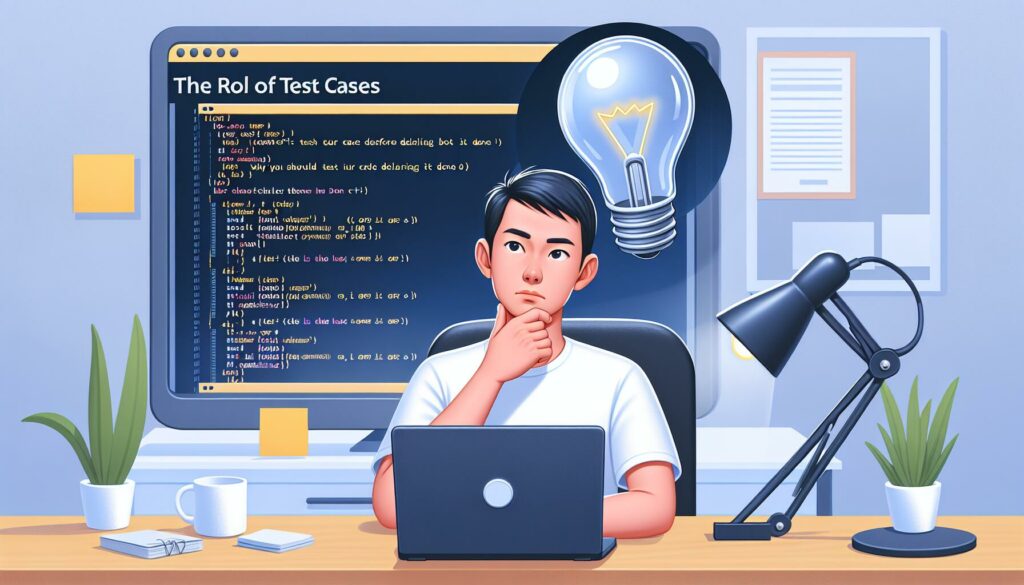
In the world of software development, writing code is just the beginning. To ensure your code is robust, efficient, and error-free, testing plays a crucial role. This is especially true in coding interviews, where demonstrating your ability to write reliable code can set you apart from other candidates. In this article, we’ll explore the importance of test cases, why you should run them even when not explicitly asked, and how to think of edge cases to make your code bulletproof.
Why Test Cases Matter in Coding Interviews
Coding interviews are designed to assess not just your ability to write code, but also your problem-solving skills, attention to detail, and overall approach to software development. Running test cases, even when not explicitly requested by the interviewer, can showcase these qualities and more. Here’s why test cases are essential in coding interviews:
1. Demonstrating Thoroughness
By running test cases, you show the interviewer that you’re thorough and methodical in your approach. This indicates that you’re not satisfied with a solution that merely works for the given example, but you’re committed to ensuring it works for all possible scenarios.
2. Proactive Problem-Solving
Taking the initiative to test your code demonstrates proactive problem-solving. It shows that you anticipate potential issues and address them before they become problems, a valuable trait in any software developer.
3. Quality Assurance Mindset
Running tests showcases your quality assurance mindset. It indicates that you understand the importance of delivering reliable, bug-free code, which is crucial in real-world software development scenarios.
4. Debugging Skills
If your tests reveal issues in your code, you have the opportunity to demonstrate your debugging skills. This can be just as impressive as writing the initial solution, as it shows your ability to identify and fix problems efficiently.
5. Communication Skills
Discussing your test cases and the reasoning behind them allows you to showcase your communication skills. It demonstrates your ability to explain your thought process and technical decisions clearly, an essential skill for working in a team environment.
How to Approach Testing in Coding Interviews
Now that we understand the importance of testing, let’s look at how to approach it effectively in a coding interview:
1. Start with the Given Examples
Begin by running your code against the examples provided in the problem statement. This ensures your solution works for the basic cases and helps you catch any immediate issues.
2. Consider Edge Cases
Think about potential edge cases that might not be covered by the given examples. These could include:
- Empty input
- Very large or very small inputs
- Negative numbers (if applicable)
- Boundary conditions
- Invalid inputs
3. Test Incrementally
As you write your code, test it incrementally. This helps you catch errors early and makes debugging easier.
4. Use Print Statements
In the absence of a proper testing framework, use print statements to output intermediate results. This can help you understand how your code is behaving and identify where issues might be occurring.
5. Explain Your Test Cases
As you run your tests, explain to the interviewer why you’ve chosen these specific test cases. This demonstrates your reasoning and problem-solving approach.
Tips for Thinking of Edge Cases
Identifying edge cases is a crucial skill in software development. Here are some tips to help you think of comprehensive edge cases:
1. Understand the Problem Domain
Thoroughly understand the problem and its constraints. This will help you identify potential edge cases specific to the problem domain.
2. Consider Extreme Values
Think about the extreme values that your input could take. For example:
- Minimum and maximum possible values
- Empty or null inputs
- Very large inputs that might cause overflow
3. Analyze Input Types
Consider different types of input that your function might receive:
- For numbers: positive, negative, zero, fractional, very large, very small
- For strings: empty string, very long string, string with special characters
- For arrays: empty array, array with one element, very large array
4. Think About Boundary Conditions
Pay attention to boundary conditions, such as:
- The first and last elements of an array
- Values just inside and outside the allowed range
- Transition points in your algorithm
5. Consider Invalid Inputs
Think about how your code should handle invalid inputs. This might include:
- Incorrect data types
- Out-of-range values
- Malformed input
6. Use the CORRECT Mnemonic
The CORRECT mnemonic can help you remember different types of edge cases:
- Conformance: Does the input conform to the expected format?
- Order: Does the order of inputs matter?
- Range: Is the input within the expected range?
- Reference: Are all referenced objects or external dependencies available?
- Existence: Does the input exist? Can it be null or empty?
- Cardinality: Are we dealing with the right number of items?
- Time: Is there any time-related aspect to consider?
Example: Implementing and Testing a Solution
Let’s walk through an example of implementing a solution and testing it thoroughly. We’ll use a common interview question: reversing a string.
Problem Statement
Write a function that reverses a given string.
Initial Implementation
Here’s a simple Python implementation:
def reverse_string(s):
return s[::-1]
Testing the Solution
Now, let’s test this solution with various test cases:
def test_reverse_string():
# Test case 1: Normal string
assert reverse_string("hello") == "olleh"
# Test case 2: Empty string
assert reverse_string("") == ""
# Test case 3: Single character
assert reverse_string("a") == "a"
# Test case 4: String with spaces
assert reverse_string("hello world") == "dlrow olleh"
# Test case 5: String with special characters
assert reverse_string("a!b@c#d$") == "$d#c@b!a"
# Test case 6: Palindrome
assert reverse_string("racecar") == "racecar"
# Test case 7: Unicode characters
assert reverse_string("ä½ å¥½ä¸–ç•Œ") == "ç•Œä¸–å¥½ä½ "
print("All test cases passed!")
test_reverse_string()
By running these test cases, we’ve covered various scenarios:
- Normal case with a simple word
- Edge case with an empty string
- Edge case with a single character
- String with multiple words (testing spaces)
- String with special characters
- Palindrome (input equals output)
- String with Unicode characters
This comprehensive set of tests helps ensure our function works correctly for various inputs.
The Importance of Testing in Real-World Software Development
While we’ve focused on the importance of testing in coding interviews, it’s crucial to understand that testing is even more critical in real-world software development. Here’s why:
1. Preventing Bugs in Production
Thorough testing helps catch bugs before they make it to production. This saves time, money, and potentially prevents damage to a company’s reputation.
2. Facilitating Refactoring and Maintenance
A good suite of tests allows developers to refactor code with confidence. When you change the implementation, running the tests can quickly verify that the functionality remains correct.
3. Documenting Expected Behavior
Tests serve as living documentation of how the code is expected to behave. This can be invaluable for new team members or when revisiting code after a long time.
4. Improving Code Design
Writing tests often leads to better code design. If code is difficult to test, it’s often a sign that the design could be improved.
5. Enabling Continuous Integration and Deployment
Automated tests are a crucial component of continuous integration and deployment pipelines, allowing for faster and more reliable software releases.
Advanced Testing Techniques
As you progress in your software development career, you’ll encounter more advanced testing techniques. Here are a few to be aware of:
1. Unit Testing
Unit tests focus on testing individual components or functions in isolation. They’re typically the most granular form of testing.
2. Integration Testing
Integration tests verify that different parts of the application work together correctly.
3. Functional Testing
Functional tests ensure that the application behaves correctly from the user’s perspective, often simulating user interactions.
4. Performance Testing
Performance tests evaluate how the system performs under various conditions, including heavy loads.
5. Test-Driven Development (TDD)
TDD is a development process where you write tests before writing the actual code. This can lead to more thoughtful design and comprehensive test coverage.
Conclusion
Testing your code is not just a best practice; it’s an essential part of the software development process. In coding interviews, running test cases demonstrates your thoroughness, proactive problem-solving, and commitment to quality. By thinking critically about edge cases and potential issues, you showcase your ability to write robust, reliable code.
Remember, the goal isn’t just to write code that works for the given example, but to create solutions that are reliable across a wide range of scenarios. By incorporating testing into your coding process, you’ll not only perform better in interviews but also develop habits that will serve you well throughout your software development career.
As you continue to grow as a developer, make testing an integral part of your coding workflow. It will help you write better code, catch issues early, and ultimately become a more effective and reliable software engineer. Whether you’re preparing for interviews or working on real-world projects, the ability to think critically about testing and edge cases will set you apart and contribute to your success in the field of software development.