Don’t Just Write Code: How to Discuss Trade-offs in Time and Space Complexity
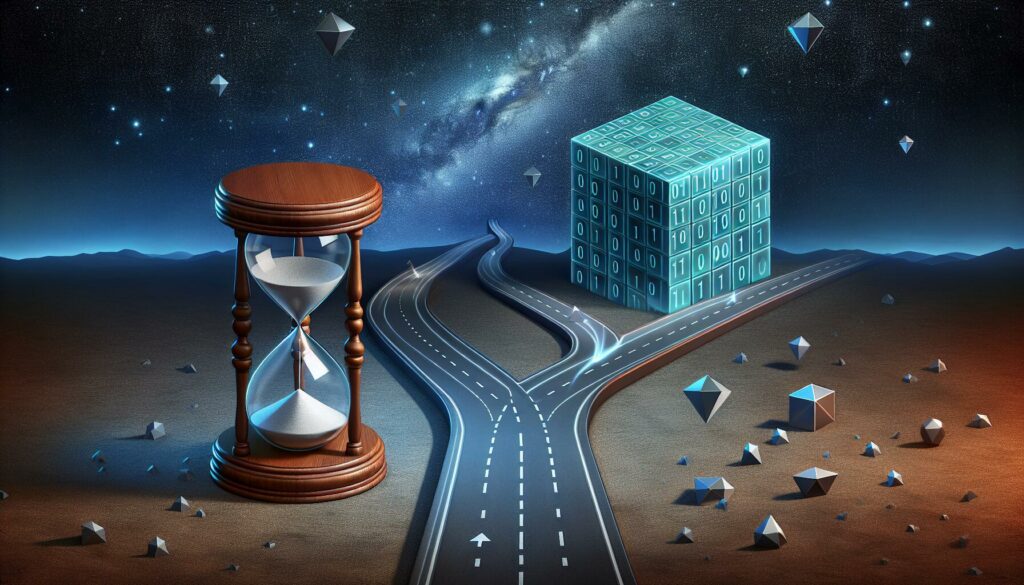
As software developers, we often find ourselves faced with multiple solutions to a single problem. While it’s essential to write code that works, it’s equally important to understand and articulate the trade-offs between different approaches. This skill becomes particularly crucial when discussing time complexity and space complexity – two fundamental concepts in algorithm analysis and optimization.
In this comprehensive guide, we’ll explore how to effectively discuss trade-offs in time and space complexity, providing you with the tools to make informed decisions and communicate your reasoning clearly. We’ll cover the following topics:
- Understanding Time and Space Complexity
- The Importance of Trade-off Discussions
- Analyzing Different Approaches
- Techniques for Clear and Concise Big O Analysis
- Real-world Examples and Case Studies
- Communicating Trade-offs to Different Audiences
- Common Pitfalls to Avoid
- Tools and Resources for Complexity Analysis
1. Understanding Time and Space Complexity
Before diving into trade-off discussions, it’s crucial to have a solid understanding of time and space complexity.
Time Complexity
Time complexity refers to the amount of time an algorithm takes to complete as a function of the input size. It’s typically expressed using Big O notation, which describes the upper bound of the growth rate of an algorithm’s running time.
Common time complexities include:
- O(1): Constant time
- O(log n): Logarithmic time
- O(n): Linear time
- O(n log n): Linearithmic time
- O(n^2): Quadratic time
- O(2^n): Exponential time
Space Complexity
Space complexity refers to the amount of memory an algorithm uses as a function of the input size. Like time complexity, it’s also expressed using Big O notation.
Common space complexities include:
- O(1): Constant space
- O(n): Linear space
- O(n^2): Quadratic space
2. The Importance of Trade-off Discussions
Discussing trade-offs in time and space complexity is crucial for several reasons:
- Optimization: Understanding trade-offs helps in optimizing algorithms for specific use cases.
- Resource Management: It allows for better management of computational resources, especially in resource-constrained environments.
- Scalability: Trade-off analysis helps in predicting how an algorithm will perform as the input size grows.
- Decision Making: It aids in making informed decisions about which algorithm to use in different scenarios.
- Communication: The ability to discuss trade-offs clearly is valuable when collaborating with team members or explaining design choices to stakeholders.
3. Analyzing Different Approaches
When analyzing different approaches to a problem, consider the following steps:
- Identify the Problem: Clearly define the problem you’re trying to solve.
- List Multiple Solutions: Come up with at least two or three different approaches to solve the problem.
- Analyze Time Complexity: Determine the time complexity of each solution.
- Analyze Space Complexity: Determine the space complexity of each solution.
- Consider Edge Cases: Think about how each solution performs in best-case, average-case, and worst-case scenarios.
- Identify Trade-offs: Compare the solutions based on their time and space complexities, as well as other factors like code readability and maintainability.
Let’s look at an example to illustrate this process.
Example: Finding a Pair with a Given Sum in an Array
Problem: Given an array of integers and a target sum, find a pair of numbers in the array that add up to the target sum.
Approach 1: Brute Force
def find_pair_brute_force(arr, target_sum):
n = len(arr)
for i in range(n):
for j in range(i+1, n):
if arr[i] + arr[j] == target_sum:
return (arr[i], arr[j])
return None
Time Complexity: O(n^2)
Space Complexity: O(1)
Approach 2: Using a Hash Table
def find_pair_hash_table(arr, target_sum):
complement_dict = {}
for num in arr:
complement = target_sum - num
if complement in complement_dict:
return (complement, num)
complement_dict[num] = True
return None
Time Complexity: O(n)
Space Complexity: O(n)
4. Techniques for Clear and Concise Big O Analysis
When explaining Big O analysis, use these techniques to make your explanations clear and concise:
- Focus on the Dominant Term: In Big O notation, we’re concerned with the term that grows the fastest as the input size increases. For example, O(n^2 + n) simplifies to O(n^2).
- Explain in Plain Language: After stating the Big O notation, explain what it means in simple terms. For example, “O(n) means the time taken grows linearly with the input size.”
- Use Analogies: Analogies can help make complex concepts more relatable. For instance, you could compare O(log n) to searching for a name in a phone book by repeatedly halving the search area.
- Provide Concrete Examples: Give examples of how the algorithm behaves with different input sizes. For example, “If we double the input size, an O(n) algorithm will take roughly twice as long to run.”
- Visualize Growth Rates: Use graphs or charts to visually represent how different complexities grow with input size.
- Highlight Key Operations: Identify the operations in your code that contribute most significantly to the time or space complexity.
5. Real-world Examples and Case Studies
Let’s examine a real-world scenario to illustrate how to discuss trade-offs in time and space complexity.
Case Study: Implementing a Cache
Scenario: You’re tasked with implementing a cache for frequently accessed data in a web application. You have two main approaches to consider:
- In-memory HashMap
- LRU (Least Recently Used) Cache
Approach 1: In-memory HashMap
class SimpleCache:
def __init__(self):
self.cache = {}
def get(self, key):
return self.cache.get(key)
def put(self, key, value):
self.cache[key] = value
Time Complexity:
- Get: O(1) average case
- Put: O(1) average case
Space Complexity: O(n), where n is the number of items in the cache
Approach 2: LRU Cache
from collections import OrderedDict
class LRUCache:
def __init__(self, capacity):
self.cache = OrderedDict()
self.capacity = capacity
def get(self, key):
if key not in self.cache:
return None
self.cache.move_to_end(key)
return self.cache[key]
def put(self, key, value):
if key in self.cache:
self.cache.move_to_end(key)
self.cache[key] = value
if len(self.cache) > self.capacity:
self.cache.popitem(last=False)
Time Complexity:
- Get: O(1)
- Put: O(1)
Space Complexity: O(capacity), where capacity is the maximum number of items the cache can hold
Trade-off Discussion
When discussing the trade-offs between these two approaches, we might say:
“Both the simple HashMap cache and the LRU cache offer constant-time O(1) operations for get and put in the average case. However, they differ in their space usage and behavior when reaching capacity limits.
The simple HashMap cache has no built-in size limit, potentially using O(n) space where n is the number of items stored. This could lead to excessive memory usage if not managed carefully. However, it’s simpler to implement and might be suitable for scenarios where we have a good estimate of the maximum number of items and memory isn’t a significant constraint.
On the other hand, the LRU cache maintains a fixed capacity, ensuring O(capacity) space complexity. It also provides the benefit of automatically evicting the least recently used items when it reaches capacity. This makes it more suitable for scenarios where we want to limit memory usage and prioritize recently accessed items.
The LRU cache comes with a slight increase in implementation complexity and a small overhead for maintaining the order of items. However, this overhead is generally negligible compared to the benefits it provides in managing memory usage and ensuring frequently accessed items are readily available.
In a real-world scenario, the choice between these two would depend on factors such as the expected number of items to be cached, the importance of limiting memory usage, and whether the recency of item access is a relevant factor for the application.”
6. Communicating Trade-offs to Different Audiences
The way you discuss trade-offs should be tailored to your audience. Here are some tips for communicating effectively with different groups:
For Technical Team Members
- Use precise technical terms and Big O notation
- Provide detailed analysis of time and space complexity
- Discuss implementation details and potential optimizations
- Use code examples to illustrate points
For Non-Technical Stakeholders
- Focus on high-level implications rather than technical details
- Use analogies and real-world examples to explain concepts
- Emphasize practical impacts on system performance, cost, or user experience
- Avoid jargon and explain necessary technical terms
For Project Managers
- Highlight trade-offs in terms of development time, maintenance effort, and scalability
- Discuss how different approaches align with project goals and constraints
- Provide clear recommendations with justifications
- Be prepared to discuss both short-term and long-term implications
7. Common Pitfalls to Avoid
When discussing trade-offs in time and space complexity, be aware of these common pitfalls:
- Overemphasizing Big O Notation: While Big O is important, it’s not the only factor. Consider average-case performance, constants, and real-world behavior.
- Ignoring Space Complexity: Time complexity often gets more attention, but space complexity can be equally important, especially in memory-constrained environments.
- Neglecting Other Factors: Don’t forget about other important aspects like code readability, maintainability, and development time.
- Assuming Worst-Case Scenarios: While it’s important to consider worst-case complexity, average-case performance might be more relevant in many real-world scenarios.
- Premature Optimization: Don’t sacrifice code clarity or development speed for minor performance gains unless profiling indicates a real need.
- Overlooking Hardware Considerations: The impact of algorithmic choices can vary depending on hardware characteristics like cache sizes and memory hierarchies.
8. Tools and Resources for Complexity Analysis
To aid in your analysis and discussions of time and space complexity, consider using these tools and resources:
- Profiling Tools: Use language-specific profilers to measure actual performance in real-world scenarios.
- Visualization Tools: Websites like Big-O Cheat Sheet provide visual comparisons of different time complexities.
- Algorithm Visualizers: Tools like VisuAlgo can help in understanding how different algorithms work.
- Online Judges: Platforms like LeetCode or HackerRank often provide time and space complexity information for submitted solutions.
- Books: “Introduction to Algorithms” by Cormen et al. and “The Algorithm Design Manual” by Skiena are excellent resources for deep dives into algorithmic analysis.
- Online Courses: Courses on platforms like Coursera or edX often cover algorithm analysis in depth.
Conclusion
Discussing trade-offs in time and space complexity is a crucial skill for any software developer. It goes beyond simply writing code that works – it’s about understanding the implications of our design choices and being able to articulate them clearly.
By following the techniques and avoiding the pitfalls outlined in this guide, you’ll be better equipped to analyze different approaches, make informed decisions, and communicate your reasoning effectively. Remember, the goal is not always to choose the solution with the best Big O complexity, but to find the approach that best balances performance, resource usage, and other practical considerations for your specific use case.
As you continue to develop this skill, you’ll find that it not only improves your own understanding and decision-making but also enhances your ability to collaborate with team members and explain technical concepts to various stakeholders. Keep practicing, stay curious about different algorithmic approaches, and always be ready to discuss not just what your code does, but why you chose to do it that way.