Mastering Documentation: Why Writing Good Docs Helps You Build Better Projects
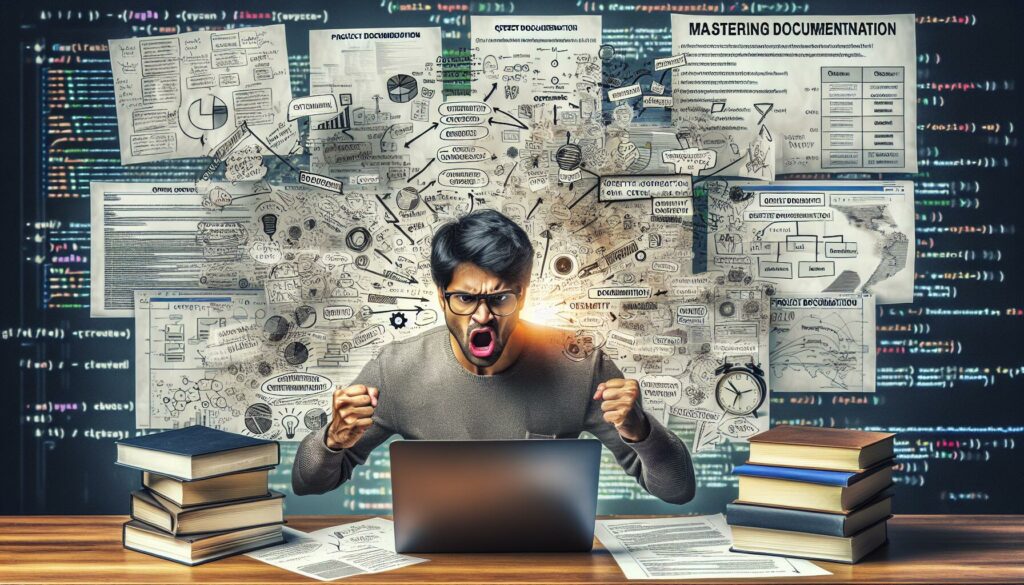
In the world of software development, writing code is only half the battle. The other crucial aspect that often gets overlooked is documentation. Whether you’re a seasoned developer or just starting your coding journey, mastering the art of documentation can significantly improve your projects and enhance your overall programming skills. In this comprehensive guide, we’ll explore why creating clear documentation is essential for both personal understanding and future collaboration, and provide you with practical examples of effective documentation practices.
The Importance of Documentation in Software Development
Documentation serves as a roadmap for your code, providing insights into its structure, functionality, and purpose. It’s not just about leaving notes for others; it’s about creating a clear, concise, and informative resource that benefits both you and your collaborators. Here’s why documentation is crucial:
- Enhances Code Understanding: Well-written documentation helps developers quickly grasp the purpose and functionality of code segments, reducing the time needed to understand and work with the codebase.
- Facilitates Collaboration: In team environments, good documentation enables smoother collaboration by providing a shared understanding of the project’s architecture and individual components.
- Improves Maintainability: As projects grow and evolve, documentation becomes invaluable for maintaining and updating code, especially when returning to a project after a long period.
- Aids in Onboarding: New team members can get up to speed more quickly with well-documented projects, reducing the learning curve and increasing productivity.
- Serves as a Learning Tool: Writing documentation forces you to think critically about your code, often leading to improvements in your coding practices and problem-solving skills.
Types of Documentation
Before diving into best practices, let’s review the main types of documentation you’ll encounter in software development:
1. README Files
README files are often the first point of contact for anyone interacting with your project. They provide an overview of the project, installation instructions, and basic usage guidelines. A well-crafted README can make your project more accessible and increase its adoption.
2. Code Comments
Code comments are in-line explanations within your source code. They clarify complex logic, explain the reasoning behind certain decisions, and provide context for future developers (including yourself) who may need to modify the code.
3. Inline Documentation
Inline documentation, such as docstrings in Python or JSDoc in JavaScript, provides detailed information about functions, classes, and modules directly in the code. This type of documentation is particularly useful for generating API references and providing context-sensitive help in IDEs.
4. External Documentation
External documentation includes user manuals, API references, and technical specifications. These documents are typically more comprehensive and are often hosted separately from the codebase.
Best Practices for Effective Documentation
Now that we understand the importance and types of documentation, let’s explore some best practices for creating effective documentation:
1. Keep It Clear and Concise
Good documentation should be easy to read and understand. Use clear language and avoid unnecessary jargon. Be concise, but don’t sacrifice clarity for brevity. Here’s an example of a clear and concise function documentation:
/**
* Calculates the average of an array of numbers.
* @param {number[]} numbers - An array of numbers.
* @returns {number} The average of the input numbers.
* @throws {Error} If the input array is empty.
*/
function calculateAverage(numbers) {
if (numbers.length === 0) {
throw new Error("Cannot calculate average of an empty array");
}
const sum = numbers.reduce((a, b) => a + b, 0);
return sum / numbers.length;
}
2. Use Consistent Formatting
Maintain a consistent style throughout your documentation. This includes using the same formatting for headings, code blocks, and examples. Consistency makes your documentation easier to navigate and understand. For example, in a README file:
# Project Title
## Installation
1. Clone the repository:
```
git clone https://github.com/username/project.git
```
2. Install dependencies:
```
npm install
```
## Usage
To run the application:
```
npm start
```
## Contributing
Please read [CONTRIBUTING.md](CONTRIBUTING.md) for details on our code of conduct and the process for submitting pull requests.
3. Keep Documentation Up-to-Date
Outdated documentation can be more harmful than no documentation at all. Make it a habit to update your documentation whenever you make changes to your code. Consider setting up automated tools to flag outdated documentation or include documentation updates in your code review process.
4. Use Examples and Code Snippets
Incorporate relevant examples and code snippets to illustrate key concepts or usage patterns. This helps readers understand how to implement or use specific features. For instance, in API documentation:
## User Authentication
To authenticate a user, make a POST request to the `/auth/login` endpoint:
```javascript
fetch("https://api.example.com/auth/login", {
method: "POST",
headers: {
"Content-Type": "application/json",
},
body: JSON.stringify({
username: "johndoe",
password: "secretpassword",
}),
})
.then((response) => response.json())
.then((data) => console.log(data))
.catch((error) => console.error("Error:", error));
```
This will return a JSON object containing the authentication token:
```json
{
"token": "eyJhbGciOiJIUzI1NiIsInR5cCI6IkpXVCJ9..."
}
```
5. Document Assumptions and Limitations
Clearly state any assumptions your code makes or limitations it has. This helps prevent misuse and provides valuable context for users and contributors. For example:
/**
* Sorts an array of integers using the quicksort algorithm.
* @param {number[]} arr - The array to be sorted.
* @returns {number[]} The sorted array.
* @note This implementation assumes all elements in the array are integers.
* @limitation The algorithm's performance may degrade for arrays with many duplicate elements.
*/
function quickSort(arr) {
// Implementation details...
}
6. Use Diagrams and Visual Aids
When appropriate, include diagrams, flowcharts, or other visual aids to explain complex processes or architectures. Tools like PlantUML or Mermaid can be used to create diagrams directly in markdown files. For example:
```mermaid
sequenceDiagram
participant User
participant Client
participant Server
participant Database
User->>Client: Enter credentials
Client->>Server: Send login request
Server->>Database: Verify credentials
Database-->>Server: Return user data
Server-->>Client: Send authentication token
Client-->>User: Display success message
```
7. Provide Context and Rationale
Explain not just what the code does, but why it does it. This context can be invaluable when revisiting code or when others are trying to understand your design decisions. For instance:
// We use a cache here to improve performance for frequently accessed items.
// The cache is limited to 1000 entries to prevent excessive memory usage.
const cache = new LRUCache(1000);
function getData(key) {
if (cache.has(key)) {
return cache.get(key);
}
// Fetch data from the database if not in cache
const data = fetchFromDatabase(key);
cache.set(key, data);
return data;
}
How Documentation Improves Your Coding Skills
Writing good documentation isn’t just about helping others understand your code; it’s also a powerful tool for improving your own coding skills and project quality. Here’s how:
1. Encourages Clear Thinking
When you document your code, you’re forced to articulate your thoughts and reasoning. This process often reveals flaws in logic or design that you might have overlooked while coding. By explaining your code in writing, you’re essentially rubber-ducking your own work, which can lead to improvements and optimizations.
2. Improves Code Structure
As you document your code, you may realize that certain parts are overly complex or poorly organized. This realization can prompt you to refactor your code, leading to cleaner, more maintainable solutions. For example, you might break down a large function into smaller, well-documented components:
// Before:
function processUserData(userData) {
// 100 lines of complex logic
}
// After:
/**
* Processes user data by validating, transforming, and storing it.
* @param {Object} userData - The user data to process.
* @returns {Object} The processed user data.
*/
function processUserData(userData) {
const validatedData = validateUserData(userData);
const transformedData = transformUserData(validatedData);
return storeUserData(transformedData);
}
/**
* Validates user data against schema.
* @param {Object} userData - The user data to validate.
* @returns {Object} The validated user data.
* @throws {ValidationError} If the data is invalid.
*/
function validateUserData(userData) {
// Validation logic
}
/**
* Transforms user data into the required format.
* @param {Object} validatedData - The validated user data.
* @returns {Object} The transformed user data.
*/
function transformUserData(validatedData) {
// Transformation logic
}
/**
* Stores user data in the database.
* @param {Object} transformedData - The transformed user data.
* @returns {Object} The stored user data with added metadata.
*/
function storeUserData(transformedData) {
// Storage logic
}
3. Enhances Problem-Solving Skills
Documenting your problem-solving process helps you develop a more structured approach to tackling coding challenges. By articulating the steps you took to solve a problem, you create a reusable template for future problem-solving. This is particularly useful when preparing for technical interviews or working on complex algorithms.
4. Builds a Personal Knowledge Base
Well-documented projects serve as a personal knowledge base. When you encounter similar problems in the future, you can refer back to your own documentation for solutions and insights. This accelerates your learning and problem-solving capabilities over time.
5. Improves Communication Skills
Writing clear and effective documentation hones your ability to communicate technical concepts. This skill is invaluable in professional settings, whether you’re explaining your work to non-technical stakeholders, collaborating with team members, or contributing to open-source projects.
Documentation in the Context of Coding Education
In the realm of coding education, particularly in platforms like AlgoCademy that focus on interactive coding tutorials and preparing for technical interviews, documentation plays a crucial role:
1. Reinforces Learning
When learning new concepts or algorithms, documenting your understanding helps reinforce the material. For example, after completing a tutorial on binary search trees, you might create a markdown file summarizing the key points:
# Binary Search Trees (BST)
## Definition
A binary search tree is a binary tree where for each node:
- All elements in the left subtree are less than the node's value
- All elements in the right subtree are greater than the node's value
## Key Operations
1. Insertion: O(log n) average, O(n) worst case
2. Deletion: O(log n) average, O(n) worst case
3. Search: O(log n) average, O(n) worst case
## Implementation
```python
class Node:
def __init__(self, value):
self.value = value
self.left = None
self.right = None
class BinarySearchTree:
def __init__(self):
self.root = None
def insert(self, value):
# Implementation details...
def search(self, value):
# Implementation details...
def delete(self, value):
# Implementation details...
```
## Common Interview Questions
1. Implement BST insertion
2. Find the lowest common ancestor of two nodes in a BST
3. Validate if a binary tree is a valid BST
## Resources
- [AlgoCademy BST Tutorial](https://algocademy.com/tutorials/bst)
- [Visualizing BST Operations](https://visualgo.net/en/bst)
2. Prepares for Technical Interviews
Many technical interviews involve explaining your thought process and coding approach. By practicing documentation as you solve problems, you’re better prepared to articulate your solutions during interviews. For instance, when solving a LeetCode problem, you might document your approach:
## Problem: Two Sum
### Problem Statement
Given an array of integers `nums` and an integer `target`, return indices of the two numbers such that they add up to `target`.
### Approach
1. Use a hash map to store complement values
2. Iterate through the array once
3. For each number, check if its complement exists in the hash map
4. If found, return the current index and the complement's index
5. If not found, add the current number and its index to the hash map
### Time Complexity: O(n)
### Space Complexity: O(n)
### Implementation
```python
class Solution:
def twoSum(self, nums: List[int], target: int) -> List[int]:
complement_map = {}
for i, num in enumerate(nums):
complement = target - num
if complement in complement_map:
return [complement_map[complement], i]
complement_map[num] = i
return [] # No solution found
```
### Test Cases
1. Input: nums = [2,7,11,15], target = 9
Output: [0,1]
2. Input: nums = [3,2,4], target = 6
Output: [1,2]
3. Input: nums = [3,3], target = 6
Output: [0,1]
### Follow-up Questions
1. How would you handle duplicate numbers in the array?
2. Can you solve this problem with constant space complexity?
3. Builds a Problem-Solving Portfolio
As you work through coding challenges and tutorials, maintaining well-documented solutions creates a valuable portfolio. This portfolio not only showcases your problem-solving skills to potential employers but also serves as a personal reference for future learning and interview preparation.
Integrating Documentation into Your Coding Workflow
To truly master documentation and reap its benefits, it’s essential to integrate it into your regular coding workflow. Here are some strategies to make documentation a natural part of your development process:
1. Start with a Documentation Template
Create templates for different types of documentation (e.g., README files, function documentation, problem-solving notes) and use them consistently across your projects. This reduces the friction of getting started and ensures you cover all necessary aspects. For example, a README template might look like this:
# Project Name
Brief description of the project.
## Installation
Steps to install the project.
## Usage
Instructions on how to use the project.
## Features
List of key features.
## Contributing
Guidelines for contributing to the project.
## License
Project license information.
## Contact
How to reach the project maintainers.
2. Use Documentation-First Development
Before writing code, start by documenting what you intend to build. This approach, similar to test-driven development, helps clarify your thoughts and design before implementation. For example, when creating a new function:
/**
* Converts a temperature from Celsius to Fahrenheit.
* @param {number} celsius - The temperature in Celsius.
* @returns {number} The temperature in Fahrenheit.
* @throws {TypeError} If the input is not a number.
*/
function celsiusToFahrenheit(celsius) {
// Implementation will go here
}
3. Set Up Automated Documentation Tools
Utilize tools that can generate documentation from your code comments, such as JSDoc for JavaScript or Sphinx for Python. This encourages you to write good inline documentation and keeps your external documentation up-to-date. For example, using JSDoc:
/**
* Represents a book.
* @class
*/
class Book {
/**
* Create a book.
* @param {string} title - The title of the book.
* @param {string} author - The author of the book.
* @param {number} year - The publication year of the book.
*/
constructor(title, author, year) {
this.title = title;
this.author = author;
this.year = year;
}
/**
* Get the age of the book.
* @returns {number} The age of the book in years.
*/
getAge() {
return new Date().getFullYear() - this.year;
}
}
// Generate documentation using JSDoc:
// jsdoc book.js
4. Include Documentation in Code Reviews
If you’re working in a team, make documentation a part of your code review process. This ensures that documentation is treated with the same importance as the code itself and helps maintain high-quality documentation across the project.
5. Practice Explaining Your Code
Regularly practice explaining your code, either through written documentation or verbal explanations. This could involve participating in code review sessions, contributing to open-source projects, or teaching coding concepts to others. The more you practice, the better you’ll become at articulating complex ideas clearly and concisely.
Conclusion
Mastering documentation is an essential skill for any programmer, whether you’re a beginner learning the ropes or an experienced developer preparing for technical interviews. By creating clear, comprehensive documentation, you not only make your projects more accessible and maintainable but also enhance your own understanding and problem-solving abilities.
Remember that good documentation is an ongoing process. It evolves with your code and your understanding of the problem domain. By integrating documentation into your regular coding workflow and treating it as an integral part of the development process, you’ll build better projects, improve your coding skills, and set yourself up for success in your programming journey.
As you continue to learn and grow as a developer, platforms like AlgoCademy can provide valuable resources and interactive tutorials to help you hone your skills. By applying the documentation practices discussed in this article to your learning process, you’ll be better prepared to tackle complex coding challenges, ace technical interviews, and contribute effectively to real-world software projects.
So, the next time you sit down to code, remember: writing good documentation isn’t just about leaving notes for others—it’s about building a deeper understanding, creating more robust solutions, and becoming a more proficient and articulate programmer. Happy coding and documenting!