Understanding the Fourier Transform Algorithm: From Theory to Implementation
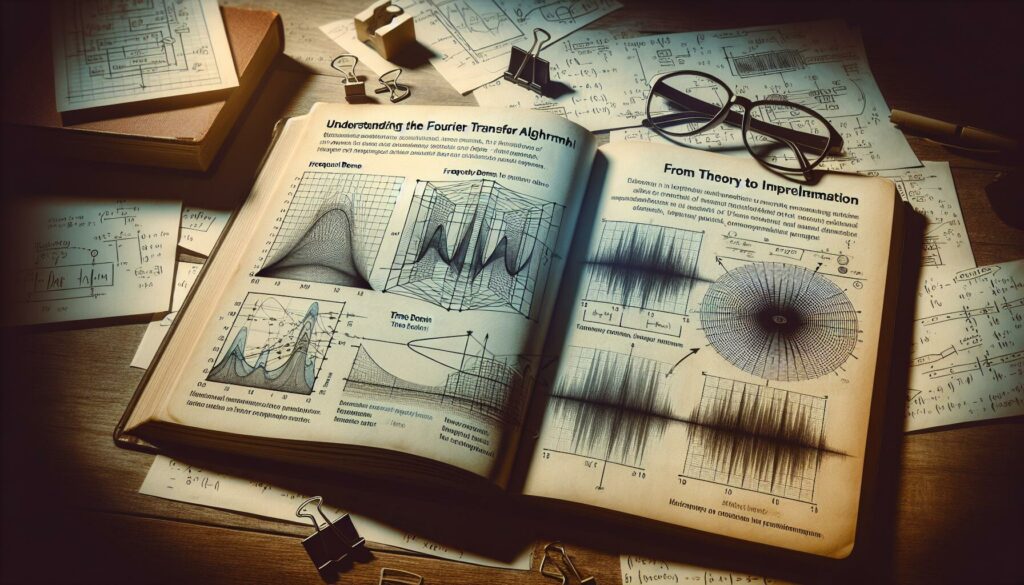
The Fourier Transform is a powerful mathematical tool that has revolutionized various fields, including signal processing, image analysis, and data compression. Named after the French mathematician Joseph Fourier, this algorithm allows us to decompose complex signals into simpler, periodic components. In this comprehensive guide, we’ll dive deep into the Fourier Transform, exploring its theory, applications, and implementation in code.
Table of Contents
- Introduction to the Fourier Transform
- The Theory Behind Fourier Transform
- Types of Fourier Transforms
- Applications of Fourier Transform
- Implementing Fourier Transform in Code
- Optimization Techniques
- Challenges and Considerations
- Conclusion
1. Introduction to the Fourier Transform
The Fourier Transform is a mathematical operation that transforms a function of time, space, or any variable into a function of frequency. It’s based on the principle that any signal can be represented as a sum of sinusoidal functions with different frequencies, amplitudes, and phases.
At its core, the Fourier Transform helps us understand the frequency content of a signal. This is particularly useful because many natural phenomena and man-made systems are better understood in the frequency domain rather than the time domain.
Key Concepts
- Time Domain vs. Frequency Domain: The time domain represents how a signal changes over time, while the frequency domain shows the signal’s frequency components.
- Decomposition: The Fourier Transform decomposes a complex signal into simpler, periodic components.
- Reversibility: The process can be reversed using the Inverse Fourier Transform, allowing us to reconstruct the original signal from its frequency components.
2. The Theory Behind Fourier Transform
The mathematical foundation of the Fourier Transform is based on the idea that any periodic function can be expressed as an infinite sum of sine and cosine terms. This concept is extended to non-periodic functions through the use of integral calculus.
The Continuous Fourier Transform
For a continuous function f(t), the Fourier Transform F(ω) is defined as:
F(ω) = ∫-∞∞ f(t) e-iωt dt
Where:
- ω represents angular frequency
- i is the imaginary unit
- e is the base of natural logarithms
The Inverse Fourier Transform
The Inverse Fourier Transform allows us to reconstruct the original function from its frequency domain representation:
f(t) = 1/(2π) ∫-∞∞ F(ω) eiωt dω
Properties of the Fourier Transform
- Linearity: The Fourier Transform of a sum of functions is the sum of their individual Fourier Transforms.
- Time Shifting: A shift in the time domain corresponds to a phase shift in the frequency domain.
- Frequency Shifting: A shift in the frequency domain corresponds to modulation in the time domain.
- Scaling: Scaling in one domain results in inverse scaling in the other domain.
- Convolution Theorem: Convolution in one domain equals multiplication in the other domain.
3. Types of Fourier Transforms
There are several types of Fourier Transforms, each suited for different types of data and applications:
Continuous Fourier Transform (CFT)
The CFT deals with continuous, non-periodic signals. It’s mostly used in theoretical analysis and serves as the foundation for other types of Fourier Transforms.
Discrete Fourier Transform (DFT)
The DFT is used for discrete, finite-duration signals. It’s the most common form used in digital signal processing and is defined as:
X[k] = Σn=0N-1 x[n] e-i2πkn/N
Where:
- x[n] is the input signal
- X[k] is the DFT output
- N is the number of samples
Fast Fourier Transform (FFT)
The FFT is an efficient algorithm for computing the DFT. It reduces the computational complexity from O(N²) to O(N log N), making it feasible for large datasets.
Short-Time Fourier Transform (STFT)
The STFT is used for analyzing non-stationary signals. It divides the signal into short, overlapping segments and applies the Fourier Transform to each segment.
4. Applications of Fourier Transform
The Fourier Transform has a wide range of applications across various fields:
Signal Processing
- Filtering noise from signals
- Analyzing audio signals for speech recognition
- Equalizing audio in music production
Image Processing
- Image compression (e.g., JPEG format)
- Edge detection and feature extraction
- Image filtering and enhancement
Communications
- Modulation and demodulation in wireless communications
- Analyzing and designing communication systems
Scientific Computing
- Solving partial differential equations
- Analyzing astronomical data
- Quantum mechanics calculations
Data Compression
- MP3 audio compression
- JPEG image compression
5. Implementing Fourier Transform in Code
Let’s look at how to implement the Discrete Fourier Transform (DFT) and the Fast Fourier Transform (FFT) in Python.
Implementing DFT
Here’s a basic implementation of the DFT in Python:
import numpy as np
def dft(x):
N = len(x)
n = np.arange(N)
k = n.reshape((N, 1))
e = np.exp(-2j * np.pi * k * n / N)
return np.dot(e, x)
# Example usage
x = np.array([1, 2, 3, 4])
X = dft(x)
print(X)
This implementation has a time complexity of O(N²), which can be slow for large inputs.
Implementing FFT
The FFT is a more efficient algorithm for computing the DFT. Here’s a basic recursive implementation of the Cooley-Tukey FFT algorithm:
import numpy as np
def fft(x):
N = len(x)
if N <= 1:
return x
else:
X_even = fft(x[0::2])
X_odd = fft(x[1::2])
factor = np.exp(-2j * np.pi * np.arange(N) / N)
return np.concatenate([X_even + factor[:N//2] * X_odd,
X_even + factor[N//2:] * X_odd])
# Example usage
x = np.array([1, 2, 3, 4, 5, 6, 7, 8])
X = fft(x)
print(X)
This implementation has a time complexity of O(N log N), making it much faster for large inputs.
Using NumPy’s FFT
For practical applications, it’s often best to use optimized libraries. NumPy provides an efficient implementation of FFT:
import numpy as np
# Example usage
x = np.array([1, 2, 3, 4, 5, 6, 7, 8])
X = np.fft.fft(x)
print(X)
# Inverse FFT
x_reconstructed = np.fft.ifft(X)
print(x_reconstructed)
6. Optimization Techniques
When working with Fourier Transforms, especially in performance-critical applications, several optimization techniques can be employed:
1. Use of Specialized Libraries
Libraries like FFTW (Fastest Fourier Transform in the West) are highly optimized for various hardware architectures. In Python, you can use the pyfftw
library which provides bindings to FFTW:
import pyfftw
# Create input array
a = pyfftw.empty_aligned(1024, dtype='complex128')
# Create FFT object
fft_object = pyfftw.builders.fft(a)
# Perform FFT
b = fft_object()
2. Parallel Computing
For large datasets, parallel computing can significantly speed up FFT computations. Libraries like scipy.fft
in Python can automatically utilize multiple CPU cores:
from scipy import fft
# Perform parallel FFT
X = fft.fft(x, workers=-1) # -1 uses all available cores
3. GPU Acceleration
GPUs can perform FFT computations much faster than CPUs for large datasets. Libraries like cuFFT for CUDA or PyOpenCL can be used for GPU-accelerated FFT:
import pycuda.autoinit
import pycuda.gpuarray as gpuarray
import skcuda.fft as cu_fft
# Transfer data to GPU
x_gpu = gpuarray.to_gpu(x.astype(np.complex64))
# Perform GPU FFT
X_gpu = cu_fft.fft(x_gpu)
# Transfer result back to CPU
X = X_gpu.get()
4. Pruning
When only a subset of the frequency components is needed, pruned FFT algorithms can be more efficient. These algorithms compute only the required output values, reducing computational complexity.
5. Memory Management
Efficient memory management is crucial for large-scale FFT computations. Techniques like in-place FFT can be used to reduce memory usage:
import numpy as np
# In-place FFT
x = np.array([1, 2, 3, 4, 5, 6, 7, 8], dtype=complex)
np.fft.fft(x, overwrite_x=True)
7. Challenges and Considerations
While the Fourier Transform is a powerful tool, there are several challenges and considerations to keep in mind when using it:
1. Aliasing
Aliasing occurs when a signal is sampled at a rate lower than twice its highest frequency component (Nyquist rate). This can lead to incorrect frequency analysis. To prevent aliasing:
- Ensure the sampling rate is at least twice the highest frequency in the signal.
- Use anti-aliasing filters before sampling.
2. Spectral Leakage
Spectral leakage happens when the signal being analyzed is not perfectly periodic within the observation window. This can be mitigated by:
- Using windowing functions (e.g., Hann, Hamming) to smooth the edges of the signal.
- Choosing an appropriate window size.
3. Computational Complexity
For very large datasets, even the O(N log N) complexity of FFT can be challenging. Strategies to address this include:
- Using distributed computing for extremely large datasets.
- Implementing approximate FFT algorithms for applications where some accuracy can be sacrificed for speed.
4. Numerical Precision
Floating-point arithmetic can lead to accumulation of errors, especially for large N. To mitigate this:
- Use higher precision data types when necessary.
- Consider using libraries that implement extended precision arithmetic.
5. Interpretation of Results
Interpreting Fourier Transform results can be challenging, especially for complex signals. It’s important to:
- Understand the meaning of magnitude and phase spectra.
- Be aware of the limitations of frequency analysis for non-stationary signals.
6. Choice of Transform
Choosing the right type of Fourier Transform is crucial. Consider:
- Using STFT or Wavelet Transform for non-stationary signals.
- Using DFT or FFT for discrete, finite signals.
- Using specialized transforms (e.g., Fractional Fourier Transform) for specific applications.
8. Conclusion
The Fourier Transform is a fundamental algorithm with wide-ranging applications in signal processing, data analysis, and scientific computing. Understanding its theory, implementation, and optimization techniques is crucial for any data scientist or engineer working with signal analysis or frequency-domain problems.
As we’ve seen, the Fourier Transform allows us to decompose complex signals into their frequency components, opening up new ways to analyze and manipulate data. From the basic Discrete Fourier Transform to the efficient Fast Fourier Transform, and even to specialized variants like the Short-Time Fourier Transform, this family of algorithms provides powerful tools for understanding and working with signals in various domains.
However, it’s important to be aware of the challenges and limitations associated with Fourier Transform, such as aliasing, spectral leakage, and computational complexity. By understanding these issues and knowing how to address them, you can effectively leverage the power of Fourier Transform in your projects.
As you continue to explore and apply the Fourier Transform, remember that practice and experimentation are key to mastering this powerful technique. Try implementing the algorithms yourself, experiment with different types of signals, and explore the various applications in fields like audio processing, image analysis, and scientific computing. With time and experience, you’ll develop a deep intuition for working in the frequency domain and be able to apply these skills to solve complex real-world problems.
The Fourier Transform is just one example of the many powerful algorithms and techniques in the field of signal processing and data analysis. As you progress in your journey as a programmer or data scientist, you’ll encounter many more such tools. Each of these will open up new possibilities and ways of thinking about data, helping you become a more versatile and effective problem solver in the ever-evolving world of technology.