Algorithms for Efficient File Searching: Mastering the Art of Quick Data Retrieval
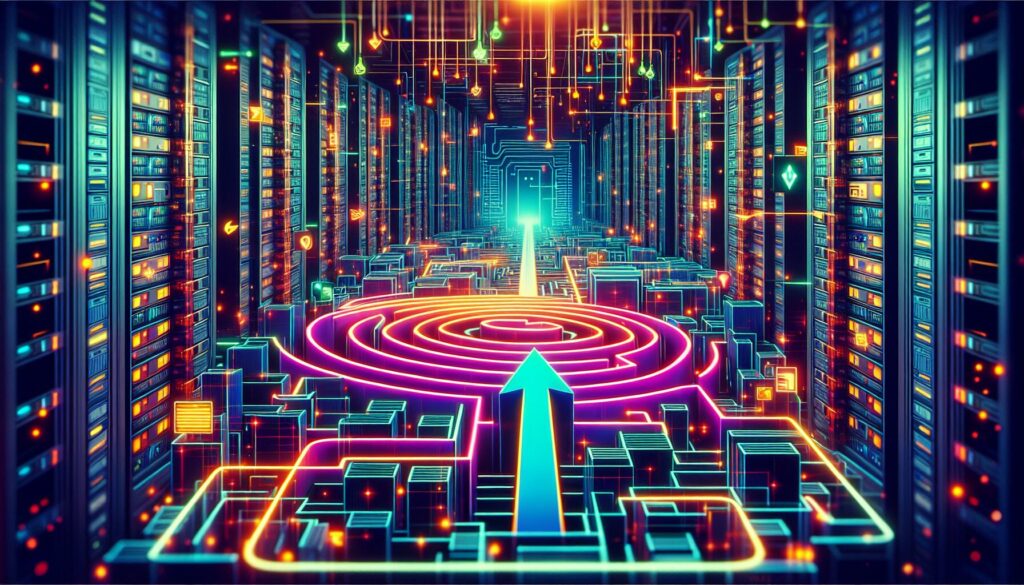
In the vast landscape of computer science and programming, efficient file searching stands as a cornerstone of optimized data retrieval. As the volume of digital information continues to grow exponentially, the need for fast and accurate file searching algorithms becomes increasingly crucial. Whether you’re a budding programmer or an experienced developer preparing for technical interviews at major tech companies, understanding and implementing efficient file searching algorithms is an essential skill.
In this comprehensive guide, we’ll dive deep into the world of file searching algorithms, exploring various techniques, their implementations, and real-world applications. By the end of this article, you’ll have a solid grasp of the fundamental concepts and be well-equipped to tackle file searching challenges in your coding projects and technical interviews.
1. Introduction to File Searching
File searching is the process of locating specific files or data within a computer’s file system or a large dataset. The efficiency of this process is critical in many applications, from simple desktop file explorers to complex database management systems. The primary goal of file searching algorithms is to minimize the time and resources required to find the desired information.
1.1 Importance of Efficient File Searching
Efficient file searching is crucial for several reasons:
- Improved user experience: Fast search results lead to better user satisfaction.
- Resource optimization: Efficient algorithms reduce CPU and memory usage.
- Scalability: As data volumes grow, efficient searching becomes even more critical.
- Time-sensitive applications: In real-time systems, quick data retrieval is essential.
1.2 Key Concepts in File Searching
Before diving into specific algorithms, let’s review some key concepts:
- Search key: The information used to identify the desired file or data.
- Search space: The collection of files or data being searched.
- Search time complexity: The efficiency of the algorithm, often expressed in Big O notation.
- Indexing: Pre-processing data to speed up future searches.
2. Linear Search: The Simplest Approach
Linear search, also known as sequential search, is the most straightforward file searching algorithm. It involves checking each file or data item sequentially until a match is found or the entire search space is exhausted.
2.1 How Linear Search Works
The algorithm follows these steps:
- Start at the beginning of the search space.
- Compare the current item with the search key.
- If a match is found, return the result.
- If no match, move to the next item.
- Repeat steps 2-4 until a match is found or the end of the search space is reached.
2.2 Implementing Linear Search in Python
Here’s a simple implementation of linear search in Python:
def linear_search(file_list, target_file):
for index, file in enumerate(file_list):
if file == target_file:
return index
return -1
# Example usage
files = ['document.txt', 'image.jpg', 'spreadsheet.xlsx', 'presentation.pptx']
result = linear_search(files, 'image.jpg')
print(f"File found at index: {result}")
2.3 Time Complexity Analysis
The time complexity of linear search is O(n), where n is the number of items in the search space. This means that in the worst-case scenario, where the target file is at the end of the list or not present at all, the algorithm will need to examine every item.
2.4 Pros and Cons of Linear Search
Pros:
- Simple to implement and understand
- Works on unsorted data
- Efficient for small datasets
Cons:
- Inefficient for large datasets
- Time complexity increases linearly with the size of the search space
3. Binary Search: Divide and Conquer
Binary search is a more efficient algorithm for searching sorted data. It follows a divide-and-conquer approach, repeatedly dividing the search space in half until the target is found or determined to be absent.
3.1 How Binary Search Works
The algorithm follows these steps:
- Start with the entire sorted search space.
- Compare the target with the middle element.
- If the target matches the middle element, return the result.
- If the target is less than the middle element, repeat the search on the left half.
- If the target is greater than the middle element, repeat the search on the right half.
- Repeat steps 2-5 until the target is found or the search space is empty.
3.2 Implementing Binary Search in Python
Here’s an implementation of binary search in Python:
def binary_search(sorted_files, target_file):
left, right = 0, len(sorted_files) - 1
while left <= right:
mid = (left + right) // 2
if sorted_files[mid] == target_file:
return mid
elif sorted_files[mid] < target_file:
left = mid + 1
else:
right = mid - 1
return -1
# Example usage
sorted_files = ['document.txt', 'image.jpg', 'presentation.pptx', 'spreadsheet.xlsx']
result = binary_search(sorted_files, 'presentation.pptx')
print(f"File found at index: {result}")
3.3 Time Complexity Analysis
The time complexity of binary search is O(log n), where n is the number of items in the search space. This logarithmic time complexity makes binary search significantly more efficient than linear search for large datasets.
3.4 Pros and Cons of Binary Search
Pros:
- Very efficient for large datasets
- Logarithmic time complexity
- Well-suited for sorted data
Cons:
- Requires sorted data
- Not suitable for frequently changing datasets that need to be kept sorted
4. Hashing: Fast Average-Case Searching
Hashing is a powerful technique that can provide constant-time average-case performance for file searching. It involves using a hash function to map file names or keys to specific locations in a hash table.
4.1 How Hashing Works
The hashing process involves these steps:
- Choose a suitable hash function.
- Create a hash table of appropriate size.
- For each file, compute its hash value and store it in the corresponding table location.
- To search, compute the hash value of the target file and check the corresponding table location.
4.2 Implementing a Simple Hash Table in Python
Here’s a basic implementation of a hash table for file searching:
class SimpleHashTable:
def __init__(self, size):
self.size = size
self.table = [[] for _ in range(size)]
def _hash(self, key):
return sum(ord(c) for c in key) % self.size
def insert(self, file_name):
index = self._hash(file_name)
self.table[index].append(file_name)
def search(self, file_name):
index = self._hash(file_name)
return file_name in self.table[index]
# Example usage
hash_table = SimpleHashTable(10)
files = ['document.txt', 'image.jpg', 'spreadsheet.xlsx', 'presentation.pptx']
for file in files:
hash_table.insert(file)
print(hash_table.search('image.jpg')) # True
print(hash_table.search('video.mp4')) # False
4.3 Time Complexity Analysis
The average-case time complexity for searching in a well-designed hash table is O(1), or constant time. However, the worst-case scenario (when many items hash to the same location) can be O(n), where n is the number of items in the table.
4.4 Pros and Cons of Hashing
Pros:
- Very fast average-case performance
- Efficient for both small and large datasets
- Can handle dynamic data efficiently
Cons:
- Requires additional space for the hash table
- Performance can degrade with poor hash functions or high collision rates
- Complex to implement correctly
5. Trie: Efficient Prefix Searching
A trie, also known as a prefix tree, is a tree-based data structure that is particularly efficient for searching strings with common prefixes. This makes it an excellent choice for file systems where files often share common name prefixes.
5.1 How Trie Works
A trie organizes data as follows:
- The root represents an empty string.
- Each node stores a character and has branches for subsequent characters.
- Paths from the root to leaf nodes represent complete strings (file names).
- Searching involves traversing the trie based on the characters of the search key.
5.2 Implementing a Trie in Python
Here’s a basic implementation of a trie for file searching:
class TrieNode:
def __init__(self):
self.children = {}
self.is_end_of_word = False
class Trie:
def __init__(self):
self.root = TrieNode()
def insert(self, word):
node = self.root
for char in word:
if char not in node.children:
node.children[char] = TrieNode()
node = node.children[char]
node.is_end_of_word = True
def search(self, word):
node = self.root
for char in word:
if char not in node.children:
return False
node = node.children[char]
return node.is_end_of_word
def starts_with(self, prefix):
node = self.root
for char in prefix:
if char not in node.children:
return False
node = node.children[char]
return True
# Example usage
trie = Trie()
files = ['document.txt', 'doc.pdf', 'image.jpg', 'img.png']
for file in files:
trie.insert(file)
print(trie.search('document.txt')) # True
print(trie.search('video.mp4')) # False
print(trie.starts_with('doc')) # True
print(trie.starts_with('vid')) # False
5.3 Time Complexity Analysis
The time complexity for searching in a trie is O(m), where m is the length of the search string. This makes tries particularly efficient for prefix-based searches and auto-completion features.
5.4 Pros and Cons of Trie
Pros:
- Efficient for prefix-based searches
- Supports fast auto-completion and wildcard searches
- Time complexity is independent of the number of strings stored
Cons:
- Can be memory-intensive, especially for large datasets
- Not as efficient for exact string matching compared to hash tables
- Implementation can be more complex than other data structures
6. Indexed Searching: Boosting Performance with Preprocessing
Indexed searching involves creating and maintaining auxiliary data structures (indexes) to speed up search operations. This approach is widely used in databases and large-scale file systems.
6.1 How Indexed Searching Works
The process of indexed searching typically involves:
- Creating an index: Preprocessing the data to build a search-efficient structure.
- Maintaining the index: Updating the index as files are added, modified, or deleted.
- Searching: Using the index to quickly locate files matching the search criteria.
6.2 Implementing a Simple Inverted Index in Python
Here’s a basic implementation of an inverted index for file content searching:
from collections import defaultdict
class InvertedIndex:
def __init__(self):
self.index = defaultdict(set)
def add_document(self, doc_id, content):
words = content.lower().split()
for word in words:
self.index[word].add(doc_id)
def search(self, query):
words = query.lower().split()
if not words:
return set()
result = self.index[words[0]]
for word in words[1:]:
result = result.intersection(self.index[word])
return result
# Example usage
index = InvertedIndex()
documents = {
1: "The quick brown fox",
2: "jumps over the lazy dog",
3: "The lazy dog sleeps"
}
for doc_id, content in documents.items():
index.add_document(doc_id, content)
print(index.search("quick fox")) # {1}
print(index.search("lazy")) # {2, 3}
print(index.search("cat")) # set()
6.3 Time Complexity Analysis
The time complexity for searching using an inverted index can vary depending on the implementation and query complexity. In general, it can achieve sub-linear time complexity, often approaching O(1) for simple queries.
6.4 Pros and Cons of Indexed Searching
Pros:
- Very fast search performance, especially for complex queries
- Supports advanced search features like full-text search and relevance ranking
- Scalable to very large datasets
Cons:
- Requires additional storage space for indexes
- Index maintenance can be computationally expensive
- Complex to implement and optimize for large-scale systems
7. Choosing the Right Algorithm for Your Use Case
Selecting the most appropriate file searching algorithm depends on various factors:
- Data size: For small datasets, simple algorithms like linear search may suffice. For larger datasets, more advanced techniques are necessary.
- Data structure: Is the data sorted? Can it be easily sorted? This affects the viability of algorithms like binary search.
- Search frequency: If searches are frequent, investing in preprocessing (like creating indexes) may be worthwhile.
- Update frequency: How often is the data modified? This impacts the maintenance overhead of complex data structures.
- Memory constraints: Some algorithms, like tries, can be memory-intensive and may not be suitable for memory-constrained environments.
- Search type: Are you performing exact matches, prefix searches, or full-text searches? Different algorithms excel at different types of searches.
8. Real-World Applications and Optimizations
File searching algorithms find applications in various domains:
- Operating Systems: File explorers and search utilities use optimized file searching algorithms.
- Databases: Database management systems employ sophisticated indexing and searching techniques.
- Search Engines: Web search engines use highly optimized algorithms for fast and relevant results.
- Version Control Systems: Git and other VCS use efficient algorithms to search through file histories.
- Content Management Systems: CMS platforms often implement advanced search capabilities.
In real-world scenarios, these algorithms are often combined and optimized:
- Hybrid approaches: Combining multiple algorithms to leverage their strengths.
- Parallelization: Utilizing multi-core processors to speed up search operations.
- Caching: Storing frequently accessed search results to reduce computation.
- Compression: Using data compression techniques to reduce storage and improve search speed.
- Machine Learning: Employing ML models to predict and optimize search patterns.
9. Preparing for Technical Interviews
When preparing for technical interviews, especially for major tech companies, consider the following:
- Understand the fundamentals: Be able to explain and implement basic search algorithms.
- Analyze trade-offs: Discuss the pros and cons of different approaches for various scenarios.
- Optimize: Practice optimizing algorithms for specific constraints (e.g., memory limitations).
- Handle edge cases: Consider and address edge cases in your implementations.
- Scale considerations: Discuss how your solutions would scale to very large datasets.
- Real-world applications: Be prepared to relate these algorithms to practical use cases.
10. Conclusion
Mastering efficient file searching algorithms is crucial for any programmer aiming to excel in software development and technical interviews. From the simplicity of linear search to the sophistication of indexed searching, each algorithm offers unique strengths and trade-offs. By understanding these algorithms, their implementations, and their applications, you’ll be well-equipped to tackle a wide range of file searching challenges in your coding projects and career.
Remember, the key to mastery lies not just in knowing these algorithms, but in understanding when and how to apply them effectively. Continue practicing, experimenting with different scenarios, and challenging yourself to optimize your solutions. With dedication and hands-on experience, you’ll develop the skills and intuition needed to excel in file searching tasks and beyond.
Happy coding, and may your searches always be swift and accurate!