Breaking Down a Big Project: How to Approach Complex Projects One Step at a Time
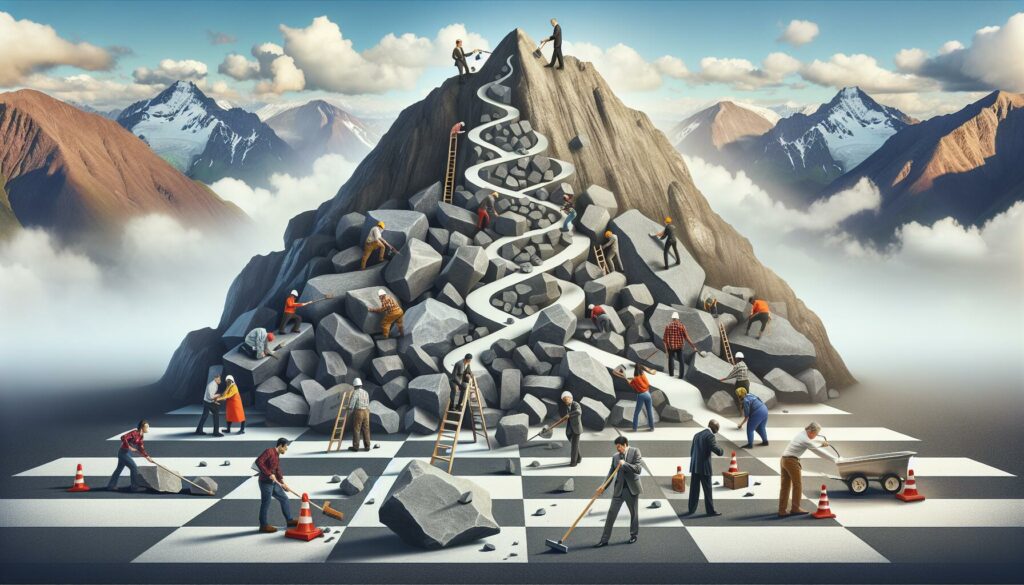
Embarking on a large coding project can be daunting, especially for those new to programming or tackling complex problems. However, with the right approach and mindset, even the most intimidating projects can be conquered. In this comprehensive guide, we’ll explore how to break down a big project into smaller, manageable milestones and tasks, using a real-world example to illustrate the process. By the end of this article, you’ll have a solid framework for tackling any large-scale coding project with confidence.
The Importance of Breaking Down Large Projects
Before we dive into the specifics, let’s understand why breaking down large projects is crucial:
- Reduced Overwhelm: Facing a massive project can be paralyzing. Smaller tasks are less intimidating and easier to start.
- Better Planning: Dividing the project helps in creating a more accurate timeline and resource allocation.
- Improved Focus: Working on smaller components allows for better concentration and productivity.
- Easier Progress Tracking: Completing smaller tasks provides a sense of accomplishment and helps monitor overall progress.
- Flexibility: Smaller components make it easier to adapt to changes or pivot if necessary.
Example Project: Building an Online Coding Education Platform
To illustrate the process of breaking down a large project, let’s use an example that aligns with AlgoCademy’s mission: creating an online coding education platform. This platform will offer interactive coding tutorials, resources for learners, and tools to help individuals progress from beginner-level coding to preparing for technical interviews.
Step 1: Define the Project Scope and Objectives
Before breaking down the project, it’s essential to have a clear understanding of what you want to achieve. For our coding education platform, let’s define the main objectives:
- Provide interactive coding tutorials for multiple programming languages
- Offer a progression path from beginner to advanced coding skills
- Include features for technical interview preparation
- Implement AI-powered assistance for personalized learning
- Create a user-friendly interface for seamless navigation and learning
Step 2: Identify Major Components
Now that we have our objectives, let’s break down the project into major components:
- User Management System
- Content Management System (CMS)
- Interactive Coding Environment
- Learning Path and Progress Tracking
- AI-powered Assistant
- Technical Interview Preparation Module
- User Interface and Experience (UI/UX)
- Backend Infrastructure
Step 3: Break Down Components into Smaller Tasks
Let’s take each component and break it down further into smaller, manageable tasks. We’ll use the User Management System as an example:
User Management System
- User Registration
- Design registration form
- Implement email verification
- Create database schema for user information
- Develop API endpoints for registration
- User Authentication
- Implement login functionality
- Develop password hashing and security measures
- Create JWT (JSON Web Token) for session management
- Implement OAuth for social media login options
- User Profile Management
- Design user profile page
- Implement profile editing functionality
- Add profile picture upload feature
- Create API endpoints for profile management
- User Roles and Permissions
- Define user roles (e.g., student, instructor, admin)
- Implement role-based access control (RBAC)
- Create an admin panel for user management
Repeat this process for each major component, breaking them down into smaller, actionable tasks.
Step 4: Prioritize and Sequence Tasks
Once you have a comprehensive list of tasks, it’s time to prioritize and sequence them. Consider the following factors:
- Dependencies: Identify tasks that rely on others being completed first.
- Critical Path: Determine which tasks are essential for the project’s core functionality.
- Resource Availability: Consider the skills and availability of your team members.
- Time Constraints: Factor in any deadlines or time-sensitive tasks.
For our coding education platform, a possible sequence might be:
- Set up backend infrastructure
- Implement basic user management system
- Develop content management system
- Create interactive coding environment
- Implement learning path and progress tracking
- Develop AI-powered assistant
- Create technical interview preparation module
- Design and implement user interface
- Conduct thorough testing and refinement
Step 5: Create a Detailed Roadmap
With your tasks prioritized and sequenced, it’s time to create a detailed roadmap. This roadmap will serve as your guide throughout the project, helping you stay on track and measure progress. Here’s how to create an effective roadmap:
1. Define Milestones
Milestones are significant checkpoints in your project. They represent major accomplishments or the completion of key components. For our coding education platform, milestones might include:
- MVP (Minimum Viable Product) Launch
- Beta Release
- Full Feature Release
- Scale and Optimization Phase
2. Set Timeframes
Assign realistic timeframes to each task and milestone. Be sure to account for potential setbacks and allow some buffer time. Here’s an example of how you might structure your timeframes:
Milestone 1: MVP Launch (3 months)
- Week 1-2: Set up backend infrastructure
- Week 3-4: Implement basic user management system
- Week 5-6: Develop content management system
- Week 7-8: Create simple interactive coding environment
- Week 9-10: Implement basic learning path
- Week 11-12: Design and implement basic UI
Milestone 2: Beta Release (3 months)
- Week 13-14: Enhance interactive coding environment
- Week 15-16: Develop AI-powered assistant (basic functionality)
- Week 17-18: Create technical interview preparation module
- Week 19-20: Improve UI/UX based on user feedback
- Week 21-22: Implement advanced user management features
- Week 23-24: Conduct thorough testing and bug fixes
Milestone 3: Full Feature Release (3 months)
- Week 25-26: Enhance AI-powered assistant
- Week 27-28: Expand content library
- Week 29-30: Implement advanced learning path features
- Week 31-32: Enhance technical interview preparation module
- Week 33-34: Optimize performance and scalability
- Week 35-36: Final testing, refinement, and preparation for launch
Milestone 4: Scale and Optimization Phase (Ongoing)
- Continuous improvement based on user feedback
- Regular content updates and expansion
- Performance optimization and scaling
- Implementation of new features and technologies
3. Assign Responsibilities
If you’re working with a team, clearly assign responsibilities for each task. This ensures accountability and helps team members understand their roles in the project. For example:
- Backend Development: John Doe
- Frontend Development: Jane Smith
- UI/UX Design: Alex Johnson
- Content Creation: Sarah Williams
- AI Development: Michael Brown
- Project Management: Emily Davis
4. Use Project Management Tools
Utilize project management tools to visualize and track your roadmap. Some popular options include:
- Trello: Great for Kanban-style project management
- Jira: Robust tool for agile project management
- Asana: Flexible tool for task management and collaboration
- GitHub Projects: Ideal for software development projects
These tools can help you create visual boards, track progress, and collaborate with team members effectively.
Step 6: Implement Agile Methodologies
For complex coding projects, consider implementing Agile methodologies to enhance flexibility and adaptability. Agile approaches, such as Scrum or Kanban, can help you manage your project more effectively:
Scrum Framework
If you choose to use Scrum, here’s how you might structure your project:
- Define Sprint Length: Typically 1-4 weeks
- Create a Product Backlog: Prioritize all tasks and features
- Plan Sprints: Select tasks from the backlog for each sprint
- Conduct Daily Stand-ups: Brief team meetings to discuss progress and obstacles
- Hold Sprint Reviews and Retrospectives: Evaluate completed work and improve processes
Here’s an example of how you might structure a sprint for the coding education platform:
Sprint 1 (2 weeks): Basic User Management System
- Design and implement user registration form
- Develop API endpoints for registration
- Implement email verification
- Create database schema for user information
- Develop basic login functionality
- Implement password hashing and security measures
Daily Stand-up Questions:
1. What did you accomplish yesterday?
2. What are you working on today?
3. Are there any obstacles in your way?
Sprint Review:
- Demo completed features
- Gather feedback from stakeholders
Sprint Retrospective:
- What went well?
- What could be improved?
- Action items for the next sprint
Step 7: Regular Progress Tracking and Adjustment
As you work through your project, it’s crucial to regularly track progress and make adjustments as needed. Here are some strategies to keep your project on track:
1. Use Burndown Charts
Burndown charts visually represent the amount of work left to do versus time. They help you see if you’re on track to meet your sprint or project goals.
2. Conduct Regular Check-ins
Schedule weekly or bi-weekly check-ins with your team to discuss progress, challenges, and any necessary adjustments to the roadmap.
3. Be Flexible
Be prepared to adjust your roadmap as you gain new insights or encounter unexpected challenges. Flexibility is key to successful project management.
4. Celebrate Small Wins
Acknowledge and celebrate the completion of tasks and milestones. This helps maintain team morale and motivation throughout the project.
Step 8: Documentation and Knowledge Sharing
As you progress through your project, maintain comprehensive documentation. This is crucial for several reasons:
- Onboarding new team members
- Troubleshooting issues
- Maintaining the project long-term
- Sharing knowledge across the team
Consider implementing the following documentation practices:
- Code Comments: Write clear, concise comments explaining complex logic or algorithms.
- README Files: Create detailed README files for each component or module.
- API Documentation: Use tools like Swagger or Postman to document your APIs.
- Architecture Diagrams: Visualize your system’s architecture using tools like Draw.io or Lucidchart.
- Knowledge Base: Set up a centralized knowledge base (e.g., using Confluence or GitBook) to store project-related information.
Conclusion: Embracing the Journey of Large-Scale Projects
Breaking down a big project into smaller, manageable tasks is an essential skill for any programmer or project manager. By following the steps outlined in this guide, you can approach complex projects with confidence and clarity.
Remember, the key to success lies in thorough planning, effective communication, and the ability to adapt as you progress. Embrace the iterative nature of software development, and don’t be afraid to adjust your approach as you learn and grow throughout the project.
As you apply these techniques to your own projects, you’ll find that even the most daunting tasks become achievable when broken down into smaller, manageable steps. Whether you’re building a coding education platform like our example or tackling any other large-scale project, these principles will guide you towards success.
Happy coding, and may your projects be ever manageable and successful!