Understanding Entropy and Information Theory: A Comprehensive Guide for Programmers
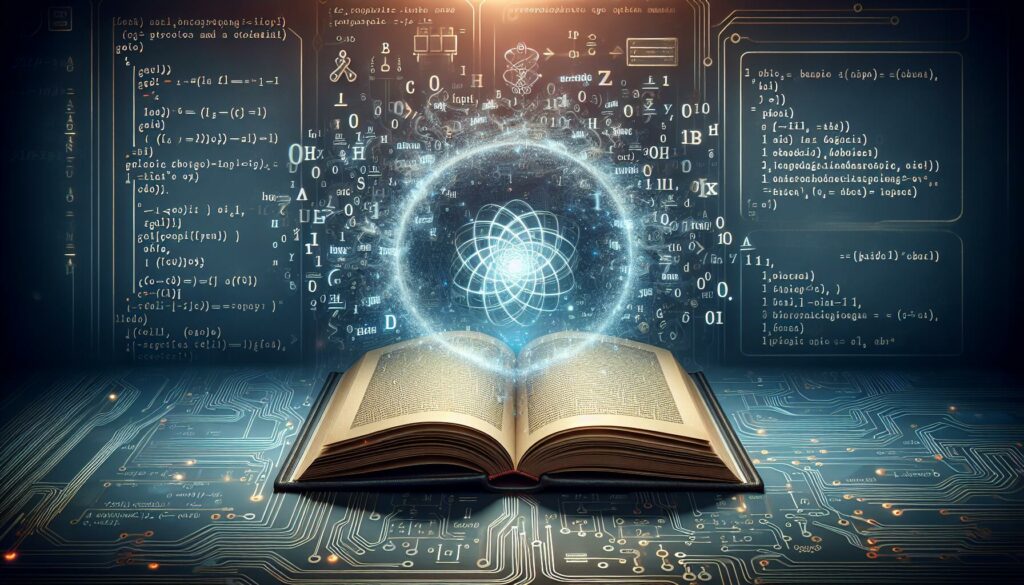
In the realm of computer science and data analysis, entropy and information theory play crucial roles in understanding and quantifying information. These concepts are fundamental to various areas of technology, including data compression, cryptography, and machine learning. For aspiring programmers and those preparing for technical interviews at major tech companies, a solid grasp of these principles is essential. In this comprehensive guide, we’ll delve deep into entropy and information theory, exploring their applications and significance in the world of coding and algorithms.
What is Entropy?
Entropy, in the context of information theory, is a measure of the average amount of information contained in a message. It quantifies the uncertainty or randomness in a set of data. The concept was introduced by Claude Shannon in his groundbreaking 1948 paper “A Mathematical Theory of Communication,” which laid the foundation for modern information theory.
In simple terms, entropy tells us how much information is conveyed by a particular message or data set. The higher the entropy, the more information (or uncertainty) is present.
Mathematical Definition of Entropy
The entropy H of a discrete random variable X with possible values {x1, …, xn} is defined as:
H(X) = -Σ p(xi) * log2(p(xi))
Where:
- p(xi) is the probability of the i-th outcome
- log2 is the base-2 logarithm
- The summation is over all possible outcomes
The unit of entropy is bits when using log base 2. If we use the natural logarithm, the unit is nats, and for base 10, it’s dits or decimal digits.
Information Theory: The Bigger Picture
Information theory, pioneered by Claude Shannon, is a branch of applied mathematics and electrical engineering that deals with the quantification, storage, and communication of information. It provides a framework for understanding the limits of data compression and the capacity of communication channels.
Key concepts in information theory include:
- Entropy: As discussed earlier, it measures the average information content of a message.
- Mutual Information: It quantifies the mutual dependence between two variables.
- Channel Capacity: The maximum rate at which information can be reliably transmitted over a communication channel.
- Data Compression: Techniques to reduce the size of data without losing essential information.
- Error-Correcting Codes: Methods to add redundancy to data to detect and correct errors during transmission.
Practical Applications of Entropy and Information Theory
Understanding entropy and information theory is crucial for several practical applications in computer science and programming. Let’s explore some of these applications:
1. Data Compression
Data compression algorithms rely heavily on the principles of entropy. The goal is to represent data using fewer bits than the original representation. Two main types of compression are:
- Lossless Compression: Preserves all original data (e.g., ZIP, GZIP)
- Lossy Compression: Allows some data loss for higher compression ratios (e.g., JPEG, MP3)
Example: Huffman coding is a popular lossless data compression technique that assigns variable-length codes to characters based on their frequency of occurrence. More frequent characters get shorter codes, reducing the overall size of the encoded data.
2. Cryptography
Entropy plays a crucial role in cryptography, particularly in generating secure encryption keys. A high-entropy key is more resistant to brute-force attacks and provides better security.
Example: In generating random numbers for cryptographic purposes, we aim for high entropy to ensure unpredictability. The Linux kernel’s random number generator (/dev/random) blocks until enough entropy is available to generate truly random numbers.
3. Machine Learning and Information Gain
In decision tree algorithms and feature selection, the concept of information gain (based on entropy) is used to determine the most informative features.
Example: In the ID3 algorithm for decision tree learning, the attribute with the highest information gain is chosen as the splitting criterion at each node.
4. Network Communication
Shannon’s channel capacity theorem sets the theoretical limit for error-free data transmission rate over a noisy channel. This has implications for designing efficient communication protocols and error-correcting codes.
5. Natural Language Processing
Entropy and related concepts are used in various NLP tasks, such as language modeling, text classification, and machine translation.
Example: Perplexity, a measure used to evaluate language models, is derived from the concept of entropy.
Implementing Entropy Calculation in Code
Let’s implement a simple entropy calculation function in Python to illustrate the concept:
import math
def calculate_entropy(data):
if not data:
return 0
entropy = 0
for x in set(data):
p_x = data.count(x) / len(data)
if p_x > 0:
entropy += p_x * math.log2(p_x)
return -entropy
# Example usage
text = "hello world"
entropy = calculate_entropy(text)
print(f"Entropy of '{text}': {entropy:.2f} bits")
This code calculates the entropy of a given string by counting the frequency of each character and applying the entropy formula.
Advanced Topics in Information Theory
For those looking to deepen their understanding of information theory, several advanced topics are worth exploring:
1. Kolmogorov Complexity
Kolmogorov complexity is a measure of the computational resources needed to specify an object. It’s related to entropy but is more general, applying to individual objects rather than random variables.
2. Mutual Information
Mutual information measures the mutual dependence between two variables. It’s used in various machine learning tasks, including feature selection and clustering.
3. Rate-Distortion Theory
This branch of information theory deals with the tradeoff between the rate of data transmission and the distortion introduced by compression, particularly relevant in lossy compression scenarios.
4. Quantum Information Theory
An extension of classical information theory to quantum systems, crucial for understanding quantum computing and communication.
Preparing for Technical Interviews
When preparing for technical interviews, especially at major tech companies, it’s essential to understand how entropy and information theory concepts might come into play:
1. Algorithmic Problems
Be prepared to solve problems related to data compression, encoding, or decoding. Understanding Huffman coding, run-length encoding, or other compression algorithms can be beneficial.
2. System Design Questions
In system design interviews, knowledge of information theory can help in discussing efficient data storage, transmission protocols, or designing scalable systems that handle large amounts of data.
3. Machine Learning Concepts
If interviewing for machine learning positions, be ready to discuss how entropy and information gain are used in decision trees, feature selection, or evaluating model performance.
4. Cryptography and Security
For security-related positions, understanding the role of entropy in generating secure keys and random numbers is crucial.
Practical Exercises to Reinforce Understanding
To solidify your grasp of entropy and information theory, consider working on the following exercises:
1. Implement a Huffman Coding Algorithm
Create a program that compresses and decompresses text using Huffman coding. This will give you hands-on experience with a practical application of entropy in data compression.
2. Analyze Entropy in Different Types of Data
Calculate and compare the entropy of various data sources, such as:
- Random strings vs. meaningful text
- Different image formats (e.g., PNG vs. JPEG)
- Various audio file formats
3. Build a Simple Encryption System
Develop a basic encryption system that uses entropy to measure the strength of encryption keys. This will help you understand the relationship between entropy and cryptographic security.
4. Implement a Decision Tree Algorithm
Create a simple decision tree classifier that uses information gain for feature selection. This will demonstrate the application of entropy in machine learning algorithms.
Conclusion
Entropy and information theory are fundamental concepts in computer science with far-reaching applications. From data compression and cryptography to machine learning and communication systems, these principles underpin many of the technologies we use daily. For programmers and aspiring tech professionals, a solid understanding of these concepts can provide valuable insights and problem-solving tools.
As you prepare for technical interviews or advance in your programming career, remember that entropy and information theory are not just theoretical concepts. They have practical implications in algorithm design, system architecture, and data analysis. By mastering these principles and their applications, you’ll be better equipped to tackle complex problems and contribute to cutting-edge technological advancements.
Continue to explore these topics, work on practical projects, and stay updated with the latest developments in the field. The world of information theory is vast and continually evolving, offering endless opportunities for learning and innovation in the realm of computer science and beyond.