Handling Multiple Solutions: How to Present Alternatives Without Confusing the Interviewer
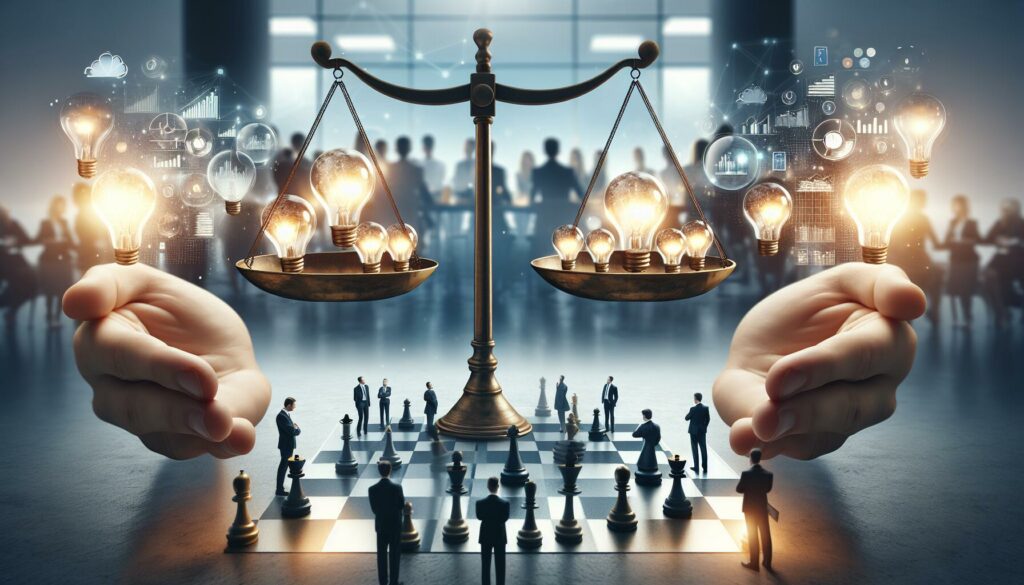
In the world of coding interviews, particularly when aiming for positions at top tech companies like FAANG (Facebook, Amazon, Apple, Netflix, Google), it’s not uncommon to encounter problems that have multiple valid solutions. While this demonstrates the richness and flexibility of programming, it can also present a challenge: How do you effectively communicate these various approaches without overwhelming or confusing your interviewer? This article will guide you through the process of presenting multiple solutions clearly and confidently, helping you showcase your problem-solving skills and adaptability.
The Importance of Clarity in Technical Interviews
Before diving into the specifics of presenting multiple solutions, it’s crucial to understand why clarity is paramount in technical interviews. Clear communication is not just about impressing the interviewer; it’s about demonstrating your ability to work effectively in a team environment, where you’ll need to explain your ideas and code to colleagues regularly.
Here are some key reasons why clarity matters:
- Demonstrating thought process: Clear explanations allow the interviewer to follow your reasoning and understand how you approach problem-solving.
- Showcasing communication skills: The ability to articulate complex ideas simply is a valuable skill in any development role.
- Avoiding misunderstandings: Clear communication reduces the risk of the interviewer misinterpreting your solution or intentions.
- Efficient use of time: In a time-constrained interview, clarity helps you convey your ideas quickly and effectively.
Strategies for Presenting Multiple Solutions
When you’ve identified multiple ways to solve a problem, follow these strategies to present them effectively:
1. Start with an Overview
Begin by briefly mentioning that you’ve identified multiple approaches. This sets the stage for your detailed explanations and shows the interviewer that you’ve considered the problem from various angles.
Example:
"I've thought of three potential solutions to this problem. Each has its own trade-offs in terms of time complexity, space complexity, and implementation simplicity. I'd like to walk you through each of them if that's okay."
2. Prioritize and Order Your Solutions
Present your solutions in a logical order. This could be from simplest to most complex, from least efficient to most efficient, or in the order you thought of them. Explain your ordering choice to the interviewer.
Example:
"I'll present these solutions in order of increasing efficiency, starting with the most straightforward approach and moving to more optimized versions."
3. Provide a High-Level Description of Each Solution
For each solution, start with a brief, high-level description before diving into the details. This gives the interviewer a mental framework to understand your subsequent explanation.
Example:
"The first solution uses a brute-force approach with nested loops. The second utilizes a hash map to improve time complexity. The third employs a two-pointer technique for optimal space efficiency."
4. Use Clear, Consistent Terminology
Stick to widely accepted programming terms and concepts. If you use any specialized terminology, briefly define it to ensure you and the interviewer are on the same page.
5. Highlight Trade-offs
For each solution, clearly articulate its advantages and disadvantages. This demonstrates your ability to critically evaluate different approaches.
Example:
"The hash map solution reduces time complexity from O(n^2) to O(n), but it increases space complexity to O(n). It's a classic space-time trade-off."
6. Use Visual Aids When Possible
If you’re in a setting where you can use a whiteboard or shared screen, utilize diagrams or pseudo-code to illustrate your solutions. Visual representations can greatly enhance understanding.
7. Invite Feedback
After presenting each solution, pause to allow the interviewer to ask questions or provide feedback. This ensures they’re following your explanation and gives you a chance to clarify any points if needed.
Deciding Which Solution to Implement First
After presenting multiple solutions, you’ll often need to choose one to implement. Here’s how to approach this decision:
1. Consider the Interview Context
Think about what the interviewer might be looking for based on the company, role, or any hints they’ve given. For example, if you’re interviewing for a role that emphasizes optimization, you might lean towards the most efficient solution.
2. Evaluate Trade-offs
Weigh the pros and cons of each solution in the context of real-world scenarios. Consider factors like:
- Time complexity
- Space complexity
- Readability and maintainability
- Scalability
- Potential for future modifications
3. State Your Preference and Reasoning
Once you’ve made a decision, clearly state which solution you prefer and explain why. This showcases your decision-making skills and ability to justify technical choices.
Example:
"Given the constraints mentioned in the problem statement, I believe the hash map solution offers the best balance of efficiency and simplicity. It significantly improves time complexity without excessive space usage, and the code remains quite readable. Would you like me to implement this version?"
4. Be Open to Guidance
Remember that the interviewer might have a specific solution in mind or want to see how you handle implementing a particular approach. Be prepared to pivot based on their feedback.
Implementing Your Chosen Solution
Once you’ve decided on a solution to implement, follow these steps to ensure a smooth coding process:
1. Outline Your Approach
Before diving into coding, briefly outline the steps you’ll take. This helps organize your thoughts and allows the interviewer to follow your implementation.
2. Write Clean, Readable Code
Even if you’re writing on a whiteboard or in a simple text editor, strive for clean, well-organized code. Use meaningful variable names, proper indentation, and clear comments where necessary.
3. Think Aloud
As you code, verbalize your thought process. This gives the interviewer insight into your problem-solving approach and allows them to provide hints if you get stuck.
4. Handle Edge Cases
Address potential edge cases in your implementation. This shows attention to detail and thorough problem-solving skills.
5. Test Your Solution
Once you’ve finished coding, walk through your solution with a few test cases, including edge cases. This demonstrates your testing and debugging skills.
Example Scenario: Two Sum Problem
Let’s walk through an example of how to present multiple solutions for a common coding interview problem: the Two Sum problem.
Problem Statement: Given an array of integers and a target sum, return the indices of two numbers in the array that add up to the target sum.
Presentation:
"I've identified three potential solutions to this problem. I'll present them in order of increasing efficiency.
1. Brute Force Approach:
- This involves using nested loops to check every pair of numbers.
- Time Complexity: O(n^2)
- Space Complexity: O(1)
- Advantage: Simple to implement and understand.
- Disadvantage: Inefficient for large inputs.
2. Hash Map Approach:
- We can use a hash map to store complements as we iterate through the array.
- Time Complexity: O(n)
- Space Complexity: O(n)
- Advantage: Significantly improved time complexity.
- Disadvantage: Uses additional space.
3. Two-Pointer Approach (if the array is sorted):
- We can use two pointers, one at the start and one at the end, moving them based on the sum.
- Time Complexity: O(n log n) if sorting is needed, O(n) if already sorted.
- Space Complexity: O(1)
- Advantage: Optimal space usage.
- Disadvantage: Requires the array to be sorted.
Given that the problem doesn't specify whether the array is sorted, I would recommend implementing the Hash Map approach. It offers the best balance of time efficiency and simplicity without making assumptions about the input. Would you like me to proceed with this implementation?"
Common Pitfalls to Avoid
When presenting multiple solutions, be wary of these common mistakes:
1. Information Overload
Avoid going into excessive detail for each solution. Provide enough information to differentiate between approaches without overwhelming the interviewer.
2. Lack of Structure
Present your solutions in a structured manner. Jumping between different ideas without a clear organization can confuse the interviewer.
3. Ignoring the Interviewer
Pay attention to the interviewer’s reactions and questions. If they seem particularly interested in one approach, be prepared to focus on that.
4. Underestimating Simple Solutions
Don’t dismiss simpler solutions too quickly. Sometimes, a straightforward approach is the most appropriate, especially if it meets all the requirements efficiently.
5. Overcomplicating Explanations
Use clear, concise language. Avoid unnecessary jargon or overly complex explanations that might obscure your main points.
Conclusion
Presenting multiple solutions in a coding interview is an opportunity to showcase your problem-solving skills, critical thinking, and communication abilities. By following the strategies outlined in this article, you can effectively communicate your ideas without confusing or overwhelming the interviewer.
Remember, the key is to be clear, organized, and thoughtful in your presentation. Start with an overview, prioritize your solutions, highlight trade-offs, and be prepared to implement your chosen approach. With practice, you’ll become more comfortable discussing multiple solutions, a skill that will serve you well not only in interviews but also in your future career as a software developer.
As you continue to prepare for coding interviews, particularly for positions at top tech companies, remember that platforms like AlgoCademy offer valuable resources and practice problems to hone your skills. By combining theoretical knowledge with practical coding experience, you’ll be well-equipped to handle any challenge that comes your way in your next technical interview.