How to Start Coding a Project from Scratch: The Ultimate Beginner’s Guide
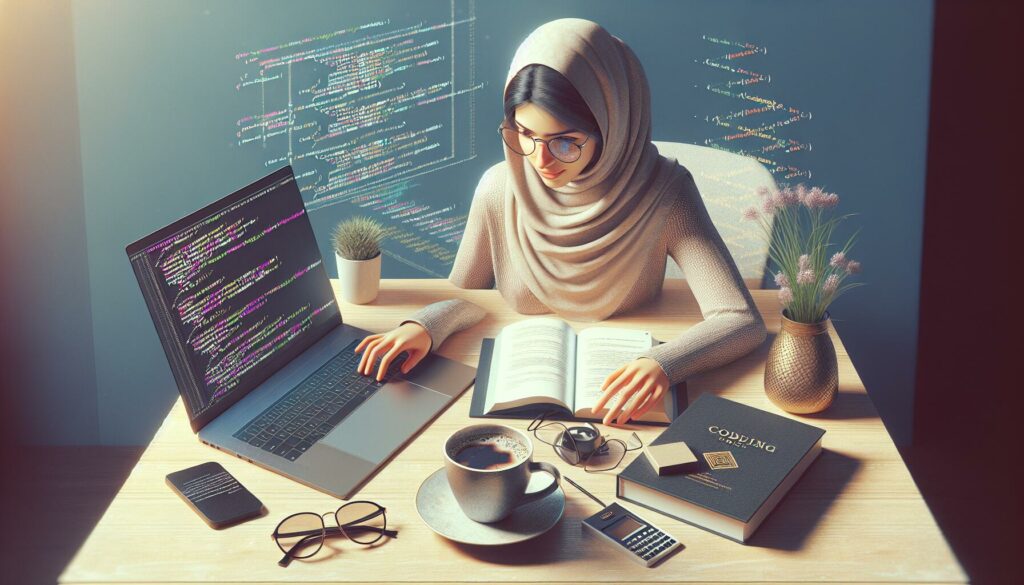
Embarking on your first coding project can be both exciting and daunting. Whether you’re a complete novice or have some basic programming knowledge, starting a project from scratch requires careful planning, dedication, and a systematic approach. This comprehensive guide will walk you through the entire process, from conceptualizing your idea to implementing and refining your code. By following these steps, you’ll be well-equipped to tackle your first coding project with confidence.
1. Conceptualize Your Project
Before you write a single line of code, it’s crucial to have a clear idea of what you want to create. This initial phase involves brainstorming, research, and defining the scope of your project.
1.1. Identify a Problem or Need
The best projects often arise from addressing a specific problem or fulfilling a particular need. Ask yourself:
- What challenges do you or others face that could be solved with software?
- Are there any existing tools or applications that you wish worked differently?
- Is there a topic or hobby you’re passionate about that could benefit from a new application?
1.2. Research Existing Solutions
Once you have an idea, investigate whether similar solutions already exist. This step will help you:
- Understand the current landscape of your chosen problem space
- Identify potential features for your project
- Find ways to differentiate your project or improve upon existing solutions
1.3. Define Your Project Scope
With a clear idea and some research under your belt, it’s time to define the scope of your project. This involves:
- Listing the core features your project must have
- Identifying “nice-to-have” features that can be added later
- Setting realistic goals for what you can achieve given your current skill level and available time
Remember, it’s often better to start small and expand later rather than taking on too much at once.
2. Plan Your Project
With a clear concept in mind, the next step is to create a detailed plan for your project. This phase will help you organize your thoughts and create a roadmap for development.
2.1. Choose Your Technology Stack
Selecting the right tools and technologies for your project is crucial. Consider:
- Programming language: Choose a language that’s suitable for your project type and your skill level. For beginners, languages like Python or JavaScript are often good choices due to their readability and extensive learning resources.
- Frameworks and libraries: Research which frameworks or libraries can help you achieve your goals more efficiently.
- Development environment: Select an Integrated Development Environment (IDE) or text editor that supports your chosen language and offers helpful features for coding.
2.2. Break Down Your Project into Smaller Tasks
Divide your project into smaller, manageable tasks. This approach, often called “chunking,” makes the overall project less overwhelming and helps you track progress more easily. For each task:
- Define what needs to be accomplished
- Estimate how long it might take
- Identify any dependencies on other tasks
2.3. Create a Project Timeline
Based on your task breakdown, create a rough timeline for your project. This doesn’t need to be set in stone, but it should give you a general idea of how long the project might take and help you stay on track.
2.4. Set Up Version Control
Even for a beginner project, it’s a good practice to use version control. Git is the most popular version control system, and platforms like GitHub or GitLab provide free repositories for your code. Setting up version control will help you:
- Track changes to your code over time
- Experiment with new features without fear of breaking your main codebase
- Collaborate with others if you decide to expand your project in the future
3. Design Your Solution
Before diving into coding, it’s beneficial to design your solution at a high level. This step helps you think through the logic of your program and can save you time during the coding phase.
3.1. Create a Flowchart
A flowchart is a visual representation of your program’s logic. It can help you understand the flow of data and decision-making processes in your application. To create a flowchart:
- Start with the main functionalities of your application
- Use shapes to represent different types of actions (e.g., rectangles for processes, diamonds for decisions)
- Connect the shapes with arrows to show the flow of the program
3.2. Write Pseudocode
Pseudocode is a way of describing your program’s logic in plain language, without adhering to the syntax of any particular programming language. Writing pseudocode can help you:
- Organize your thoughts about how the program should work
- Identify potential issues or edge cases before you start coding
- Make the transition to actual code easier
Here’s a simple example of pseudocode for a basic calculator function:
FUNCTION add_numbers(num1, num2):
result = num1 + num2
RETURN result
FUNCTION subtract_numbers(num1, num2):
result = num1 - num2
RETURN result
WHILE true:
PRINT "Enter an operation (+, -, or q to quit): "
operation = GET_USER_INPUT()
IF operation is "q":
BREAK
PRINT "Enter first number: "
num1 = GET_USER_INPUT()
PRINT "Enter second number: "
num2 = GET_USER_INPUT()
IF operation is "+":
result = add_numbers(num1, num2)
ELSE IF operation is "-":
result = subtract_numbers(num1, num2)
ELSE:
PRINT "Invalid operation"
CONTINUE
PRINT "Result: " + result
PRINT "Calculator closed."
3.3. Plan Your Data Structures
Consider what kind of data your program will be working with and how it should be organized. Will you need lists, dictionaries, custom objects, or database tables? Planning your data structures in advance can help you write more efficient and organized code.
4. Set Up Your Development Environment
Before you start coding, you need to set up your development environment. This involves installing the necessary software and configuring your workspace.
4.1. Install Your Chosen Programming Language
Download and install the latest stable version of your chosen programming language. Most languages have straightforward installation processes:
- For Python: Visit python.org and download the installer for your operating system.
- For JavaScript: You might not need to install anything if you’re focusing on front-end development, as browsers can run JavaScript. For back-end development, install Node.js from nodejs.org.
4.2. Choose and Install an IDE or Text Editor
An Integrated Development Environment (IDE) or a good text editor can significantly improve your coding experience. Some popular options include:
- Visual Studio Code: A versatile, free editor that supports multiple languages and has a wide range of extensions.
- PyCharm: An IDE specifically designed for Python development.
- Sublime Text: A lightweight, customizable text editor popular among developers.
4.3. Set Up Version Control
If you haven’t already, install Git on your computer and create an account on a platform like GitHub or GitLab. Then, create a new repository for your project and clone it to your local machine.
4.4. Install Necessary Libraries or Frameworks
If your project requires any specific libraries or frameworks, install them now. For Python, you can use pip, the package installer. For JavaScript with Node.js, you can use npm (Node Package Manager).
5. Start Coding: Creating a Basic Prototype
With your environment set up and your plan in place, it’s time to start coding! Begin by creating a basic prototype that implements the core functionality of your project.
5.1. Set Up Your Project Structure
Create a logical folder structure for your project. A basic structure might look like this:
project_name/
│
├── src/
│ ├── main.py (or index.js, etc.)
│ └── other_modules/
│
├── tests/
│
├── docs/
│
└── README.md
5.2. Implement Core Functionality
Start by implementing the most basic version of your project that still accomplishes its main goal. This might involve:
- Creating the main entry point for your program
- Implementing key functions or classes
- Setting up a basic user interface (if applicable)
Here’s a simple example of a basic calculator in Python:
def add(x, y):
return x + y
def subtract(x, y):
return x - y
def multiply(x, y):
return x * y
def divide(x, y):
if y == 0:
return "Error: Division by zero"
return x / y
while True:
print("Select operation:")
print("1. Add")
print("2. Subtract")
print("3. Multiply")
print("4. Divide")
print("5. Exit")
choice = input("Enter choice (1/2/3/4/5): ")
if choice == '5':
print("Exiting the calculator. Goodbye!")
break
if choice in ('1', '2', '3', '4'):
num1 = float(input("Enter first number: "))
num2 = float(input("Enter second number: "))
if choice == '1':
print("Result:", add(num1, num2))
elif choice == '2':
print("Result:", subtract(num1, num2))
elif choice == '3':
print("Result:", multiply(num1, num2))
elif choice == '4':
print("Result:", divide(num1, num2))
else:
print("Invalid input")
5.3. Test Your Prototype
As you build your prototype, regularly test your code to ensure it’s working as expected. This can involve:
- Manual testing: Running your program and interacting with it to check its behavior
- Writing simple test cases: Creating basic scenarios to verify your functions are working correctly
- Using print statements or a debugger to track the flow of your program and identify issues
5.4. Commit Your Changes
As you make progress, regularly commit your changes to your version control system. Good commit messages describe what changes were made and why. For example:
git add .
git commit -m "Implement basic calculator functions and user interface"
git push origin main
6. Iterate and Improve
Once you have a working prototype, it’s time to refine and expand your project. This phase is where you’ll add more features, improve the user experience, and polish your code.
6.1. Implement Additional Features
Refer back to your project plan and start implementing the features you identified earlier. As you add each feature:
- Break it down into smaller tasks if necessary
- Implement the feature
- Test the new functionality thoroughly
- Commit your changes with descriptive commit messages
6.2. Refactor Your Code
As your project grows, you may find opportunities to improve your code structure or efficiency. Refactoring involves restructuring existing code without changing its external behavior. Some refactoring techniques include:
- Extracting repeated code into functions
- Improving naming conventions for better readability
- Optimizing algorithms for better performance
6.3. Handle Edge Cases and Errors
Think about potential edge cases or errors that could occur in your program. Implement error handling to make your code more robust. This might involve:
- Adding try/except blocks to catch and handle exceptions
- Validating user input to prevent unexpected behavior
- Providing meaningful error messages to guide users
6.4. Improve User Experience
If your project has a user interface, consider ways to enhance the user experience:
- Improve the layout and design of your interface
- Add helpful prompts or instructions for users
- Implement keyboard shortcuts or other convenience features
6.5. Optimize Performance
As you add more features, keep an eye on your program’s performance. Look for opportunities to optimize:
- Use appropriate data structures for efficient data manipulation
- Minimize redundant calculations or operations
- Consider using caching mechanisms for frequently accessed data
7. Document Your Project
Documentation is a crucial part of any coding project, even for beginners. Good documentation helps others understand your code and can even help you remember how your project works when you return to it later.
7.1. Write a README File
Create a README.md file in the root of your project directory. This file should include:
- A brief description of your project
- Instructions for setting up and running the project
- Any dependencies or prerequisites
- Basic usage instructions
- Information about how to contribute (if you want to open your project to contributions)
7.2. Add Comments to Your Code
Include comments in your code to explain complex logic or provide context for certain decisions. However, aim for a balance – good code should be largely self-explanatory, with comments providing additional clarity where needed.
7.3. Generate API Documentation
If your project includes functions or classes that others might use, consider generating API documentation. Many languages have tools for this, such as:
- Python: Sphinx or pdoc
- JavaScript: JSDoc
8. Test Thoroughly
Before considering your project complete, it’s important to test it thoroughly to ensure it works as expected and is free of major bugs.
8.1. Write Unit Tests
Unit tests are small, focused tests that check individual components of your code. Most programming languages have built-in or widely-used testing frameworks:
- Python: unittest or pytest
- JavaScript: Jest or Mocha
Here’s a simple example of a unit test in Python using the unittest framework:
import unittest
from calculator import add, subtract, multiply, divide
class TestCalculator(unittest.TestCase):
def test_add(self):
self.assertEqual(add(2, 3), 5)
self.assertEqual(add(-1, 1), 0)
self.assertEqual(add(-1, -1), -2)
def test_subtract(self):
self.assertEqual(subtract(5, 3), 2)
self.assertEqual(subtract(1, 2), -1)
self.assertEqual(subtract(-1, -1), 0)
def test_multiply(self):
self.assertEqual(multiply(2, 3), 6)
self.assertEqual(multiply(-1, 3), -3)
self.assertEqual(multiply(-2, -2), 4)
def test_divide(self):
self.assertEqual(divide(6, 3), 2)
self.assertEqual(divide(-6, 3), -2)
self.assertEqual(divide(5, 2), 2.5)
self.assertEqual(divide(1, 0), "Error: Division by zero")
if __name__ == '__main__':
unittest.main()
8.2. Perform Integration Testing
If your project consists of multiple components or modules, perform integration tests to ensure they work together correctly.
8.3. Conduct User Testing
If possible, have others use your project and provide feedback. This can help you identify usability issues or bugs that you might have overlooked.
9. Deploy Your Project
Once your project is complete and thoroughly tested, you might want to deploy it so others can use it.
9.1. Choose a Hosting Platform
Depending on your project type, you might consider platforms like:
- GitHub Pages for static websites
- Heroku or PythonAnywhere for web applications
- Google Play Store or Apple App Store for mobile apps
9.2. Prepare Your Project for Deployment
This might involve steps like:
- Configuring environment variables
- Setting up a production database
- Optimizing assets for production use
9.3. Deploy and Monitor
Follow the deployment process for your chosen platform, then monitor your application to ensure it’s running smoothly in the production environment.
10. Reflect and Plan Next Steps
Congratulations on completing your first coding project! Take some time to reflect on the process:
- What did you learn?
- What challenges did you face, and how did you overcome them?
- What would you do differently next time?
Consider sharing your project with others, either by open-sourcing it on GitHub or showcasing it in your portfolio. Finally, start thinking about your next project – what new skills or technologies would you like to explore?
Conclusion
Starting a coding project from scratch can seem daunting, but by breaking it down into manageable steps, you can successfully bring your ideas to life. Remember, the key to becoming a proficient programmer is practice and persistence. Each project you complete will build your skills and confidence, preparing you for more complex challenges in the future.
As you continue your coding journey, consider exploring more advanced topics like data structures, algorithms, and software design patterns. Platforms like AlgoCademy offer interactive tutorials and resources to help you progress from beginner-level coding to preparing for technical interviews at major tech companies.
Don’t be discouraged if you encounter difficulties along the way – problem-solving is a crucial part of programming, and each obstacle you overcome is an opportunity to learn and grow. Happy coding!