Algorithms Behind Voice Recognition Technology: Decoding the Magic of Speech-to-Text
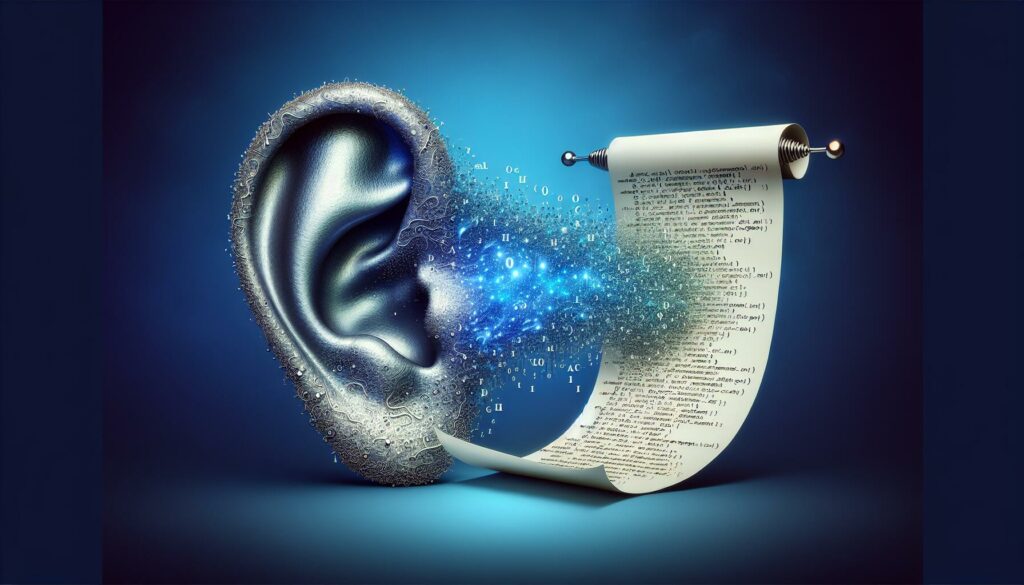
In today’s digital age, voice recognition technology has become an integral part of our daily lives. From virtual assistants like Siri and Alexa to automated customer service systems, the ability of machines to understand and interpret human speech has revolutionized how we interact with technology. But have you ever wondered about the complex algorithms that power these seemingly magical systems? In this comprehensive guide, we’ll dive deep into the world of voice recognition technology, exploring the intricate algorithms that make it possible for machines to understand human speech.
Understanding Voice Recognition Technology
Before we delve into the specific algorithms, it’s important to understand what voice recognition technology actually is. Voice recognition, also known as speech recognition, is the ability of a machine or program to identify words and phrases in spoken language and convert them into a machine-readable format. This technology has come a long way since its inception, evolving from simple command-and-control systems to sophisticated AI-powered assistants capable of understanding natural language and context.
The Voice Recognition Process
The process of voice recognition can be broken down into several key steps:
- Audio Input: Capturing the spoken words through a microphone or other audio input device.
- Signal Processing: Converting the analog audio signal into a digital format that can be processed by a computer.
- Feature Extraction: Identifying and extracting relevant features from the digital audio signal.
- Acoustic Modeling: Matching the extracted features to known phonemes (the smallest units of sound in speech).
- Language Modeling: Analyzing the sequence of phonemes to determine the most likely words and phrases.
- Text Output: Converting the recognized speech into text or executing a corresponding command.
Now, let’s explore the key algorithms that power each of these steps.
1. Signal Processing Algorithms
Fast Fourier Transform (FFT)
The Fast Fourier Transform is a fundamental algorithm in signal processing. It converts the time-domain audio signal into a frequency-domain representation, which is crucial for analyzing the spectral content of speech.
Here’s a simplified implementation of the FFT algorithm in Python:
import numpy as np
def fft(x):
N = len(x)
if N <= 1:
return x
even = fft(x[0::2])
odd = fft(x[1::2])
T = [np.exp(-2j * np.pi * k / N) * odd[k] for k in range(N // 2)]
return [even[k] + T[k] for k in range(N // 2)] + [even[k] - T[k] for k in range(N // 2)]
# Example usage
signal = [1, 2, 3, 4]
spectrum = fft(signal)
print(spectrum)
Mel-frequency Cepstral Coefficients (MFCCs)
MFCCs are widely used in speech recognition systems to represent the short-term power spectrum of a sound. They are derived from a type of cepstral representation of the audio clip.
Here’s a basic implementation of MFCC extraction using the librosa library:
import librosa
def extract_mfcc(audio_file, n_mfcc=13):
y, sr = librosa.load(audio_file)
mfccs = librosa.feature.mfcc(y=y, sr=sr, n_mfcc=n_mfcc)
return mfccs
# Example usage
audio_file = "speech_sample.wav"
mfccs = extract_mfcc(audio_file)
print(mfccs.shape)
2. Feature Extraction Algorithms
Linear Predictive Coding (LPC)
LPC is a tool used in audio signal processing and speech processing for representing the spectral envelope of a digital signal of speech in compressed form. It works by estimating the formants, removing their effects from the speech signal, and estimating the intensity and frequency of the remaining buzz.
Here’s a simplified implementation of LPC in Python:
import numpy as np
def lpc(signal, order):
n = len(signal)
r = np.zeros(order + 1)
for i in range(order + 1):
r[i] = np.sum(signal[i:n] * signal[:n-i])
R = toeplitz(r[:-1])
a = np.linalg.solve(R, -r[1:])
return np.concatenate(([1], a))
# Example usage
signal = np.random.randn(1000)
lpc_coeffs = lpc(signal, order=10)
print(lpc_coeffs)
3. Acoustic Modeling Algorithms
Hidden Markov Models (HMMs)
Hidden Markov Models have been the backbone of speech recognition systems for decades. They model the temporal structure of speech and the relationship between observed acoustic features and hidden phonetic states.
Here’s a basic implementation of a Hidden Markov Model in Python:
import numpy as np
class HiddenMarkovModel:
def __init__(self, A, B, pi):
self.A = A # State transition probabilities
self.B = B # Emission probabilities
self.pi = pi # Initial state probabilities
def forward(self, observations):
N = len(self.pi)
T = len(observations)
alpha = np.zeros((N, T))
alpha[:, 0] = self.pi * self.B[:, observations[0]]
for t in range(1, T):
for j in range(N):
alpha[j, t] = np.sum(alpha[:, t-1] * self.A[:, j]) * self.B[j, observations[t]]
return alpha
def backward(self, observations):
N = len(self.pi)
T = len(observations)
beta = np.zeros((N, T))
beta[:, -1] = 1
for t in range(T-2, -1, -1):
for j in range(N):
beta[j, t] = np.sum(self.A[j, :] * self.B[:, observations[t+1]] * beta[:, t+1])
return beta
def viterbi(self, observations):
N = len(self.pi)
T = len(observations)
delta = np.zeros((N, T))
psi = np.zeros((N, T), dtype=int)
delta[:, 0] = self.pi * self.B[:, observations[0]]
for t in range(1, T):
for j in range(N):
delta[j, t] = np.max(delta[:, t-1] * self.A[:, j]) * self.B[j, observations[t]]
psi[j, t] = np.argmax(delta[:, t-1] * self.A[:, j])
state_sequence = np.zeros(T, dtype=int)
state_sequence[-1] = np.argmax(delta[:, -1])
for t in range(T-2, -1, -1):
state_sequence[t] = psi[state_sequence[t+1], t+1]
return state_sequence
# Example usage
A = np.array([[0.7, 0.3], [0.4, 0.6]])
B = np.array([[0.5, 0.4, 0.1], [0.1, 0.3, 0.6]])
pi = np.array([0.6, 0.4])
hmm = HiddenMarkovModel(A, B, pi)
observations = [0, 1, 2, 1]
alpha = hmm.forward(observations)
beta = hmm.backward(observations)
state_sequence = hmm.viterbi(observations)
print("Forward probabilities:", alpha)
print("Backward probabilities:", beta)
print("Most likely state sequence:", state_sequence)
Deep Neural Networks (DNNs)
In recent years, Deep Neural Networks have largely replaced HMMs in state-of-the-art speech recognition systems. DNNs can learn complex patterns in speech data and have significantly improved recognition accuracy.
Here’s a simple implementation of a DNN for speech recognition using TensorFlow:
import tensorflow as tf
def create_dnn_model(input_shape, num_classes):
model = tf.keras.Sequential([
tf.keras.layers.Input(shape=input_shape),
tf.keras.layers.Dense(256, activation='relu'),
tf.keras.layers.Dense(128, activation='relu'),
tf.keras.layers.Dense(64, activation='relu'),
tf.keras.layers.Dense(num_classes, activation='softmax')
])
model.compile(optimizer='adam',
loss='categorical_crossentropy',
metrics=['accuracy'])
return model
# Example usage
input_shape = (13,) # Assuming 13 MFCC features
num_classes = 10 # Number of phonemes or words to recognize
model = create_dnn_model(input_shape, num_classes)
model.summary()
4. Language Modeling Algorithms
N-gram Models
N-gram models are widely used in speech recognition to predict the likelihood of a sequence of words. They estimate the probability of a word based on the N-1 preceding words.
Here’s a simple implementation of a bigram (2-gram) model in Python:
from collections import defaultdict
class BigramModel:
def __init__(self):
self.unigram_counts = defaultdict(int)
self.bigram_counts = defaultdict(lambda: defaultdict(int))
self.vocab = set()
def train(self, corpus):
for sentence in corpus:
tokens = ["<s>"] + sentence.split() + ["</s>"]
self.vocab.update(tokens)
for i in range(len(tokens)):
self.unigram_counts[tokens[i]] += 1
if i > 0:
self.bigram_counts[tokens[i-1]][tokens[i]] += 1
def probability(self, word, previous):
numerator = self.bigram_counts[previous][word] + 1 # Add-one smoothing
denominator = sum(self.bigram_counts[previous].values()) + len(self.vocab)
return numerator / denominator
def generate(self, num_words):
current = "<s>"
result = []
for _ in range(num_words):
next_word = max(self.vocab, key=lambda w: self.probability(w, current))
if next_word == "</s>":
break
result.append(next_word)
current = next_word
return ' '.join(result)
# Example usage
corpus = [
"the cat sat on the mat",
"the dog chased the cat",
"the bird flew over the house"
]
model = BigramModel()
model.train(corpus)
print(model.probability("cat", "the"))
print(model.generate(5))
Recurrent Neural Networks (RNNs)
RNNs, particularly Long Short-Term Memory (LSTM) networks, have become popular for language modeling in speech recognition systems. They can capture long-term dependencies in language, improving the accuracy of speech recognition.
Here’s a basic implementation of an LSTM-based language model using TensorFlow:
import tensorflow as tf
def create_lstm_language_model(vocab_size, embedding_dim, rnn_units):
model = tf.keras.Sequential([
tf.keras.layers.Embedding(vocab_size, embedding_dim),
tf.keras.layers.LSTM(rnn_units, return_sequences=True),
tf.keras.layers.Dense(vocab_size)
])
return model
# Example usage
vocab_size = 10000
embedding_dim = 256
rnn_units = 1024
model = create_lstm_language_model(vocab_size, embedding_dim, rnn_units)
model.summary()
Putting It All Together: A Complete Voice Recognition System
Now that we’ve explored the individual algorithms that power voice recognition technology, let’s look at how they all come together in a complete system. Here’s a high-level overview of a basic voice recognition pipeline:
import numpy as np
import librosa
import tensorflow as tf
# Step 1: Audio Input and Signal Processing
def preprocess_audio(audio_file):
y, sr = librosa.load(audio_file)
mfccs = librosa.feature.mfcc(y=y, sr=sr, n_mfcc=13)
return mfccs.T
# Step 2: Acoustic Model (using a simple DNN)
def create_acoustic_model(input_shape, num_phonemes):
model = tf.keras.Sequential([
tf.keras.layers.Input(shape=input_shape),
tf.keras.layers.Dense(256, activation='relu'),
tf.keras.layers.Dense(128, activation='relu'),
tf.keras.layers.Dense(num_phonemes, activation='softmax')
])
model.compile(optimizer='adam', loss='categorical_crossentropy', metrics=['accuracy'])
return model
# Step 3: Language Model (using a simple bigram model)
class BigramLanguageModel:
def __init__(self, vocab):
self.vocab = vocab
self.bigram_counts = defaultdict(lambda: defaultdict(int))
def train(self, corpus):
for sentence in corpus:
tokens = sentence.split()
for i in range(len(tokens) - 1):
self.bigram_counts[tokens[i]][tokens[i+1]] += 1
def predict_next_word(self, previous_word):
if previous_word not in self.bigram_counts:
return random.choice(list(self.vocab))
return max(self.bigram_counts[previous_word], key=self.bigram_counts[previous_word].get)
# Step 4: Decoding (using a simple greedy approach)
def decode_sequence(acoustic_probs, language_model):
sequence = []
previous_word = "<s>"
for probs in acoustic_probs:
phoneme = np.argmax(probs)
word = phoneme_to_word(phoneme) # This function would map phonemes to words
if language_model.predict_next_word(previous_word) == word:
sequence.append(word)
previous_word = word
return ' '.join(sequence)
# Main voice recognition function
def recognize_speech(audio_file, acoustic_model, language_model):
# Preprocess audio
features = preprocess_audio(audio_file)
# Get acoustic probabilities
acoustic_probs = acoustic_model.predict(features)
# Decode the sequence
recognized_text = decode_sequence(acoustic_probs, language_model)
return recognized_text
# Example usage
audio_file = "speech_sample.wav"
num_phonemes = 50
vocab = ["hello", "world", "voice", "recognition"]
corpus = ["hello world", "voice recognition", "hello voice recognition"]
acoustic_model = create_acoustic_model((13,), num_phonemes)
language_model = BigramLanguageModel(vocab)
language_model.train(corpus)
recognized_text = recognize_speech(audio_file, acoustic_model, language_model)
print("Recognized text:", recognized_text)
This example provides a simplified version of a complete voice recognition system. In practice, state-of-the-art systems are much more complex, often incorporating advanced deep learning techniques, sophisticated language models, and additional post-processing steps to improve accuracy.
Challenges and Future Directions
While voice recognition technology has come a long way, there are still several challenges to overcome:
- Accents and Dialects: Recognizing speech from speakers with diverse accents and dialects remains a challenge.
- Background Noise: Accurately recognizing speech in noisy environments is an ongoing area of research.
- Contextual Understanding: Improving the ability of systems to understand context and intent beyond just recognizing words.
- Multilingual Recognition: Developing systems that can seamlessly switch between multiple languages.
- Continuous Learning: Creating systems that can adapt and improve their performance over time through continuous learning.
Future directions in voice recognition technology include:
- Advanced neural network architectures, such as Transformer models, for improved acoustic and language modeling.
- Integration of multimodal information (e.g., combining audio and visual cues) for more robust recognition.
- Personalized models that adapt to individual users’ speech patterns and preferences.
- Edge computing solutions for low-latency, privacy-preserving voice recognition on local devices.
Conclusion
Voice recognition technology is a fascinating field that combines signal processing, machine learning, and linguistics. The algorithms behind this technology are constantly evolving, pushing the boundaries of what’s possible in human-computer interaction. As we’ve seen, creating a voice recognition system involves multiple steps, each powered by sophisticated algorithms.
For aspiring developers and AI enthusiasts, understanding these algorithms is crucial for building the next generation of voice-enabled applications. Whether you’re interested in creating virtual assistants, developing accessibility tools, or exploring new ways for humans to interact with technology, voice recognition is likely to play a significant role in shaping the future of computing.
As you continue your journey in coding and AI, remember that voice recognition is just one of many exciting applications of algorithms and machine learning. The skills you develop in this area – from signal processing to deep learning – will be valuable across a wide range of domains in computer science and artificial intelligence.
Keep exploring, keep coding, and who knows? You might be the one to develop the next breakthrough in voice recognition technology!