Refactoring Code: When and How to Clean Up Your Project for Long-Term Maintenance
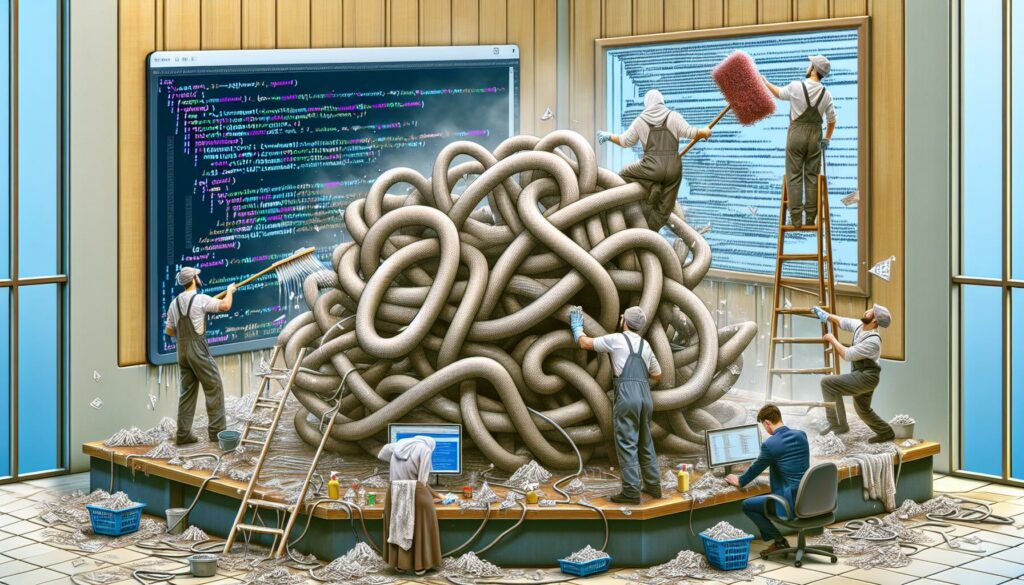
In the world of software development, writing code is just the beginning. As projects grow and evolve, maintaining clean, efficient, and readable code becomes increasingly important. This is where refactoring comes into play. Refactoring is the process of restructuring existing code without changing its external behavior. It’s a crucial skill for any developer looking to improve their craft and ensure the longevity of their projects.
In this comprehensive guide, we’ll explore the importance of refactoring, discuss when it’s appropriate to refactor your code, and provide practical examples of how to clean up common programming patterns. Whether you’re a beginner looking to improve your coding skills or an experienced developer preparing for technical interviews at major tech companies, understanding the art of refactoring is essential for your growth as a programmer.
The Importance of Refactoring
Before we dive into the specifics of when and how to refactor, let’s first understand why refactoring is so important in software development:
1. Improved Readability
Code that is easy to read is easy to understand. When you refactor your code to improve its readability, you’re not just doing yourself a favor – you’re helping every developer who will work on the project in the future. Clear, well-structured code reduces the time needed to comprehend and modify the codebase, leading to faster development cycles and fewer bugs.
2. Enhanced Maintainability
As projects grow, maintaining them becomes increasingly challenging. Refactoring helps keep your code manageable by breaking down complex functions, eliminating duplications, and organizing your codebase in a logical manner. This makes it easier to add new features, fix bugs, and adapt to changing requirements without introducing new problems.
3. Increased Efficiency
Refactoring often leads to more efficient code. By simplifying complex algorithms, removing redundant operations, and optimizing data structures, you can improve your program’s performance. This is particularly important when preparing for technical interviews at major tech companies, where efficiency and optimization are key focus areas.
4. Better Extensibility
Well-refactored code is typically more modular and follows good design principles. This makes it easier to extend your codebase with new features or adapt it to new requirements. Extensibility is crucial for long-term project success and can save significant time and resources in the future.
5. Easier Debugging
When code is clean and well-organized, identifying and fixing bugs becomes much simpler. Refactoring can help isolate problematic areas of your code, making it easier to pinpoint the source of issues and implement fixes without introducing new bugs.
When to Refactor Your Code
Knowing when to refactor is just as important as knowing how to refactor. Here are some key indicators that it might be time to clean up your code:
1. Code Smells
“Code smells” are surface indications that usually correspond to deeper problems in the system. Some common code smells include:
- Duplicated code
- Long methods or functions
- Large classes
- Long parameter lists
- Excessive comments (often indicating overly complex code)
- Nested conditionals
If you notice these patterns in your code, it’s often a sign that refactoring could improve your codebase.
2. Adding New Features
When you’re about to add a new feature to your project, it’s an excellent opportunity to refactor. Cleaning up the existing code can make it easier to integrate new functionality and ensure that your additions fit well with the existing structure.
3. Fixing Bugs
When you’re fixing a bug, you’re already diving deep into a specific part of your code. This is a perfect time to refactor the surrounding code to prevent similar bugs in the future and improve the overall quality of that section.
4. Code Reviews
Code reviews are an excellent opportunity to identify areas that need refactoring. Fresh eyes can often spot inefficiencies or confusing structures that the original author might have missed.
5. Before Major Updates or Migrations
If you’re planning a significant update to your project or migrating to a new framework or language version, refactoring beforehand can make the transition smoother and help identify potential issues early.
6. Performance Optimization
When you need to optimize your code for better performance, refactoring is often the first step. It can help you identify bottlenecks and streamline your algorithms.
How to Refactor: Common Patterns and Examples
Now that we understand the importance of refactoring and when to do it, let’s look at some common refactoring patterns with examples. These patterns are particularly relevant for those preparing for technical interviews at major tech companies, as they demonstrate your ability to write clean, efficient, and maintainable code.
1. Extract Method
The Extract Method pattern involves taking a piece of code from within a method and turning it into its own method. This is useful for breaking down long methods and improving readability.
Before refactoring:
def calculate_total_price(items):
total = 0
for item in items:
if item.is_on_sale():
total += item.price * 0.9
else:
total += item.price
if total > 100:
total *= 0.95
return total
After refactoring:
def calculate_total_price(items):
total = sum_item_prices(items)
return apply_bulk_discount(total)
def sum_item_prices(items):
return sum(item.price * 0.9 if item.is_on_sale() else item.price for item in items)
def apply_bulk_discount(total):
return total * 0.95 if total > 100 else total
In this example, we’ve extracted the price calculation and discount application into separate methods. This makes the code more readable and easier to maintain.
2. Replace Conditional with Polymorphism
This pattern is useful when you have a conditional that chooses different behavior depending on the type of an object. It’s a great way to make your code more object-oriented and extensible.
Before refactoring:
class Animal:
def __init__(self, type):
self.type = type
def make_sound(self):
if self.type == 'dog':
return 'Woof!'
elif self.type == 'cat':
return 'Meow!'
elif self.type == 'cow':
return 'Moo!'
else:
return 'Unknown animal'
After refactoring:
class Animal:
def make_sound(self):
pass
class Dog(Animal):
def make_sound(self):
return 'Woof!'
class Cat(Animal):
def make_sound(self):
return 'Meow!'
class Cow(Animal):
def make_sound(self):
return 'Moo!'
This refactored version uses polymorphism to handle different animal types. It’s more extensible (easy to add new animal types) and follows the Open/Closed Principle of SOLID design principles.
3. Remove Duplicate Code
Duplicate code is a common issue that can lead to maintenance nightmares. Refactoring to remove duplication can significantly improve your codebase.
Before refactoring:
def process_user_input(input):
# Validate input
if not input:
print("Input cannot be empty")
return False
if len(input) < 3:
print("Input must be at least 3 characters long")
return False
# Process input
processed_input = input.strip().lower()
# Save to database
save_to_db(processed_input)
return True
def process_admin_input(input):
# Validate input
if not input:
print("Input cannot be empty")
return False
if len(input) < 3:
print("Input must be at least 3 characters long")
return False
# Process input
processed_input = input.strip().upper()
# Save to database
save_to_db(processed_input)
return True
After refactoring:
def validate_input(input):
if not input:
print("Input cannot be empty")
return False
if len(input) < 3:
print("Input must be at least 3 characters long")
return False
return True
def process_input(input, transform_func):
if not validate_input(input):
return False
processed_input = transform_func(input.strip())
save_to_db(processed_input)
return True
def process_user_input(input):
return process_input(input, str.lower)
def process_admin_input(input):
return process_input(input, str.upper)
In this refactored version, we’ve extracted the common validation logic into a separate function and used a higher-order function to handle the different processing requirements for user and admin inputs.
4. Replace Temp with Query
This refactoring technique involves replacing temporary variables with method calls. It can make your code more flexible and easier to understand.
Before refactoring:
class Order:
def __init__(self, quantity, item_price):
self.quantity = quantity
self.item_price = item_price
def get_total_price(self):
base_price = self.quantity * self.item_price
if base_price > 1000:
discount_factor = 0.95
else:
discount_factor = 0.98
return base_price * discount_factor
After refactoring:
class Order:
def __init__(self, quantity, item_price):
self.quantity = quantity
self.item_price = item_price
def get_total_price(self):
return self.base_price() * self.discount_factor()
def base_price(self):
return self.quantity * self.item_price
def discount_factor(self):
return 0.95 if self.base_price() > 1000 else 0.98
In this refactored version, we’ve replaced the temporary variables with method calls. This makes the code more flexible (e.g., we can easily override the discount_factor method in a subclass) and self-documenting.
5. Introduce Parameter Object
When a method has a long list of parameters, it can be beneficial to group some of these parameters into a single object. This can make your code more organized and easier to understand.
Before refactoring:
def create_user(name, email, age, address, city, country, phone):
# Create user logic here
pass
create_user("John Doe", "john@example.com", 30, "123 Main St", "New York", "USA", "1234567890")
After refactoring:
class UserInfo:
def __init__(self, name, email, age, address, city, country, phone):
self.name = name
self.email = email
self.age = age
self.address = address
self.city = city
self.country = country
self.phone = phone
def create_user(user_info):
# Create user logic here
pass
user_info = UserInfo("John Doe", "john@example.com", 30, "123 Main St", "New York", "USA", "1234567890")
create_user(user_info)
This refactoring makes the code more organized and easier to extend in the future. If we need to add more user information, we can simply add it to the UserInfo class without changing the create_user function signature.
Best Practices for Refactoring
While refactoring can greatly improve your code, it’s important to approach it systematically to avoid introducing new bugs or complications. Here are some best practices to keep in mind:
1. Test Before and After
Always have a solid set of tests in place before you start refactoring. Run these tests before and after each refactoring step to ensure you haven’t changed the behavior of your code. This is especially crucial when preparing for technical interviews, where the correctness of your code is paramount.
2. Refactor in Small Steps
Don’t try to refactor everything at once. Make small, incremental changes and test after each change. This approach, often called “baby steps” in refactoring, helps you maintain control over the process and makes it easier to identify and fix any issues that arise.
3. Use Version Control
Always use version control when refactoring. This allows you to easily revert changes if something goes wrong and helps you keep track of the improvements you’ve made. Git, for example, is an essential tool for any developer and is often a topic of discussion in technical interviews.
4. Understand the Code First
Before refactoring any piece of code, make sure you fully understand what it does. This might involve adding temporary debug statements, using a debugger, or discussing the code with team members who are familiar with it.
5. Follow Coding Standards
When refactoring, ensure that your changes adhere to your project’s or team’s coding standards. Consistency in code style is important for readability and maintainability.
6. Document Your Changes
If your refactoring involves significant changes to the code structure or API, make sure to update any relevant documentation. This includes inline comments, function documentation, and any external documentation or README files.
7. Review Your Refactored Code
If possible, have a colleague review your refactored code. A fresh pair of eyes can often spot issues or suggest further improvements that you might have missed.
Refactoring and Technical Interviews
Understanding and applying refactoring techniques can be a significant advantage when preparing for technical interviews, especially at major tech companies. Here’s why:
1. Demonstrates Code Quality Awareness
By refactoring your code during an interview or discussing potential refactorings, you show that you’re aware of code quality issues and are proactive about improving them. This is a highly valued trait in professional software development.
2. Shows Problem-Solving Skills
Refactoring often involves creative problem-solving. Being able to identify areas for improvement and come up with effective solutions demonstrates your analytical and problem-solving abilities.
3. Indicates Experience and Maturity
Knowledge of refactoring techniques often comes with experience. By applying these techniques, you demonstrate a level of maturity in your coding practices that can set you apart from other candidates.
4. Aligns with Best Practices
Many refactoring techniques align closely with software development best practices and design patterns. Showing familiarity with these concepts can impress interviewers and demonstrate your commitment to writing high-quality code.
5. Improves Code Efficiency
Many refactoring techniques can lead to more efficient code. In technical interviews, where optimal solutions are often sought, the ability to refactor for improved performance can be a significant advantage.
Conclusion
Refactoring is an essential skill for any developer looking to write clean, efficient, and maintainable code. By understanding when to refactor and how to apply common refactoring patterns, you can significantly improve the quality of your projects and your effectiveness as a programmer.
Remember, refactoring is not just about cleaning up code – it’s about continuously improving your codebase to make it more readable, efficient, and extensible. Whether you’re working on a personal project, collaborating in a team, or preparing for technical interviews at major tech companies, the ability to refactor effectively will serve you well throughout your programming career.
As you continue to develop your coding skills, make refactoring a regular part of your development process. Practice identifying code smells, experiment with different refactoring techniques, and always strive to leave the code better than you found it. With time and practice, refactoring will become second nature, helping you write better code and solve complex problems more effectively.
Remember, the journey to becoming a skilled programmer is ongoing, and refactoring is a powerful tool in your development toolkit. Keep learning, keep practicing, and keep refactoring!