Fail Gracefully: How to Handle Mistakes in a Coding Interview
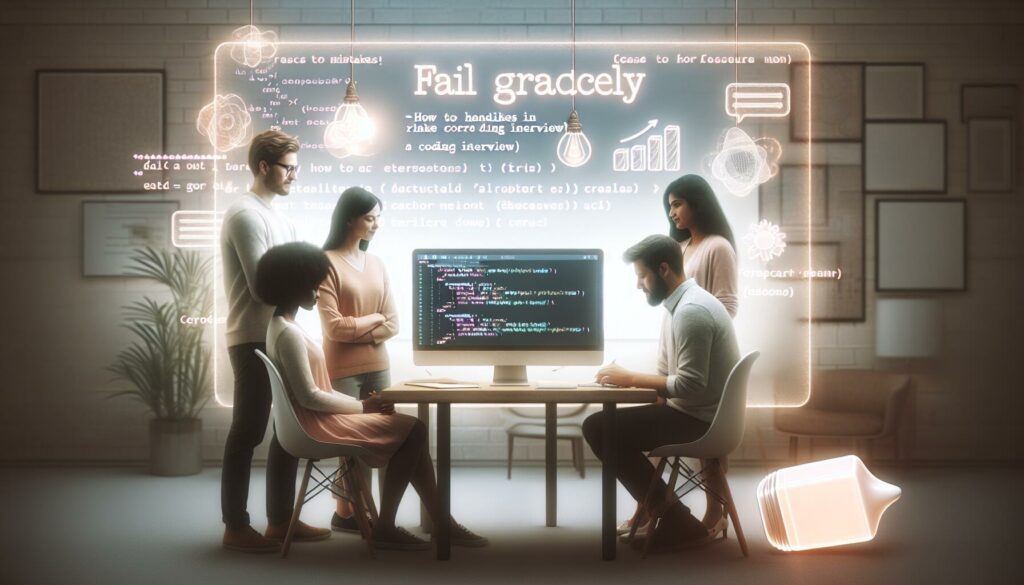
Coding interviews can be nerve-wracking experiences, even for seasoned developers. The pressure to perform well, coupled with the scrutiny of potential employers, can sometimes lead to mistakes. However, it’s not the mistakes themselves that define your performance, but rather how you handle them. In this comprehensive guide, we’ll explore the art of failing gracefully in a coding interview, providing you with strategies to turn potential setbacks into opportunities to showcase your problem-solving skills and professional demeanor.
The Importance of Graceful Failure in Coding Interviews
Before we dive into specific strategies, it’s crucial to understand why handling mistakes gracefully is so important in a coding interview:
- Demonstrates Professionalism: How you respond to errors shows your maturity and ability to handle pressure.
- Highlights Problem-Solving Skills: Your approach to fixing mistakes can showcase your analytical thinking.
- Reveals Communication Abilities: Clearly explaining your thought process during error correction is valuable.
- Shows Adaptability: Adjusting your approach when faced with challenges is a key skill in software development.
- Reflects Real-World Scenarios: In actual work environments, how you handle and learn from mistakes is often more important than avoiding them entirely.
Strategies for Handling Mistakes Gracefully
1. Stay Calm and Composed
When you realize you’ve made a mistake, it’s natural to feel a surge of panic. However, maintaining composure is crucial. Take a deep breath and remember that mistakes are a normal part of the coding process, even for experienced developers.
Example: If you notice an error in your code, you might say something like, “I see there’s an issue here. Let me take a moment to review and address it.”
2. Acknowledge the Mistake
Once you’ve identified an error, acknowledge it openly. Trying to hide or glossing over mistakes can come across as dishonest or lacking in self-awareness.
Example: “I realize I’ve made an error in my logic here. Thank you for your patience as I work through this.”
3. Explain Your Thought Process
As you work to correct the mistake, verbalize your thought process. This gives the interviewer insight into your problem-solving approach and demonstrates your ability to think critically under pressure.
Example: “I initially thought this approach would work because [explanation], but now I see that [issue]. Let me walk you through how I’m going to correct this.”
4. Take Ownership
Avoid making excuses or deflecting blame. Taking responsibility for your mistakes shows maturity and a growth mindset.
Example: “I made an assumption about the input data that wasn’t correct. That’s on me, and I’ll be more careful to validate my assumptions moving forward.”
5. Focus on the Solution
While it’s important to acknowledge the mistake, don’t dwell on it. Shift your focus quickly to finding and implementing a solution.
Example: “Now that I’ve identified the issue, here’s how I plan to resolve it: First, I’ll…”
6. Learn and Improve
Use the mistake as an opportunity to demonstrate your ability to learn and adapt quickly. Show the interviewer that you can take feedback constructively and apply it immediately.
Example: “Thank you for pointing that out. I see now how this approach is more efficient. I’ll keep this in mind for similar problems in the future.”
7. Ask for Clarification if Needed
If you’re unsure about something, it’s better to ask for clarification than to make assumptions that could lead to more mistakes.
Example: “Before I proceed with this correction, could you clarify if [specific aspect] is a requirement for this problem?”
Real-World Scenarios: Handling Common Coding Errors
Let’s look at some specific scenarios you might encounter in a coding interview and how to handle them gracefully:
Scenario 1: Syntax Error
You’re writing a function and realize you’ve made a syntax error.
function calculateSum(a, b { // Missing parenthesis
return a + b;
}
Graceful Response: “I see I’ve made a syntax error here. I forgot to close the parenthesis in the function declaration. Let me correct that right away.”
function calculateSum(a, b) { // Corrected
return a + b;
}
“There, I’ve added the missing parenthesis. It’s a small mistake, but it’s a good reminder of the importance of careful syntax checking. In a real development environment, I would typically catch this with linting tools or compiler errors.”
Scenario 2: Logic Error
You’re implementing a function to find the maximum number in an array, but your logic is flawed.
function findMax(arr) {
let max = arr[0];
for (let i = 0; i < arr.length; i++) {
if (arr[i] < max) { // Incorrect comparison
max = arr[i];
}
}
return max;
}
Graceful Response: “I’ve just realized there’s a logic error in my function. I’m currently finding the minimum value instead of the maximum. Let me correct that and explain the change.”
function findMax(arr) {
let max = arr[0];
for (let i = 0; i < arr.length; i++) {
if (arr[i] > max) { // Corrected comparison
max = arr[i];
}
}
return max;
}
“I’ve changed the comparison operator from ‘<‘ to ‘>’. This ensures we’re updating our ‘max’ variable when we find a larger number. Thank you for your patience as I made that correction. It’s a good reminder to always double-check the logic, especially in comparison operations.”
Scenario 3: Inefficient Algorithm
You’ve implemented a solution that works but is not optimized for performance.
function hasDuplicates(arr) {
for (let i = 0; i < arr.length; i++) {
for (let j = i + 1; j < arr.length; j++) {
if (arr[i] === arr[j]) {
return true;
}
}
}
return false;
}
Graceful Response: “I’ve implemented a solution that works, but I realize it’s not the most efficient approach. This nested loop gives us a time complexity of O(n^2). Let me think about how we can optimize this.”
“We could improve this by using a Set data structure, which would give us O(n) time complexity:”
function hasDuplicates(arr) {
const seen = new Set();
for (const item of arr) {
if (seen.has(item)) {
return true;
}
seen.add(item);
}
return false;
}
“This optimized version uses a Set to keep track of elements we’ve seen. It only requires a single pass through the array, significantly improving the time complexity. Thank you for allowing me to revisit and improve my initial solution.”
The Power of Self-Correction
One of the most impressive skills you can demonstrate in a coding interview is the ability to self-correct. This shows that you have:
- A deep understanding of the problem and potential solutions
- The ability to critically evaluate your own work
- The confidence to admit and correct mistakes
- A commitment to producing high-quality code
When you catch and correct your own mistakes before the interviewer points them out, you’re showcasing these valuable traits. However, it’s important to balance this with maintaining a good pace during the interview. Don’t get bogged down in perfectionism – if you’ve produced a working solution, you can always mention potential optimizations or improvements as a follow-up.
Learning from Mistakes: The Growth Mindset
Adopting a growth mindset is crucial for success in coding interviews and in your career as a developer. This mindset views challenges and mistakes not as failures, but as opportunities for learning and improvement. Here’s how you can apply this in a coding interview:
- Embrace Challenges: View difficult problems as opportunities to grow your skills.
- Persist in the Face of Setbacks: Don’t get discouraged by mistakes; use them as motivation to improve.
- Learn from Criticism: Take feedback constructively and use it to enhance your approach.
- Find Lessons in Others’ Success: If the interviewer suggests a better solution, show enthusiasm for learning this new approach.
By demonstrating a growth mindset, you show potential employers that you’re committed to continuous learning and improvement – highly valued traits in the fast-paced world of software development.
Communicating Effectively During Error Correction
Clear communication is vital when handling mistakes in a coding interview. Here are some tips to ensure you’re communicating effectively:
- Be Clear and Concise: Explain your thoughts succinctly without rambling.
- Use Technical Terms Accurately: Demonstrate your knowledge by using correct terminology.
- Ask Thoughtful Questions: If you need clarification, ask specific, well-formulated questions.
- Provide Context: Explain why you’re making certain decisions or changes.
- Be Honest About Uncertainty: If you’re not sure about something, it’s okay to say so.
Example: “I’m considering using a hash map to optimize this solution. It would change our space complexity to O(n), but reduce our time complexity from O(n^2) to O(n). Do you think that trade-off would be acceptable in this context?”
Handling Feedback Gracefully
Sometimes, the interviewer may point out mistakes or areas for improvement in your code. How you handle this feedback can significantly impact their perception of you. Here are some tips:
- Listen Actively: Pay close attention to the feedback without interrupting.
- Thank the Interviewer: Show appreciation for their insights.
- Ask for Clarification: If you don’t fully understand the feedback, ask for more details.
- Demonstrate Adaptability: Show how you can quickly incorporate the feedback into your solution.
- Reflect on the Feedback: Briefly mention how you’ll apply this learning in the future.
Example: “Thank you for that feedback. I see how using a binary search here would be more efficient. Let me implement that change now. This is a great reminder of the importance of considering logarithmic time complexity solutions for sorted data.”
Preparing for Graceful Failure
While it’s impossible to predict every mistake you might make in an interview, you can prepare yourself to handle errors gracefully. Here are some strategies:
- Practice Debugging: Regularly practice finding and fixing errors in code.
- Simulate Interview Conditions: Practice coding under time pressure to get used to the interview environment.
- Review Common Pitfalls: Familiarize yourself with common coding mistakes and how to avoid them.
- Develop a Checklist: Create a mental checklist to review your code for common errors.
- Learn to Think Aloud: Practice explaining your thought process as you code.
Remember, the goal isn’t to never make mistakes – it’s to handle them professionally when they do occur.
Conclusion: Embracing Imperfection in the Pursuit of Excellence
In the world of software development, mistakes are inevitable. What sets great developers apart is not an absence of errors, but the ability to handle them with grace, learn from them quickly, and use them as stepping stones to better solutions.
By mastering the art of failing gracefully in coding interviews, you demonstrate not just your technical skills, but also your professionalism, adaptability, and commitment to growth. These qualities are often just as important to potential employers as your ability to write perfect code.
Remember, a coding interview is not just a test of your programming skills – it’s an opportunity to showcase your problem-solving process, your ability to communicate technical concepts, and your capacity to perform under pressure. By approaching mistakes as learning opportunities and handling them with confidence and clarity, you can turn potential stumbling blocks into chances to shine.
As you prepare for your next coding interview, practice these strategies for graceful failure. Embrace challenges, communicate clearly, and always be ready to learn. With this mindset, you’ll be well-equipped to impress interviewers, not just with your successes, but with how you handle the bumps along the way.
Remember, in the journey of coding mastery, it’s not about never falling – it’s about how gracefully you get back up and continue forward. Good luck in your coding interviews, and may your failures be as impressive as your successes!