Implementing Priority Queues with Heaps: A Comprehensive Guide
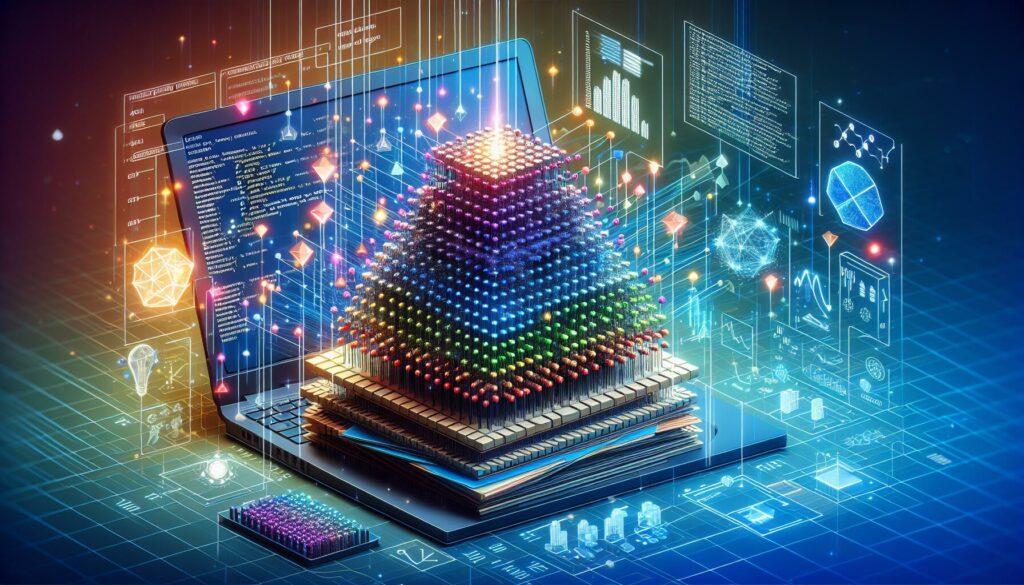
In the world of computer science and programming, efficient data structures play a crucial role in solving complex problems and optimizing algorithms. Among these structures, priority queues stand out as a versatile tool for managing elements based on their priority. When it comes to implementing priority queues, heaps emerge as an excellent choice due to their efficiency and performance characteristics. In this comprehensive guide, we’ll dive deep into the concept of priority queues, explore the intricacies of heaps, and learn how to implement priority queues using heaps.
Understanding Priority Queues
Before we delve into the implementation details, let’s first understand what priority queues are and why they’re important in programming.
What is a Priority Queue?
A priority queue is an abstract data type that operates similarly to a regular queue, with one key difference: each element in a priority queue has an associated priority. When removing elements from the queue, the element with the highest priority is always removed first. If two elements have the same priority, they are served according to their order in the queue.
Real-world Applications of Priority Queues
Priority queues find applications in various domains, including:
- Task scheduling in operating systems
- Dijkstra’s algorithm for finding the shortest path in graphs
- Huffman coding in data compression
- Event-driven simulation
- Bandwidth management in network routers
Basic Operations of a Priority Queue
The fundamental operations of a priority queue include:
- Insert(element, priority): Add an element with its associated priority to the queue.
- DeleteMax() or DeleteMin(): Remove and return the element with the highest (or lowest) priority.
- Peek(): Return the element with the highest (or lowest) priority without removing it.
Introduction to Heaps
Now that we understand priority queues, let’s explore heaps, which serve as an efficient underlying data structure for implementing priority queues.
What is a Heap?
A heap is a specialized tree-based data structure that satisfies the heap property. There are two types of heaps:
- Max Heap: The parent node is always greater than or equal to its children.
- Min Heap: The parent node is always less than or equal to its children.
Heaps are commonly implemented as binary trees, where each node has at most two children.
Properties of a Binary Heap
Binary heaps possess several important properties:
- Shape Property: A binary heap is a complete binary tree, meaning all levels are fully filled except possibly the last level, which is filled from left to right.
- Heap Property: For a max heap, the key of each node is greater than or equal to the keys of its children. For a min heap, the key of each node is less than or equal to the keys of its children.
- Efficient Operations: Insert and DeleteMax/DeleteMin operations can be performed in O(log n) time, where n is the number of elements in the heap.
Implementing a Priority Queue with a Binary Heap
Now, let’s implement a priority queue using a binary max heap. We’ll use Python for our implementation, but the concepts can be easily translated to other programming languages.
Basic Structure
First, let’s define the basic structure of our PriorityQueue class:
class PriorityQueue:
def __init__(self):
self.heap = []
def parent(self, i):
return (i - 1) // 2
def left_child(self, i):
return 2 * i + 1
def right_child(self, i):
return 2 * i + 2
def swap(self, i, j):
self.heap[i], self.heap[j] = self.heap[j], self.heap[i]
def is_empty(self):
return len(self.heap) == 0
def size(self):
return len(self.heap)
This basic structure provides methods to calculate parent and child indices, swap elements, and check if the queue is empty or get its size.
Inserting Elements
To insert an element into the priority queue, we add it to the end of the heap and then “bubble it up” to its correct position:
def insert(self, key):
self.heap.append(key)
self._bubble_up(len(self.heap) - 1)
def _bubble_up(self, i):
parent = self.parent(i)
if i > 0 and self.heap[i] > self.heap[parent]:
self.swap(i, parent)
self._bubble_up(parent)
The _bubble_up
method compares the newly inserted element with its parent and swaps them if the heap property is violated. This process continues until the heap property is satisfied.
Removing the Maximum Element
To remove the maximum element (the root in a max heap), we replace it with the last element in the heap and then “bubble it down” to its correct position:
def delete_max(self):
if self.is_empty():
raise IndexError("Priority queue is empty")
max_val = self.heap[0]
self.heap[0] = self.heap[-1]
self.heap.pop()
self._bubble_down(0)
return max_val
def _bubble_down(self, i):
max_index = i
left = self.left_child(i)
right = self.right_child(i)
if left < len(self.heap) and self.heap[left] > self.heap[max_index]:
max_index = left
if right < len(self.heap) and self.heap[right] > self.heap[max_index]:
max_index = right
if i != max_index:
self.swap(i, max_index)
self._bubble_down(max_index)
The _bubble_down
method compares the element with its children and swaps it with the larger child if necessary. This process continues until the heap property is restored.
Peeking at the Maximum Element
To peek at the maximum element without removing it, we simply return the root of the heap:
def peek(self):
if self.is_empty():
raise IndexError("Priority queue is empty")
return self.heap[0]
Time Complexity Analysis
Let’s analyze the time complexity of the main operations in our priority queue implementation:
- Insert: O(log n) – In the worst case, we need to bubble up the element from the bottom to the top of the heap.
- DeleteMax: O(log n) – We need to bubble down the element from the root to its correct position.
- Peek: O(1) – We simply return the root element.
The space complexity of the priority queue is O(n), where n is the number of elements in the queue.
Advanced Concepts and Optimizations
While our basic implementation is functional, there are several advanced concepts and optimizations we can consider:
1. Array-based Implementation
Instead of using a tree structure, heaps are often implemented using arrays. This approach offers better memory efficiency and cache performance. In an array-based implementation:
- The root is at index 0
- For a node at index i:
- Its left child is at index 2i + 1
- Its right child is at index 2i + 2
- Its parent is at index (i – 1) // 2
2. Heapify Operation
When building a heap from an existing array of elements, we can use the “heapify” operation, which has a time complexity of O(n) and is more efficient than inserting n elements individually (which would take O(n log n)).
def heapify(self, arr):
self.heap = arr
for i in range(len(arr) // 2 - 1, -1, -1):
self._bubble_down(i)
3. Priority Queue with Custom Comparators
To make our priority queue more flexible, we can allow custom comparators. This enables us to prioritize elements based on different criteria:
class PriorityQueue:
def __init__(self, comparator=lambda x, y: x > y):
self.heap = []
self.comparator = comparator
# ... (other methods remain the same)
def _bubble_up(self, i):
parent = self.parent(i)
if i > 0 and self.comparator(self.heap[i], self.heap[parent]):
self.swap(i, parent)
self._bubble_up(parent)
def _bubble_down(self, i):
max_index = i
left = self.left_child(i)
right = self.right_child(i)
if left < len(self.heap) and self.comparator(self.heap[left], self.heap[max_index]):
max_index = left
if right < len(self.heap) and self.comparator(self.heap[right], self.heap[max_index]):
max_index = right
if i != max_index:
self.swap(i, max_index)
self._bubble_down(max_index)
4. Decrease Key and Delete Operations
For some applications, it’s useful to implement “decrease key” (for min heaps) or “increase key” (for max heaps) operations, as well as the ability to delete arbitrary elements from the heap. These operations can be implemented with O(log n) time complexity.
5. Fibonacci Heaps
For advanced applications requiring faster amortized performance for some operations, Fibonacci heaps can be considered. They offer O(1) amortized time for insert and decrease-key operations, while still maintaining O(log n) for delete-min.
Practical Applications and Coding Challenges
To solidify your understanding of priority queues and heaps, consider tackling the following coding challenges:
- Merge K Sorted Lists: Given k sorted linked lists, merge them into a single sorted list using a priority queue.
- Top K Frequent Elements: Find the k most frequent elements in an array using a priority queue.
- Median in a Stream: Design a data structure that can efficiently insert numbers and find the median of the stored numbers.
- Dijkstra’s Algorithm: Implement Dijkstra’s algorithm for finding the shortest path in a weighted graph using a priority queue.
- Task Scheduler: Given a set of tasks with cooldown periods, schedule them efficiently using a priority queue.
Conclusion
Priority queues implemented with heaps are powerful data structures that find applications in various domains of computer science and software engineering. By understanding their underlying principles and implementation details, you can leverage their efficiency to solve complex problems and optimize algorithms.
As you continue your journey in coding education and programming skills development, remember that mastering data structures like priority queues is crucial for technical interviews, especially when preparing for positions at major tech companies. Practice implementing priority queues from scratch, solve related coding challenges, and explore their applications in real-world scenarios to strengthen your algorithmic thinking and problem-solving skills.
With a solid grasp of priority queues and heaps, you’ll be well-equipped to tackle a wide range of programming challenges and design efficient solutions to complex problems. Keep practicing, exploring, and pushing your boundaries to become a more skilled and versatile programmer.