Implementing Game Algorithms: Minimax and Alpha-Beta Pruning
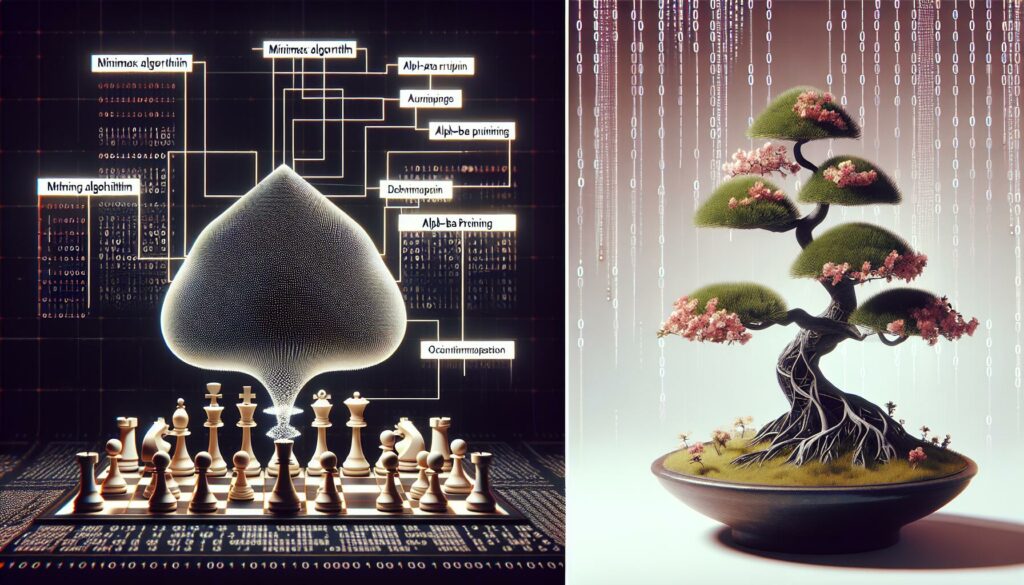
In the world of game development and artificial intelligence, implementing efficient algorithms is crucial for creating challenging and intelligent opponents. Two fundamental algorithms that form the backbone of many game AI systems are Minimax and Alpha-Beta Pruning. These algorithms are particularly useful in turn-based, two-player games with perfect information, such as chess, checkers, and tic-tac-toe. In this comprehensive guide, we’ll explore the concepts behind these algorithms, their implementation, and how they can be used to create formidable AI opponents in games.
Understanding Minimax Algorithm
The Minimax algorithm is a decision-making algorithm used in two-player turn-based games. It’s based on the idea that the AI player tries to maximize its chances of winning while assuming that the opponent will play optimally to minimize the AI’s chances. This algorithm explores all possible moves and their consequences to determine the best move for the current player.
How Minimax Works
- The algorithm creates a game tree representing all possible moves and their outcomes.
- It assigns a score to each leaf node (end game state) based on whether it’s a win, loss, or draw for the AI player.
- It then works backwards from the leaf nodes, alternating between choosing the maximum and minimum scores at each level.
- The AI chooses the move that leads to the highest score, assuming the opponent will always choose the move that leads to the lowest score for the AI.
Implementing Minimax in Python
Let’s implement a basic version of the Minimax algorithm for a simple game like Tic-Tac-Toe:
def minimax(board, depth, is_maximizing):
if check_win(board, "X"):
return 1
if check_win(board, "O"):
return -1
if check_draw(board):
return 0
if is_maximizing:
best_score = float("-inf")
for move in get_available_moves(board):
board[move] = "X"
score = minimax(board, depth + 1, False)
board[move] = " "
best_score = max(score, best_score)
return best_score
else:
best_score = float("inf")
for move in get_available_moves(board):
board[move] = "O"
score = minimax(board, depth + 1, True)
board[move] = " "
best_score = min(score, best_score)
return best_score
def get_best_move(board):
best_score = float("-inf")
best_move = None
for move in get_available_moves(board):
board[move] = "X"
score = minimax(board, 0, False)
board[move] = " "
if score > best_score:
best_score = score
best_move = move
return best_move
In this implementation, we assume that “X” is the AI player (maximizing) and “O” is the human player (minimizing). The minimax
function recursively evaluates all possible moves, and the get_best_move
function uses Minimax to determine the optimal move for the AI player.
Introducing Alpha-Beta Pruning
While Minimax is effective, it can be computationally expensive for complex games with large game trees. This is where Alpha-Beta Pruning comes in. Alpha-Beta Pruning is an optimization technique for the Minimax algorithm that reduces the number of nodes evaluated in the game tree.
How Alpha-Beta Pruning Works
Alpha-Beta Pruning maintains two values, alpha and beta, which represent the minimum score that the maximizing player is assured of and the maximum score that the minimizing player is assured of, respectively. As the algorithm searches through the game tree, it updates these values and uses them to “prune” branches that cannot possibly influence the final decision.
Implementing Alpha-Beta Pruning in Python
Let’s modify our Minimax implementation to include Alpha-Beta Pruning:
def alpha_beta(board, depth, alpha, beta, is_maximizing):
if check_win(board, "X"):
return 1
if check_win(board, "O"):
return -1
if check_draw(board):
return 0
if is_maximizing:
best_score = float("-inf")
for move in get_available_moves(board):
board[move] = "X"
score = alpha_beta(board, depth + 1, alpha, beta, False)
board[move] = " "
best_score = max(score, best_score)
alpha = max(alpha, best_score)
if beta <= alpha:
break
return best_score
else:
best_score = float("inf")
for move in get_available_moves(board):
board[move] = "O"
score = alpha_beta(board, depth + 1, alpha, beta, True)
board[move] = " "
best_score = min(score, best_score)
beta = min(beta, best_score)
if beta <= alpha:
break
return best_score
def get_best_move_alpha_beta(board):
best_score = float("-inf")
best_move = None
alpha = float("-inf")
beta = float("inf")
for move in get_available_moves(board):
board[move] = "X"
score = alpha_beta(board, 0, alpha, beta, False)
board[move] = " "
if score > best_score:
best_score = score
best_move = move
alpha = max(alpha, best_score)
return best_move
The key difference in this implementation is the addition of alpha and beta parameters, which are used to prune branches of the game tree that don’t need to be explored.
Comparing Minimax and Alpha-Beta Pruning
To understand the benefits of Alpha-Beta Pruning, let’s compare the performance of both algorithms:
import time
def compare_algorithms(board):
start_time = time.time()
minimax_move = get_best_move(board)
minimax_time = time.time() - start_time
start_time = time.time()
alpha_beta_move = get_best_move_alpha_beta(board)
alpha_beta_time = time.time() - start_time
print(f"Minimax move: {minimax_move}, Time: {minimax_time:.4f} seconds")
print(f"Alpha-Beta move: {alpha_beta_move}, Time: {alpha_beta_time:.4f} seconds")
# Example usage
board = [" "] * 9
board[0] = "X"
board[4] = "O"
compare_algorithms(board)
Running this comparison on different board states will demonstrate that Alpha-Beta Pruning generally performs faster than pure Minimax, especially as the game tree becomes more complex.
Optimizing Game AI Performance
While Minimax with Alpha-Beta Pruning is a powerful algorithm, there are several techniques we can use to further optimize its performance and make it more suitable for complex games:
1. Transposition Tables
Transposition tables are used to store previously evaluated board positions. This can significantly reduce computation time by avoiding redundant calculations:
from functools import lru_cache
@lru_cache(maxsize=None)
def alpha_beta_with_cache(board_tuple, depth, alpha, beta, is_maximizing):
# Convert tuple back to list for easier manipulation
board = list(board_tuple)
# Rest of the alpha_beta function remains the same
# ...
def get_best_move_alpha_beta_cached(board):
# Convert board to tuple for caching
board_tuple = tuple(board)
# Rest of the get_best_move function remains the same
# ...
Using Python’s lru_cache
decorator, we can easily implement a simple transposition table.
2. Iterative Deepening
Iterative deepening involves running the algorithm multiple times with increasing depth limits. This can be beneficial in time-constrained scenarios:
def iterative_deepening(board, max_depth, time_limit):
start_time = time.time()
best_move = None
for depth in range(1, max_depth + 1):
move = get_best_move_alpha_beta(board, depth)
if time.time() - start_time > time_limit:
break
best_move = move
return best_move
3. Move Ordering
Ordering moves based on their likely quality can significantly improve the efficiency of Alpha-Beta Pruning:
def order_moves(board, moves):
# Simple move ordering: prefer center and corners
move_scores = []
for move in moves:
if move == 4: # Center
score = 3
elif move in [0, 2, 6, 8]: # Corners
score = 2
else: # Edges
score = 1
move_scores.append((move, score))
return [move for move, score in sorted(move_scores, key=lambda x: x[1], reverse=True)]
# Use this in the alpha_beta function:
for move in order_moves(board, get_available_moves(board)):
# Rest of the code remains the same
Applying Minimax and Alpha-Beta Pruning to Different Games
While we’ve focused on Tic-Tac-Toe in our examples, these algorithms can be applied to a wide range of two-player, turn-based games. Let’s explore how to adapt our implementation for different games:
Chess
Implementing Minimax and Alpha-Beta Pruning for chess requires some modifications:
- Board representation: Use a more complex board structure, typically a 2D array.
- Move generation: Implement rules for how each piece can move.
- Evaluation function: Create a sophisticated function to evaluate board positions, considering factors like piece values, position, and game phase.
- Depth limitation: Due to the complexity of chess, limit the search depth and use iterative deepening.
def evaluate_chess_position(board):
# Implement chess-specific evaluation
pass
def generate_chess_moves(board):
# Generate all legal moves for the current player
pass
def alpha_beta_chess(board, depth, alpha, beta, is_maximizing):
if depth == 0 or is_game_over(board):
return evaluate_chess_position(board)
if is_maximizing:
best_score = float("-inf")
for move in generate_chess_moves(board):
make_move(board, move)
score = alpha_beta_chess(board, depth - 1, alpha, beta, False)
undo_move(board, move)
best_score = max(score, best_score)
alpha = max(alpha, best_score)
if beta <= alpha:
break
return best_score
else:
# Similar logic for minimizing player
pass
Connect Four
Connect Four is simpler than chess but more complex than Tic-Tac-Toe. Here’s how we can adapt our algorithm:
def evaluate_connect_four(board):
# Evaluate the board state for Connect Four
pass
def generate_connect_four_moves(board):
# Generate possible moves (column choices)
return [col for col in range(7) if board[0][col] == " "]
def alpha_beta_connect_four(board, depth, alpha, beta, is_maximizing):
if depth == 0 or is_game_over(board):
return evaluate_connect_four(board)
if is_maximizing:
best_score = float("-inf")
for move in generate_connect_four_moves(board):
make_move(board, move, "X")
score = alpha_beta_connect_four(board, depth - 1, alpha, beta, False)
undo_move(board, move)
best_score = max(score, best_score)
alpha = max(alpha, best_score)
if beta <= alpha:
break
return best_score
else:
# Similar logic for minimizing player
pass
Advanced Techniques and Considerations
As we delve deeper into game AI implementation, there are several advanced techniques and considerations to keep in mind:
1. Quiescence Search
In games like chess, evaluating a position immediately after a capture can lead to inaccurate results. Quiescence search extends the search in “volatile” positions to reach a more stable state before evaluation:
def quiescence_search(board, alpha, beta):
stand_pat = evaluate_position(board)
if stand_pat >= beta:
return beta
if alpha < stand_pat:
alpha = stand_pat
for move in generate_capturing_moves(board):
make_move(board, move)
score = -quiescence_search(board, -beta, -alpha)
undo_move(board, move)
if score >= beta:
return beta
if score > alpha:
alpha = score
return alpha
2. Null Move Pruning
Null Move Pruning is based on the idea that if a position is so good that it remains good even after giving the opponent a free move, we can prune large portions of the search tree:
def alpha_beta_null_move(board, depth, alpha, beta, is_maximizing):
if depth <= 0:
return evaluate_position(board)
if not is_maximizing and depth >= 3:
score = -alpha_beta_null_move(board, depth - 3, -beta, -beta + 1, True)
if score >= beta:
return beta
# Rest of alpha-beta implementation
# ...
3. Late Move Reduction
Late Move Reduction (LMR) is based on the assumption that later moves in an ordered move list are less likely to be good. It reduces the depth of the search for these moves:
def alpha_beta_lmr(board, depth, alpha, beta, is_maximizing):
if depth <= 0:
return evaluate_position(board)
moves = order_moves(generate_moves(board))
for i, move in enumerate(moves):
make_move(board, move)
if i < 3 or depth <= 2:
score = -alpha_beta_lmr(board, depth - 1, -beta, -alpha, not is_maximizing)
else:
score = -alpha_beta_lmr(board, depth - 2, -alpha - 1, -alpha, not is_maximizing)
if alpha < score < beta:
score = -alpha_beta_lmr(board, depth - 1, -beta, -alpha, not is_maximizing)
undo_move(board, move)
# Rest of alpha-beta implementation
# ...
4. Opening Books
For many games, the early moves have been thoroughly analyzed. Using an opening book can save computation time and improve play in the early game:
def get_move_from_opening_book(board):
# Look up the current board position in the opening book
# Return a pre-calculated good move if found, otherwise return None
pass
def get_best_move(board):
book_move = get_move_from_opening_book(board)
if book_move:
return book_move
# If no book move, proceed with regular alpha-beta search
return alpha_beta_search(board)
5. Endgame Tablebases
For endgame positions with few pieces, pre-calculated tablebases can provide perfect play:
def lookup_tablebase(board):
# Check if the current position is in the endgame tablebase
# Return the optimal move and outcome if found, otherwise return None
pass
def get_best_move(board):
tablebase_result = lookup_tablebase(board)
if tablebase_result:
return tablebase_result.best_move
# If not in tablebase, proceed with regular search
return alpha_beta_search(board)
Balancing AI Strength and Player Experience
While creating a strong AI is an impressive technical achievement, it’s important to consider the player’s experience. An unbeatable AI can be frustrating for players. Here are some strategies to balance AI strength:
1. Difficulty Levels
Implement multiple difficulty levels by adjusting the search depth or introducing deliberate “mistakes”:
def get_ai_move(board, difficulty):
if difficulty == "easy":
return alpha_beta_search(board, depth=2)
elif difficulty == "medium":
return alpha_beta_search(board, depth=4)
else: # "hard"
return alpha_beta_search(board, depth=6)
2. Randomized Play
Introduce some randomness to make the AI less predictable:
import random
def get_ai_move(board):
best_moves = []
best_score = float("-inf")
for move in generate_moves(board):
score = evaluate_move(board, move)
if score > best_score:
best_moves = [move]
best_score = score
elif score == best_score:
best_moves.append(move)
return random.choice(best_moves)
3. Personality-based AI
Create different AI “personalities” that favor certain types of moves or strategies:
def evaluate_position_with_personality(board, personality):
base_score = evaluate_position(board)
if personality == "aggressive":
base_score += count_attacking_pieces(board) * 0.1
elif personality == "defensive":
base_score += count_defending_pieces(board) * 0.1
return base_score
Conclusion
Implementing game algorithms like Minimax and Alpha-Beta Pruning is a fascinating journey into the world of artificial intelligence and game theory. These algorithms form the foundation of many game AI systems, from simple games like Tic-Tac-Toe to complex ones like chess.
As we’ve seen, the basic principles of these algorithms are relatively straightforward, but their power comes from careful optimization and adaptation to specific game scenarios. Techniques like transposition tables, iterative deepening, and move ordering can significantly enhance the performance of these algorithms, making them suitable for more complex games.
However, creating a strong game AI is just part of the challenge. Balancing AI strength with player experience is crucial for developing engaging games. By implementing difficulty levels, introducing controlled randomness, or creating AI personalities, developers can create AIs that are challenging yet enjoyable to play against.
As you continue your journey in game development and AI programming, remember that these algorithms are just the beginning. The field of game AI is constantly evolving, with new techniques like machine learning and neural networks opening up exciting possibilities for creating even more sophisticated and adaptable game opponents.
Whether you’re developing a simple board game or a complex strategy game, the principles we’ve discussed here will provide a solid foundation for creating intelligent, engaging AI opponents. Happy coding, and may your AIs provide endless challenges and entertainment for your players!