Introduction to Ant Colony Optimization Algorithms: Nature-Inspired Problem Solving
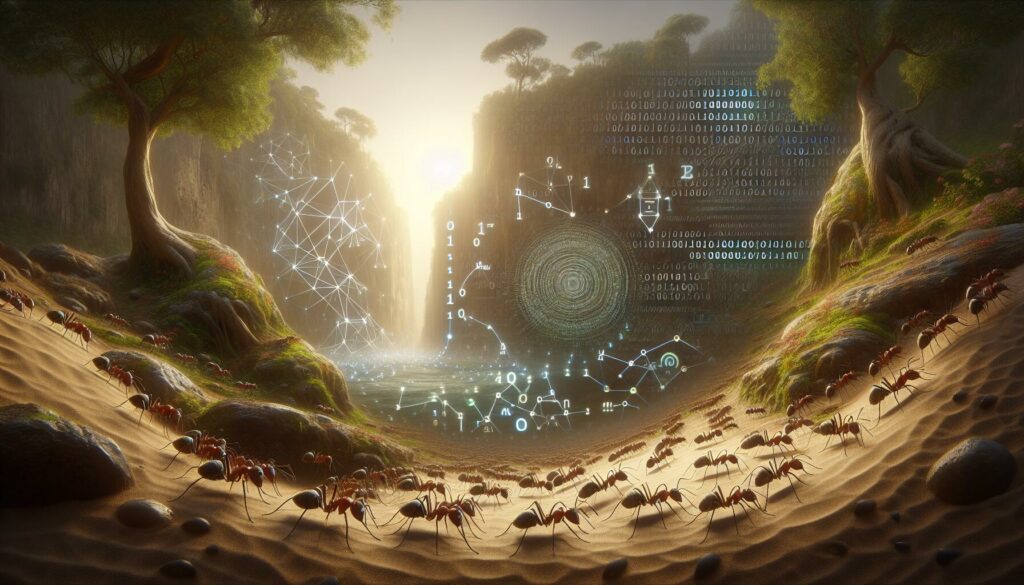
In the vast world of algorithms and computational problem-solving, nature has often been a source of inspiration. One such fascinating example is the Ant Colony Optimization (ACO) algorithm, which draws its inspiration from the foraging behavior of ants. This powerful optimization technique has found applications in various fields, from logistics and telecommunications to bioinformatics and machine learning. In this comprehensive guide, we’ll delve deep into the world of Ant Colony Optimization algorithms, exploring their origins, mechanics, applications, and implementation.
What is Ant Colony Optimization?
Ant Colony Optimization is a probabilistic technique for solving computational problems that can be reduced to finding good paths through graphs. Developed by Marco Dorigo in the early 1990s, ACO algorithms are inspired by the behavior of ants searching for food. These algorithms belong to the class of swarm intelligence methods, which focus on the collective behavior of decentralized, self-organized systems.
The key idea behind ACO is that ants, despite their simple individual behavior, can collectively solve complex problems through indirect communication. This communication happens through pheromones, chemical substances that ants deposit on the ground as they move. Other ants can detect these pheromones and are more likely to follow paths with higher pheromone concentrations.
The Natural Inspiration: How Ants Find Optimal Paths
To understand ACO better, let’s first look at how ants behave in nature:
- Ants leave the nest in search of food, initially moving in random directions.
- As they move, they deposit pheromones on the ground, creating a trail.
- When an ant finds food, it returns to the nest, reinforcing the pheromone trail on its way back.
- Other ants are more likely to follow paths with stronger pheromone concentrations.
- Shorter paths will be traversed more quickly, leading to more pheromone deposits over time.
- Pheromones evaporate over time, reducing the attractiveness of longer or less optimal paths.
Through this simple mechanism, ants can collectively find the shortest path between their nest and a food source, even in the presence of obstacles.
The ACO Algorithm: Translating Nature to Computation
The Ant Colony Optimization algorithm translates this natural behavior into a computational method for solving optimization problems. Here’s a high-level overview of how ACO works:
- Problem Representation: The problem is represented as a graph where the edges represent possible paths and the vertices represent decision points.
- Initialization: Artificial ants are placed on the graph, and pheromone levels are initialized on all edges.
- Solution Construction: Each ant constructs a solution by moving from vertex to vertex, making probabilistic decisions based on pheromone levels and heuristic information.
- Pheromone Update: After all ants have constructed their solutions, the pheromone levels on the edges are updated. Edges that are part of better solutions receive more pheromones.
- Pheromone Evaporation: A portion of the pheromones evaporates from all edges, allowing the algorithm to forget poor solutions over time.
- Iteration: Steps 3-5 are repeated for a specified number of iterations or until a termination condition is met.
Key Components of ACO Algorithms
To implement an effective ACO algorithm, several key components need to be defined:
1. Pheromone Representation
Pheromones are typically represented as numerical values associated with each edge of the graph. These values are updated throughout the algorithm’s execution.
2. Heuristic Information
In addition to pheromones, ACO algorithms often use problem-specific heuristic information to guide the ants. This could be, for example, the distance between cities in a traveling salesman problem.
3. Probabilistic Decision Rule
Ants make decisions probabilistically based on both pheromone levels and heuristic information. The probability of an ant choosing a particular edge is often calculated using the following formula:
P(i,j) = (τ[i,j]^α * η[i,j]^β) / Σ (τ[i,k]^α * η[i,k]^β)
Where:
- P(i,j) is the probability of moving from node i to node j
- Ï„[i,j] is the pheromone level on edge (i,j)
- η[i,j] is the heuristic information for edge (i,j)
- α and β are parameters that control the relative importance of pheromone versus heuristic information
4. Pheromone Update Rule
After each iteration, pheromone levels are updated. This typically involves two steps:
- Evaporation: A fraction of pheromone evaporates from all edges.
- Deposition: Ants deposit pheromones on the edges they traversed, with the amount dependent on the quality of their solution.
A common pheromone update rule is:
Ï„[i,j] = (1 - Ï) * Ï„[i,j] + Σ Δτ[i,j]^k
Where:
- Ï is the evaporation rate
- Δτ[i,j]^k is the amount of pheromone deposited by ant k on edge (i,j)
Variants of ACO Algorithms
Since its inception, several variants of the ACO algorithm have been developed to improve performance or adapt to specific problem domains:
1. Ant System (AS)
The original ACO algorithm proposed by Dorigo. It forms the basis for most other ACO variants.
2. Elitist Ant System (EAS)
This variant gives extra weight to the best-so-far solution when updating pheromones, helping to intensify the search around good solutions.
3. Rank-Based Ant System (ASrank)
In this variant, ants are ranked according to their solution quality, and pheromone updates are weighted based on this ranking.
4. Max-Min Ant System (MMAS)
MMAS introduces upper and lower bounds on pheromone levels to prevent premature convergence to suboptimal solutions.
5. Ant Colony System (ACS)
ACS introduces a local pheromone update rule applied while ants construct their solutions, in addition to the global update rule.
Applications of Ant Colony Optimization
ACO algorithms have found applications in a wide range of fields due to their ability to solve complex optimization problems. Some notable applications include:
1. Traveling Salesman Problem (TSP)
One of the most common applications of ACO, where the goal is to find the shortest possible route that visits each city exactly once and returns to the starting city.
2. Vehicle Routing Problems
ACO has been successfully applied to various vehicle routing problems, including those with time windows, multiple depots, and pickup and delivery constraints.
3. Job Shop Scheduling
ACO algorithms have been used to optimize job scheduling in manufacturing environments, minimizing makespan and improving resource utilization.
4. Network Routing
In telecommunications, ACO has been applied to routing problems in both wired and wireless networks, optimizing for factors like latency, bandwidth, and load balancing.
5. Image Processing
ACO techniques have been used in image processing tasks such as edge detection, image segmentation, and feature selection.
6. Bioinformatics
In the field of bioinformatics, ACO has been applied to problems like protein folding, DNA sequencing, and phylogenetic tree construction.
Implementing ACO: A Simple Example
To illustrate how ACO works in practice, let’s implement a simple version of the algorithm to solve the Traveling Salesman Problem. We’ll use Python for this example.
import numpy as np
class AntColonyOptimizer:
def __init__(self, distances, n_ants, n_iterations, alpha, beta, rho, q):
self.distances = distances
self.n_ants = n_ants
self.n_iterations = n_iterations
self.alpha = alpha # pheromone importance
self.beta = beta # distance importance
self.rho = rho # pheromone evaporation rate
self.q = q # pheromone deposit factor
self.n_cities = len(distances)
self.pheromones = np.ones((self.n_cities, self.n_cities))
def run(self):
best_path = None
best_path_length = float('inf')
for _ in range(self.n_iterations):
paths = self.construct_solutions()
self.update_pheromones(paths)
iteration_best_path = min(paths, key=lambda x: self.path_length(x))
iteration_best_path_length = self.path_length(iteration_best_path)
if iteration_best_path_length < best_path_length:
best_path = iteration_best_path
best_path_length = iteration_best_path_length
return best_path, best_path_length
def construct_solutions(self):
paths = []
for _ in range(self.n_ants):
path = self.construct_path()
paths.append(path)
return paths
def construct_path(self):
path = [np.random.randint(self.n_cities)]
while len(path) < self.n_cities:
current_city = path[-1]
unvisited = list(set(range(self.n_cities)) - set(path))
probabilities = self.calculate_probabilities(current_city, unvisited)
next_city = np.random.choice(unvisited, p=probabilities)
path.append(next_city)
return path
def calculate_probabilities(self, current_city, unvisited):
pheromone = np.array([self.pheromones[current_city][j] for j in unvisited])
distance = np.array([1 / self.distances[current_city][j] for j in unvisited])
probabilities = (pheromone ** self.alpha) * (distance ** self.beta)
return probabilities / sum(probabilities)
def update_pheromones(self, paths):
self.pheromones *= (1 - self.rho)
for path in paths:
path_length = self.path_length(path)
for i in range(len(path)):
from_city, to_city = path[i], path[(i + 1) % len(path)]
self.pheromones[from_city][to_city] += self.q / path_length
def path_length(self, path):
return sum(self.distances[path[i]][path[(i + 1) % len(path)]] for i in range(len(path)))
# Example usage
distances = np.array([
[0, 2, 3, 4],
[2, 0, 5, 3],
[3, 5, 0, 1],
[4, 3, 1, 0]
])
aco = AntColonyOptimizer(distances, n_ants=5, n_iterations=100, alpha=1, beta=2, rho=0.1, q=1)
best_path, best_path_length = aco.run()
print(f"Best path: {best_path}")
print(f"Best path length: {best_path_length}")
This implementation includes the core components of an ACO algorithm:
- Initialization of pheromones
- Construction of solutions by ants
- Probabilistic decision-making based on pheromones and heuristic information
- Pheromone update rule with evaporation and deposition
Advantages and Limitations of ACO
Like any algorithm, ACO has its strengths and weaknesses. Understanding these can help in deciding when to apply ACO to a problem.
Advantages:
- Adaptability: ACO can adapt to changes in the environment, making it suitable for dynamic problems.
- Parallelism: The algorithm is inherently parallel, as each ant constructs its solution independently.
- Versatility: ACO can be applied to a wide range of optimization problems.
- Robustness: The algorithm is less likely to get stuck in local optima compared to some other optimization techniques.
Limitations:
- Parameter Tuning: ACO algorithms often require careful tuning of parameters for optimal performance.
- Convergence Time: For large problems, ACO may require a significant number of iterations to converge to a good solution.
- Theoretical Analysis: The theoretical foundations of ACO are less developed compared to some other optimization techniques.
- Memory Requirements: Storing pheromone information for large problems can be memory-intensive.
Future Directions and Research in ACO
As ACO continues to evolve, several areas of research and development are being pursued:
1. Hybridization
Combining ACO with other optimization techniques or machine learning algorithms to create more powerful hybrid approaches.
2. Multi-objective Optimization
Extending ACO to handle problems with multiple, potentially conflicting objectives.
3. Dynamic and Stochastic Problems
Adapting ACO to better handle problems where the environment or constraints change over time or involve uncertainty.
4. Theoretical Foundations
Developing stronger theoretical foundations for ACO, including convergence proofs and performance guarantees.
5. Parameter Adaptation
Creating methods for automatic parameter tuning and adaptation during the algorithm’s execution.
6. Large-scale Problems
Scaling ACO to efficiently handle very large problem instances, potentially through distributed or parallel implementations.
Conclusion
Ant Colony Optimization algorithms represent a fascinating intersection of nature-inspired computing and practical problem-solving. By mimicking the collective behavior of ants, these algorithms can tackle complex optimization problems in a wide range of fields. While ACO has already proven its worth in many applications, ongoing research continues to expand its capabilities and effectiveness.
As we’ve explored in this introduction, understanding ACO involves grasping its biological inspiration, core components, and various implementations. Whether you’re a computer science student, a researcher, or a practitioner in fields like logistics or telecommunications, ACO offers a powerful tool for approaching optimization problems.
The journey into Ant Colony Optimization is not just about learning an algorithm; it’s about appreciating the ingenuity of nature and how it can inspire computational approaches to solving complex problems. As you delve deeper into ACO and related swarm intelligence techniques, you’ll find a rich field of study with numerous opportunities for innovation and application.
Remember, the key to mastering ACO lies not just in understanding its mechanics, but in practicing its implementation, experimenting with different variants, and applying it to real-world problems. Happy optimizing!