Implementing Flood Fill Algorithms: A Comprehensive Guide
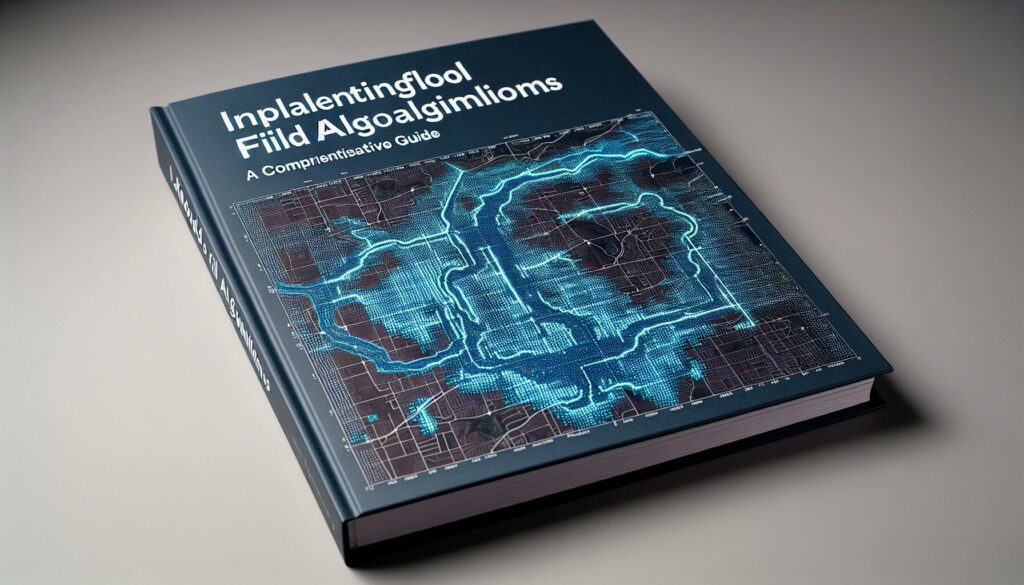
Welcome to our in-depth exploration of flood fill algorithms! If you’ve ever used the “paint bucket” tool in image editing software or played Minesweeper, you’ve interacted with flood fill algorithms. These powerful algorithms are essential in various applications, from image processing to game development. In this article, we’ll dive deep into the world of flood fill algorithms, their implementations, and their practical applications.
Table of Contents
- Introduction to Flood Fill Algorithms
- How Flood Fill Works
- Implementations of Flood Fill
- Optimizations and Variations
- Real-world Applications
- Common Challenges and Solutions
- Conclusion
1. Introduction to Flood Fill Algorithms
Flood fill is a fundamental algorithm used to determine and modify connected regions in a multi-dimensional array. It’s called “flood fill” because it spreads like a flood, filling all connected cells that meet a certain criterion. The algorithm starts from a given cell (seed point) and progressively “fills” neighboring cells that share a specific property, such as color or value.
Key concepts to understand about flood fill algorithms include:
- Connectivity: Defines how cells are considered adjacent (4-way or 8-way in 2D grids).
- Boundary conditions: Criteria that stop the fill from spreading further.
- Fill criteria: The condition that determines whether a cell should be filled.
2. How Flood Fill Works
The basic principle of flood fill is straightforward:
- Start at a given cell (seed point).
- Check if the current cell meets the fill criteria.
- If it does, mark it as filled and check its neighbors.
- Repeat steps 2-3 for each valid neighbor until no more cells can be filled.
This process creates a “flood” effect, spreading outwards from the starting point until it reaches boundaries or cells that don’t meet the fill criteria.
3. Implementations of Flood Fill
There are several ways to implement flood fill algorithms. We’ll explore three common approaches: recursive, iterative using a stack, and iterative using a queue.
3.1 Recursive Approach
The recursive approach is the most intuitive implementation of flood fill. Here’s a Python example:
def flood_fill(image, sr, sc, new_color):
rows, cols = len(image), len(image[0])
original_color = image[sr][sc]
if original_color == new_color:
return image
def dfs(r, c):
if (r < 0 or r >= rows or c < 0 or c >= cols or
image[r][c] != original_color):
return
image[r][c] = new_color
dfs(r+1, c)
dfs(r-1, c)
dfs(r, c+1)
dfs(r, c-1)
dfs(sr, sc)
return image
This recursive approach uses depth-first search (DFS) to explore and fill connected cells. While simple to understand and implement, it can lead to stack overflow errors for large images or deep recursions.
3.2 Iterative Approach using Stack
To avoid the potential stack overflow issues of the recursive approach, we can use an iterative method with a stack:
def flood_fill_stack(image, sr, sc, new_color):
rows, cols = len(image), len(image[0])
original_color = image[sr][sc]
if original_color == new_color:
return image
stack = [(sr, sc)]
while stack:
r, c = stack.pop()
if (0 <= r < rows and 0 <= c < cols and
image[r][c] == original_color):
image[r][c] = new_color
stack.extend([(r+1, c), (r-1, c), (r, c+1), (r, c-1)])
return image
This implementation uses a stack to keep track of cells to be processed, mimicking the call stack of the recursive approach but with better control over memory usage.
3.3 Iterative Approach using Queue
Another iterative approach uses a queue, which implements a breadth-first search (BFS) strategy:
from collections import deque
def flood_fill_queue(image, sr, sc, new_color):
rows, cols = len(image), len(image[0])
original_color = image[sr][sc]
if original_color == new_color:
return image
queue = deque([(sr, sc)])
while queue:
r, c = queue.popleft()
if (0 <= r < rows and 0 <= c < cols and
image[r][c] == original_color):
image[r][c] = new_color
queue.extend([(r+1, c), (r-1, c), (r, c+1), (r, c-1)])
return image
This queue-based approach processes cells in a breadth-first manner, which can be more efficient for certain types of images or fill patterns.
4. Optimizations and Variations
While the basic flood fill algorithm is powerful, there are several optimizations and variations to consider:
4.1 Scanline Flood Fill
Scanline flood fill is an optimization that reduces the number of cells pushed onto the stack or queue. It works by filling horizontal lines (scanlines) and only pushing the endpoints of unfilled neighboring scanlines.
def scanline_flood_fill(image, x, y, new_color):
rows, cols = len(image), len(image[0])
original_color = image[y][x]
if original_color == new_color:
return image
stack = [(x, y)]
while stack:
x, y = stack.pop()
# Find the leftmost and rightmost pixels to fill on this scanline
left_x = x
while left_x > 0 and image[y][left_x - 1] == original_color:
left_x -= 1
right_x = x
while right_x < cols - 1 and image[y][right_x + 1] == original_color:
right_x += 1
# Fill the scanline
for fill_x in range(left_x, right_x + 1):
image[y][fill_x] = new_color
# Check scanlines above and below
if y > 0:
stack.extend((x, y - 1) for x in range(left_x, right_x + 1)
if image[y - 1][x] == original_color)
if y < rows - 1:
stack.extend((x, y + 1) for x in range(left_x, right_x + 1)
if image[y + 1][x] == original_color)
return image
This optimization can significantly reduce the number of individual cell checks and stack/queue operations, especially for large areas of uniform color.
4.2 Boundary Fill
Boundary fill is a variation of flood fill that fills an area until it reaches a specified boundary color, rather than filling based on a matching color:
def boundary_fill(image, x, y, fill_color, boundary_color):
if (x < 0 or x >= len(image[0]) or y < 0 or y >= len(image) or
image[y][x] == boundary_color or image[y][x] == fill_color):
return
image[y][x] = fill_color
boundary_fill(image, x + 1, y, fill_color, boundary_color)
boundary_fill(image, x - 1, y, fill_color, boundary_color)
boundary_fill(image, x, y + 1, fill_color, boundary_color)
boundary_fill(image, x, y - 1, fill_color, boundary_color)
This variation is particularly useful in scenarios where you want to fill an enclosed area defined by a specific boundary color.
4.3 Multi-threading and Parallel Processing
For very large images or grids, you can implement parallel flood fill algorithms that divide the image into sections and process them concurrently. This approach can significantly speed up the fill process on multi-core systems.
5. Real-world Applications
Flood fill algorithms have numerous practical applications across various fields:
5.1 Image Processing
- Paint bucket tool: In image editing software to fill areas with color.
- Image segmentation: To identify and isolate objects or regions in images.
- Noise removal: To clean up small artifacts in images.
5.2 Game Development
- Map generation: Creating connected regions in procedurally generated maps.
- Path finding: Identifying traversable areas in game worlds.
- Gameplay mechanics: Implementing game logic in titles like Minesweeper or Go.
5.3 Computer Graphics
- Polygon filling: Efficiently filling complex shapes in rendering pipelines.
- Texture synthesis: Generating seamless textures by propagating patterns.
5.4 Geographic Information Systems (GIS)
- Terrain analysis: Identifying connected regions with similar elevation or characteristics.
- Flood modeling: Simulating the spread of water in flood prediction systems.
6. Common Challenges and Solutions
While implementing flood fill algorithms, you may encounter several challenges. Here are some common issues and their solutions:
6.1 Stack Overflow in Recursive Implementations
Problem: For large areas or deep recursions, the recursive approach can lead to stack overflow errors.
Solution: Use iterative implementations with a stack or queue, or implement tail-call optimization if your language supports it.
6.2 Performance Issues with Large Datasets
Problem: Basic flood fill can be slow for very large images or grids.
Solution: Implement optimizations like scanline fill, use more efficient data structures, or consider parallel processing for large datasets.
6.3 Handling Different Connectivity Types
Problem: Different applications may require 4-way or 8-way connectivity.
Solution: Parameterize the neighbor checking function to support different connectivity types:
def get_neighbors(x, y, connectivity):
if connectivity == 4:
return [(x+1, y), (x-1, y), (x, y+1), (x, y-1)]
elif connectivity == 8:
return [(x+1, y), (x-1, y), (x, y+1), (x, y-1),
(x+1, y+1), (x+1, y-1), (x-1, y+1), (x-1, y-1)]
else:
raise ValueError("Invalid connectivity type")
6.4 Dealing with Floating Point Precision
Problem: When working with floating-point values, exact equality checks can lead to issues due to precision limitations.
Solution: Use an epsilon value for approximate equality checks:
def is_similar_color(color1, color2, epsilon=1e-6):
return all(abs(c1 - c2) < epsilon for c1, c2 in zip(color1, color2))
6.5 Memory Management in Large Datasets
Problem: For very large datasets, storing the entire grid in memory may not be feasible.
Solution: Implement chunking strategies to process the data in manageable portions, or use memory-mapped files for efficient access to large datasets.
7. Conclusion
Flood fill algorithms are a powerful tool in a programmer’s arsenal, with applications spanning from simple image editing to complex geographical analysis. By understanding the core principles and various implementations of flood fill, you can tackle a wide range of problems efficiently.
As you’ve seen, there are multiple ways to implement flood fill, each with its own strengths and potential optimizations. The choice between recursive and iterative approaches, as well as specific optimizations like scanline fill, depends on the particular requirements of your application and the characteristics of your data.
Remember that while flood fill is conceptually simple, its efficient implementation can require careful consideration of factors like memory usage, performance optimization, and edge cases. As you apply flood fill algorithms in your projects, continually assess and refine your approach to ensure it meets the specific needs of your application.
Whether you’re developing the next big image editing tool, creating innovative game mechanics, or analyzing complex geographical data, mastering flood fill algorithms will undoubtedly enhance your problem-solving toolkit and open up new possibilities in your programming journey.
Happy coding, and may your flood fills always find their boundaries!