Understanding Computational Geometry Algorithms: A Comprehensive Guide
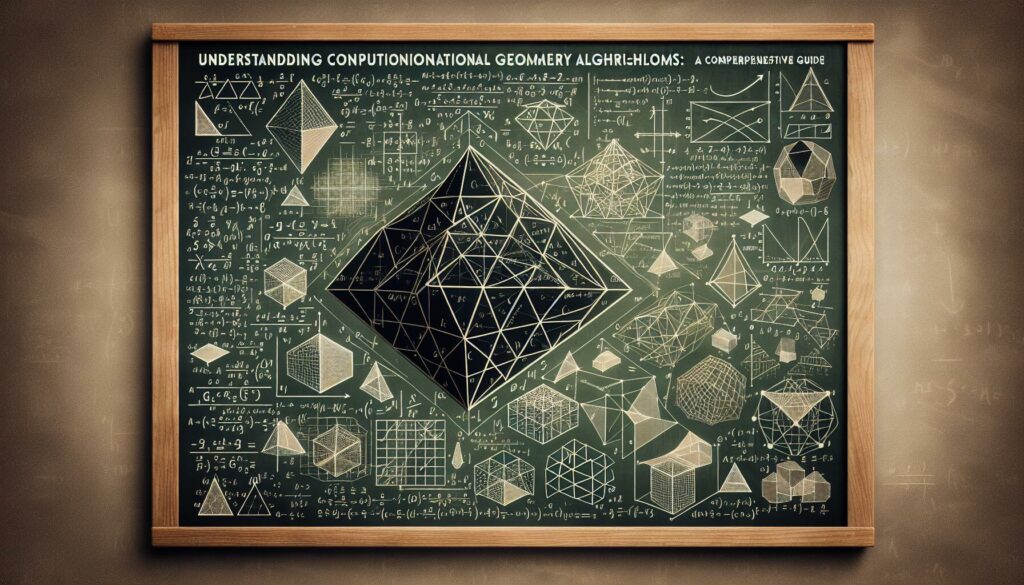
Computational geometry is a fascinating branch of computer science that deals with the study of algorithms for solving geometric problems. It plays a crucial role in various fields, including computer graphics, robotics, geographic information systems (GIS), and computer-aided design (CAD). In this comprehensive guide, we’ll explore the fundamental concepts and algorithms in computational geometry, providing you with a solid foundation to tackle complex geometric problems in your coding projects.
Table of Contents
- Introduction to Computational Geometry
- Basic Concepts in Computational Geometry
- Convex Hull Algorithms
- Line Segment Intersection
- Polygon Triangulation
- Voronoi Diagrams
- Delaunay Triangulation
- Point Location
- Range Searching
- Applications of Computational Geometry
- Conclusion
1. Introduction to Computational Geometry
Computational geometry is the study of algorithms and data structures for solving geometric problems efficiently. It combines elements of pure mathematics, computer science, and computational complexity theory to develop efficient solutions for geometric queries and manipulations.
The field emerged in the 1970s as computer graphics and computer-aided design became more prevalent. Today, computational geometry algorithms are essential in various applications, from video game development to autonomous vehicle navigation.
2. Basic Concepts in Computational Geometry
Before diving into specific algorithms, it’s important to understand some fundamental concepts in computational geometry:
2.1 Points and Vectors
Points are the most basic geometric objects, typically represented by their coordinates in a Cartesian plane. Vectors are directed line segments, often used to represent directions or displacements between points.
2.2 Lines and Line Segments
Lines are infinite straight paths in a plane, while line segments are finite portions of a line with defined endpoints.
2.3 Polygons
Polygons are closed shapes formed by connecting a series of points (vertices) with straight line segments (edges). Common types include triangles, rectangles, and convex polygons.
2.4 Convexity
A shape is convex if, for any two points within the shape, the line segment connecting them is entirely contained within the shape. This property is crucial in many computational geometry algorithms.
3. Convex Hull Algorithms
The convex hull of a set of points is the smallest convex polygon that encloses all the points. It’s a fundamental concept in computational geometry with various applications.
3.1 Graham Scan Algorithm
The Graham Scan algorithm is an efficient method for computing the convex hull of a set of points in 2D space. It works by first finding the point with the lowest y-coordinate (and leftmost if there’s a tie), then sorting the remaining points by polar angle with respect to this point.
Here’s a Python implementation of the Graham Scan algorithm:
import math
def orientation(p, q, r):
return (q[1] - p[1]) * (r[0] - q[0]) - (q[0] - p[0]) * (r[1] - q[1])
def graham_scan(points):
# Find the bottommost point (and leftmost if there's a tie)
bottom_point = min(points, key=lambda p: (p[1], p[0]))
# Sort points based on polar angle with respect to bottom_point
sorted_points = sorted(points, key=lambda p: (math.atan2(p[1] - bottom_point[1], p[0] - bottom_point[0]), p))
stack = [bottom_point, sorted_points[0]]
for point in sorted_points[1:]:
while len(stack) > 1 and orientation(stack[-2], stack[-1], point) <= 0:
stack.pop()
stack.append(point)
return stack
# Example usage
points = [(0, 0), (1, 1), (2, 2), (4, 4), (0, 2), (1, 2), (3, 1), (3, 3)]
convex_hull = graham_scan(points)
print("Convex Hull:", convex_hull)
3.2 Jarvis March (Gift Wrapping) Algorithm
The Jarvis March algorithm, also known as the Gift Wrapping algorithm, is another method for computing the convex hull. It works by iteratively selecting the next point on the hull by finding the point with the smallest right turn.
Here’s a Python implementation of the Jarvis March algorithm:
def orientation(p, q, r):
return (q[1] - p[1]) * (r[0] - q[0]) - (q[0] - p[0]) * (r[1] - q[1])
def jarvis_march(points):
n = len(points)
if n < 3:
return points
hull = []
# Find the leftmost point
l = min(range(n), key=lambda i: points[i][0])
p = l
while True:
hull.append(points[p])
q = (p + 1) % n
for i in range(n):
if orientation(points[p], points[i], points[q]) < 0:
q = i
p = q
if p == l:
break
return hull
# Example usage
points = [(0, 0), (1, 1), (2, 2), (4, 4), (0, 2), (1, 2), (3, 1), (3, 3)]
convex_hull = jarvis_march(points)
print("Convex Hull:", convex_hull)
4. Line Segment Intersection
Detecting intersections between line segments is a common problem in computational geometry. The Bentley-Ottmann algorithm is an efficient sweep line algorithm for finding all intersections among a set of line segments.
Here’s a simplified implementation of line segment intersection detection:
def orientation(p, q, r):
return (q[1] - p[1]) * (r[0] - q[0]) - (q[0] - p[0]) * (r[1] - q[1])
def on_segment(p, q, r):
return (min(p[0], r[0]) <= q[0] <= max(p[0], r[0]) and
min(p[1], r[1]) <= q[1] <= max(p[1], r[1]))
def intersect(seg1, seg2):
p1, q1 = seg1
p2, q2 = seg2
o1 = orientation(p1, q1, p2)
o2 = orientation(p1, q1, q2)
o3 = orientation(p2, q2, p1)
o4 = orientation(p2, q2, q1)
if o1 != o2 and o3 != o4:
return True
if o1 == 0 and on_segment(p1, p2, q1):
return True
if o2 == 0 and on_segment(p1, q2, q1):
return True
if o3 == 0 and on_segment(p2, p1, q2):
return True
if o4 == 0 and on_segment(p2, q1, q2):
return True
return False
# Example usage
seg1 = ((0, 0), (10, 10))
seg2 = ((0, 10), (10, 0))
print("Segments intersect:", intersect(seg1, seg2))
5. Polygon Triangulation
Polygon triangulation is the process of dividing a polygon into a set of triangles. This is useful in computer graphics for rendering complex shapes and in computational geometry for various algorithms.
One popular algorithm for polygon triangulation is the Ear Clipping method:
def is_ear(polygon, i, n):
prev = (i - 1) % n
next = (i + 1) % n
if orientation(polygon[prev], polygon[i], polygon[next]) >= 0:
return False
for j in range(n):
if j == prev or j == i or j == next:
continue
if point_in_triangle(polygon[j], polygon[prev], polygon[i], polygon[next]):
return False
return True
def ear_clipping(polygon):
n = len(polygon)
if n < 3:
return []
triangles = []
ears = [is_ear(polygon, i, n) for i in range(n)]
while n > 3:
for i in range(n):
if ears[i]:
prev = (i - 1) % n
next = (i + 1) % n
triangles.append((polygon[prev], polygon[i], polygon[next]))
polygon.pop(i)
n -= 1
if n > 3:
ears[(i - 1) % n] = is_ear(polygon, (i - 1) % n, n)
ears[i % n] = is_ear(polygon, i % n, n)
break
triangles.append(tuple(polygon))
return triangles
# Helper functions
def orientation(p, q, r):
return (q[1] - p[1]) * (r[0] - q[0]) - (q[0] - p[0]) * (r[1] - q[1])
def point_in_triangle(p, a, b, c):
def sign(p1, p2, p3):
return (p1[0] - p3[0]) * (p2[1] - p3[1]) - (p2[0] - p3[0]) * (p1[1] - p3[1])
d1 = sign(p, a, b)
d2 = sign(p, b, c)
d3 = sign(p, c, a)
has_neg = (d1 < 0) or (d2 < 0) or (d3 < 0)
has_pos = (d1 > 0) or (d2 > 0) or (d3 > 0)
return not (has_neg and has_pos)
# Example usage
polygon = [(0, 0), (2, 0), (2, 2), (1, 1), (0, 2)]
triangles = ear_clipping(polygon)
print("Triangulation:", triangles)
6. Voronoi Diagrams
A Voronoi diagram is a partitioning of a plane into regions based on distance to a specific set of points. For each point, there is a corresponding region consisting of all points closer to that point than to any other.
While implementing an efficient algorithm for Voronoi diagrams is complex, we can create a simple approximation using a grid-based approach:
import numpy as np
import matplotlib.pyplot as plt
def simple_voronoi(points, width, height, resolution=100):
x = np.linspace(0, width, resolution)
y = np.linspace(0, height, resolution)
xx, yy = np.meshgrid(x, y)
voronoi = np.zeros((resolution, resolution), dtype=int)
for i in range(resolution):
for j in range(resolution):
distances = [((xx[i, j] - p[0])**2 + (yy[i, j] - p[1])**2) for p in points]
voronoi[i, j] = np.argmin(distances)
return voronoi
# Example usage
points = [(1, 1), (2, 4), (3, 2), (4, 3)]
width, height = 5, 5
voronoi = simple_voronoi(points, width, height)
plt.imshow(voronoi, cmap='viridis', extent=[0, width, 0, height])
plt.scatter(*zip(*points), c='red')
plt.title("Approximate Voronoi Diagram")
plt.show()
7. Delaunay Triangulation
Delaunay triangulation is the dual of the Voronoi diagram and has the property that no point in the point set falls inside the circumcircle of any triangle in the triangulation. It’s widely used in computer graphics and terrain modeling.
Here’s a simple implementation of the Bowyer-Watson algorithm for Delaunay triangulation:
import math
class Triangle:
def __init__(self, p1, p2, p3):
self.vertices = [p1, p2, p3]
self.calculate_circumcircle()
def calculate_circumcircle(self):
x1, y1 = self.vertices[0]
x2, y2 = self.vertices[1]
x3, y3 = self.vertices[2]
D = 2 * (x1 * (y2 - y3) + x2 * (y3 - y1) + x3 * (y1 - y2))
self.circumcenter = (
((x1*x1 + y1*y1) * (y2 - y3) + (x2*x2 + y2*y2) * (y3 - y1) + (x3*x3 + y3*y3) * (y1 - y2)) / D,
((x1*x1 + y1*y1) * (x3 - x2) + (x2*x2 + y2*y2) * (x1 - x3) + (x3*x3 + y3*y3) * (x2 - x1)) / D
)
self.circumradius = math.sqrt((self.circumcenter[0] - x1)**2 + (self.circumcenter[1] - y1)**2)
def contains_point(self, point):
return math.sqrt((point[0] - self.circumcenter[0])**2 + (point[1] - self.circumcenter[1])**2) <= self.circumradius
def bowyer_watson(points):
# Create a super-triangle that contains all points
min_x = min(p[0] for p in points)
max_x = max(p[0] for p in points)
min_y = min(p[1] for p in points)
max_y = max(p[1] for p in points)
dx = max_x - min_x
dy = max_y - min_y
dmax = max(dx, dy)
mid_x = (min_x + max_x) / 2
mid_y = (min_y + max_y) / 2
p1 = (mid_x - 20 * dmax, mid_y - dmax)
p2 = (mid_x, mid_y + 20 * dmax)
p3 = (mid_x + 20 * dmax, mid_y - dmax)
triangulation = [Triangle(p1, p2, p3)]
for point in points:
bad_triangles = []
for triangle in triangulation:
if triangle.contains_point(point):
bad_triangles.append(triangle)
polygon = []
for triangle in bad_triangles:
for i in range(3):
edge = (triangle.vertices[i], triangle.vertices[(i+1)%3])
if sum(1 for t in bad_triangles if edge[1] in t.vertices and edge[0] in t.vertices) == 1:
polygon.append(edge)
for triangle in bad_triangles:
triangulation.remove(triangle)
for edge in polygon:
triangulation.append(Triangle(point, edge[0], edge[1]))
# Remove triangles that share vertices with the super-triangle
return [t for t in triangulation if not any(v in (p1, p2, p3) for v in t.vertices)]
# Example usage
points = [(0, 0), (1, 0), (0, 1), (1, 1), (0.5, 0.5)]
triangles = bowyer_watson(points)
import matplotlib.pyplot as plt
for triangle in triangles:
plt.plot([v[0] for v in triangle.vertices + [triangle.vertices[0]]],
[v[1] for v in triangle.vertices + [triangle.vertices[0]]], 'b-')
plt.scatter(*zip(*points), c='red')
plt.title("Delaunay Triangulation")
plt.show()
8. Point Location
Point location is the problem of determining which region of a planar subdivision contains a given query point. This is useful in many applications, such as GIS and computer graphics.
Here’s a simple implementation of point location in a triangulation:
def orientation(p, q, r):
return (q[1] - p[1]) * (r[0] - q[0]) - (q[0] - p[0]) * (r[1] - q[1])
def point_in_triangle(p, triangle):
a, b, c = triangle
o1 = orientation(a, b, p)
o2 = orientation(b, c, p)
o3 = orientation(c, a, p)
return (o1 >= 0 and o2 >= 0 and o3 >= 0) or (o1 <= 0 and o2 <= 0 and o3 <= 0)
def point_location(point, triangulation):
for i, triangle in enumerate(triangulation):
if point_in_triangle(point, triangle):
return i
return None
# Example usage
triangulation = [
[(0, 0), (1, 0), (0, 1)],
[(1, 0), (1, 1), (0, 1)],
[(1, 1), (2, 1), (1, 2)],
]
query_point = (0.5, 0.5)
location = point_location(query_point, triangulation)
print(f"Point {query_point} is located in triangle {location}")
9. Range Searching
Range searching involves preprocessing a set of points so that the points lying inside a query range can be reported efficiently. One common data structure for range searching is the k-d tree.
Here’s an implementation of a 2D k-d tree for range searching:
class Node:
def __init__(self, point, left=None, right=None):
self.point = point
self.left = left
self.right = right
def build_kdtree(points, depth=0):
if not points:
return None
k = len(points[0])
axis = depth % k
points.sort(key=lambda x: x[axis])
median = len(points) // 2
return Node(
point=points[median],
left=build_kdtree(points[:median], depth + 1),
right=build_kdtree(points[median + 1:], depth + 1)
)
def range_search(node, range_min, range_max, depth=0, result=None):
if result is None:
result = []
if node is None:
return result
k = len(range_min)
axis = depth % k
if all(range_min[i] <= node.point[i] <= range_max[i] for i in range(k)):
result.append(node.point)
if range_min[axis] <= node.point[axis]:
range_search(node.left, range_min, range_max, depth + 1, result)
if node.point[axis] <= range_max[axis]:
range_search(node.right, range_min, range_max, depth + 1, result)
return result
# Example usage
points = [(2, 3), (5, 4), (9, 6), (4, 7), (8, 1), (7, 2)]
kdtree = build_kdtree(points)
range_min = (3, 2)
range_max = (7, 5)
result = range_search(kdtree, range_min, range_max)
print(f"Points in range {range_min} to {range_max}: {result}")
10. Applications of Computational Geometry
Computational geometry algorithms find applications in various fields:
- Computer Graphics: For rendering 3D scenes, collision detection, and visibility computations.
- Geographic Information Systems (GIS): For spatial analysis, map overlay operations, and terrain modeling.
- Robotics: For path planning, obstacle avoidance, and motion planning.
- Computer-Aided Design (CAD): For designing and modeling complex shapes and structures.
- Medical Imaging: For image segmentation, 3D reconstruction, and surgical planning.
- Virtual Reality and Augmented Reality: For creating immersive environments and realistic interactions.
- Pattern Recognition: For shape analysis and feature extraction in computer vision tasks.
- Molecular Biology: For protein folding simulations and drug design.
11. Conclusion
Computational geometry is a fascinating field that combines mathematical concepts with algorithmic thinking to solve complex geometric problems efficiently. The algorithms and concepts we’ve explored in this guide form the foundation for many advanced applications in computer science and beyond.
As you continue your journey in coding education and programming skills development, remember that mastering these computational geometry algorithms can significantly enhance your problem-solving abilities and open up new possibilities in various domains.
Keep practicing these algorithms, explore their variations, and don’t hesitate to apply them to real-world problems. With time and experience, you’ll develop a strong intuition for geometric problem-solving, which will prove invaluable in your coding career, especially when preparing for technical interviews at major tech companies.