Understanding the Knapsack Problem and Solutions
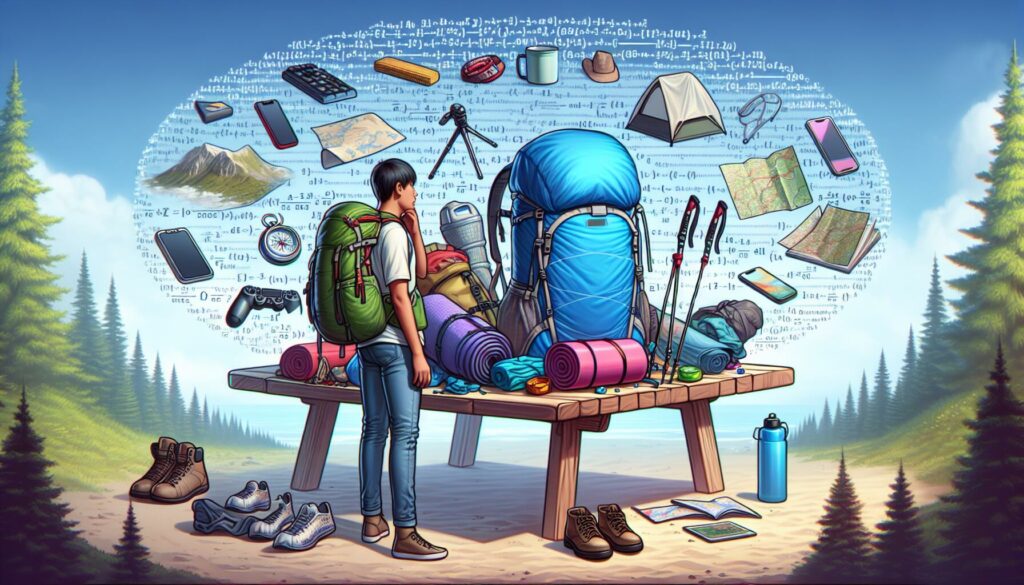
The Knapsack Problem is a classic optimization problem in computer science and mathematics that has wide-ranging applications in various fields, including economics, cryptography, and resource allocation. In this comprehensive guide, we’ll delve deep into the Knapsack Problem, exploring its variants, solutions, and real-world applications. Whether you’re preparing for technical interviews at top tech companies or simply looking to enhance your algorithmic problem-solving skills, understanding the Knapsack Problem is crucial.
What is the Knapsack Problem?
The Knapsack Problem is named after a scenario where a thief has a knapsack (or backpack) with a limited weight capacity and must decide which items to steal to maximize the total value of the loot while staying within the weight constraint. In more formal terms:
Given a set of items, each with a weight and a value, determine the number of each item to include in a collection so that the total weight is less than or equal to a given limit and the total value is as large as possible.
Variants of the Knapsack Problem
There are several variants of the Knapsack Problem, each with its own unique constraints and challenges:
- 0/1 Knapsack Problem: Each item can be included only once (0 or 1 times).
- Bounded Knapsack Problem: Each item can be included up to a specified maximum number of times.
- Unbounded Knapsack Problem: Each item can be included any number of times.
- Fractional Knapsack Problem: Items can be broken into smaller pieces, allowing the thief to take fractions of items.
- Multiple Knapsack Problem: There are multiple knapsacks with different capacities.
In this article, we’ll primarily focus on the 0/1 Knapsack Problem, as it’s the most common variant and forms the basis for understanding the others.
The 0/1 Knapsack Problem: A Closer Look
In the 0/1 Knapsack Problem, we are given:
- A set of n items
- Each item i has a weight w[i] and a value v[i]
- A knapsack with a maximum weight capacity W
The goal is to find a subset of items that maximizes the total value while ensuring that the total weight does not exceed W. Each item can be included only once (hence the name “0/1”).
Example Scenario
Let’s consider a simple example to illustrate the problem:
Items: [A, B, C, D]
Weights: [2, 3, 4, 5]
Values: [3, 4, 5, 6]
Knapsack capacity: 10
In this scenario, we need to choose items that will give us the maximum value without exceeding the weight limit of 10.
Approaches to Solving the Knapsack Problem
There are several approaches to solving the Knapsack Problem, each with its own trade-offs between time complexity and space complexity. Let’s explore the most common methods:
1. Brute Force Approach
The brute force approach involves generating all possible combinations of items and selecting the one with the highest value that doesn’t exceed the weight limit. While this method guarantees finding the optimal solution, it’s highly inefficient for large inputs.
Time Complexity: O(2^n), where n is the number of items
Here’s a simple Python implementation of the brute force approach:
def knapsack_brute_force(values, weights, capacity):
n = len(values)
def knapsack_recursive(i, current_weight, current_value):
if i == n or current_weight > capacity:
return current_value if current_weight <= capacity else 0
# Don't include the item
without_item = knapsack_recursive(i + 1, current_weight, current_value)
# Include the item
with_item = knapsack_recursive(i + 1, current_weight + weights[i], current_value + values[i])
return max(without_item, with_item)
return knapsack_recursive(0, 0, 0)
# Example usage
values = [3, 4, 5, 6]
weights = [2, 3, 4, 5]
capacity = 10
max_value = knapsack_brute_force(values, weights, capacity)
print(f"Maximum value: {max_value}")
While this approach works for small inputs, it becomes impractical for larger datasets due to its exponential time complexity.
2. Dynamic Programming Approach
Dynamic Programming (DP) is a more efficient approach to solving the Knapsack Problem. It breaks down the problem into smaller subproblems and stores their solutions to avoid redundant computations. There are two main ways to implement DP for the Knapsack Problem: bottom-up (tabulation) and top-down (memoization).
Bottom-up Dynamic Programming (Tabulation)
The bottom-up approach builds a table of solutions for subproblems, starting from the smallest and working up to the full problem. Here’s a Python implementation:
def knapsack_dp_bottom_up(values, weights, capacity):
n = len(values)
dp = [[0 for _ in range(capacity + 1)] for _ in range(n + 1)]
for i in range(1, n + 1):
for w in range(1, capacity + 1):
if weights[i-1] <= w:
dp[i][w] = max(values[i-1] + dp[i-1][w-weights[i-1]], dp[i-1][w])
else:
dp[i][w] = dp[i-1][w]
return dp[n][capacity]
# Example usage
values = [3, 4, 5, 6]
weights = [2, 3, 4, 5]
capacity = 10
max_value = knapsack_dp_bottom_up(values, weights, capacity)
print(f"Maximum value: {max_value}")
Time Complexity: O(n * W), where n is the number of items and W is the knapsack capacity
Space Complexity: O(n * W)
Top-down Dynamic Programming (Memoization)
The top-down approach uses recursion with memoization to solve the problem. Here’s a Python implementation:
def knapsack_dp_top_down(values, weights, capacity):
n = len(values)
memo = {}
def knapsack_recursive(i, remaining_capacity):
if i == n or remaining_capacity == 0:
return 0
if (i, remaining_capacity) in memo:
return memo[(i, remaining_capacity)]
if weights[i] > remaining_capacity:
result = knapsack_recursive(i + 1, remaining_capacity)
else:
# Max of including or not including the current item
result = max(
knapsack_recursive(i + 1, remaining_capacity),
values[i] + knapsack_recursive(i + 1, remaining_capacity - weights[i])
)
memo[(i, remaining_capacity)] = result
return result
return knapsack_recursive(0, capacity)
# Example usage
values = [3, 4, 5, 6]
weights = [2, 3, 4, 5]
capacity = 10
max_value = knapsack_dp_top_down(values, weights, capacity)
print(f"Maximum value: {max_value}")
Time Complexity: O(n * W), where n is the number of items and W is the knapsack capacity
Space Complexity: O(n * W)
3. Greedy Approach (for Fractional Knapsack)
While the greedy approach doesn’t work for the 0/1 Knapsack Problem, it’s worth mentioning as it provides an optimal solution for the Fractional Knapsack Problem. In this variant, we can take fractions of items, so we sort the items by their value-to-weight ratio and take as much as possible of the most valuable items first.
def fractional_knapsack(values, weights, capacity):
n = len(values)
# Calculate value-to-weight ratios
ratios = [(v / w, v, w) for v, w in zip(values, weights)]
ratios.sort(reverse=True)
total_value = 0
for ratio, value, weight in ratios:
if capacity == 0:
break
if weight <= capacity:
# Take the whole item
total_value += value
capacity -= weight
else:
# Take a fraction of the item
fraction = capacity / weight
total_value += value * fraction
capacity = 0
return total_value
# Example usage
values = [3, 4, 5, 6]
weights = [2, 3, 4, 5]
capacity = 10
max_value = fractional_knapsack(values, weights, capacity)
print(f"Maximum value: {max_value}")
Time Complexity: O(n log n) due to sorting
Space Complexity: O(n)
Advanced Techniques and Optimizations
While the dynamic programming approach is efficient for most practical purposes, there are advanced techniques and optimizations that can further improve the performance of Knapsack Problem solutions:
1. Branch and Bound
Branch and Bound is an algorithmic paradigm that systematically enumerates candidate solutions by means of state space search. It can be more efficient than dynamic programming for some instances of the Knapsack Problem, especially when the optimal solution can be found quickly.
2. Meet in the Middle
This technique splits the input into two halves, solves each half separately, and then combines the results. It can be particularly effective when the number of items is relatively small but the knapsack capacity is large.
3. Bit Manipulation
For small to medium-sized inputs, bit manipulation techniques can be used to optimize the space complexity of the dynamic programming solution.
4. Approximation Algorithms
For very large instances where exact solutions are impractical, approximation algorithms can provide near-optimal solutions in polynomial time.
Real-world Applications of the Knapsack Problem
The Knapsack Problem and its variants have numerous real-world applications across various domains:
1. Finance and Investment
- Portfolio Optimization: Selecting a mix of investments that maximizes return while adhering to budget constraints.
- Capital Budgeting: Choosing which projects to invest in given limited resources.
2. Logistics and Transportation
- Cargo Loading: Optimizing the loading of cargo into vehicles or containers.
- Airline Cargo Management: Determining which items to load onto an aircraft to maximize profit while staying within weight limits.
3. Resource Allocation
- Computing Resources: Allocating CPU time or memory to various processes.
- Project Management: Selecting which projects to undertake given limited time and budget.
4. Cryptography
- Subset Sum Problem: A variant of the Knapsack Problem used in certain cryptographic systems.
5. Industrial Manufacturing
- Cutting Stock Problem: Minimizing waste when cutting larger sheets of material into smaller pieces.
Preparing for Technical Interviews
The Knapsack Problem is a popular topic in technical interviews, especially for positions at top tech companies. Here are some tips for preparing:
- Understand the variants: Be familiar with the different types of Knapsack Problems and their characteristics.
- Master the DP approach: Both bottom-up and top-down dynamic programming solutions are essential.
- Practice implementation: Implement the solutions in your preferred programming language, focusing on clean and efficient code.
- Analyze time and space complexity: Be prepared to discuss the time and space complexity of your solutions.
- Consider edge cases: Think about and handle edge cases, such as empty input or zero capacity.
- Optimize: Look for opportunities to optimize your solutions, such as reducing space complexity.
- Relate to real-world scenarios: Be ready to discuss how the Knapsack Problem relates to real-world applications.
Conclusion
The Knapsack Problem is a fundamental concept in computer science and optimization. Its various forms and solutions provide a rich ground for developing algorithmic thinking and problem-solving skills. By understanding the Knapsack Problem and its applications, you’ll be better equipped to tackle a wide range of optimization challenges in both technical interviews and real-world scenarios.
As you continue your journey in coding education and skill development, remember that mastering problems like the Knapsack Problem is not just about memorizing solutions. It’s about developing a deeper understanding of algorithmic patterns and the ability to apply them creatively to new challenges. Keep practicing, exploring different variants, and challenging yourself with increasingly complex scenarios to truly internalize these concepts and become a more proficient problem solver.