The Role of Algorithms in Music and Audio Processing
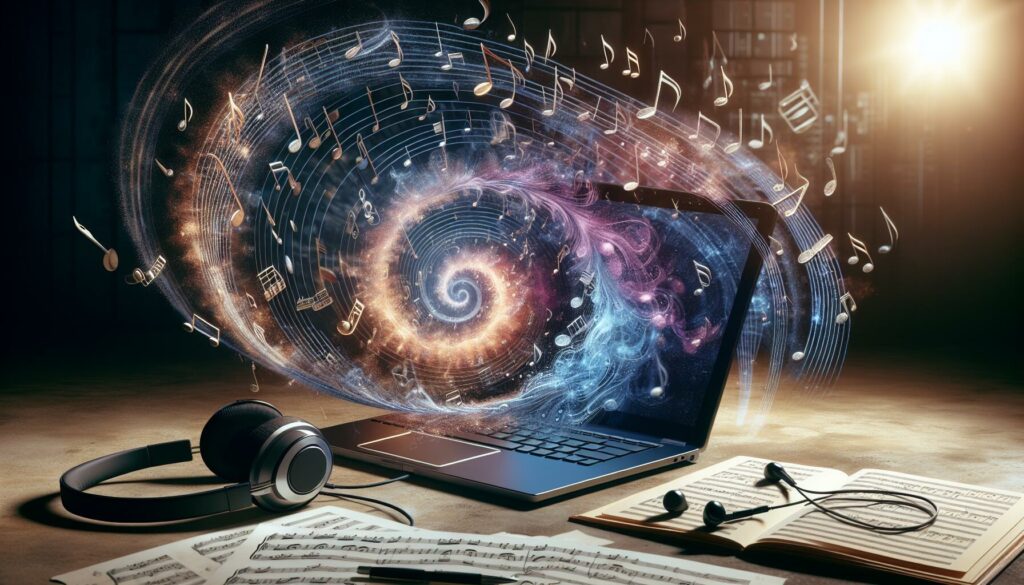
In the ever-evolving landscape of technology, algorithms play a crucial role in shaping various aspects of our digital experiences. One area where algorithms have made significant strides is in music and audio processing. From the way we discover new songs to how we create and manipulate sound, algorithms are at the heart of many innovations in the music industry. In this comprehensive guide, we’ll explore the fascinating world of algorithms in music and audio processing, their applications, and how they’re changing the way we interact with sound.
Understanding Algorithms in Music and Audio
Before diving into the specifics, it’s essential to understand what we mean by algorithms in the context of music and audio processing. An algorithm is a step-by-step procedure or formula for solving a problem or accomplishing a task. In the realm of music and audio, algorithms are used to analyze, manipulate, and generate sound in various ways.
Types of Algorithms Used in Music and Audio Processing
- Digital Signal Processing (DSP) Algorithms
- Machine Learning Algorithms
- Compression Algorithms
- Synthesis Algorithms
- Music Information Retrieval (MIR) Algorithms
Each of these algorithm types serves different purposes in the music and audio processing pipeline, from enhancing sound quality to generating entirely new compositions.
Digital Signal Processing (DSP) Algorithms
Digital Signal Processing algorithms are fundamental to modern audio processing. These algorithms work with digital representations of audio signals to modify, enhance, or analyze them.
Common DSP Algorithms in Audio
- Fast Fourier Transform (FFT): Used to convert time-domain signals to frequency-domain representations, enabling spectral analysis and manipulation.
- Filters: Low-pass, high-pass, and band-pass filters to shape the frequency content of audio signals.
- Convolution: Applied in reverb effects and acoustic space simulation.
- Compression: Dynamic range compression to control the volume levels of audio signals.
Implementation Example: Simple Low-Pass Filter
Here’s a basic implementation of a low-pass filter in Python:
import numpy as np
def low_pass_filter(signal, cutoff_frequency, sample_rate):
# Create frequency domain representation
fft = np.fft.fft(signal)
frequencies = np.fft.fftfreq(len(signal), 1/sample_rate)
# Create low-pass filter
mask = np.abs(frequencies) < cutoff_frequency
# Apply filter
fft_filtered = fft * mask
# Convert back to time domain
filtered_signal = np.fft.ifft(fft_filtered).real
return filtered_signal
# Usage
sample_rate = 44100 # Hz
cutoff_frequency = 1000 # Hz
t = np.linspace(0, 1, sample_rate, endpoint=False)
signal = np.sin(2*np.pi*440*t) + np.sin(2*np.pi*5000*t)
filtered_signal = low_pass_filter(signal, cutoff_frequency, sample_rate)
This simple example demonstrates how a low-pass filter can be implemented using the Fast Fourier Transform to remove high-frequency components from an audio signal.
Machine Learning Algorithms in Music
Machine learning has revolutionized many aspects of music processing and generation. These algorithms can learn patterns from vast amounts of data and apply that knowledge to various tasks.
Applications of Machine Learning in Music
- Music Recommendation Systems: Platforms like Spotify and Pandora use collaborative filtering and content-based algorithms to suggest music to users.
- Genre Classification: Automatic categorization of songs into genres based on audio features.
- Mood Detection: Analyzing the emotional content of music for playlist creation or music therapy applications.
- Automatic Music Generation: Creating new melodies, harmonies, or even entire compositions using deep learning models.
Example: Simple Genre Classification with Scikit-learn
Here’s a basic example of how you might approach genre classification using machine learning:
from sklearn.model_selection import train_test_split
from sklearn.ensemble import RandomForestClassifier
from sklearn.metrics import accuracy_score
import numpy as np
# Assume we have extracted features and labels
features = np.random.rand(1000, 20) # 1000 songs, 20 features each
labels = np.random.randint(0, 5, 1000) # 5 genres
# Split the data
X_train, X_test, y_train, y_test = train_test_split(features, labels, test_size=0.2, random_state=42)
# Train a Random Forest classifier
clf = RandomForestClassifier(n_estimators=100, random_state=42)
clf.fit(X_train, y_train)
# Make predictions
predictions = clf.predict(X_test)
# Calculate accuracy
accuracy = accuracy_score(y_test, predictions)
print(f"Accuracy: {accuracy:.2f}")
This example uses a Random Forest classifier to categorize songs into genres based on extracted audio features. In a real-world scenario, you would need to extract meaningful features from audio files and have a labeled dataset of songs with known genres.
Compression Algorithms in Audio
Audio compression algorithms are essential for efficient storage and transmission of digital audio. These algorithms aim to reduce the file size of audio data while maintaining acceptable sound quality.
Types of Audio Compression
- Lossless Compression: Reduces file size without any loss of audio quality (e.g., FLAC, ALAC).
- Lossy Compression: Achieves greater file size reduction by discarding some audio data (e.g., MP3, AAC).
Popular Audio Compression Algorithms
- MP3 (MPEG-1 Audio Layer III): A widely used lossy compression format that uses perceptual coding to remove less noticeable audio components.
- AAC (Advanced Audio Coding): Designed to be the successor to MP3, offering better sound quality at similar bit rates.
- Opus: A versatile codec that can handle both speech and music, used in applications like VoIP and streaming.
- FLAC (Free Lossless Audio Codec): A popular lossless format that typically reduces file sizes by 50-60% without quality loss.
Implementing a Simple Run-Length Encoding
While not suitable for actual audio compression, run-length encoding is a simple compression technique that can illustrate the concept:
def run_length_encode(data):
encoded = []
count = 1
for i in range(1, len(data)):
if data[i] == data[i-1]:
count += 1
else:
encoded.append((data[i-1], count))
count = 1
encoded.append((data[-1], count))
return encoded
def run_length_decode(encoded):
return [val for val, count in encoded for _ in range(count)]
# Example usage
original = [1, 1, 1, 2, 2, 3, 3, 3, 3, 1, 1]
encoded = run_length_encode(original)
decoded = run_length_decode(encoded)
print(f"Original: {original}")
print(f"Encoded: {encoded}")
print(f"Decoded: {decoded}")
This simple example demonstrates the concept of run-length encoding, which compresses data by replacing sequences of identical values with a single value and a count.
Synthesis Algorithms
Synthesis algorithms are used to generate audio signals, often to create musical sounds or special effects. These algorithms form the basis of many digital synthesizers and sound design tools.
Common Synthesis Techniques
- Additive Synthesis: Combining multiple sine waves to create complex tones.
- Subtractive Synthesis: Starting with a harmonically rich waveform and applying filters to shape the sound.
- FM Synthesis: Using frequency modulation to create complex, evolving sounds.
- Granular Synthesis: Combining small fragments of sound (grains) to create new textures.
- Physical Modeling: Simulating the physical properties of instruments to generate realistic sounds.
Example: Simple Additive Synthesis in Python
Here’s a basic implementation of additive synthesis to create a simple chord:
import numpy as np
import sounddevice as sd
def generate_sine_wave(frequency, duration, sample_rate=44100):
t = np.linspace(0, duration, int(sample_rate * duration), False)
return np.sin(2 * np.pi * frequency * t)
def create_chord(frequencies, duration, sample_rate=44100):
chord = np.zeros(int(sample_rate * duration))
for freq in frequencies:
chord += generate_sine_wave(freq, duration, sample_rate)
return chord / len(frequencies) # Normalize amplitude
# Create a C major chord (C4, E4, G4)
chord_frequencies = [261.63, 329.63, 392.00]
duration = 2.0 # seconds
sample_rate = 44100
chord = create_chord(chord_frequencies, duration, sample_rate)
# Play the chord
sd.play(chord, sample_rate)
sd.wait()
This example demonstrates how multiple sine waves can be combined to create a simple chord using additive synthesis. In practice, more complex waveforms and envelopes would be used to create more realistic and interesting sounds.
Music Information Retrieval (MIR) Algorithms
Music Information Retrieval algorithms focus on extracting meaningful information from music. These algorithms are crucial for various applications, from music recommendation systems to automatic music transcription.
Common MIR Tasks
- Beat Detection: Identifying the rhythmic pulse of a piece of music.
- Chord Recognition: Automatically determining the chords used in a song.
- Melody Extraction: Isolating the main melodic line from a complex audio mixture.
- Instrument Recognition: Identifying the instruments present in a recording.
- Music Similarity: Determining how similar two pieces of music are based on various features.
Example: Basic Beat Detection
Here’s a simplified example of beat detection using the librosa library:
import librosa
import numpy as np
def detect_beats(audio_file):
# Load the audio file
y, sr = librosa.load(audio_file)
# Use librosa's beat detection
tempo, beat_frames = librosa.beat.beat_track(y=y, sr=sr)
# Convert beat frames to time
beat_times = librosa.frames_to_time(beat_frames, sr=sr)
return tempo, beat_times
# Usage
audio_file = "path/to/your/audio/file.mp3"
tempo, beat_times = detect_beats(audio_file)
print(f"Estimated tempo: {tempo:.2f} BPM")
print(f"Beat times: {beat_times[:10]}...") # Print first 10 beat times
This example uses librosa’s beat tracking algorithm to estimate the tempo and locate the beats in an audio file. In practice, more sophisticated techniques might be used for improved accuracy, especially for complex or variable-tempo music.
The Future of Algorithms in Music and Audio Processing
As technology continues to advance, we can expect to see even more innovative applications of algorithms in music and audio processing. Some emerging trends include:
- AI-powered Music Composition: More sophisticated algorithms for generating original music in various styles.
- Improved Audio Restoration: Better algorithms for removing noise and restoring old or damaged recordings.
- Real-time Audio Processing: Advanced DSP algorithms for live performance and studio applications.
- Personalized Audio Experiences: Algorithms that adapt audio content based on individual preferences and listening environments.
- Cross-modal Music Analysis: Integrating audio analysis with other data sources like lyrics, music videos, and social media.
Conclusion
Algorithms play a vital role in shaping the modern music and audio processing landscape. From the way we create and manipulate sound to how we discover and enjoy music, these mathematical constructs are at the heart of many innovations. As we’ve explored in this article, various types of algorithms – from DSP and machine learning to synthesis and MIR – each contribute uniquely to different aspects of audio technology.
For aspiring programmers and audio enthusiasts, understanding these algorithms opens up a world of possibilities. Whether you’re interested in developing music apps, creating digital instruments, or pushing the boundaries of audio technology, a solid grasp of these algorithmic concepts is invaluable.
As we look to the future, the intersection of music, audio, and technology promises to be an exciting and rapidly evolving field. By staying informed about these developments and honing your skills in areas like signal processing, machine learning, and creative coding, you’ll be well-positioned to contribute to and benefit from the ongoing revolution in music and audio technology.
Remember, the examples provided in this article are just starting points. To truly master these concepts, practice implementing them, experiment with different approaches, and don’t be afraid to push the boundaries of what’s possible. Happy coding, and may your algorithmic explorations in music and audio be both enlightening and harmonious!