Solving Coding Problems Without Overthinking: How to Trust Your Instincts
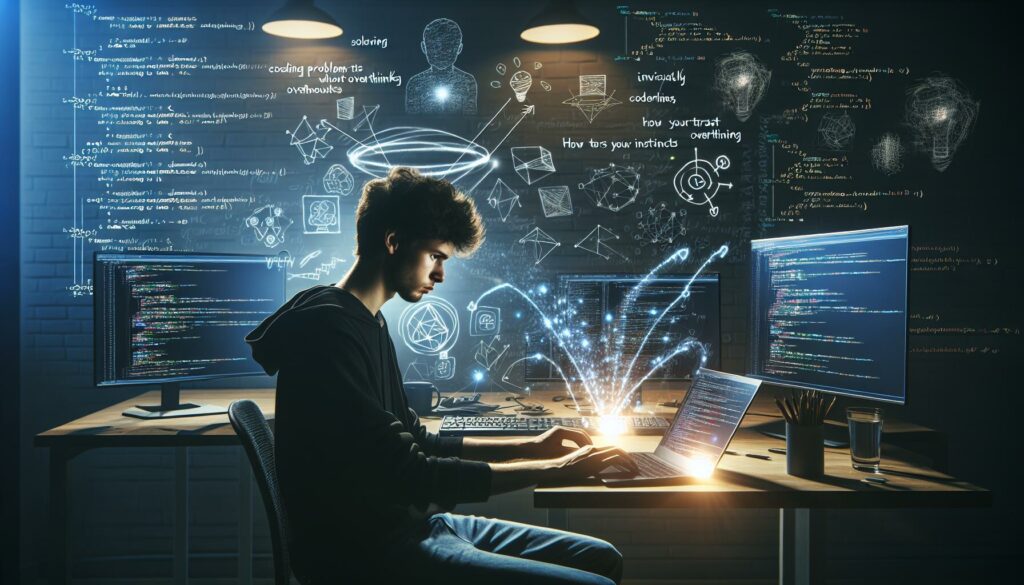
As a programmer, you’ve likely encountered moments during coding interviews or while tackling complex problems where you find yourself stuck in an endless loop of overthinking. This mental trap can be paralyzing, causing you to second-guess your instincts and potentially miss out on elegant solutions that are right in front of you. In this comprehensive guide, we’ll explore strategies to help you avoid overthinking, trust your coding instincts, and approach problem-solving with confidence, especially in high-pressure interview settings.
Understanding the Overthinking Trap
Before we dive into strategies for overcoming overthinking, it’s crucial to understand why it happens in the first place. Overthinking in coding often stems from:
- Fear of making mistakes
- Pressure to impress interviewers or peers
- Lack of confidence in one’s abilities
- The desire to find the “perfect” solution
- Analysis paralysis due to too many options
Recognizing these triggers is the first step in learning how to trust your instincts and avoid getting caught in the overthinking trap.
Strategies for Trusting Your Coding Instincts
1. Start with the Simplest Solution
When faced with a coding problem, resist the urge to immediately jump to complex algorithms or data structures. Instead, start with the simplest solution that comes to mind. This approach has several benefits:
- It helps you build momentum and confidence
- It provides a working solution that you can iterate upon
- It demonstrates your problem-solving process to interviewers
Remember, you can always optimize your solution later if needed. The key is to get started and trust your initial instincts.
2. Time-Box Your Thinking
Set a strict time limit for your initial brainstorming phase. This technique, known as time-boxing, can help prevent overthinking by forcing you to act on your instincts. Here’s how to implement it:
- Give yourself 2-3 minutes to think about the problem
- Write down your initial thoughts and approach
- Start coding based on your initial plan
By limiting your thinking time, you’re more likely to trust your first instincts and avoid falling into an overthinking spiral.
3. Verbalize Your Thought Process
In an interview setting, talking through your thought process can help you organize your ideas and prevent overthinking. This technique, often called “thinking aloud,” has several advantages:
- It helps you stay focused on the problem at hand
- It allows interviewers to understand your reasoning
- It can lead to valuable hints or guidance from the interviewer
Don’t be afraid to share your initial thoughts, even if you’re not 100% sure they’re correct. This openness demonstrates your problem-solving skills and ability to communicate effectively.
4. Break Down Complex Problems
When faced with a complex coding problem, break it down into smaller, more manageable sub-problems. This approach can help you avoid overthinking by allowing you to focus on one piece at a time. Here’s a step-by-step guide:
- Identify the main components of the problem
- Tackle each sub-problem individually
- Combine your solutions to solve the overall problem
By breaking down complex problems, you can trust your instincts for each smaller component, leading to a more confident overall solution.
5. Use Pseudocode to Outline Your Approach
Before diving into actual code, use pseudocode to outline your approach. This technique can help you organize your thoughts and prevent overthinking by providing a clear roadmap. Here’s an example of how pseudocode can help:
// Problem: Find the maximum element in an array
// Pseudocode:
// 1. Initialize max_element as the first element of the array
// 2. Iterate through the array starting from the second element
// 3. If current element > max_element, update max_element
// 4. Return max_element
// Actual code implementation:
function findMaxElement(arr) {
let maxElement = arr[0];
for (let i = 1; i < arr.length; i++) {
if (arr[i] > maxElement) {
maxElement = arr[i];
}
}
return maxElement;
}
By using pseudocode, you can quickly outline your solution and trust your instincts without getting bogged down in syntax details.
6. Practice Active Recall
To build confidence in your coding instincts, practice active recall of common algorithms and data structures. This technique involves regularly testing yourself on key concepts without referring to notes or resources. Here’s how to implement active recall:
- Create flashcards with common coding problems and their solutions
- Regularly quiz yourself on these problems
- Try to recall the solution before checking the answer
By strengthening your recall abilities, you’ll be more likely to trust your instincts when faced with similar problems in interviews or real-world scenarios.
7. Embrace the Iterative Process
Remember that coding is an iterative process. Your first solution doesn’t have to be perfect. Embrace the idea of continuous improvement and trust that you can refine your code as you go. Here’s how to approach coding iteratively:
- Write a basic working solution
- Test your code with various inputs
- Identify areas for improvement
- Refactor and optimize your code
- Repeat steps 2-4 until you’re satisfied with the result
By adopting an iterative mindset, you can avoid overthinking and trust that you’ll arrive at an optimal solution through continuous refinement.
Common Pitfalls to Avoid
While learning to trust your coding instincts, be aware of these common pitfalls that can lead to overthinking:
1. Premature Optimization
Resist the urge to optimize your code before you have a working solution. Premature optimization can lead to unnecessary complexity and wasted time. Instead, focus on getting a functional solution first, then optimize if needed.
2. Analysis Paralysis
Don’t get stuck comparing multiple approaches indefinitely. If you find yourself unable to decide between different solutions, choose one and start implementing it. You can always adjust your approach later if necessary.
3. Perfectionism
Accept that there’s rarely a “perfect” solution in coding. Striving for perfection can lead to overthinking and unnecessary stress. Instead, aim for a solution that is correct, efficient, and maintainable.
4. Ignoring Time Constraints
In interview settings or timed coding challenges, be mindful of time constraints. Don’t spend too much time on a single problem at the expense of other questions. Trust your instincts and move on if you’re stuck.
Building Confidence in Your Coding Abilities
Trusting your coding instincts is closely tied to your overall confidence as a programmer. Here are some strategies to build and maintain confidence in your abilities:
1. Regular Practice
Consistent practice is key to building confidence. Set aside time each day or week to work on coding problems, even if it’s just for 30 minutes. Platforms like AlgoCademy offer a wide range of problems to help you sharpen your skills.
2. Review and Reflect
After solving a problem, take time to review your solution and reflect on your approach. Ask yourself:
- What went well?
- What could I have done differently?
- How can I apply this learning to future problems?
This reflective practice can help reinforce your learning and boost your confidence in tackling similar problems in the future.
3. Seek Feedback
Don’t hesitate to seek feedback on your code from peers, mentors, or online communities. Constructive criticism can help you identify areas for improvement and validate your strengths, ultimately boosting your confidence.
4. Celebrate Small Wins
Acknowledge and celebrate your coding achievements, no matter how small. Successfully solving a tricky problem or optimizing an algorithm are victories worth recognizing. These positive reinforcements can help build your confidence over time.
Applying These Strategies in Interview Settings
Interviews can be particularly challenging when it comes to trusting your coding instincts. Here are some tips specifically for interview scenarios:
1. Communicate Clearly
Don’t be afraid to think out loud during the interview. Clearly communicating your thought process can help you organize your ideas and prevent overthinking. It also allows the interviewer to provide guidance if needed.
2. Ask Clarifying Questions
If you’re unsure about any aspect of the problem, ask for clarification. This shows initiative and helps ensure you’re solving the right problem. Trust your instinct when you feel something needs further explanation.
3. Use the Whiteboard Effectively
In whiteboard coding interviews, use the space to your advantage. Write down key information, draw diagrams, or outline your approach. This visual aid can help you trust your instincts and avoid getting lost in mental loops.
4. Be Open to Hints
If the interviewer offers a hint, don’t dismiss it out of hand. Be open to their guidance while still trusting your problem-solving instincts. The hint may align with your initial thoughts and boost your confidence.
5. Practice Mock Interviews
Regularly participate in mock interviews to simulate the pressure of real interview scenarios. This practice can help you become more comfortable trusting your instincts under stress.
Embracing Continuous Learning
Remember that becoming confident in your coding instincts is an ongoing process. Embrace a mindset of continuous learning and improvement. Here are some ways to support your growth:
1. Stay Updated with Industry Trends
Keep abreast of new programming languages, frameworks, and best practices. This knowledge can help you feel more confident in your coding decisions.
2. Contribute to Open Source Projects
Contributing to open source projects can expose you to different coding styles and approaches, helping you refine your instincts and build confidence in your abilities.
3. Teach Others
Teaching coding concepts to others can reinforce your own understanding and boost your confidence. Consider mentoring junior developers or creating coding tutorials.
4. Participate in Coding Challenges
Regularly participate in coding challenges or competitions. These time-constrained events can help you practice trusting your instincts under pressure.
Conclusion: Trusting Your Coding Journey
Learning to trust your coding instincts is a crucial skill that can significantly improve your problem-solving abilities and performance in technical interviews. By implementing the strategies outlined in this guide, you can avoid the trap of overthinking and approach coding challenges with confidence.
Remember, the goal is not to eliminate all doubt or to always have the perfect solution immediately. Instead, aim to strike a balance between trusting your instincts and remaining open to learning and improvement. With practice and persistence, you’ll develop a strong intuition for solving coding problems efficiently and effectively.
As you continue your coding journey, platforms like AlgoCademy can provide valuable resources and practice opportunities to help you hone your skills and build confidence in your abilities. Embrace the process of continuous learning, trust in your growing expertise, and approach each coding challenge as an opportunity to further develop your instincts.
By mastering the art of trusting your coding instincts, you’ll not only perform better in interviews but also become a more efficient and confident programmer in your day-to-day work. So, the next time you face a coding problem, take a deep breath, trust your instincts, and code on with confidence!