Implementing Multithreading in Algorithms: Boosting Performance and Efficiency
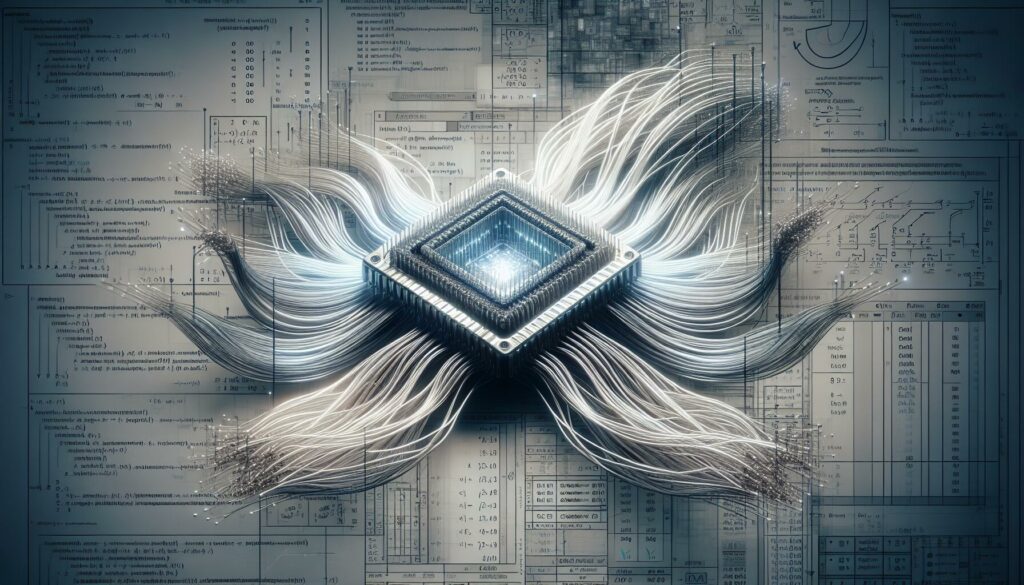
In the world of modern computing, where processing power is abundant and multi-core processors are the norm, understanding and implementing multithreading in algorithms has become increasingly important. As we delve deeper into the realm of coding education and programming skills development, it’s crucial to explore how multithreading can significantly enhance the performance and efficiency of our algorithms. This article will guide you through the concept of multithreading, its benefits, and practical implementations in various algorithmic scenarios.
Understanding Multithreading
Before we dive into the implementation details, let’s first understand what multithreading is and why it’s essential in today’s programming landscape.
What is Multithreading?
Multithreading is a programming concept that allows multiple threads of execution to run concurrently within a single program. A thread is the smallest unit of execution within a process, and multithreading enables a program to perform multiple tasks simultaneously, taking advantage of modern multi-core processors.
Why is Multithreading Important?
In the context of algorithmic problem-solving and software development, multithreading offers several advantages:
- Improved Performance: By utilizing multiple cores, multithreaded algorithms can significantly reduce execution time.
- Enhanced Responsiveness: In applications with user interfaces, multithreading can keep the UI responsive while performing heavy computations in the background.
- Efficient Resource Utilization: Multithreading allows for better utilization of system resources, particularly in I/O-bound operations.
- Scalability: Multithreaded algorithms can easily scale with the number of available cores, making them future-proof.
Basics of Implementing Multithreading
Now that we understand the importance of multithreading, let’s look at how to implement it in our algorithms. We’ll use Python as our primary language for examples, but the concepts can be applied to other programming languages as well.
The threading Module in Python
Python provides the threading
module, which allows us to create and manage threads. Here’s a basic example of how to create and start a thread:
import threading
def print_numbers():
for i in range(1, 6):
print(f"Thread 1: {i}")
def print_letters():
for letter in 'ABCDE':
print(f"Thread 2: {letter}")
# Create thread objects
thread1 = threading.Thread(target=print_numbers)
thread2 = threading.Thread(target=print_letters)
# Start the threads
thread1.start()
thread2.start()
# Wait for both threads to complete
thread1.join()
thread2.join()
print("Both threads have finished execution.")
In this example, we create two threads that run concurrently, one printing numbers and the other printing letters.
Synchronization and Locks
When working with multithreaded algorithms, it’s crucial to handle shared resources properly to avoid race conditions and ensure thread safety. Python provides the Lock
class for this purpose:
import threading
counter = 0
lock = threading.Lock()
def increment_counter():
global counter
for _ in range(100000):
with lock:
counter += 1
# Create and start two threads
thread1 = threading.Thread(target=increment_counter)
thread2 = threading.Thread(target=increment_counter)
thread1.start()
thread2.start()
thread1.join()
thread2.join()
print(f"Final counter value: {counter}")
In this example, we use a lock to ensure that only one thread can increment the counter at a time, preventing race conditions.
Multithreading in Common Algorithms
Now that we’ve covered the basics, let’s explore how multithreading can be applied to common algorithms to improve their performance.
1. Parallel Merge Sort
Merge Sort is a divide-and-conquer algorithm that can benefit significantly from multithreading. Here’s an implementation of a parallel merge sort:
import threading
def merge(left, right):
result = []
i, j = 0, 0
while i < len(left) and j < len(right):
if left[i] <= right[j]:
result.append(left[i])
i += 1
else:
result.append(right[j])
j += 1
result.extend(left[i:])
result.extend(right[j:])
return result
def merge_sort(arr):
if len(arr) <= 1:
return arr
mid = len(arr) // 2
left = arr[:mid]
right = arr[mid:]
# Create threads for sorting left and right halves
left_thread = threading.Thread(target=lambda: left.extend(merge_sort(left)))
right_thread = threading.Thread(target=lambda: right.extend(merge_sort(right)))
left_thread.start()
right_thread.start()
left_thread.join()
right_thread.join()
return merge(left, right)
# Example usage
arr = [64, 34, 25, 12, 22, 11, 90]
sorted_arr = merge_sort(arr)
print(f"Sorted array: {sorted_arr}")
This implementation creates separate threads for sorting the left and right halves of the array, potentially utilizing multiple cores and reducing the overall execution time.
2. Parallel Matrix Multiplication
Matrix multiplication is a computationally intensive task that can be significantly sped up using multithreading. Here’s an example of how to implement parallel matrix multiplication:
import threading
import numpy as np
def multiply_row(A, B, result, row):
n = len(B[0])
for j in range(n):
result[row][j] = sum(A[row][k] * B[k][j] for k in range(len(B)))
def parallel_matrix_multiply(A, B):
if len(A[0]) != len(B):
raise ValueError("Matrix dimensions do not match for multiplication")
result = [[0 for _ in range(len(B[0]))] for _ in range(len(A))]
threads = []
for i in range(len(A)):
thread = threading.Thread(target=multiply_row, args=(A, B, result, i))
threads.append(thread)
thread.start()
for thread in threads:
thread.join()
return result
# Example usage
A = [[1, 2, 3], [4, 5, 6]]
B = [[7, 8], [9, 10], [11, 12]]
result = parallel_matrix_multiply(A, B)
print("Resulting matrix:")
for row in result:
print(row)
In this implementation, we create a separate thread for each row of the resulting matrix, allowing for parallel computation of the matrix multiplication.
3. Parallel Graph Traversal
Graph traversal algorithms, such as Breadth-First Search (BFS) or Depth-First Search (DFS), can also benefit from multithreading, especially for large graphs. Here’s an example of a multithreaded BFS implementation:
import threading
from queue import Queue
class Graph:
def __init__(self):
self.graph = {}
self.lock = threading.Lock()
def add_edge(self, u, v):
with self.lock:
if u not in self.graph:
self.graph[u] = []
self.graph[u].append(v)
def bfs_worker(graph, start, visited, queue):
while not queue.empty():
vertex = queue.get()
if vertex not in visited:
print(f"Visited: {vertex}")
visited.add(vertex)
for neighbor in graph.graph.get(vertex, []):
if neighbor not in visited:
queue.put(neighbor)
def parallel_bfs(graph, start):
visited = set()
queue = Queue()
queue.put(start)
num_threads = 4 # You can adjust this based on your system
threads = []
for _ in range(num_threads):
thread = threading.Thread(target=bfs_worker, args=(graph, start, visited, queue))
threads.append(thread)
thread.start()
for thread in threads:
thread.join()
# Example usage
g = Graph()
g.add_edge(0, 1)
g.add_edge(0, 2)
g.add_edge(1, 2)
g.add_edge(2, 0)
g.add_edge(2, 3)
g.add_edge(3, 3)
print("Parallel BFS starting from vertex 2:")
parallel_bfs(g, 2)
This implementation uses multiple threads to process the BFS queue concurrently, potentially speeding up the traversal of large graphs.
Best Practices and Considerations
While implementing multithreading in algorithms can lead to significant performance improvements, it’s essential to keep the following best practices and considerations in mind:
1. Thread Safety
Ensure that shared resources are properly protected using locks or other synchronization mechanisms to prevent race conditions and data corruption.
2. Granularity
Choose the right level of granularity for your multithreaded tasks. Too fine-grained parallelism can lead to overhead from thread creation and management, while too coarse-grained parallelism may not fully utilize available resources.
3. Load Balancing
Try to distribute work evenly among threads to maximize performance gains. Uneven distribution can lead to some threads finishing early while others continue to work, reducing overall efficiency.
4. Scalability
Design your multithreaded algorithms to scale with the number of available cores. This often involves making the number of threads configurable or dynamically adjusting based on system resources.
5. Deadlock Prevention
Be aware of potential deadlock situations when using multiple locks, and implement strategies to prevent or detect deadlocks in your multithreaded algorithms.
6. Testing and Debugging
Thoroughly test your multithreaded implementations, as concurrency issues can be challenging to reproduce and debug. Use tools like thread sanitizers and profilers to identify potential problems.
Advanced Multithreading Concepts
As you become more comfortable with basic multithreading, consider exploring these advanced concepts to further enhance your algorithmic implementations:
1. Thread Pools
Instead of creating new threads for each task, use thread pools to manage a fixed number of worker threads efficiently. Python’s concurrent.futures
module provides a convenient ThreadPoolExecutor for this purpose:
from concurrent.futures import ThreadPoolExecutor
import math
def calculate_prime(n):
if n < 2:
return False
for i in range(2, int(math.sqrt(n)) + 1):
if n % i == 0:
return False
return True
def find_primes(start, end):
with ThreadPoolExecutor() as executor:
numbers = range(start, end + 1)
results = list(executor.map(calculate_prime, numbers))
return [num for num, is_prime in zip(numbers, results) if is_prime]
# Example usage
primes = find_primes(1, 100000)
print(f"Found {len(primes)} prime numbers")
2. Asynchronous Programming
For I/O-bound tasks, consider using asynchronous programming techniques alongside or instead of multithreading. Python’s asyncio
module provides powerful tools for writing asynchronous code:
import asyncio
import aiohttp
async def fetch_url(session, url):
async with session.get(url) as response:
return await response.text()
async def main():
urls = [
"https://api.github.com",
"https://api.github.com/events",
"https://api.github.com/repos/python/cpython",
]
async with aiohttp.ClientSession() as session:
tasks = [fetch_url(session, url) for url in urls]
responses = await asyncio.gather(*tasks)
for url, response in zip(urls, responses):
print(f"Response from {url}: {len(response)} bytes")
asyncio.run(main())
3. Multiprocessing
For CPU-bound tasks, consider using multiprocessing instead of or in addition to multithreading. This allows you to utilize multiple CPU cores more effectively:
import multiprocessing
def cpu_bound_task(n):
return sum(i * i for i in range(n))
def main():
numbers = [10**7, 10**7, 10**7, 10**7]
with multiprocessing.Pool() as pool:
results = pool.map(cpu_bound_task, numbers)
print(f"Results: {results}")
if __name__ == '__main__':
main()
Conclusion
Implementing multithreading in algorithms is a powerful technique for improving performance and efficiency in modern software development. By understanding the basics of multithreading, applying it to common algorithms, and following best practices, you can create more responsive and scalable applications.
As you continue to develop your programming skills, remember that multithreading is just one tool in your algorithmic toolkit. It’s essential to analyze each problem and choose the most appropriate solution, whether it’s multithreading, asynchronous programming, or a single-threaded approach.
Keep practicing and experimenting with multithreaded implementations, and you’ll soon find yourself creating more efficient and powerful algorithms that can tackle even the most complex computational challenges. Happy coding!