Algorithms for Real-Time Translation: Bridging Language Barriers Instantly
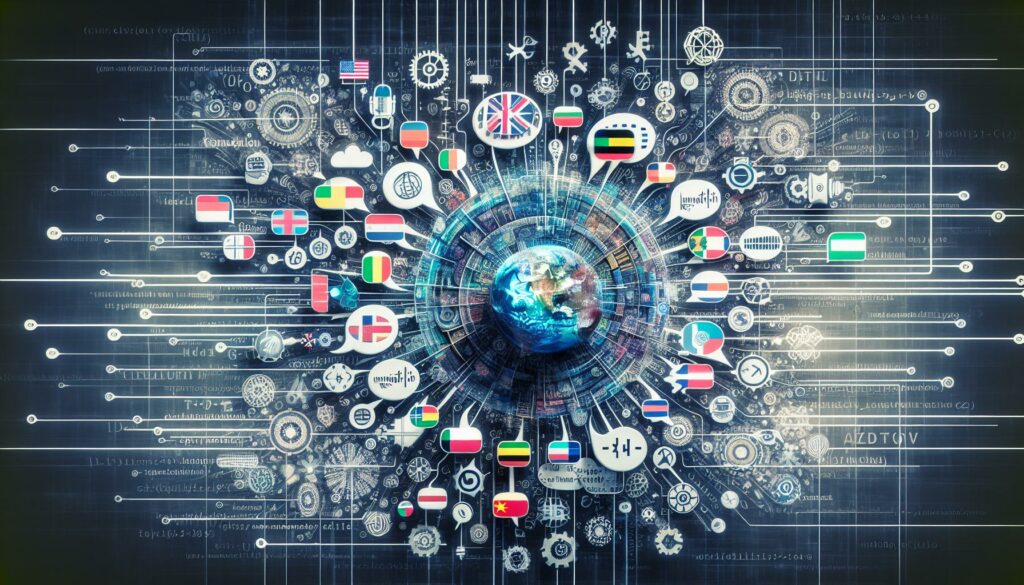
In today’s interconnected world, the ability to communicate across language barriers is more important than ever. Real-time translation algorithms have emerged as a powerful tool to facilitate instant communication between people speaking different languages. These algorithms leverage advanced natural language processing (NLP) techniques, machine learning, and artificial intelligence to provide quick and accurate translations. In this comprehensive guide, we’ll explore the intricacies of real-time translation algorithms, their applications, challenges, and the future of this rapidly evolving technology.
Understanding Real-Time Translation
Real-time translation, also known as simultaneous translation or live translation, refers to the process of converting spoken or written language from one language to another with minimal delay. This technology aims to provide near-instantaneous translation, allowing for seamless communication between individuals who speak different languages.
Key Components of Real-Time Translation Algorithms
- Speech Recognition: Converting spoken language into text
- Machine Translation: Translating the recognized text into the target language
- Text-to-Speech Synthesis: Converting the translated text back into spoken language
- Natural Language Processing: Understanding context, idioms, and nuances
The Evolution of Translation Algorithms
The journey of translation algorithms has been marked by significant advancements over the years:
1. Rule-Based Machine Translation (RBMT)
Early translation systems relied on predefined linguistic rules and dictionaries. While these systems were straightforward, they struggled with complex sentence structures and idiomatic expressions.
2. Statistical Machine Translation (SMT)
SMT systems use statistical models to learn translation patterns from large parallel corpora of translated texts. This approach improved translation quality but still faced challenges with rare words and long-distance dependencies.
3. Neural Machine Translation (NMT)
The current state-of-the-art in translation algorithms, NMT uses deep learning techniques to model the entire translation process end-to-end. This approach has significantly improved translation quality and fluency.
Core Algorithms in Real-Time Translation
Let’s delve into some of the key algorithms and techniques used in real-time translation systems:
1. Recurrent Neural Networks (RNNs)
RNNs, particularly Long Short-Term Memory (LSTM) networks, have been widely used in sequence-to-sequence models for machine translation. They are effective at capturing context and long-term dependencies in language.
import tensorflow as tf
# Simple LSTM model for sequence-to-sequence translation
model = tf.keras.Sequential([
tf.keras.layers.Embedding(input_dim=vocab_size, output_dim=embedding_dim),
tf.keras.layers.LSTM(units=64, return_sequences=True),
tf.keras.layers.LSTM(units=64),
tf.keras.layers.Dense(units=vocab_size, activation='softmax')
])
2. Transformer Architecture
The Transformer model, introduced in the paper “Attention Is All You Need,” has revolutionized machine translation. It uses self-attention mechanisms to capture relationships between words, regardless of their position in the sentence.
import tensorflow as tf
# Simplified Transformer model
class TransformerModel(tf.keras.Model):
def __init__(self, num_layers, d_model, num_heads, dff, input_vocab_size, target_vocab_size, pe_input, pe_target, rate=0.1):
super(TransformerModel, self).__init__()
self.encoder = Encoder(num_layers, d_model, num_heads, dff, input_vocab_size, pe_input, rate)
self.decoder = Decoder(num_layers, d_model, num_heads, dff, target_vocab_size, pe_target, rate)
self.final_layer = tf.keras.layers.Dense(target_vocab_size)
def call(self, inputs, targets, training, enc_padding_mask, look_ahead_mask, dec_padding_mask):
enc_output = self.encoder(inputs, training, enc_padding_mask)
dec_output, attention_weights = self.decoder(targets, enc_output, training, look_ahead_mask, dec_padding_mask)
final_output = self.final_layer(dec_output)
return final_output, attention_weights
3. Byte Pair Encoding (BPE)
BPE is a data compression technique adapted for NLP tasks. It helps handle rare words and improves translation of compound words by breaking them into subword units.
from tokenizers import Tokenizer
from tokenizers.models import BPE
from tokenizers.trainers import BpeTrainer
# Initialize BPE tokenizer
tokenizer = Tokenizer(BPE(unk_token="[UNK]"))
trainer = BpeTrainer(special_tokens=["[UNK]", "[CLS]", "[SEP]", "[PAD]", "[MASK]"])
# Train the tokenizer
files = ["path/to/file1.txt", "path/to/file2.txt"]
tokenizer.train(files, trainer)
# Save the tokenizer
tokenizer.save("path/to/tokenizer.json")
Challenges in Real-Time Translation
While real-time translation algorithms have made significant progress, several challenges remain:
1. Handling Context and Ambiguity
Languages often contain words with multiple meanings or context-dependent interpretations. Real-time translation algorithms must quickly determine the correct context to provide accurate translations.
2. Idiomatic Expressions and Cultural Nuances
Translating idioms and culturally specific expressions poses a significant challenge. Literal translations often fail to convey the intended meaning.
3. Low-Resource Languages
For languages with limited available data, training accurate translation models becomes challenging. This is particularly true for many indigenous and less commonly spoken languages.
4. Latency and Computational Requirements
Real-time translation requires fast processing to maintain a natural flow of conversation. Balancing speed and accuracy is a constant challenge, especially on mobile devices with limited computational resources.
Optimizing Real-Time Translation Algorithms
To address these challenges and improve the performance of real-time translation systems, researchers and developers employ various optimization techniques:
1. Transfer Learning
Transfer learning allows models trained on high-resource language pairs to be fine-tuned for low-resource languages, improving translation quality with limited data.
import tensorflow as tf
# Load pre-trained model
base_model = tf.keras.models.load_model('pretrained_translation_model.h5')
# Freeze base layers
for layer in base_model.layers[:-2]:
layer.trainable = False
# Add new layers for fine-tuning
x = base_model.output
x = tf.keras.layers.Dense(64, activation='relu')(x)
output = tf.keras.layers.Dense(target_vocab_size, activation='softmax')(x)
# Create new model
fine_tuned_model = tf.keras.Model(inputs=base_model.input, outputs=output)
# Compile and train
fine_tuned_model.compile(optimizer='adam', loss='sparse_categorical_crossentropy', metrics=['accuracy'])
fine_tuned_model.fit(low_resource_data, epochs=10, validation_split=0.2)
2. Attention Mechanisms
Attention mechanisms allow models to focus on relevant parts of the input when generating translations, improving accuracy and handling of long-distance dependencies.
import tensorflow as tf
def scaled_dot_product_attention(q, k, v, mask):
matmul_qk = tf.matmul(q, k, transpose_b=True)
dk = tf.cast(tf.shape(k)[-1], tf.float32)
scaled_attention_logits = matmul_qk / tf.math.sqrt(dk)
if mask is not None:
scaled_attention_logits += (mask * -1e9)
attention_weights = tf.nn.softmax(scaled_attention_logits, axis=-1)
output = tf.matmul(attention_weights, v)
return output, attention_weights
3. Multilingual Models
Training a single model on multiple language pairs can improve overall translation quality and reduce the need for language-specific models.
import tensorflow as tf
class MultilingualTransformer(tf.keras.Model):
def __init__(self, num_languages, *args, **kwargs):
super(MultilingualTransformer, self).__init__()
self.language_embedding = tf.keras.layers.Embedding(num_languages, 64)
self.transformer = TransformerModel(*args, **kwargs)
def call(self, inputs, language_id, *args, **kwargs):
lang_emb = self.language_embedding(language_id)
# Combine language embedding with input
enhanced_input = tf.concat([inputs, tf.tile(tf.expand_dims(lang_emb, 1), [1, tf.shape(inputs)[1], 1])], axis=-1)
return self.transformer(enhanced_input, *args, **kwargs)
4. Knowledge Distillation
Knowledge distillation can be used to create smaller, faster models that retain much of the accuracy of larger models, making them more suitable for real-time applications.
import tensorflow as tf
# Teacher model (large, accurate model)
teacher_model = tf.keras.models.load_model('large_translation_model.h5')
# Student model (smaller, faster model)
student_model = create_small_model()
# Knowledge distillation loss
def distillation_loss(y_true, y_pred, teacher_pred, temperature=2.0, alpha=0.1):
hard_loss = tf.keras.losses.sparse_categorical_crossentropy(y_true, y_pred)
soft_targets = tf.nn.softmax(teacher_pred / temperature)
soft_prob = tf.nn.softmax(y_pred / temperature)
soft_loss = tf.keras.losses.categorical_crossentropy(soft_targets, soft_prob)
return alpha * hard_loss + (1 - alpha) * soft_loss * (temperature ** 2)
# Training loop
for epoch in range(num_epochs):
for batch in dataset:
with tf.GradientTape() as tape:
student_pred = student_model(batch['input'])
teacher_pred = teacher_model(batch['input'])
loss = distillation_loss(batch['target'], student_pred, teacher_pred)
gradients = tape.gradient(loss, student_model.trainable_variables)
optimizer.apply_gradients(zip(gradients, student_model.trainable_variables))
Applications of Real-Time Translation Algorithms
Real-time translation algorithms have found applications in various domains:
1. Travel and Tourism
Mobile apps with real-time translation capabilities help travelers communicate with locals, read signs, and navigate foreign countries more easily.
2. Business and International Relations
Real-time translation facilitates smoother communication in multinational business meetings and diplomatic exchanges.
3. Education
Language learning apps and platforms use real-time translation to provide immediate feedback and explanations to learners.
4. Healthcare
In medical settings, real-time translation can help healthcare providers communicate with patients who speak different languages, ensuring accurate diagnosis and treatment.
5. Social Media and Online Communities
Real-time translation enables users from different linguistic backgrounds to interact on social media platforms and online forums.
The Future of Real-Time Translation Algorithms
As technology continues to advance, we can expect several exciting developments in the field of real-time translation:
1. Improved Contextual Understanding
Future algorithms will likely have a better grasp of context, allowing for more nuanced and accurate translations, especially for idiomatic expressions and cultural references.
2. Multimodal Translation
Integrating visual and auditory cues alongside text could lead to more accurate translations by providing additional context.
3. Personalized Translation Models
Adaptive models that learn from user feedback and personal communication styles could provide more natural and personalized translations.
4. Real-Time Speech-to-Speech Translation
Advancements in speech recognition and synthesis could lead to more seamless voice-based translation systems, potentially eliminating the need for text as an intermediary.
5. Edge Computing for Lower Latency
Deploying translation models on edge devices could reduce latency and improve the real-time aspect of translations, especially in areas with limited internet connectivity.
Ethical Considerations in Real-Time Translation
As real-time translation technology becomes more prevalent, it’s crucial to consider the ethical implications:
1. Privacy Concerns
Real-time translation often involves processing sensitive conversations. Ensuring the privacy and security of this data is paramount.
2. Bias in Translation
Translation models can inadvertently perpetuate biases present in their training data. Efforts must be made to identify and mitigate these biases.
3. Impact on Language Learning
While real-time translation can facilitate communication, it’s important to consider its potential impact on language learning and cultural exchange.
4. Accuracy and Liability
In critical situations, such as legal or medical contexts, the accuracy of translations is crucial. Clear guidelines on the limitations and liability of real-time translation systems are necessary.
Conclusion
Real-time translation algorithms represent a fascinating intersection of linguistics, artificial intelligence, and computer science. As these algorithms continue to evolve, they promise to break down language barriers and foster global communication in unprecedented ways. From neural machine translation to advanced optimization techniques, the field is ripe with innovation and potential.
For developers and AI enthusiasts, understanding and working with real-time translation algorithms offers an exciting opportunity to contribute to a technology that has the power to connect people across linguistic and cultural divides. As we look to the future, the continued advancement of these algorithms will undoubtedly play a crucial role in shaping our increasingly interconnected world.
Whether you’re a seasoned developer looking to integrate real-time translation into your applications or a budding AI researcher exploring the frontiers of natural language processing, the field of real-time translation algorithms offers a wealth of challenges and opportunities. By staying informed about the latest developments and best practices in this rapidly evolving field, you can position yourself at the forefront of a technology that is truly changing the way we communicate globally.