Implementing Secure Communication Algorithms: A Comprehensive Guide
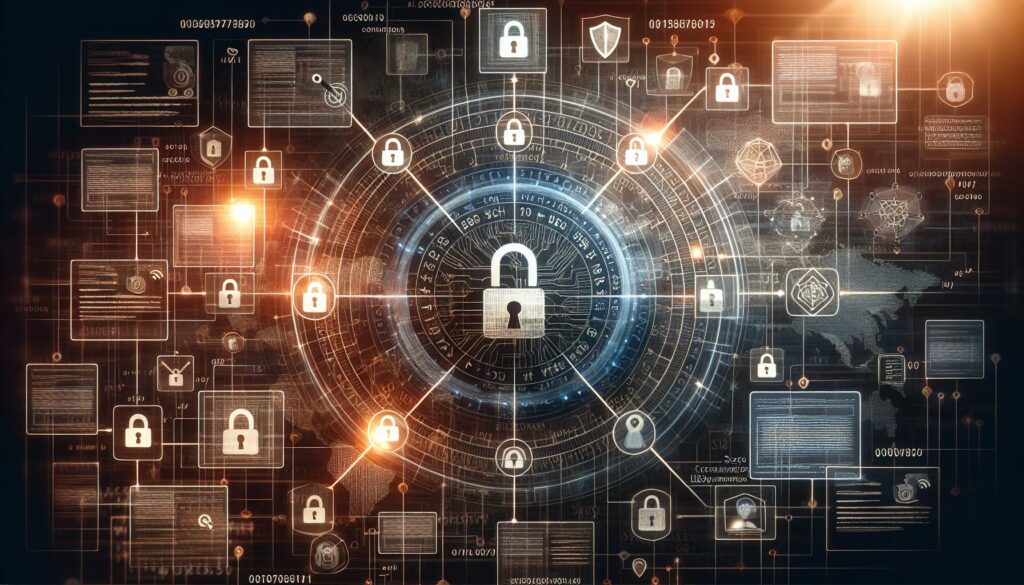
In today’s digital age, secure communication is more important than ever. As we increasingly rely on technology for personal and professional interactions, the need to protect sensitive information from unauthorized access has become paramount. This comprehensive guide will explore the world of secure communication algorithms, their implementation, and best practices for ensuring the confidentiality and integrity of your data.
Table of Contents
- Introduction to Secure Communication
- Encryption Algorithms
- Key Exchange Protocols
- Digital Signatures
- Cryptographic Hash Functions
- Implementing Secure Communication
- Best Practices and Security Considerations
- The Future of Secure Communication
- Conclusion
1. Introduction to Secure Communication
Secure communication refers to the process of exchanging information between parties in a way that prevents unauthorized access or tampering. The primary goals of secure communication are:
- Confidentiality: Ensuring that only intended recipients can read the message
- Integrity: Verifying that the message has not been altered during transmission
- Authentication: Confirming the identity of the sender
- Non-repudiation: Preventing the sender from denying they sent the message
To achieve these goals, we rely on various cryptographic algorithms and protocols. Let’s dive into the core components of secure communication.
2. Encryption Algorithms
Encryption is the process of converting plaintext (readable data) into ciphertext (encrypted data) using an algorithm and a key. There are two main types of encryption algorithms:
2.1 Symmetric Encryption
Symmetric encryption uses the same key for both encryption and decryption. Popular symmetric encryption algorithms include:
- AES (Advanced Encryption Standard)
- DES (Data Encryption Standard) and 3DES (Triple DES)
- Blowfish
- Twofish
Here’s a simple example of AES encryption in Python using the PyCryptodome library:
from Crypto.Cipher import AES
from Crypto.Random import get_random_bytes
def encrypt_aes(plaintext, key):
cipher = AES.new(key, AES.MODE_EAX)
ciphertext, tag = cipher.encrypt_and_digest(plaintext.encode())
return (cipher.nonce, ciphertext, tag)
# Generate a random 256-bit key
key = get_random_bytes(32)
# Encrypt a message
message = "Hello, World!"
encrypted = encrypt_aes(message, key)
print(f"Encrypted: {encrypted}")
2.2 Asymmetric Encryption
Asymmetric encryption, also known as public-key cryptography, uses a pair of keys: a public key for encryption and a private key for decryption. Common asymmetric encryption algorithms include:
- RSA (Rivest-Shamir-Adleman)
- ECC (Elliptic Curve Cryptography)
- DSA (Digital Signature Algorithm)
Here’s an example of RSA encryption using Python’s cryptography library:
from cryptography.hazmat.primitives.asymmetric import rsa, padding
from cryptography.hazmat.primitives import hashes
def generate_rsa_keys():
private_key = rsa.generate_private_key(
public_exponent=65537,
key_size=2048
)
public_key = private_key.public_key()
return private_key, public_key
def encrypt_rsa(message, public_key):
ciphertext = public_key.encrypt(
message.encode(),
padding.OAEP(
mgf=padding.MGF1(algorithm=hashes.SHA256()),
algorithm=hashes.SHA256(),
label=None
)
)
return ciphertext
# Generate RSA key pair
private_key, public_key = generate_rsa_keys()
# Encrypt a message
message = "Hello, World!"
encrypted = encrypt_rsa(message, public_key)
print(f"Encrypted: {encrypted}")
3. Key Exchange Protocols
Key exchange protocols allow two parties to securely agree on a shared secret key over an insecure channel. These protocols are crucial for establishing secure communication sessions. Some popular key exchange protocols include:
3.1 Diffie-Hellman Key Exchange
The Diffie-Hellman key exchange is a method that allows two parties to generate a shared secret key without ever transmitting the key itself. Here’s a simplified implementation of the Diffie-Hellman algorithm in Python:
import random
def generate_prime():
# This is a simplified prime generation function
# In practice, use a cryptographically secure method
return 23 # Example prime number
def generate_primitive_root(prime):
# This is a simplified primitive root generation function
# In practice, use a cryptographically secure method
return 5 # Example primitive root
def diffie_hellman(prime, primitive_root):
# Generate private key
private_key = random.randint(1, prime - 1)
# Calculate public key
public_key = pow(primitive_root, private_key, prime)
return private_key, public_key
# Simulate key exchange between Alice and Bob
prime = generate_prime()
primitive_root = generate_primitive_root(prime)
alice_private, alice_public = diffie_hellman(prime, primitive_root)
bob_private, bob_public = diffie_hellman(prime, primitive_root)
# Calculate shared secret
alice_shared_secret = pow(bob_public, alice_private, prime)
bob_shared_secret = pow(alice_public, bob_private, prime)
print(f"Alice's shared secret: {alice_shared_secret}")
print(f"Bob's shared secret: {bob_shared_secret}")
assert alice_shared_secret == bob_shared_secret, "Shared secrets do not match!"
3.2 Elliptic Curve Diffie-Hellman (ECDH)
ECDH is a variant of the Diffie-Hellman protocol that uses elliptic curve cryptography. It provides the same level of security as traditional Diffie-Hellman but with smaller key sizes, making it more efficient.
4. Digital Signatures
Digital signatures provide a way to verify the authenticity and integrity of a message or document. They use asymmetric cryptography to create a unique signature that can be verified using the sender’s public key.
Here’s an example of creating and verifying a digital signature using RSA in Python:
from cryptography.hazmat.primitives import hashes
from cryptography.hazmat.primitives.asymmetric import padding, rsa
def generate_rsa_keys():
private_key = rsa.generate_private_key(
public_exponent=65537,
key_size=2048
)
public_key = private_key.public_key()
return private_key, public_key
def sign_message(message, private_key):
signature = private_key.sign(
message.encode(),
padding.PSS(
mgf=padding.MGF1(hashes.SHA256()),
salt_length=padding.PSS.MAX_LENGTH
),
hashes.SHA256()
)
return signature
def verify_signature(message, signature, public_key):
try:
public_key.verify(
signature,
message.encode(),
padding.PSS(
mgf=padding.MGF1(hashes.SHA256()),
salt_length=padding.PSS.MAX_LENGTH
),
hashes.SHA256()
)
return True
except:
return False
# Generate RSA key pair
private_key, public_key = generate_rsa_keys()
# Sign a message
message = "Hello, World!"
signature = sign_message(message, private_key)
# Verify the signature
is_valid = verify_signature(message, signature, public_key)
print(f"Signature is valid: {is_valid}")
# Try to verify with a tampered message
tampered_message = "Hello, World? "
is_valid = verify_signature(tampered_message, signature, public_key)
print(f"Tampered signature is valid: {is_valid}")
5. Cryptographic Hash Functions
Cryptographic hash functions play a crucial role in secure communication by providing a way to create a fixed-size, unique representation of data. These functions have several important properties:
- One-way: It should be computationally infeasible to reverse the hash and obtain the original input
- Deterministic: The same input always produces the same hash
- Avalanche effect: A small change in the input results in a significantly different hash
- Collision-resistant: It should be extremely difficult to find two different inputs that produce the same hash
Common cryptographic hash functions include:
- SHA-256 (part of the SHA-2 family)
- SHA-3
- BLAKE2
- MD5 (no longer considered secure for cryptographic purposes)
Here’s an example of using SHA-256 in Python:
import hashlib
def sha256_hash(data):
return hashlib.sha256(data.encode()).hexdigest()
# Hash a message
message = "Hello, World!"
hashed = sha256_hash(message)
print(f"SHA-256 hash: {hashed}")
# Demonstrate the avalanche effect
slightly_different_message = "Hello, World?"
hashed2 = sha256_hash(slightly_different_message)
print(f"SHA-256 hash of slightly different message: {hashed2}")
6. Implementing Secure Communication
Now that we’ve covered the fundamental algorithms and protocols, let’s look at how to implement secure communication in a practical scenario. We’ll create a simple client-server application that uses asymmetric encryption for key exchange and symmetric encryption for message transmission.
First, let’s implement the server:
import socket
from cryptography.hazmat.primitives.asymmetric import rsa, padding
from cryptography.hazmat.primitives import hashes, serialization
from cryptography.hazmat.primitives.ciphers import Cipher, algorithms, modes
import os
def generate_rsa_keys():
private_key = rsa.generate_private_key(
public_exponent=65537,
key_size=2048
)
public_key = private_key.public_key()
return private_key, public_key
def encrypt_aes(key, plaintext):
iv = os.urandom(16)
cipher = Cipher(algorithms.AES(key), modes.CFB(iv))
encryptor = cipher.encryptor()
ciphertext = encryptor.update(plaintext) + encryptor.finalize()
return iv + ciphertext
def decrypt_aes(key, ciphertext):
iv = ciphertext[:16]
cipher = Cipher(algorithms.AES(key), modes.CFB(iv))
decryptor = cipher.decryptor()
return decryptor.update(ciphertext[16:]) + decryptor.finalize()
def server():
private_key, public_key = generate_rsa_keys()
server_socket = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
server_socket.bind(('localhost', 12345))
server_socket.listen(1)
print("Server is listening...")
client_socket, address = server_socket.accept()
print(f"Connection from {address} has been established!")
# Send public key to client
public_key_bytes = public_key.public_bytes(
encoding=serialization.Encoding.PEM,
format=serialization.PublicFormat.SubjectPublicKeyInfo
)
client_socket.send(public_key_bytes)
# Receive encrypted AES key from client
encrypted_aes_key = client_socket.recv(256)
# Decrypt AES key
aes_key = private_key.decrypt(
encrypted_aes_key,
padding.OAEP(
mgf=padding.MGF1(algorithm=hashes.SHA256()),
algorithm=hashes.SHA256(),
label=None
)
)
# Receive and decrypt message
encrypted_message = client_socket.recv(1024)
decrypted_message = decrypt_aes(aes_key, encrypted_message)
print(f"Received message: {decrypted_message.decode()}")
client_socket.close()
server_socket.close()
if __name__ == "__main__":
server()
Now, let’s implement the client:
import socket
from cryptography.hazmat.primitives.asymmetric import padding
from cryptography.hazmat.primitives import hashes, serialization
from cryptography.hazmat.primitives.ciphers import Cipher, algorithms, modes
import os
def encrypt_aes(key, plaintext):
iv = os.urandom(16)
cipher = Cipher(algorithms.AES(key), modes.CFB(iv))
encryptor = cipher.encryptor()
ciphertext = encryptor.update(plaintext) + encryptor.finalize()
return iv + ciphertext
def client():
client_socket = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
client_socket.connect(('localhost', 12345))
# Receive public key from server
public_key_bytes = client_socket.recv(1024)
public_key = serialization.load_pem_public_key(public_key_bytes)
# Generate AES key
aes_key = os.urandom(32)
# Encrypt AES key with server's public key
encrypted_aes_key = public_key.encrypt(
aes_key,
padding.OAEP(
mgf=padding.MGF1(algorithm=hashes.SHA256()),
algorithm=hashes.SHA256(),
label=None
)
)
# Send encrypted AES key to server
client_socket.send(encrypted_aes_key)
# Encrypt and send message
message = "Hello, secure world!"
encrypted_message = encrypt_aes(aes_key, message.encode())
client_socket.send(encrypted_message)
print("Message sent securely.")
client_socket.close()
if __name__ == "__main__":
client()
This implementation demonstrates a basic secure communication setup using RSA for key exchange and AES for message encryption. In a real-world scenario, you would need to add error handling, implement proper key management, and consider using established protocols like TLS/SSL for more robust security.
7. Best Practices and Security Considerations
When implementing secure communication algorithms, it’s crucial to follow best practices and consider various security aspects:
- Use well-established, peer-reviewed cryptographic libraries and algorithms. Don’t implement cryptographic primitives from scratch unless you’re an expert in the field.
- Keep cryptographic keys secure and use proper key management techniques. Consider using hardware security modules (HSMs) for key storage in high-security environments.
- Regularly update and patch your cryptographic libraries to address any discovered vulnerabilities.
- Use sufficiently large key sizes and strong algorithms. For example, use at least 2048-bit keys for RSA and 256-bit keys for AES.
- Implement proper authentication mechanisms alongside encryption to ensure the identity of communicating parties.
- Use secure random number generators for generating keys and initialization vectors.
- Implement perfect forward secrecy by using ephemeral keys for each session.
- Be aware of side-channel attacks and implement appropriate countermeasures.
- Regularly audit and test your security implementations to identify and address potential vulnerabilities.
- Stay informed about the latest developments in cryptography and security, as new attacks and vulnerabilities are discovered regularly.
8. The Future of Secure Communication
As technology advances, so do the threats to secure communication. Here are some areas to watch for future developments:
8.1 Post-Quantum Cryptography
With the advent of quantum computers, many current cryptographic algorithms (especially those based on factoring large numbers or discrete logarithms) will become vulnerable. Post-quantum cryptography aims to develop algorithms that are resistant to attacks by quantum computers. Some promising candidates include:
- Lattice-based cryptography
- Hash-based signatures
- Code-based cryptography
- Multivariate polynomial cryptography
8.2 Homomorphic Encryption
Homomorphic encryption allows computations to be performed on encrypted data without decrypting it first. This has significant implications for cloud computing and data privacy. While fully homomorphic encryption is still computationally expensive, partially homomorphic schemes are becoming more practical.
8.3 Quantum Key Distribution (QKD)
QKD uses quantum mechanical properties to generate and distribute encryption keys. It provides a way to detect any eavesdropping attempts during key exchange, making it theoretically unbreakable. However, practical implementations still face challenges in terms of distance limitations and hardware requirements.
8.4 Blockchain-based Secure Communication
Blockchain technology, with its decentralized and tamper-resistant nature, is being explored for secure communication applications. This includes secure messaging platforms, identity verification systems, and secure data storage solutions.
9. Conclusion
Implementing secure communication algorithms is a critical aspect of modern software development. By understanding the fundamental concepts of encryption, key exchange, digital signatures, and cryptographic hash functions, you can build robust and secure communication systems.
Remember that security is an ongoing process, not a one-time implementation. Stay informed about the latest developments in cryptography, follow best practices, and regularly update your security measures to protect against evolving threats.
As we move towards a future with quantum computers and increasingly sophisticated cyber threats, the field of secure communication will continue to evolve. By staying ahead of these developments and implementing strong security measures, you can ensure that your communications remain confidential, authentic, and secure.