Writing Tests for Your Project: Why TDD (Test-Driven Development) Should Be Your New Best Friend
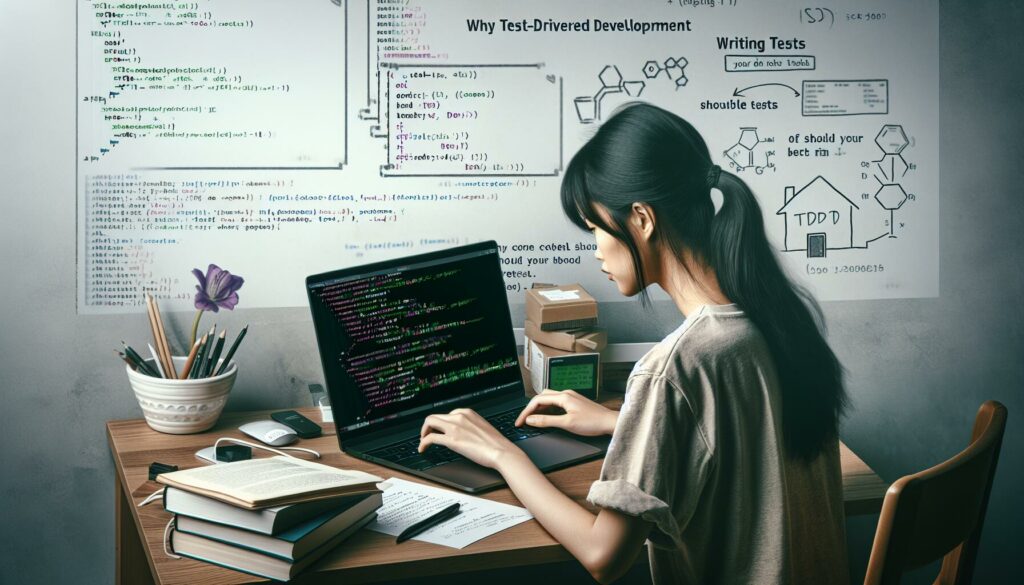
In the ever-evolving world of software development, writing clean, efficient, and bug-free code is paramount. As projects grow in complexity, maintaining code quality becomes increasingly challenging. This is where Test-Driven Development (TDD) comes into play, offering a powerful approach to ensure your code is robust, reliable, and ready for any curveball that might come its way. In this comprehensive guide, we’ll explore the concept of TDD, its benefits, and how you can implement it in your projects to level up your coding game.
What is Test-Driven Development (TDD)?
Test-Driven Development is a software development approach where tests are written before the actual code. This might sound counterintuitive at first, but it’s a practice that has gained significant traction in the programming community due to its numerous benefits. The TDD process typically follows these steps:
- Write a test for a specific function or feature
- Run the test (which should fail since the code doesn’t exist yet)
- Write the minimal amount of code to make the test pass
- Run the test again (it should pass this time)
- Refactor the code to improve its structure and readability
- Repeat the process for the next function or feature
This cycle is often referred to as “Red-Green-Refactor,” where red represents the failing test, green represents the passing test, and refactor is the step where you improve your code without changing its functionality.
Why TDD Should Be Your New Best Friend
Now that we understand what TDD is, let’s dive into why it should become an integral part of your development process:
1. Cleaner, More Focused Code
When you write tests first, you’re forced to think about the specific requirements and behavior of your code before you start implementing it. This leads to more focused and purposeful code that does exactly what it’s supposed to do, nothing more and nothing less. You’re less likely to write unnecessary or redundant code when you have a clear test guiding your implementation.
2. Improved Code Design
TDD encourages modular and loosely coupled code. Since you’re writing tests for individual functions or components, you naturally tend to design your code in a way that’s easily testable. This often results in better overall architecture and more maintainable code in the long run.
3. Faster Debugging
With a comprehensive test suite, identifying and fixing bugs becomes much easier. When a test fails, you know exactly which part of your code is causing the issue, allowing you to pinpoint and resolve problems quickly. This can save countless hours of debugging time, especially in larger projects.
4. Confidence in Code Changes
Having a robust set of tests gives you the confidence to make changes or refactor your code without fear of breaking existing functionality. If your tests still pass after making changes, you can be reasonably sure that your modifications haven’t introduced new bugs.
5. Living Documentation
Well-written tests serve as documentation for your code. They describe the expected behavior of your functions and components, making it easier for other developers (or your future self) to understand how the code is supposed to work.
6. Improved Collaboration
TDD can enhance collaboration within development teams. When everyone follows TDD practices, it’s easier to review code, understand its purpose, and contribute to the project without inadvertently breaking existing functionality.
Getting Started with TDD: A Practical Example
Now that we’ve covered the benefits of TDD, let’s walk through a practical example to see how it works in action. We’ll create a simple calculator module with basic arithmetic operations and write tests for each function.
For this example, we’ll use JavaScript and the Jest testing framework, but the principles apply to any programming language and testing framework.
Step 1: Setting Up the Project
First, let’s set up a new Node.js project and install Jest:
mkdir calculator-tdd
cd calculator-tdd
npm init -y
npm install --save-dev jest
Update the package.json
file to use Jest as the test runner:
{
"scripts": {
"test": "jest"
}
}
Step 2: Writing Our First Test
Let’s start by writing a test for an addition function. Create a new file called calculator.test.js
:
const calculator = require('./calculator');
describe('Calculator', () => {
test('adds two numbers correctly', () => {
expect(calculator.add(2, 3)).toBe(5);
});
});
This test expects an add
function that takes two numbers and returns their sum.
Step 3: Running the Test (Red)
Run the test using the command:
npm test
The test should fail because we haven’t implemented the calculator
module or the add
function yet.
Step 4: Implementing the Code (Green)
Now, let’s create the calculator.js
file and implement the add
function:
const calculator = {
add: (a, b) => a + b
};
module.exports = calculator;
Run the test again, and it should pass.
Step 5: Refactoring (if necessary)
In this case, our implementation is simple and doesn’t require refactoring. However, in more complex scenarios, this is where you’d improve your code structure without changing its functionality.
Step 6: Repeat for Other Functions
Let’s add tests and implementations for subtraction, multiplication, and division:
// In calculator.test.js
describe('Calculator', () => {
test('adds two numbers correctly', () => {
expect(calculator.add(2, 3)).toBe(5);
});
test('subtracts two numbers correctly', () => {
expect(calculator.subtract(5, 3)).toBe(2);
});
test('multiplies two numbers correctly', () => {
expect(calculator.multiply(2, 3)).toBe(6);
});
test('divides two numbers correctly', () => {
expect(calculator.divide(6, 2)).toBe(3);
});
test('throws an error when dividing by zero', () => {
expect(() => calculator.divide(6, 0)).toThrow('Cannot divide by zero');
});
});
Now, let’s implement these functions in calculator.js
:
const calculator = {
add: (a, b) => a + b,
subtract: (a, b) => a - b,
multiply: (a, b) => a * b,
divide: (a, b) => {
if (b === 0) {
throw new Error('Cannot divide by zero');
}
return a / b;
}
};
module.exports = calculator;
Run the tests again, and they should all pass.
Advanced TDD Concepts
As you become more comfortable with TDD, you can explore more advanced concepts and techniques:
1. Test Coverage
Test coverage is a metric that measures how much of your code is executed by your tests. While 100% coverage doesn’t guarantee bug-free code, it’s a good indicator of how comprehensive your tests are. Many testing frameworks, including Jest, provide built-in coverage reporting tools.
2. Mocking and Stubbing
When testing complex systems, you often need to isolate the component you’re testing from its dependencies. Mocking and stubbing are techniques that allow you to create fake versions of these dependencies, giving you more control over the testing environment.
3. Integration Tests
While unit tests focus on individual functions or components, integration tests ensure that different parts of your application work correctly together. These tests are crucial for catching issues that might arise from the interaction between components.
4. Behavior-Driven Development (BDD)
BDD is an extension of TDD that focuses on describing the behavior of the system from the user’s perspective. It often uses a more natural language syntax for writing tests, making them more accessible to non-technical stakeholders.
Common TDD Pitfalls and How to Avoid Them
While TDD offers numerous benefits, there are some common pitfalls to be aware of:
1. Over-testing
It’s possible to write too many tests, which can lead to increased maintenance overhead. Focus on writing meaningful tests that cover important functionality and edge cases, rather than trying to test every possible scenario.
2. Tightly Coupled Tests
If your tests are too closely tied to the implementation details of your code, they can become brittle and break easily when you refactor. Write tests that focus on the behavior and output of your functions, not their internal workings.
3. Neglecting Test Maintenance
As your project evolves, your tests need to evolve with it. Regularly review and update your tests to ensure they remain relevant and effective.
4. Slow Test Suites
As your project grows, your test suite can become slow to run, which can discourage developers from running tests frequently. Optimize your tests, use parallel testing when possible, and consider separating quick unit tests from slower integration tests.
Integrating TDD into Your Development Workflow
Adopting TDD can be challenging, especially if you’re used to writing code first and tests later (or not at all). Here are some tips to help you integrate TDD into your development workflow:
1. Start Small
Begin by applying TDD to a small, manageable part of your project. As you become more comfortable with the process, gradually expand its use to other areas.
2. Use Continuous Integration (CI)
Implement a CI system that runs your tests automatically whenever code is pushed to your repository. This ensures that all changes are tested and helps catch issues early.
3. Pair Programming
Practice TDD with a colleague through pair programming. This can help reinforce good habits and provide immediate feedback on your approach.
4. Refactor Regularly
Don’t skip the refactoring step in the TDD cycle. Regular refactoring helps keep your code clean and maintainable.
5. Educate Your Team
If you’re working in a team, ensure everyone understands the principles and benefits of TDD. Consider organizing workshops or code reviews focused on TDD practices.
TDD and AlgoCademy: Enhancing Your Coding Skills
As you progress in your coding journey with AlgoCademy, incorporating TDD into your practice can significantly enhance your skills and prepare you for real-world development scenarios. Here’s how TDD aligns with AlgoCademy’s focus areas:
1. Algorithmic Thinking
TDD encourages you to think critically about the problem at hand before writing any code. This aligns perfectly with AlgoCademy’s emphasis on algorithmic thinking. By writing tests first, you’re forced to consider edge cases and different scenarios, which is crucial when designing efficient algorithms.
2. Problem-Solving Skills
The process of writing tests before implementation hones your problem-solving skills. It trains you to break down complex problems into smaller, testable units – a valuable skill for tackling the challenging coding problems you’ll encounter in technical interviews.
3. Practical Coding Skills
TDD isn’t just a theoretical concept; it’s a practical skill used in many professional development environments. By incorporating TDD into your coding practice, you’re developing skills that are directly applicable to real-world software development, especially in major tech companies.
4. Preparation for Technical Interviews
Many technical interviews, especially at FAANG companies, involve live coding exercises. The ability to think through a problem, consider edge cases, and write clean, testable code – all skills developed through TDD – can give you a significant advantage in these high-pressure situations.
5. Code Quality and Efficiency
AlgoCademy emphasizes not just solving problems, but solving them efficiently and writing high-quality code. TDD naturally leads to cleaner, more efficient code by encouraging you to write only what’s necessary to pass the tests.
Conclusion: Embracing TDD for Better Code and Better Skills
Test-Driven Development is more than just a coding practice; it’s a mindset that can transform the way you approach software development. By writing tests first, you’re not only ensuring the reliability and maintainability of your code but also developing crucial skills that will serve you well throughout your programming career.
As you continue your journey with AlgoCademy, consider incorporating TDD into your coding practice. Start small, perhaps by writing tests for the solutions to coding challenges before implementing them. As you become more comfortable with the process, you’ll likely find that it not only improves your code quality but also enhances your problem-solving skills and prepares you better for technical interviews.
Remember, becoming proficient in TDD takes time and practice. Be patient with yourself, embrace the learning process, and soon you’ll find that TDD is indeed your new best friend in the world of coding. Happy testing, and may your code always be bug-free!