The Mathematics of Algorithm Analysis: Unraveling Complexity
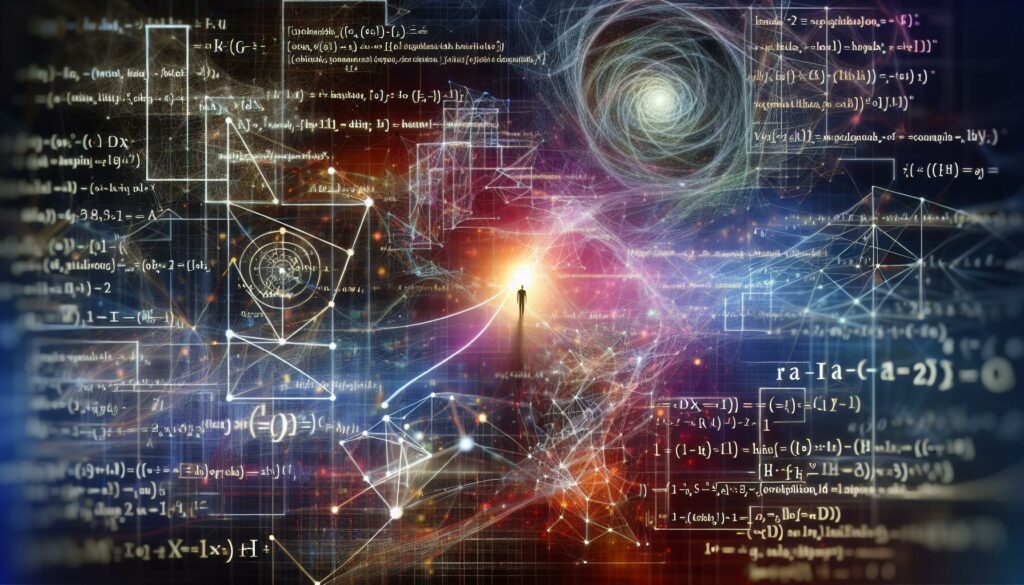
In the world of computer science and programming, algorithms are the backbone of efficient problem-solving. But how do we determine which algorithm is better? How can we predict an algorithm’s performance as the input size grows? This is where the mathematics of algorithm analysis comes into play. In this comprehensive guide, we’ll dive deep into the fundamental concepts, techniques, and importance of algorithm analysis, equipping you with the tools to become a more effective programmer and problem-solver.
1. Introduction to Algorithm Analysis
Algorithm analysis is the process of evaluating the efficiency and performance of algorithms. It helps us understand how an algorithm’s runtime and memory usage scale with increasing input sizes. This knowledge is crucial for several reasons:
- Choosing the most appropriate algorithm for a given problem
- Predicting an algorithm’s behavior for large-scale applications
- Optimizing existing algorithms and code
- Designing scalable software systems
The primary focus of algorithm analysis is on two key aspects:
- Time Complexity: How the runtime of an algorithm grows with input size
- Space Complexity: How the memory usage of an algorithm grows with input size
2. Asymptotic Notation
To describe the growth rate of algorithms, we use asymptotic notation. The three most common forms are:
2.1 Big O Notation (O)
Big O notation provides an upper bound on the growth rate of an algorithm. It represents the worst-case scenario and is the most commonly used notation in algorithm analysis.
For example, if an algorithm has a time complexity of O(n²), it means that the runtime grows no faster than n² as the input size (n) increases.
2.2 Omega Notation (Ω)
Omega notation provides a lower bound on the growth rate of an algorithm. It represents the best-case scenario.
For instance, Ω(n) means that the algorithm’s runtime grows at least as fast as n.
2.3 Theta Notation (Θ)
Theta notation provides both upper and lower bounds on the growth rate of an algorithm. It represents the average-case scenario and is used when the upper and lower bounds are the same.
For example, Θ(n log n) means that the algorithm’s runtime grows exactly at the rate of n log n.
3. Common Time Complexities
Let’s explore some of the most common time complexities you’ll encounter in algorithm analysis:
3.1 Constant Time: O(1)
Algorithms with constant time complexity perform the same number of operations regardless of input size. Examples include:
- Accessing an array element by index
- Performing basic arithmetic operations
3.2 Linear Time: O(n)
Linear time algorithms have a runtime that grows linearly with the input size. Examples include:
- Iterating through an array once
- Linear search in an unsorted array
3.3 Logarithmic Time: O(log n)
Logarithmic time algorithms have a runtime that grows logarithmically with the input size. Examples include:
- Binary search in a sorted array
- Certain divide-and-conquer algorithms
3.4 Linearithmic Time: O(n log n)
Linearithmic time algorithms combine linear and logarithmic growth. Examples include:
- Efficient sorting algorithms like Merge Sort and Quick Sort
- Certain divide-and-conquer algorithms
3.5 Quadratic Time: O(n²)
Quadratic time algorithms have a runtime that grows quadratically with the input size. Examples include:
- Nested loops iterating over the input
- Simple sorting algorithms like Bubble Sort and Selection Sort
3.6 Exponential Time: O(2^n)
Exponential time algorithms have a runtime that doubles with each additional input element. Examples include:
- Recursive algorithms for solving the Traveling Salesman Problem
- Brute-force solutions to NP-complete problems
4. Analyzing Algorithms: Step-by-Step
Now that we understand the basics of asymptotic notation and common time complexities, let’s walk through the process of analyzing an algorithm:
4.1 Identify the Input
Determine what constitutes the input to your algorithm and how it affects the number of operations performed.
4.2 Count the Operations
Identify the basic operations in your algorithm (e.g., comparisons, arithmetic operations) and count how many times they are executed.
4.3 Express the Count as a Function
Write an expression that relates the number of operations to the input size.
4.4 Simplify and Determine the Dominant Term
Simplify the expression and focus on the term that grows the fastest as the input size increases.
4.5 Express in Asymptotic Notation
Use the appropriate asymptotic notation (usually Big O) to describe the simplified expression.
Example: Analyzing a Simple Algorithm
Let’s analyze the following algorithm that finds the maximum element in an array:
function findMax(arr):
max = arr[0]
for i from 1 to arr.length - 1:
if arr[i] > max:
max = arr[i]
return max
Analysis:
- Input: An array of n elements
- Operations: One comparison and potential assignment per iteration
- Count: n – 1 iterations (we start from index 1)
- Expression: T(n) = n – 1
- Simplification: The dominant term is n
- Asymptotic notation: O(n) – linear time complexity
5. Recurrence Relations and Master Theorem
When analyzing recursive algorithms, we often encounter recurrence relations. These are equations that describe the runtime of an algorithm in terms of its input size and the runtime of smaller subproblems.
The Master Theorem is a powerful tool for solving many common recurrence relations. It provides a systematic way to analyze divide-and-conquer algorithms.
5.1 The Master Theorem
For a recurrence relation of the form:
T(n) = aT(n/b) + f(n)
Where:
- a ≥ 1 (number of subproblems)
- b > 1 (factor by which subproblem size is reduced)
- f(n) is a polynomial function
The Master Theorem states that:
- If f(n) = O(n^(log_b(a) – ε)) for some ε > 0, then T(n) = Θ(n^(log_b(a)))
- If f(n) = Θ(n^(log_b(a))), then T(n) = Θ(n^(log_b(a)) * log n)
- If f(n) = Ω(n^(log_b(a) + ε)) for some ε > 0, and af(n/b) ≤ cf(n) for some c
Example: Analyzing Merge Sort
Let’s apply the Master Theorem to analyze the time complexity of Merge Sort:
Recurrence relation for Merge Sort: T(n) = 2T(n/2) + Θ(n)
Here, a = 2, b = 2, and f(n) = Θ(n)
We can see that f(n) = Θ(n^(log_2(2))) = Θ(n)
This falls under case 2 of the Master Theorem, so:
T(n) = Θ(n^(log_2(2)) * log n) = Θ(n log n)
Therefore, Merge Sort has a time complexity of Θ(n log n).
6. Space Complexity Analysis
While time complexity is often the primary focus, space complexity is equally important, especially in memory-constrained environments. Space complexity analysis follows similar principles to time complexity analysis but focuses on memory usage instead of runtime.
6.1 Types of Space Usage
- Input Space: Memory used to store the input
- Auxiliary Space: Extra memory used by the algorithm (excluding input space)
- Total Space: Sum of input space and auxiliary space
6.2 Common Space Complexities
- O(1): Constant space (e.g., using a fixed number of variables)
- O(n): Linear space (e.g., creating an array of size n)
- O(n²): Quadratic space (e.g., creating a 2D array of size n x n)
- O(log n): Logarithmic space (e.g., recursive algorithms with log n depth)
Example: Analyzing Space Complexity
Let’s analyze the space complexity of a recursive fibonacci function:
function fibonacci(n):
if n <= 1:
return n
return fibonacci(n-1) + fibonacci(n-2)
Analysis:
- Input Space: O(1) – we only store the input n
- Auxiliary Space: O(n) – the recursive call stack can go up to n levels deep
- Total Space: O(n)
7. Amortized Analysis
Amortized analysis is a method for analyzing algorithms that perform a sequence of operations. It provides a way to understand the average performance of an algorithm over a worst-case sequence of operations, rather than focusing on the worst-case cost of a single operation.
7.1 Techniques for Amortized Analysis
- Aggregate Method: Compute the total cost of a sequence of operations and divide by the number of operations.
- Accounting Method: Assign different charges to different operations, some higher and some lower than their actual cost.
- Potential Method: Define a potential function that captures the state of the data structure and analyze how it changes with each operation.
Example: Amortized Analysis of Dynamic Array
Consider a dynamic array that doubles in size when it’s full. While a single resize operation takes O(n) time, we can show that the amortized cost per insertion is O(1).
Using the aggregate method:
- Start with an array of size 1
- For n insertions, we’ll have resizes at sizes 1, 2, 4, 8, …, up to the nearest power of 2 less than or equal to n
- Total cost = n + (1 + 2 + 4 + 8 + … + nearest power of 2 ≤ n) ≤ n + 2n = 3n
- Amortized cost per operation = 3n / n = O(1)
8. NP-Completeness and Computational Complexity Classes
As we delve deeper into algorithm analysis, it’s important to understand the concept of computational complexity classes, particularly NP-completeness.
8.1 Complexity Classes
- P: Problems solvable in polynomial time by a deterministic Turing machine
- NP: Problems verifiable in polynomial time by a deterministic Turing machine
- NP-Complete: The hardest problems in NP, to which all other NP problems can be reduced in polynomial time
- NP-Hard: Problems at least as hard as NP-Complete problems, but not necessarily in NP
8.2 Importance of NP-Completeness
Understanding NP-completeness is crucial because:
- It helps identify problems that are likely to be computationally intractable
- It guides algorithm design towards approximation algorithms or heuristics for NP-complete problems
- It has implications for cryptography and security, as many cryptographic systems rely on the assumed difficulty of certain NP-complete problems
9. Practical Applications of Algorithm Analysis
Algorithm analysis isn’t just a theoretical exercise. It has numerous practical applications in software development and system design:
9.1 Optimizing Database Queries
Understanding the time complexity of different query operations helps in designing efficient database schemas and writing optimized queries.
9.2 Scaling Web Applications
Analyzing the complexity of key algorithms in web applications helps predict how they’ll perform under increased load and guides optimization efforts.
9.3 Machine Learning Model Selection
Different machine learning algorithms have different time and space complexities. Algorithm analysis helps in choosing the most appropriate model for a given dataset and computational resources.
9.4 Network Protocol Design
Analyzing the complexity of network protocols helps in designing efficient communication systems that can handle large numbers of nodes and high traffic volumes.
10. Tools and Techniques for Algorithm Analysis
While manual analysis is crucial for understanding algorithms, there are also tools and techniques that can aid in the process:
10.1 Profiling Tools
Profilers like gprof (for C/C++), cProfile (for Python), or built-in profilers in IDEs can help measure the actual runtime of algorithms and identify bottlenecks.
10.2 Visualization Tools
Tools like Algorithm Visualizer or VisuAlgo can help visualize how algorithms work, making it easier to understand their behavior and complexity.
10.3 Benchmarking Frameworks
Frameworks like Google Benchmark (C++) or timeit (Python) allow for systematic measurement of algorithm performance across different inputs.
10.4 Asymptotic Analysis Libraries
Libraries like Big-O (JavaScript) or scipy.stats (Python) can help with fitting empirical data to asymptotic functions.
Conclusion
The mathematics of algorithm analysis is a fundamental skill for any serious programmer or computer scientist. It provides the tools to understand, compare, and optimize algorithms, enabling the development of efficient and scalable software systems.
By mastering concepts like asymptotic notation, recurrence relations, and complexity classes, you’ll be better equipped to:
- Choose the right algorithm for a given problem
- Optimize existing code and systems
- Design scalable software architectures
- Tackle complex computational problems
- Prepare for technical interviews at top tech companies
Remember, algorithm analysis is not just about memorizing Big O notations. It’s about developing a deep understanding of how algorithms behave as inputs grow, and how to apply this knowledge to real-world problems. As you continue your journey in programming and computer science, make algorithm analysis a regular part of your problem-solving toolkit. With practice and application, you’ll find it an invaluable skill in your quest to become a more effective and efficient programmer.