The Role of Algebra in Algorithm Development: Bridging Mathematics and Computer Science
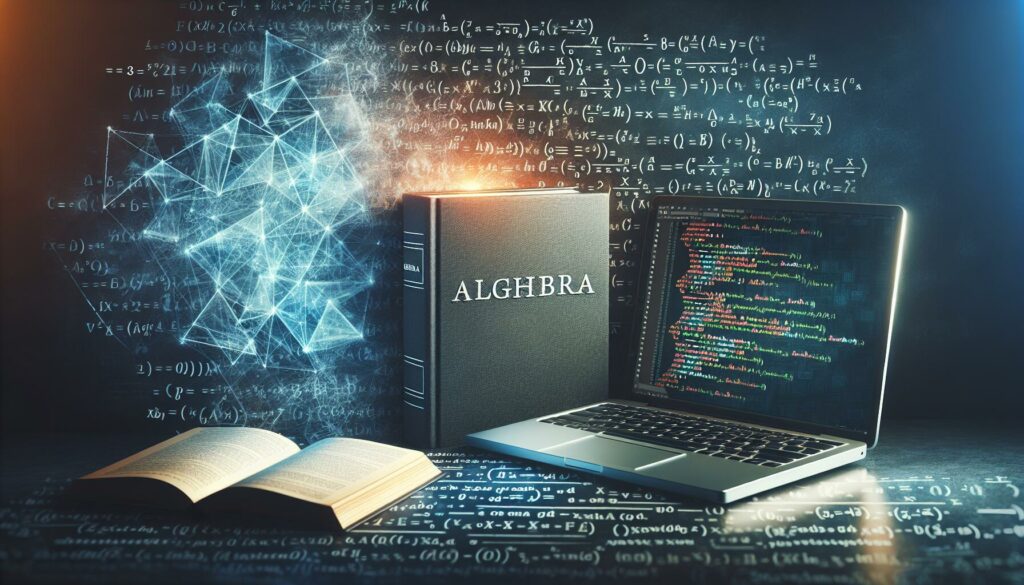
In the ever-evolving world of computer science and programming, the importance of strong mathematical foundations cannot be overstated. Among the various branches of mathematics, algebra plays a pivotal role in algorithm development and analysis. This article delves deep into the intricate relationship between algebra and algorithms, exploring how algebraic concepts contribute to the creation, optimization, and understanding of computational processes.
Understanding the Basics: What is Algebra?
Before we dive into the connection between algebra and algorithms, let’s briefly refresh our understanding of algebra. Algebra is a branch of mathematics that deals with symbols and the rules for manipulating these symbols. It is a way of expressing mathematical relationships and solving problems using variables and equations.
Key concepts in algebra include:
- Variables and constants
- Equations and inequalities
- Functions and their properties
- Algebraic structures (groups, rings, fields)
- Polynomials and their operations
These fundamental concepts form the backbone of many computational processes and are essential in algorithm development.
The Intersection of Algebra and Algorithms
Algorithms are step-by-step procedures for solving problems or performing tasks. They are the building blocks of computer programs and are essential in software development. The connection between algebra and algorithms is profound and multifaceted:
1. Problem Formulation
Algebraic thinking helps in formulating computational problems. By expressing problems in terms of variables, equations, and functions, we can create abstract representations that are easier to manipulate and solve programmatically.
2. Algorithm Design
Many algorithms rely on algebraic concepts for their design. For example, linear algebra is crucial in developing algorithms for machine learning and computer graphics.
3. Complexity Analysis
Algebraic expressions are used to describe the time and space complexity of algorithms, helping developers understand and optimize their code’s performance.
4. Data Structures
Algebraic structures like groups and rings often inspire the design of efficient data structures, such as hash tables and balanced trees.
5. Optimization
Algebraic techniques are employed in algorithm optimization, helping to simplify expressions and improve efficiency.
Algebraic Concepts in Common Algorithms
Let’s explore how specific algebraic concepts manifest in some well-known algorithms:
Linear Algebra in Matrix Operations
Matrix operations are fundamental in many algorithms, especially in areas like computer graphics, machine learning, and scientific computing. Linear algebra provides the theoretical foundation for these operations.
For example, consider the problem of rotating a 2D point (x, y) by an angle θ. This can be achieved using matrix multiplication:
[x'] [cos θ -sin θ] [x]
[y'] = [sin θ cos θ] [y]
In code, this might look like:
def rotate_point(x, y, theta):
cos_theta = math.cos(theta)
sin_theta = math.sin(theta)
x_new = x * cos_theta - y * sin_theta
y_new = x * sin_theta + y * cos_theta
return x_new, y_new
Polynomial Algebra in Cryptography
Polynomial algebra plays a crucial role in many cryptographic algorithms. For instance, the RSA algorithm, widely used for secure data transmission, relies on properties of polynomials and modular arithmetic.
The core of RSA involves operations like:
c = m^e mod n (encryption)
m = c^d mod n (decryption)
Where m is the message, c is the ciphertext, e and d are the public and private exponents, and n is the modulus.
Graph Theory and Abstract Algebra
Graph algorithms often leverage concepts from abstract algebra. For example, the Floyd-Warshall algorithm for finding shortest paths in a weighted graph can be viewed as operations on a semiring algebraic structure.
The core of the Floyd-Warshall algorithm can be expressed as:
for k in range(n):
for i in range(n):
for j in range(n):
dist[i][j] = min(dist[i][j], dist[i][k] + dist[k][j])
This triple nested loop structure mirrors the algebraic operation of matrix multiplication over the semiring (min, +).
Algebraic Thinking in Algorithm Development
Incorporating algebraic thinking into algorithm development can lead to more elegant and efficient solutions. Here are some ways algebra influences algorithm design:
1. Problem Abstraction
Algebra allows us to abstract away the specifics of a problem and focus on its underlying structure. This abstraction can lead to more general and reusable algorithms.
2. Pattern Recognition
Algebraic thinking helps in recognizing patterns in data and processes, which is crucial for developing efficient algorithms. For example, recognizing arithmetic or geometric progressions can lead to optimized solutions for certain problems.
3. Complexity Reduction
Algebraic manipulations can often simplify complex expressions, leading to more efficient algorithms. For instance, simplifying nested loops or reducing the number of operations in a formula can significantly improve an algorithm’s performance.
4. Proof of Correctness
Algebraic methods are often used to prove the correctness of algorithms. Techniques like loop invariants and induction, which have roots in algebra, are essential tools for verifying that an algorithm produces the correct output for all valid inputs.
Case Study: The Fast Fourier Transform (FFT)
The Fast Fourier Transform (FFT) is a perfect example of how algebraic thinking can lead to revolutionary algorithms. The FFT is an algorithm for computing the Discrete Fourier Transform (DFT) of a sequence, or its inverse. It’s widely used in signal processing and data analysis.
The naive approach to computing the DFT has a time complexity of O(n^2) for a sequence of length n. However, by leveraging algebraic properties of complex roots of unity, the FFT achieves a time complexity of O(n log n).
The key insight of the FFT comes from the algebraic manipulation of the DFT formula:
X[k] = Σ(n-1, j=0) x[j] * e^(-2πijk/N)
By recognizing that e^(-2Ï€i/N) is an Nth root of unity and exploiting its properties, the algorithm can recursively break down the problem into smaller subproblems, dramatically reducing the number of computations required.
Algebraic Structures in Data Structures
Algebraic structures not only influence algorithm design but also play a crucial role in the development of efficient data structures. Understanding these structures can lead to more effective use of existing data structures and the creation of new ones.
1. Groups and Hash Tables
The concept of groups from abstract algebra is fundamental to the design of hash tables. A good hash function should distribute keys uniformly across the table, which is analogous to the group theory concept of cosets partitioning a group.
For example, a simple hash function for strings might look like:
def hash_string(s, table_size):
hash_value = 0
for char in s:
hash_value = (hash_value * 31 + ord(char)) % table_size
return hash_value
This function uses properties of modular arithmetic (a concept from group theory) to ensure the hash value falls within the table size.
2. Rings and Polynomial Hash Functions
Ring theory, particularly polynomial rings, is used in designing more advanced hash functions. Polynomial hash functions are especially useful in string matching algorithms like Rabin-Karp.
A polynomial hash function might look like:
def poly_hash(s, p, m):
hash_value = 0
for char in reversed(s):
hash_value = (hash_value * p + ord(char)) % m
return hash_value
Here, p is a prime number, and m is the modulus. This function treats the string as coefficients of a polynomial, evaluated at p modulo m.
3. Lattices and Binary Search Trees
The concept of lattices from order theory (a branch of abstract algebra) is closely related to the structure of binary search trees. The partial ordering of elements in a BST forms a lattice structure.
Understanding this relationship can lead to insights in tree balancing algorithms and operations. For instance, the rotation operation in self-balancing trees like AVL or Red-Black trees can be viewed as preserving the lattice structure while changing the tree’s shape.
Algebraic Optimization Techniques
Algebra provides powerful tools for optimizing algorithms. Here are some common optimization techniques rooted in algebraic thinking:
1. Algebraic Simplification
Often, complex mathematical expressions in algorithms can be simplified using algebraic rules. This can lead to fewer operations and improved efficiency.
For example, consider the quadratic formula:
x = (-b ± √(b^2 - 4ac)) / (2a)
In code, a naive implementation might look like:
def quadratic_formula(a, b, c):
discriminant = math.sqrt(b**2 - 4*a*c)
x1 = (-b + discriminant) / (2*a)
x2 = (-b - discriminant) / (2*a)
return x1, x2
However, this can be algebraically simplified to avoid potential loss of significance:
def quadratic_formula_optimized(a, b, c):
discriminant = math.sqrt(b**2 - 4*a*c)
if b > 0:
x1 = (-b - discriminant) / (2*a)
x2 = (2*c) / (-b - discriminant)
else:
x1 = (2*c) / (-b + discriminant)
x2 = (-b + discriminant) / (2*a)
return x1, x2
2. Horner’s Method
Horner’s method is an algorithm for polynomial evaluation that reduces the number of multiplications and additions required. It’s a prime example of algebraic optimization.
For a polynomial a_n * x^n + a_(n-1) * x^(n-1) + … + a_1 * x + a_0, instead of computing each term separately, Horner’s method computes:
(...((a_n * x + a_(n-1)) * x + a_(n-2)) * x + ... + a_1) * x + a_0
This reduces the number of multiplications from O(n^2) to O(n). In code:
def horner_method(coefficients, x):
result = 0
for coefficient in reversed(coefficients):
result = result * x + coefficient
return result
3. Fast Exponentiation
The fast exponentiation algorithm, also known as exponentiation by squaring, is another example of algebraic optimization. It reduces the number of multiplications needed to compute x^n from O(n) to O(log n).
The algorithm is based on the observation that:
x^n = (x^2)^(n/2) if n is even
x^n = x * (x^2)^((n-1)/2) if n is odd
Implemented recursively:
def fast_exponentiation(x, n):
if n == 0:
return 1
elif n % 2 == 0:
half_pow = fast_exponentiation(x, n // 2)
return half_pow * half_pow
else:
return x * fast_exponentiation(x, n - 1)
Algebra in Algorithm Analysis
Algebra is not only crucial in algorithm development but also plays a significant role in algorithm analysis. Here are some ways algebraic techniques are used to analyze algorithms:
1. Big O Notation
The Big O notation, used to describe the upper bound of an algorithm’s time or space complexity, is fundamentally an algebraic concept. It involves analyzing the growth rate of functions and comparing them using algebraic properties.
For example, proving that n^2 + 3n + 1 is O(n^2) involves showing that there exist constants c and n_0 such that:
n^2 + 3n + 1 ≤ c * n^2 for all n ≥ n_0
This proof relies on algebraic manipulation and inequalities.
2. Recurrence Relations
Many algorithms, especially recursive ones, are analyzed using recurrence relations. Solving these relations often requires algebraic techniques like the master theorem or substitution method.
For instance, the recurrence relation for the time complexity of Merge Sort is:
T(n) = 2T(n/2) + O(n)
Solving this using the master theorem involves algebraic comparisons of logarithmic functions.
3. Amortized Analysis
Amortized analysis, used to analyze the time complexity of a sequence of operations, often involves algebraic techniques like the potential method. This method assigns a potential function to the data structure and uses algebraic manipulations to prove bounds on the amortized cost of operations.
Challenges and Future Directions
While algebra has been instrumental in algorithm development, there are still challenges and areas for future research:
1. Quantum Algorithms
As quantum computing advances, new algebraic structures and techniques are needed to develop and analyze quantum algorithms. The field of quantum algebra is emerging to meet this challenge.
2. Machine Learning Algorithms
The increasing complexity of machine learning algorithms, especially in deep learning, requires more sophisticated algebraic tools for analysis and optimization. Tensor algebra, for instance, is becoming increasingly important in this field.
3. Algebraic Complexity Theory
This field aims to classify computational problems based on the algebraic operations needed to solve them. Advances in this area could lead to new lower bounds on algorithm complexity and potentially new algorithmic techniques.
4. Automated Algorithm Design
As AI advances, there’s potential for automated algorithm design using algebraic principles. This could lead to the discovery of novel algorithms that humans might not have conceived.
Conclusion
The role of algebra in algorithm development is profound and multifaceted. From problem formulation to algorithm design, optimization, and analysis, algebraic thinking permeates every aspect of computational problem-solving. As we face increasingly complex computational challenges, the synergy between algebra and algorithms will continue to drive innovation in computer science.
For aspiring programmers and computer scientists, a strong foundation in algebra is not just beneficial—it’s essential. It provides the tools to think abstractly about problems, recognize patterns, and develop efficient solutions. As you continue your journey in coding and algorithm development, remember that behind every elegant algorithm lies a beautiful algebraic structure waiting to be discovered and exploited.
The interplay between algebra and algorithms is a testament to the deep connection between mathematics and computer science. It reminds us that at the heart of computation lies the elegant language of mathematics, forever shaping the way we solve problems in the digital age.